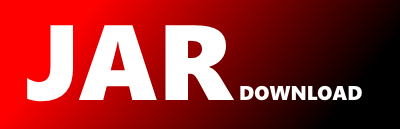
main.com.wire.crypto.CoreCrypto.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core-crypto-jvm Show documentation
Show all versions of core-crypto-jvm Show documentation
MLS/Proteus multiplexer abstraction with encrypted persistent storage in Rust
// This file was autogenerated by some hot garbage in the `uniffi` crate.
// Trust me, you don't want to mess with it!
@file:Suppress("NAME_SHADOWING")
package com.wire.crypto
// Common helper code.
//
// Ideally this would live in a separate .kt file where it can be unittested etc
// in isolation, and perhaps even published as a re-useable package.
//
// However, it's important that the details of how this helper code works (e.g. the
// way that different builtin types are passed across the FFI) exactly match what's
// expected by the Rust code on the other side of the interface. In practice right
// now that means coming from the exact some version of `uniffi` that was used to
// compile the Rust component. The easiest way to ensure this is to bundle the Kotlin
// helpers directly inline like we're doing here.
import com.sun.jna.Library
import com.sun.jna.IntegerType
import com.sun.jna.Native
import com.sun.jna.Pointer
import com.sun.jna.Structure
import com.sun.jna.Callback
import com.sun.jna.ptr.*
import java.nio.ByteBuffer
import java.nio.ByteOrder
import java.nio.CharBuffer
import java.nio.charset.CodingErrorAction
import java.util.concurrent.atomic.AtomicLong
import java.util.concurrent.ConcurrentHashMap
import java.util.concurrent.atomic.AtomicBoolean
import kotlin.coroutines.resume
import kotlinx.coroutines.CancellableContinuation
import kotlinx.coroutines.DelicateCoroutinesApi
import kotlinx.coroutines.GlobalScope
import kotlinx.coroutines.Job
import kotlinx.coroutines.launch
import kotlinx.coroutines.suspendCancellableCoroutine
import uniffi.core_crypto.CryptoError
import uniffi.core_crypto.E2eIdentityError
import uniffi.core_crypto.FfiConverterTypeCryptoError
import uniffi.core_crypto.FfiConverterTypeE2eIdentityError
import uniffi.core_crypto.RustBuffer as RustBufferCryptoError
import uniffi.core_crypto.RustBuffer as RustBufferE2eIdentityError
// This is a helper for safely working with byte buffers returned from the Rust code.
// A rust-owned buffer is represented by its capacity, its current length, and a
// pointer to the underlying data.
@Structure.FieldOrder("capacity", "len", "data")
open class RustBuffer : Structure() {
// Note: `capacity` and `len` are actually `ULong` values, but JVM only supports signed values.
// When dealing with these fields, make sure to call `toULong()`.
@JvmField var capacity: Long = 0
@JvmField var len: Long = 0
@JvmField var data: Pointer? = null
class ByValue: RustBuffer(), Structure.ByValue
class ByReference: RustBuffer(), Structure.ByReference
internal fun setValue(other: RustBuffer) {
capacity = other.capacity
len = other.len
data = other.data
}
companion object {
internal fun alloc(size: ULong = 0UL) = uniffiRustCall() { status ->
// Note: need to convert the size to a `Long` value to make this work with JVM.
UniffiLib.INSTANCE.ffi_core_crypto_ffi_rustbuffer_alloc(size.toLong(), status)
}.also {
if(it.data == null) {
throw RuntimeException("RustBuffer.alloc() returned null data pointer (size=${size})")
}
}
internal fun create(capacity: ULong, len: ULong, data: Pointer?): RustBuffer.ByValue {
var buf = RustBuffer.ByValue()
buf.capacity = capacity.toLong()
buf.len = len.toLong()
buf.data = data
return buf
}
internal fun free(buf: RustBuffer.ByValue) = uniffiRustCall() { status ->
UniffiLib.INSTANCE.ffi_core_crypto_ffi_rustbuffer_free(buf, status)
}
}
@Suppress("TooGenericExceptionThrown")
fun asByteBuffer() =
this.data?.getByteBuffer(0, this.len.toLong())?.also {
it.order(ByteOrder.BIG_ENDIAN)
}
}
/**
* The equivalent of the `*mut RustBuffer` type.
* Required for callbacks taking in an out pointer.
*
* Size is the sum of all values in the struct.
*/
class RustBufferByReference : ByReference(16) {
/**
* Set the pointed-to `RustBuffer` to the given value.
*/
fun setValue(value: RustBuffer.ByValue) {
// NOTE: The offsets are as they are in the C-like struct.
val pointer = getPointer()
pointer.setLong(0, value.capacity)
pointer.setLong(8, value.len)
pointer.setPointer(16, value.data)
}
/**
* Get a `RustBuffer.ByValue` from this reference.
*/
fun getValue(): RustBuffer.ByValue {
val pointer = getPointer()
val value = RustBuffer.ByValue()
value.writeField("capacity", pointer.getLong(0))
value.writeField("len", pointer.getLong(8))
value.writeField("data", pointer.getLong(16))
return value
}
}
// This is a helper for safely passing byte references into the rust code.
// It's not actually used at the moment, because there aren't many things that you
// can take a direct pointer to in the JVM, and if we're going to copy something
// then we might as well copy it into a `RustBuffer`. But it's here for API
// completeness.
@Structure.FieldOrder("len", "data")
open class ForeignBytes : Structure() {
@JvmField var len: Int = 0
@JvmField var data: Pointer? = null
class ByValue : ForeignBytes(), Structure.ByValue
}
// The FfiConverter interface handles converter types to and from the FFI
//
// All implementing objects should be public to support external types. When a
// type is external we need to import it's FfiConverter.
public interface FfiConverter {
// Convert an FFI type to a Kotlin type
fun lift(value: FfiType): KotlinType
// Convert an Kotlin type to an FFI type
fun lower(value: KotlinType): FfiType
// Read a Kotlin type from a `ByteBuffer`
fun read(buf: ByteBuffer): KotlinType
// Calculate bytes to allocate when creating a `RustBuffer`
//
// This must return at least as many bytes as the write() function will
// write. It can return more bytes than needed, for example when writing
// Strings we can't know the exact bytes needed until we the UTF-8
// encoding, so we pessimistically allocate the largest size possible (3
// bytes per codepoint). Allocating extra bytes is not really a big deal
// because the `RustBuffer` is short-lived.
fun allocationSize(value: KotlinType): ULong
// Write a Kotlin type to a `ByteBuffer`
fun write(value: KotlinType, buf: ByteBuffer)
// Lower a value into a `RustBuffer`
//
// This method lowers a value into a `RustBuffer` rather than the normal
// FfiType. It's used by the callback interface code. Callback interface
// returns are always serialized into a `RustBuffer` regardless of their
// normal FFI type.
fun lowerIntoRustBuffer(value: KotlinType): RustBuffer.ByValue {
val rbuf = RustBuffer.alloc(allocationSize(value))
try {
val bbuf = rbuf.data!!.getByteBuffer(0, rbuf.capacity).also {
it.order(ByteOrder.BIG_ENDIAN)
}
write(value, bbuf)
rbuf.writeField("len", bbuf.position().toLong())
return rbuf
} catch (e: Throwable) {
RustBuffer.free(rbuf)
throw e
}
}
// Lift a value from a `RustBuffer`.
//
// This here mostly because of the symmetry with `lowerIntoRustBuffer()`.
// It's currently only used by the `FfiConverterRustBuffer` class below.
fun liftFromRustBuffer(rbuf: RustBuffer.ByValue): KotlinType {
val byteBuf = rbuf.asByteBuffer()!!
try {
val item = read(byteBuf)
if (byteBuf.hasRemaining()) {
throw RuntimeException("junk remaining in buffer after lifting, something is very wrong!!")
}
return item
} finally {
RustBuffer.free(rbuf)
}
}
}
// FfiConverter that uses `RustBuffer` as the FfiType
public interface FfiConverterRustBuffer: FfiConverter {
override fun lift(value: RustBuffer.ByValue) = liftFromRustBuffer(value)
override fun lower(value: KotlinType) = lowerIntoRustBuffer(value)
}
// A handful of classes and functions to support the generated data structures.
// This would be a good candidate for isolating in its own ffi-support lib.
internal const val UNIFFI_CALL_SUCCESS = 0.toByte()
internal const val UNIFFI_CALL_ERROR = 1.toByte()
internal const val UNIFFI_CALL_UNEXPECTED_ERROR = 2.toByte()
@Structure.FieldOrder("code", "error_buf")
internal open class UniffiRustCallStatus : Structure() {
@JvmField var code: Byte = 0
@JvmField var error_buf: RustBuffer.ByValue = RustBuffer.ByValue()
class ByValue: UniffiRustCallStatus(), Structure.ByValue
fun isSuccess(): Boolean {
return code == UNIFFI_CALL_SUCCESS
}
fun isError(): Boolean {
return code == UNIFFI_CALL_ERROR
}
fun isPanic(): Boolean {
return code == UNIFFI_CALL_UNEXPECTED_ERROR
}
companion object {
fun create(code: Byte, errorBuf: RustBuffer.ByValue): UniffiRustCallStatus.ByValue {
val callStatus = UniffiRustCallStatus.ByValue()
callStatus.code = code
callStatus.error_buf = errorBuf
return callStatus
}
}
}
class InternalException(message: String) : kotlin.Exception(message)
// Each top-level error class has a companion object that can lift the error from the call status's rust buffer
interface UniffiRustCallStatusErrorHandler {
fun lift(error_buf: RustBuffer.ByValue): E;
}
// Helpers for calling Rust
// In practice we usually need to be synchronized to call this safely, so it doesn't
// synchronize itself
// Call a rust function that returns a Result<>. Pass in the Error class companion that corresponds to the Err
private inline fun uniffiRustCallWithError(errorHandler: UniffiRustCallStatusErrorHandler, callback: (UniffiRustCallStatus) -> U): U {
var status = UniffiRustCallStatus()
val return_value = callback(status)
uniffiCheckCallStatus(errorHandler, status)
return return_value
}
// Check UniffiRustCallStatus and throw an error if the call wasn't successful
private fun uniffiCheckCallStatus(errorHandler: UniffiRustCallStatusErrorHandler, status: UniffiRustCallStatus) {
if (status.isSuccess()) {
return
} else if (status.isError()) {
throw errorHandler.lift(status.error_buf)
} else if (status.isPanic()) {
// when the rust code sees a panic, it tries to construct a rustbuffer
// with the message. but if that code panics, then it just sends back
// an empty buffer.
if (status.error_buf.len > 0) {
throw InternalException(FfiConverterString.lift(status.error_buf))
} else {
throw InternalException("Rust panic")
}
} else {
throw InternalException("Unknown rust call status: $status.code")
}
}
// UniffiRustCallStatusErrorHandler implementation for times when we don't expect a CALL_ERROR
object UniffiNullRustCallStatusErrorHandler: UniffiRustCallStatusErrorHandler {
override fun lift(error_buf: RustBuffer.ByValue): InternalException {
RustBuffer.free(error_buf)
return InternalException("Unexpected CALL_ERROR")
}
}
// Call a rust function that returns a plain value
private inline fun uniffiRustCall(callback: (UniffiRustCallStatus) -> U): U {
return uniffiRustCallWithError(UniffiNullRustCallStatusErrorHandler, callback)
}
internal inline fun uniffiTraitInterfaceCall(
callStatus: UniffiRustCallStatus,
makeCall: () -> T,
writeReturn: (T) -> Unit,
) {
try {
writeReturn(makeCall())
} catch(e: kotlin.Exception) {
callStatus.code = UNIFFI_CALL_UNEXPECTED_ERROR
callStatus.error_buf = FfiConverterString.lower(e.toString())
}
}
internal inline fun uniffiTraitInterfaceCallWithError(
callStatus: UniffiRustCallStatus,
makeCall: () -> T,
writeReturn: (T) -> Unit,
lowerError: (E) -> RustBuffer.ByValue
) {
try {
writeReturn(makeCall())
} catch(e: kotlin.Exception) {
if (e is E) {
callStatus.code = UNIFFI_CALL_ERROR
callStatus.error_buf = lowerError(e)
} else {
callStatus.code = UNIFFI_CALL_UNEXPECTED_ERROR
callStatus.error_buf = FfiConverterString.lower(e.toString())
}
}
}
// Map handles to objects
//
// This is used pass an opaque 64-bit handle representing a foreign object to the Rust code.
internal class UniffiHandleMap {
private val map = ConcurrentHashMap()
private val counter = java.util.concurrent.atomic.AtomicLong(0)
val size: Int
get() = map.size
// Insert a new object into the handle map and get a handle for it
fun insert(obj: T): Long {
val handle = counter.getAndAdd(1)
map.put(handle, obj)
return handle
}
// Get an object from the handle map
fun get(handle: Long): T {
return map.get(handle) ?: throw InternalException("UniffiHandleMap.get: Invalid handle")
}
// Remove an entry from the handlemap and get the Kotlin object back
fun remove(handle: Long): T {
return map.remove(handle) ?: throw InternalException("UniffiHandleMap: Invalid handle")
}
}
// Contains loading, initialization code,
// and the FFI Function declarations in a com.sun.jna.Library.
@Synchronized
private fun findLibraryName(componentName: String): String {
val libOverride = System.getProperty("uniffi.component.$componentName.libraryOverride")
if (libOverride != null) {
return libOverride
}
return "core_crypto_ffi"
}
private inline fun loadIndirect(
componentName: String
): Lib {
return Native.load(findLibraryName(componentName), Lib::class.java)
}
// Define FFI callback types
internal interface UniffiRustFutureContinuationCallback : com.sun.jna.Callback {
fun callback(`data`: Long,`pollResult`: Byte,)
}
internal interface UniffiForeignFutureFree : com.sun.jna.Callback {
fun callback(`handle`: Long,)
}
internal interface UniffiCallbackInterfaceFree : com.sun.jna.Callback {
fun callback(`handle`: Long,)
}
@Structure.FieldOrder("handle", "free")
internal open class UniffiForeignFuture(
@JvmField internal var `handle`: Long = 0.toLong(),
@JvmField internal var `free`: UniffiForeignFutureFree? = null,
) : Structure() {
class UniffiByValue(
`handle`: Long = 0.toLong(),
`free`: UniffiForeignFutureFree? = null,
): UniffiForeignFuture(`handle`,`free`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFuture) {
`handle` = other.`handle`
`free` = other.`free`
}
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructU8(
@JvmField internal var `returnValue`: Byte = 0.toByte(),
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Byte = 0.toByte(),
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructU8(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructU8) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteU8 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructU8.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructI8(
@JvmField internal var `returnValue`: Byte = 0.toByte(),
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Byte = 0.toByte(),
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructI8(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructI8) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteI8 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructI8.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructU16(
@JvmField internal var `returnValue`: Short = 0.toShort(),
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Short = 0.toShort(),
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructU16(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructU16) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteU16 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructU16.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructI16(
@JvmField internal var `returnValue`: Short = 0.toShort(),
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Short = 0.toShort(),
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructI16(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructI16) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteI16 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructI16.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructU32(
@JvmField internal var `returnValue`: Int = 0,
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Int = 0,
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructU32(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructU32) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteU32 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructU32.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructI32(
@JvmField internal var `returnValue`: Int = 0,
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Int = 0,
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructI32(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructI32) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteI32 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructI32.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructU64(
@JvmField internal var `returnValue`: Long = 0.toLong(),
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Long = 0.toLong(),
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructU64(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructU64) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteU64 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructU64.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructI64(
@JvmField internal var `returnValue`: Long = 0.toLong(),
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Long = 0.toLong(),
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructI64(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructI64) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteI64 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructI64.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructF32(
@JvmField internal var `returnValue`: Float = 0.0f,
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Float = 0.0f,
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructF32(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructF32) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteF32 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructF32.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructF64(
@JvmField internal var `returnValue`: Double = 0.0,
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Double = 0.0,
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructF64(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructF64) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteF64 : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructF64.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructPointer(
@JvmField internal var `returnValue`: Pointer = Pointer.NULL,
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: Pointer = Pointer.NULL,
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructPointer(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructPointer) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompletePointer : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructPointer.UniffiByValue,)
}
@Structure.FieldOrder("returnValue", "callStatus")
internal open class UniffiForeignFutureStructRustBuffer(
@JvmField internal var `returnValue`: RustBuffer.ByValue = RustBuffer.ByValue(),
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`returnValue`: RustBuffer.ByValue = RustBuffer.ByValue(),
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructRustBuffer(`returnValue`,`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructRustBuffer) {
`returnValue` = other.`returnValue`
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteRustBuffer : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructRustBuffer.UniffiByValue,)
}
@Structure.FieldOrder("callStatus")
internal open class UniffiForeignFutureStructVoid(
@JvmField internal var `callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
) : Structure() {
class UniffiByValue(
`callStatus`: UniffiRustCallStatus.ByValue = UniffiRustCallStatus.ByValue(),
): UniffiForeignFutureStructVoid(`callStatus`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiForeignFutureStructVoid) {
`callStatus` = other.`callStatus`
}
}
internal interface UniffiForeignFutureCompleteVoid : com.sun.jna.Callback {
fun callback(`callbackData`: Long,`result`: UniffiForeignFutureStructVoid.UniffiByValue,)
}
internal interface UniffiCallbackInterfaceCoreCryptoCallbacksMethod0 : com.sun.jna.Callback {
fun callback(`uniffiHandle`: Long,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,`uniffiFutureCallback`: UniffiForeignFutureCompleteI8,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,)
}
internal interface UniffiCallbackInterfaceCoreCryptoCallbacksMethod1 : com.sun.jna.Callback {
fun callback(`uniffiHandle`: Long,`conversationId`: RustBuffer.ByValue,`externalClientId`: RustBuffer.ByValue,`existingClients`: RustBuffer.ByValue,`uniffiFutureCallback`: UniffiForeignFutureCompleteI8,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,)
}
internal interface UniffiCallbackInterfaceCoreCryptoCallbacksMethod2 : com.sun.jna.Callback {
fun callback(`uniffiHandle`: Long,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,`existingClients`: RustBuffer.ByValue,`parentConversationClients`: RustBuffer.ByValue,`uniffiFutureCallback`: UniffiForeignFutureCompleteI8,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,)
}
internal interface UniffiCallbackInterfaceCoreCryptoCommandMethod0 : com.sun.jna.Callback {
fun callback(`uniffiHandle`: Long,`context`: Pointer,`uniffiFutureCallback`: UniffiForeignFutureCompleteVoid,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,)
}
internal interface UniffiCallbackInterfaceCoreCryptoLoggerMethod0 : com.sun.jna.Callback {
fun callback(`uniffiHandle`: Long,`level`: RustBuffer.ByValue,`jsonMsg`: RustBuffer.ByValue,`uniffiOutReturn`: Pointer,uniffiCallStatus: UniffiRustCallStatus,)
}
@Structure.FieldOrder("authorize", "userAuthorize", "clientIsExistingGroupUser", "uniffiFree")
internal open class UniffiVTableCallbackInterfaceCoreCryptoCallbacks(
@JvmField internal var `authorize`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod0? = null,
@JvmField internal var `userAuthorize`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod1? = null,
@JvmField internal var `clientIsExistingGroupUser`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod2? = null,
@JvmField internal var `uniffiFree`: UniffiCallbackInterfaceFree? = null,
) : Structure() {
class UniffiByValue(
`authorize`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod0? = null,
`userAuthorize`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod1? = null,
`clientIsExistingGroupUser`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod2? = null,
`uniffiFree`: UniffiCallbackInterfaceFree? = null,
): UniffiVTableCallbackInterfaceCoreCryptoCallbacks(`authorize`,`userAuthorize`,`clientIsExistingGroupUser`,`uniffiFree`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiVTableCallbackInterfaceCoreCryptoCallbacks) {
`authorize` = other.`authorize`
`userAuthorize` = other.`userAuthorize`
`clientIsExistingGroupUser` = other.`clientIsExistingGroupUser`
`uniffiFree` = other.`uniffiFree`
}
}
@Structure.FieldOrder("execute", "uniffiFree")
internal open class UniffiVTableCallbackInterfaceCoreCryptoCommand(
@JvmField internal var `execute`: UniffiCallbackInterfaceCoreCryptoCommandMethod0? = null,
@JvmField internal var `uniffiFree`: UniffiCallbackInterfaceFree? = null,
) : Structure() {
class UniffiByValue(
`execute`: UniffiCallbackInterfaceCoreCryptoCommandMethod0? = null,
`uniffiFree`: UniffiCallbackInterfaceFree? = null,
): UniffiVTableCallbackInterfaceCoreCryptoCommand(`execute`,`uniffiFree`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiVTableCallbackInterfaceCoreCryptoCommand) {
`execute` = other.`execute`
`uniffiFree` = other.`uniffiFree`
}
}
@Structure.FieldOrder("log", "uniffiFree")
internal open class UniffiVTableCallbackInterfaceCoreCryptoLogger(
@JvmField internal var `log`: UniffiCallbackInterfaceCoreCryptoLoggerMethod0? = null,
@JvmField internal var `uniffiFree`: UniffiCallbackInterfaceFree? = null,
) : Structure() {
class UniffiByValue(
`log`: UniffiCallbackInterfaceCoreCryptoLoggerMethod0? = null,
`uniffiFree`: UniffiCallbackInterfaceFree? = null,
): UniffiVTableCallbackInterfaceCoreCryptoLogger(`log`,`uniffiFree`,), Structure.ByValue
internal fun uniffiSetValue(other: UniffiVTableCallbackInterfaceCoreCryptoLogger) {
`log` = other.`log`
`uniffiFree` = other.`uniffiFree`
}
}
// A JNA Library to expose the extern-C FFI definitions.
// This is an implementation detail which will be called internally by the public API.
internal interface UniffiLib : Library {
companion object {
internal val INSTANCE: UniffiLib by lazy {
loadIndirect(componentName = "core_crypto_ffi")
.also { lib: UniffiLib ->
uniffiCheckContractApiVersion(lib)
uniffiCheckApiChecksums(lib)
uniffiCallbackInterfaceCoreCryptoCallbacks.register(lib)
uniffiCallbackInterfaceCoreCryptoCommand.register(lib)
uniffiCallbackInterfaceCoreCryptoLogger.register(lib)
}
}
// The Cleaner for the whole library
internal val CLEANER: UniffiCleaner by lazy {
UniffiCleaner.create()
}
}
fun uniffi_core_crypto_ffi_fn_clone_corecrypto(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Pointer
fun uniffi_core_crypto_ffi_fn_free_corecrypto(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_constructor_corecrypto_new(`path`: RustBuffer.ByValue,`key`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,`ciphersuites`: RustBuffer.ByValue,`nbKeyPackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_add_clients_to_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`keyPackages`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_clear_pending_commit(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_clear_pending_group_from_external_commit(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_clear_pending_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`proposalRef`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_client_keypackages(`ptr`: Pointer,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,`amountRequested`: Int,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_client_public_key(`ptr`: Pointer,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_client_valid_keypackages_count(`ptr`: Pointer,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_commit_accepted(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_commit_pending_proposals(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_conversation_ciphersuite(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_conversation_epoch(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_conversation_exists(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_create_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`creatorCredentialType`: RustBuffer.ByValue,`config`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_decrypt_message(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`payload`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_delete_keypackages(`ptr`: Pointer,`refs`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_conversation_state(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_dump_pki_env(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_enrollment_stash(`ptr`: Pointer,`enrollment`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_enrollment_stash_pop(`ptr`: Pointer,`handle`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_is_enabled(`ptr`: Pointer,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_is_pki_env_setup(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_mls_init_only(`ptr`: Pointer,`enrollment`: Pointer,`certificateChain`: RustBuffer.ByValue,`nbKeyPackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_new_activation_enrollment(`ptr`: Pointer,`displayName`: RustBuffer.ByValue,`handle`: RustBuffer.ByValue,`team`: RustBuffer.ByValue,`expirySec`: Int,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_new_enrollment(`ptr`: Pointer,`clientId`: RustBuffer.ByValue,`displayName`: RustBuffer.ByValue,`handle`: RustBuffer.ByValue,`team`: RustBuffer.ByValue,`expirySec`: Int,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_new_rotate_enrollment(`ptr`: Pointer,`displayName`: RustBuffer.ByValue,`handle`: RustBuffer.ByValue,`team`: RustBuffer.ByValue,`expirySec`: Int,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_register_acme_ca(`ptr`: Pointer,`trustAnchorPem`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_register_crl(`ptr`: Pointer,`crlDp`: RustBuffer.ByValue,`crlDer`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_register_intermediate_ca(`ptr`: Pointer,`certPem`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_rotate(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_rotate_all(`ptr`: Pointer,`enrollment`: Pointer,`certificateChain`: RustBuffer.ByValue,`newKeyPackagesCount`: Int,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_encrypt_message(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`message`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_export_secret_key(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`keyLength`: Int,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_get_client_ids(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_get_credential_in_use(`ptr`: Pointer,`groupInfo`: RustBuffer.ByValue,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_get_device_identities(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`deviceIds`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_get_external_sender(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_get_user_identities(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`userIds`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_join_by_external_commit(`ptr`: Pointer,`groupInfo`: RustBuffer.ByValue,`customConfiguration`: RustBuffer.ByValue,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_mark_conversation_as_child_of(`ptr`: Pointer,`childId`: RustBuffer.ByValue,`parentId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_merge_pending_group_from_external_commit(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_mls_generate_keypairs(`ptr`: Pointer,`ciphersuites`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_mls_init(`ptr`: Pointer,`clientId`: RustBuffer.ByValue,`ciphersuites`: RustBuffer.ByValue,`nbKeyPackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_mls_init_with_client_id(`ptr`: Pointer,`clientId`: RustBuffer.ByValue,`tmpClientIds`: RustBuffer.ByValue,`ciphersuites`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_new_add_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`keypackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_new_external_add_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`epoch`: Long,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_new_remove_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_new_update_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_process_welcome_message(`ptr`: Pointer,`welcomeMessage`: RustBuffer.ByValue,`customConfiguration`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_cryptobox_migrate(`ptr`: Pointer,`path`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_decrypt(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`ciphertext`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_encrypt(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`plaintext`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_encrypt_batched(`ptr`: Pointer,`sessions`: RustBuffer.ByValue,`plaintext`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint_local(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint_prekeybundle(`ptr`: Pointer,`prekey`: RustBuffer.ByValue,uniffi_out_err: UniffiRustCallStatus,
): RustBuffer.ByValue
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint_remote(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_init(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_last_error_code(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Int
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_last_resort_prekey(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_last_resort_prekey_id(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Short
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_new_prekey(`ptr`: Pointer,`prekeyId`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_new_prekey_auto(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_delete(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_exists(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_from_message(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`envelope`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_from_prekey(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`prekey`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_save(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_random_bytes(`ptr`: Pointer,`len`: Int,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_remove_clients_from_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`clients`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_reseed_rng(`ptr`: Pointer,`seed`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_restore_from_disk(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_set_callbacks(`ptr`: Pointer,`callbacks`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_transaction(`ptr`: Pointer,`command`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_unload(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_update_keying_material(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_wipe(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecrypto_wipe_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_clone_corecryptocallbacks(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Pointer
fun uniffi_core_crypto_ffi_fn_free_corecryptocallbacks(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_init_callback_vtable_corecryptocallbacks(`vtable`: UniffiVTableCallbackInterfaceCoreCryptoCallbacks,
): Unit
fun uniffi_core_crypto_ffi_fn_method_corecryptocallbacks_authorize(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocallbacks_user_authorize(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`externalClientId`: RustBuffer.ByValue,`existingClients`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocallbacks_client_is_existing_group_user(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,`existingClients`: RustBuffer.ByValue,`parentConversationClients`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_clone_corecryptocommand(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Pointer
fun uniffi_core_crypto_ffi_fn_free_corecryptocommand(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_init_callback_vtable_corecryptocommand(`vtable`: UniffiVTableCallbackInterfaceCoreCryptoCommand,
): Unit
fun uniffi_core_crypto_ffi_fn_method_corecryptocommand_execute(`ptr`: Pointer,`context`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_clone_corecryptocontext(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Pointer
fun uniffi_core_crypto_ffi_fn_free_corecryptocontext(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_add_clients_to_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`keyPackages`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_clear_pending_commit(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_clear_pending_group_from_external_commit(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_clear_pending_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`proposalRef`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_client_keypackages(`ptr`: Pointer,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,`amountRequested`: Int,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_client_public_key(`ptr`: Pointer,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_client_valid_keypackages_count(`ptr`: Pointer,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_commit_accepted(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_commit_pending_proposals(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_conversation_ciphersuite(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_conversation_epoch(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_conversation_exists(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_create_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`creatorCredentialType`: RustBuffer.ByValue,`config`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_decrypt_message(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`payload`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_delete_keypackages(`ptr`: Pointer,`refs`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_conversation_state(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_dump_pki_env(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_enrollment_stash(`ptr`: Pointer,`enrollment`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_enrollment_stash_pop(`ptr`: Pointer,`handle`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_is_enabled(`ptr`: Pointer,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_is_pki_env_setup(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_mls_init_only(`ptr`: Pointer,`enrollment`: Pointer,`certificateChain`: RustBuffer.ByValue,`nbKeyPackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_new_activation_enrollment(`ptr`: Pointer,`displayName`: RustBuffer.ByValue,`handle`: RustBuffer.ByValue,`team`: RustBuffer.ByValue,`expirySec`: Int,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_new_enrollment(`ptr`: Pointer,`clientId`: RustBuffer.ByValue,`displayName`: RustBuffer.ByValue,`handle`: RustBuffer.ByValue,`team`: RustBuffer.ByValue,`expirySec`: Int,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_new_rotate_enrollment(`ptr`: Pointer,`displayName`: RustBuffer.ByValue,`handle`: RustBuffer.ByValue,`team`: RustBuffer.ByValue,`expirySec`: Int,`ciphersuite`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_register_acme_ca(`ptr`: Pointer,`trustAnchorPem`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_register_crl(`ptr`: Pointer,`crlDp`: RustBuffer.ByValue,`crlDer`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_register_intermediate_ca(`ptr`: Pointer,`certPem`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_rotate(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_rotate_all(`ptr`: Pointer,`enrollment`: Pointer,`certificateChain`: RustBuffer.ByValue,`newKeyPackagesCount`: Int,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_encrypt_message(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`message`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_export_secret_key(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`keyLength`: Int,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_client_ids(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_credential_in_use(`ptr`: Pointer,`groupInfo`: RustBuffer.ByValue,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_data(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_device_identities(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`deviceIds`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_external_sender(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_user_identities(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`userIds`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_join_by_external_commit(`ptr`: Pointer,`groupInfo`: RustBuffer.ByValue,`customConfiguration`: RustBuffer.ByValue,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_mark_conversation_as_child_of(`ptr`: Pointer,`childId`: RustBuffer.ByValue,`parentId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_merge_pending_group_from_external_commit(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_mls_generate_keypairs(`ptr`: Pointer,`ciphersuites`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_mls_init(`ptr`: Pointer,`clientId`: RustBuffer.ByValue,`ciphersuites`: RustBuffer.ByValue,`nbKeyPackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_mls_init_with_client_id(`ptr`: Pointer,`clientId`: RustBuffer.ByValue,`tmpClientIds`: RustBuffer.ByValue,`ciphersuites`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_add_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`keypackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_external_add_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`epoch`: Long,`ciphersuite`: Short,`credentialType`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_remove_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_update_proposal(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_process_welcome_message(`ptr`: Pointer,`welcomeMessage`: RustBuffer.ByValue,`customConfiguration`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_cryptobox_migrate(`ptr`: Pointer,`path`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_decrypt(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`ciphertext`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_encrypt(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`plaintext`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_encrypt_batched(`ptr`: Pointer,`sessions`: RustBuffer.ByValue,`plaintext`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint_local(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint_prekeybundle(`ptr`: Pointer,`prekey`: RustBuffer.ByValue,uniffi_out_err: UniffiRustCallStatus,
): RustBuffer.ByValue
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint_remote(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_last_error_code(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Int
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_last_resort_prekey(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_last_resort_prekey_id(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Short
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_new_prekey(`ptr`: Pointer,`prekeyId`: Short,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_new_prekey_auto(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_delete(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_exists(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_from_message(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`envelope`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_from_prekey(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,`prekey`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_save(`ptr`: Pointer,`sessionId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_remove_clients_from_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,`clients`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_set_data(`ptr`: Pointer,`data`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_update_keying_material(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_corecryptocontext_wipe_conversation(`ptr`: Pointer,`conversationId`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_clone_corecryptologger(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Pointer
fun uniffi_core_crypto_ffi_fn_free_corecryptologger(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_init_callback_vtable_corecryptologger(`vtable`: UniffiVTableCallbackInterfaceCoreCryptoLogger,
): Unit
fun uniffi_core_crypto_ffi_fn_method_corecryptologger_log(`ptr`: Pointer,`level`: RustBuffer.ByValue,`jsonMsg`: RustBuffer.ByValue,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_clone_e2eienrollment(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Pointer
fun uniffi_core_crypto_ffi_fn_free_e2eienrollment(`ptr`: Pointer,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_certificate_request(`ptr`: Pointer,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_check_order_request(`ptr`: Pointer,`orderUrl`: RustBuffer.ByValue,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_check_order_response(`ptr`: Pointer,`order`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_context_new_oidc_challenge_response(`ptr`: Pointer,`cc`: Pointer,`challenge`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_create_dpop_token(`ptr`: Pointer,`expirySecs`: Int,`backendNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_directory_response(`ptr`: Pointer,`directory`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_finalize_request(`ptr`: Pointer,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_finalize_response(`ptr`: Pointer,`finalize`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_get_refresh_token(`ptr`: Pointer,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_account_request(`ptr`: Pointer,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_account_response(`ptr`: Pointer,`account`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_authz_request(`ptr`: Pointer,`url`: RustBuffer.ByValue,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_authz_response(`ptr`: Pointer,`authz`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_dpop_challenge_request(`ptr`: Pointer,`accessToken`: RustBuffer.ByValue,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_dpop_challenge_response(`ptr`: Pointer,`challenge`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_oidc_challenge_request(`ptr`: Pointer,`idToken`: RustBuffer.ByValue,`refreshToken`: RustBuffer.ByValue,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_oidc_challenge_response(`ptr`: Pointer,`cc`: Pointer,`challenge`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_order_request(`ptr`: Pointer,`previousNonce`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_order_response(`ptr`: Pointer,`order`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_func_core_crypto_deferred_init(`path`: RustBuffer.ByValue,`key`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_func_core_crypto_new(`path`: RustBuffer.ByValue,`key`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,`ciphersuites`: RustBuffer.ByValue,`nbKeyPackage`: RustBuffer.ByValue,
): Long
fun uniffi_core_crypto_ffi_fn_func_set_logger(`logger`: Pointer,`level`: RustBuffer.ByValue,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_fn_func_version(uniffi_out_err: UniffiRustCallStatus,
): RustBuffer.ByValue
fun ffi_core_crypto_ffi_rustbuffer_alloc(`size`: Long,uniffi_out_err: UniffiRustCallStatus,
): RustBuffer.ByValue
fun ffi_core_crypto_ffi_rustbuffer_from_bytes(`bytes`: ForeignBytes.ByValue,uniffi_out_err: UniffiRustCallStatus,
): RustBuffer.ByValue
fun ffi_core_crypto_ffi_rustbuffer_free(`buf`: RustBuffer.ByValue,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun ffi_core_crypto_ffi_rustbuffer_reserve(`buf`: RustBuffer.ByValue,`additional`: Long,uniffi_out_err: UniffiRustCallStatus,
): RustBuffer.ByValue
fun ffi_core_crypto_ffi_rust_future_poll_u8(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_u8(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_u8(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_u8(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Byte
fun ffi_core_crypto_ffi_rust_future_poll_i8(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_i8(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_i8(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_i8(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Byte
fun ffi_core_crypto_ffi_rust_future_poll_u16(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_u16(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_u16(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_u16(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Short
fun ffi_core_crypto_ffi_rust_future_poll_i16(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_i16(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_i16(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_i16(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Short
fun ffi_core_crypto_ffi_rust_future_poll_u32(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_u32(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_u32(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_u32(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Int
fun ffi_core_crypto_ffi_rust_future_poll_i32(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_i32(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_i32(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_i32(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Int
fun ffi_core_crypto_ffi_rust_future_poll_u64(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_u64(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_u64(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_u64(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Long
fun ffi_core_crypto_ffi_rust_future_poll_i64(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_i64(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_i64(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_i64(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Long
fun ffi_core_crypto_ffi_rust_future_poll_f32(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_f32(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_f32(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_f32(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Float
fun ffi_core_crypto_ffi_rust_future_poll_f64(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_f64(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_f64(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_f64(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Double
fun ffi_core_crypto_ffi_rust_future_poll_pointer(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_pointer(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_pointer(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_pointer(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Pointer
fun ffi_core_crypto_ffi_rust_future_poll_rust_buffer(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_rust_buffer(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_rust_buffer(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_rust_buffer(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): RustBuffer.ByValue
fun ffi_core_crypto_ffi_rust_future_poll_void(`handle`: Long,`callback`: UniffiRustFutureContinuationCallback,`callbackData`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_cancel_void(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_free_void(`handle`: Long,
): Unit
fun ffi_core_crypto_ffi_rust_future_complete_void(`handle`: Long,uniffi_out_err: UniffiRustCallStatus,
): Unit
fun uniffi_core_crypto_ffi_checksum_func_core_crypto_deferred_init(
): Short
fun uniffi_core_crypto_ffi_checksum_func_core_crypto_new(
): Short
fun uniffi_core_crypto_ffi_checksum_func_set_logger(
): Short
fun uniffi_core_crypto_ffi_checksum_func_version(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_add_clients_to_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_clear_pending_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_clear_pending_group_from_external_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_clear_pending_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_client_keypackages(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_client_public_key(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_client_valid_keypackages_count(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_commit_accepted(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_commit_pending_proposals(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_conversation_ciphersuite(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_conversation_epoch(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_conversation_exists(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_create_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_decrypt_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_delete_keypackages(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_conversation_state(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_dump_pki_env(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_enrollment_stash(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_enrollment_stash_pop(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_is_enabled(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_is_pki_env_setup(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_mls_init_only(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_new_activation_enrollment(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_new_enrollment(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_new_rotate_enrollment(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_register_acme_ca(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_register_crl(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_register_intermediate_ca(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_rotate(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_rotate_all(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_encrypt_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_export_secret_key(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_get_client_ids(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_get_credential_in_use(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_get_device_identities(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_get_external_sender(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_get_user_identities(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_join_by_external_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_mark_conversation_as_child_of(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_merge_pending_group_from_external_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_mls_generate_keypairs(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_mls_init(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_mls_init_with_client_id(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_new_add_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_new_external_add_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_new_remove_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_new_update_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_process_welcome_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_cryptobox_migrate(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_decrypt(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_encrypt(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_encrypt_batched(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint_local(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint_prekeybundle(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint_remote(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_init(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_last_error_code(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_last_resort_prekey(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_last_resort_prekey_id(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_new_prekey(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_new_prekey_auto(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_delete(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_exists(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_from_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_from_prekey(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_save(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_random_bytes(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_remove_clients_from_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_reseed_rng(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_restore_from_disk(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_set_callbacks(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_transaction(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_unload(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_update_keying_material(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_wipe(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecrypto_wipe_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocallbacks_authorize(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocallbacks_user_authorize(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocallbacks_client_is_existing_group_user(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocommand_execute(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_add_clients_to_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_clear_pending_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_clear_pending_group_from_external_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_clear_pending_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_client_keypackages(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_client_public_key(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_client_valid_keypackages_count(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_commit_accepted(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_commit_pending_proposals(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_conversation_ciphersuite(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_conversation_epoch(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_conversation_exists(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_create_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_decrypt_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_delete_keypackages(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_conversation_state(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_dump_pki_env(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_enrollment_stash(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_enrollment_stash_pop(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_is_enabled(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_is_pki_env_setup(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_mls_init_only(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_new_activation_enrollment(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_new_enrollment(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_new_rotate_enrollment(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_register_acme_ca(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_register_crl(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_register_intermediate_ca(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_rotate(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_rotate_all(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_encrypt_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_export_secret_key(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_client_ids(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_credential_in_use(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_data(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_device_identities(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_external_sender(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_user_identities(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_join_by_external_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mark_conversation_as_child_of(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_merge_pending_group_from_external_commit(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mls_generate_keypairs(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mls_init(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mls_init_with_client_id(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_add_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_external_add_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_remove_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_update_proposal(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_process_welcome_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_cryptobox_migrate(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_decrypt(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_encrypt(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_encrypt_batched(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint_local(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint_prekeybundle(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint_remote(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_last_error_code(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_last_resort_prekey(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_last_resort_prekey_id(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_new_prekey(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_new_prekey_auto(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_delete(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_exists(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_from_message(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_from_prekey(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_save(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_remove_clients_from_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_set_data(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_update_keying_material(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptocontext_wipe_conversation(
): Short
fun uniffi_core_crypto_ffi_checksum_method_corecryptologger_log(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_certificate_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_check_order_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_check_order_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_context_new_oidc_challenge_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_create_dpop_token(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_directory_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_finalize_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_finalize_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_get_refresh_token(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_account_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_account_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_authz_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_authz_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_dpop_challenge_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_dpop_challenge_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_oidc_challenge_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_oidc_challenge_response(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_order_request(
): Short
fun uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_order_response(
): Short
fun uniffi_core_crypto_ffi_checksum_constructor_corecrypto_new(
): Short
fun ffi_core_crypto_ffi_uniffi_contract_version(
): Int
}
private fun uniffiCheckContractApiVersion(lib: UniffiLib) {
// Get the bindings contract version from our ComponentInterface
val bindings_contract_version = 26
// Get the scaffolding contract version by calling the into the dylib
val scaffolding_contract_version = lib.ffi_core_crypto_ffi_uniffi_contract_version()
if (bindings_contract_version != scaffolding_contract_version) {
throw RuntimeException("UniFFI contract version mismatch: try cleaning and rebuilding your project")
}
}
@Suppress("UNUSED_PARAMETER")
private fun uniffiCheckApiChecksums(lib: UniffiLib) {
if (lib.uniffi_core_crypto_ffi_checksum_func_core_crypto_deferred_init() != 41714.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_func_core_crypto_new() != 45043.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_func_set_logger() != 47135.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_func_version() != 21675.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_add_clients_to_conversation() != 51134.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_clear_pending_commit() != 5252.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_clear_pending_group_from_external_commit() != 968.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_clear_pending_proposal() != 20561.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_client_keypackages() != 9693.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_client_public_key() != 43475.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_client_valid_keypackages_count() != 53401.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_commit_accepted() != 51244.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_commit_pending_proposals() != 936.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_conversation_ciphersuite() != 37252.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_conversation_epoch() != 13474.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_conversation_exists() != 40976.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_create_conversation() != 64478.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_decrypt_message() != 24074.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_delete_keypackages() != 13956.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_conversation_state() != 39527.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_dump_pki_env() != 36695.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_enrollment_stash() != 62384.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_enrollment_stash_pop() != 40957.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_is_enabled() != 11310.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_is_pki_env_setup() != 51211.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_mls_init_only() != 36813.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_new_activation_enrollment() != 35694.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_new_enrollment() != 55311.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_new_rotate_enrollment() != 2322.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_register_acme_ca() != 18514.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_register_crl() != 27319.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_register_intermediate_ca() != 57774.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_rotate() != 3530.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_e2ei_rotate_all() != 22173.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_encrypt_message() != 12728.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_export_secret_key() != 25919.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_get_client_ids() != 34697.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_get_credential_in_use() != 12035.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_get_device_identities() != 15593.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_get_external_sender() != 22306.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_get_user_identities() != 57578.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_join_by_external_commit() != 14384.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_mark_conversation_as_child_of() != 15798.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_merge_pending_group_from_external_commit() != 52719.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_mls_generate_keypairs() != 9148.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_mls_init() != 23524.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_mls_init_with_client_id() != 13529.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_new_add_proposal() != 55414.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_new_external_add_proposal() != 4442.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_new_remove_proposal() != 29405.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_new_update_proposal() != 6488.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_process_welcome_message() != 62252.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_cryptobox_migrate() != 24126.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_decrypt() != 37634.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_encrypt() != 43631.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_encrypt_batched() != 41773.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint() != 31126.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint_local() != 14939.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint_prekeybundle() != 34474.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_fingerprint_remote() != 24839.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_init() != 1771.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_last_error_code() != 30369.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_last_resort_prekey() != 59352.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_last_resort_prekey_id() != 12420.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_new_prekey() != 42395.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_new_prekey_auto() != 49736.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_delete() != 36438.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_exists() != 48377.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_from_message() != 52528.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_from_prekey() != 16642.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_proteus_session_save() != 51811.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_random_bytes() != 933.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_remove_clients_from_conversation() != 28945.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_reseed_rng() != 65396.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_restore_from_disk() != 64078.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_set_callbacks() != 20336.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_transaction() != 60114.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_unload() != 46489.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_update_keying_material() != 25396.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_wipe() != 5281.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecrypto_wipe_conversation() != 29780.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocallbacks_authorize() != 30420.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocallbacks_user_authorize() != 9674.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocallbacks_client_is_existing_group_user() != 4052.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocommand_execute() != 27930.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_add_clients_to_conversation() != 29479.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_clear_pending_commit() != 10411.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_clear_pending_group_from_external_commit() != 9795.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_clear_pending_proposal() != 57638.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_client_keypackages() != 63876.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_client_public_key() != 44527.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_client_valid_keypackages_count() != 22428.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_commit_accepted() != 32788.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_commit_pending_proposals() != 28871.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_conversation_ciphersuite() != 50321.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_conversation_epoch() != 34194.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_conversation_exists() != 28328.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_create_conversation() != 21977.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_decrypt_message() != 45862.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_delete_keypackages() != 4101.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_conversation_state() != 15889.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_dump_pki_env() != 28491.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_enrollment_stash() != 50230.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_enrollment_stash_pop() != 33429.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_is_enabled() != 60133.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_is_pki_env_setup() != 16708.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_mls_init_only() != 11146.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_new_activation_enrollment() != 56248.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_new_enrollment() != 55616.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_new_rotate_enrollment() != 54571.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_register_acme_ca() != 30107.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_register_crl() != 45988.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_register_intermediate_ca() != 54589.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_rotate() != 57302.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_e2ei_rotate_all() != 36721.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_encrypt_message() != 56099.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_export_secret_key() != 7502.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_client_ids() != 37503.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_credential_in_use() != 47165.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_data() != 44920.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_device_identities() != 62999.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_external_sender() != 63438.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_get_user_identities() != 8939.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_join_by_external_commit() != 14250.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mark_conversation_as_child_of() != 54971.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_merge_pending_group_from_external_commit() != 56937.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mls_generate_keypairs() != 5138.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mls_init() != 23082.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_mls_init_with_client_id() != 37213.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_add_proposal() != 29984.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_external_add_proposal() != 55299.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_remove_proposal() != 55939.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_new_update_proposal() != 2430.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_process_welcome_message() != 21775.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_cryptobox_migrate() != 17708.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_decrypt() != 52452.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_encrypt() != 63305.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_encrypt_batched() != 3504.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint() != 29135.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint_local() != 55049.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint_prekeybundle() != 2508.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_fingerprint_remote() != 22420.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_last_error_code() != 38886.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_last_resort_prekey() != 10198.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_last_resort_prekey_id() != 7494.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_new_prekey() != 7954.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_new_prekey_auto() != 46579.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_delete() != 45271.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_exists() != 37153.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_from_message() != 27324.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_from_prekey() != 12061.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_proteus_session_save() != 3989.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_remove_clients_from_conversation() != 26278.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_set_data() != 16727.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_update_keying_material() != 55623.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptocontext_wipe_conversation() != 64598.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_corecryptologger_log() != 39376.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_certificate_request() != 30291.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_check_order_request() != 33107.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_check_order_response() != 63180.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_context_new_oidc_challenge_response() != 22177.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_create_dpop_token() != 1308.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_directory_response() != 55128.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_finalize_request() != 3915.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_finalize_response() != 42348.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_get_refresh_token() != 6455.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_account_request() != 35214.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_account_response() != 47907.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_authz_request() != 57732.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_authz_response() != 41996.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_dpop_challenge_request() != 26253.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_dpop_challenge_response() != 6262.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_oidc_challenge_request() != 17956.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_oidc_challenge_response() != 2098.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_order_request() != 37522.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_method_e2eienrollment_new_order_response() != 54401.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
if (lib.uniffi_core_crypto_ffi_checksum_constructor_corecrypto_new() != 42469.toShort()) {
throw RuntimeException("UniFFI API checksum mismatch: try cleaning and rebuilding your project")
}
}
// Async support
// Async return type handlers
internal const val UNIFFI_RUST_FUTURE_POLL_READY = 0.toByte()
internal const val UNIFFI_RUST_FUTURE_POLL_MAYBE_READY = 1.toByte()
internal val uniffiContinuationHandleMap = UniffiHandleMap>()
// FFI type for Rust future continuations
internal object uniffiRustFutureContinuationCallbackImpl: UniffiRustFutureContinuationCallback {
override fun callback(data: Long, pollResult: Byte) {
uniffiContinuationHandleMap.remove(data).resume(pollResult)
}
}
internal suspend fun uniffiRustCallAsync(
rustFuture: Long,
pollFunc: (Long, UniffiRustFutureContinuationCallback, Long) -> Unit,
completeFunc: (Long, UniffiRustCallStatus) -> F,
freeFunc: (Long) -> Unit,
liftFunc: (F) -> T,
errorHandler: UniffiRustCallStatusErrorHandler
): T {
try {
do {
val pollResult = suspendCancellableCoroutine { continuation ->
pollFunc(
rustFuture,
uniffiRustFutureContinuationCallbackImpl,
uniffiContinuationHandleMap.insert(continuation)
)
}
} while (pollResult != UNIFFI_RUST_FUTURE_POLL_READY);
return liftFunc(
uniffiRustCallWithError(errorHandler, { status -> completeFunc(rustFuture, status) })
)
} finally {
freeFunc(rustFuture)
}
}
internal inline fun uniffiTraitInterfaceCallAsync(
crossinline makeCall: suspend () -> T,
crossinline handleSuccess: (T) -> Unit,
crossinline handleError: (UniffiRustCallStatus.ByValue) -> Unit,
): UniffiForeignFuture {
// Using `GlobalScope` is labeled as a "delicate API" and generally discouraged in Kotlin programs, since it breaks structured concurrency.
// However, our parent task is a Rust future, so we're going to need to break structure concurrency in any case.
//
// Uniffi does its best to support structured concurrency across the FFI.
// If the Rust future is dropped, `uniffiForeignFutureFreeImpl` is called, which will cancel the Kotlin coroutine if it's still running.
@OptIn(DelicateCoroutinesApi::class)
val job = GlobalScope.launch {
try {
handleSuccess(makeCall())
} catch(e: Exception) {
handleError(
UniffiRustCallStatus.create(
UNIFFI_CALL_UNEXPECTED_ERROR,
FfiConverterString.lower(e.toString()),
)
)
}
}
val handle = uniffiForeignFutureHandleMap.insert(job)
return UniffiForeignFuture(handle, uniffiForeignFutureFreeImpl)
}
internal inline fun uniffiTraitInterfaceCallAsyncWithError(
crossinline makeCall: suspend () -> T,
crossinline handleSuccess: (T) -> Unit,
crossinline handleError: (UniffiRustCallStatus.ByValue) -> Unit,
crossinline lowerError: (E) -> RustBuffer.ByValue,
): UniffiForeignFuture {
// See uniffiTraitInterfaceCallAsync for details on `DelicateCoroutinesApi`
@OptIn(DelicateCoroutinesApi::class)
val job = GlobalScope.launch {
try {
handleSuccess(makeCall())
} catch(e: Exception) {
if (e is E) {
handleError(
UniffiRustCallStatus.create(
UNIFFI_CALL_ERROR,
lowerError(e),
)
)
} else {
handleError(
UniffiRustCallStatus.create(
UNIFFI_CALL_UNEXPECTED_ERROR,
FfiConverterString.lower(e.toString()),
)
)
}
}
}
val handle = uniffiForeignFutureHandleMap.insert(job)
return UniffiForeignFuture(handle, uniffiForeignFutureFreeImpl)
}
internal val uniffiForeignFutureHandleMap = UniffiHandleMap()
internal object uniffiForeignFutureFreeImpl: UniffiForeignFutureFree {
override fun callback(handle: Long) {
val job = uniffiForeignFutureHandleMap.remove(handle)
if (!job.isCompleted) {
job.cancel()
}
}
}
// For testing
public fun uniffiForeignFutureHandleCount() = uniffiForeignFutureHandleMap.size
// Public interface members begin here.
// Interface implemented by anything that can contain an object reference.
//
// Such types expose a `destroy()` method that must be called to cleanly
// dispose of the contained objects. Failure to call this method may result
// in memory leaks.
//
// The easiest way to ensure this method is called is to use the `.use`
// helper method to execute a block and destroy the object at the end.
interface Disposable {
fun destroy()
companion object {
fun destroy(vararg args: Any?) {
args.filterIsInstance()
.forEach(Disposable::destroy)
}
}
}
inline fun T.use(block: (T) -> R) =
try {
block(this)
} finally {
try {
// N.B. our implementation is on the nullable type `Disposable?`.
this?.destroy()
} catch (e: Throwable) {
// swallow
}
}
/** Used to instantiate an interface without an actual pointer, for fakes in tests, mostly. */
object NoPointer
public object FfiConverterUShort: FfiConverter {
override fun lift(value: Short): UShort {
return value.toUShort()
}
override fun read(buf: ByteBuffer): UShort {
return lift(buf.getShort())
}
override fun lower(value: UShort): Short {
return value.toShort()
}
override fun allocationSize(value: UShort) = 2UL
override fun write(value: UShort, buf: ByteBuffer) {
buf.putShort(value.toShort())
}
}
public object FfiConverterUInt: FfiConverter {
override fun lift(value: Int): UInt {
return value.toUInt()
}
override fun read(buf: ByteBuffer): UInt {
return lift(buf.getInt())
}
override fun lower(value: UInt): Int {
return value.toInt()
}
override fun allocationSize(value: UInt) = 4UL
override fun write(value: UInt, buf: ByteBuffer) {
buf.putInt(value.toInt())
}
}
public object FfiConverterULong: FfiConverter {
override fun lift(value: Long): ULong {
return value.toULong()
}
override fun read(buf: ByteBuffer): ULong {
return lift(buf.getLong())
}
override fun lower(value: ULong): Long {
return value.toLong()
}
override fun allocationSize(value: ULong) = 8UL
override fun write(value: ULong, buf: ByteBuffer) {
buf.putLong(value.toLong())
}
}
public object FfiConverterBoolean: FfiConverter {
override fun lift(value: Byte): Boolean {
return value.toInt() != 0
}
override fun read(buf: ByteBuffer): Boolean {
return lift(buf.get())
}
override fun lower(value: Boolean): Byte {
return if (value) 1.toByte() else 0.toByte()
}
override fun allocationSize(value: Boolean) = 1UL
override fun write(value: Boolean, buf: ByteBuffer) {
buf.put(lower(value))
}
}
public object FfiConverterString: FfiConverter {
// Note: we don't inherit from FfiConverterRustBuffer, because we use a
// special encoding when lowering/lifting. We can use `RustBuffer.len` to
// store our length and avoid writing it out to the buffer.
override fun lift(value: RustBuffer.ByValue): String {
try {
val byteArr = ByteArray(value.len.toInt())
value.asByteBuffer()!!.get(byteArr)
return byteArr.toString(Charsets.UTF_8)
} finally {
RustBuffer.free(value)
}
}
override fun read(buf: ByteBuffer): String {
val len = buf.getInt()
val byteArr = ByteArray(len)
buf.get(byteArr)
return byteArr.toString(Charsets.UTF_8)
}
fun toUtf8(value: String): ByteBuffer {
// Make sure we don't have invalid UTF-16, check for lone surrogates.
return Charsets.UTF_8.newEncoder().run {
onMalformedInput(CodingErrorAction.REPORT)
encode(CharBuffer.wrap(value))
}
}
override fun lower(value: String): RustBuffer.ByValue {
val byteBuf = toUtf8(value)
// Ideally we'd pass these bytes to `ffi_bytebuffer_from_bytes`, but doing so would require us
// to copy them into a JNA `Memory`. So we might as well directly copy them into a `RustBuffer`.
val rbuf = RustBuffer.alloc(byteBuf.limit().toULong())
rbuf.asByteBuffer()!!.put(byteBuf)
return rbuf
}
// We aren't sure exactly how many bytes our string will be once it's UTF-8
// encoded. Allocate 3 bytes per UTF-16 code unit which will always be
// enough.
override fun allocationSize(value: String): ULong {
val sizeForLength = 4UL
val sizeForString = value.length.toULong() * 3UL
return sizeForLength + sizeForString
}
override fun write(value: String, buf: ByteBuffer) {
val byteBuf = toUtf8(value)
buf.putInt(byteBuf.limit())
buf.put(byteBuf)
}
}
public object FfiConverterByteArray: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): ByteArray {
val len = buf.getInt()
val byteArr = ByteArray(len)
buf.get(byteArr)
return byteArr
}
override fun allocationSize(value: ByteArray): ULong {
return 4UL + value.size.toULong()
}
override fun write(value: ByteArray, buf: ByteBuffer) {
buf.putInt(value.size)
buf.put(value)
}
}
public object FfiConverterDuration: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): java.time.Duration {
// Type mismatch (should be u64) but we check for overflow/underflow below
val seconds = buf.getLong()
// Type mismatch (should be u32) but we check for overflow/underflow below
val nanoseconds = buf.getInt().toLong()
if (seconds < 0) {
throw java.time.DateTimeException("Duration exceeds minimum or maximum value supported by uniffi")
}
if (nanoseconds < 0) {
throw java.time.DateTimeException("Duration nanoseconds exceed minimum or maximum supported by uniffi")
}
return java.time.Duration.ofSeconds(seconds, nanoseconds)
}
// 8 bytes for seconds, 4 bytes for nanoseconds
override fun allocationSize(value: java.time.Duration) = 12UL
override fun write(value: java.time.Duration, buf: ByteBuffer) {
if (value.seconds < 0) {
// Rust does not support negative Durations
throw IllegalArgumentException("Invalid duration, must be non-negative")
}
if (value.nano < 0) {
// Java docs provide guarantee that nano will always be positive, so this should be impossible
// See: https://docs.oracle.com/javase/8/docs/api/java/time/Duration.html
throw IllegalArgumentException("Invalid duration, nano value must be non-negative")
}
// Type mismatch (should be u64) but since Rust doesn't support negative durations we should be OK
buf.putLong(value.seconds)
// Type mismatch (should be u32) but since values will always be between 0 and 999,999,999 it should be OK
buf.putInt(value.nano)
}
}
// This template implements a class for working with a Rust struct via a Pointer/Arc
// to the live Rust struct on the other side of the FFI.
//
// Each instance implements core operations for working with the Rust `Arc` and the
// Kotlin Pointer to work with the live Rust struct on the other side of the FFI.
//
// There's some subtlety here, because we have to be careful not to operate on a Rust
// struct after it has been dropped, and because we must expose a public API for freeing
// theq Kotlin wrapper object in lieu of reliable finalizers. The core requirements are:
//
// * Each instance holds an opaque pointer to the underlying Rust struct.
// Method calls need to read this pointer from the object's state and pass it in to
// the Rust FFI.
//
// * When an instance is no longer needed, its pointer should be passed to a
// special destructor function provided by the Rust FFI, which will drop the
// underlying Rust struct.
//
// * Given an instance, calling code is expected to call the special
// `destroy` method in order to free it after use, either by calling it explicitly
// or by using a higher-level helper like the `use` method. Failing to do so risks
// leaking the underlying Rust struct.
//
// * We can't assume that calling code will do the right thing, and must be prepared
// to handle Kotlin method calls executing concurrently with or even after a call to
// `destroy`, and to handle multiple (possibly concurrent!) calls to `destroy`.
//
// * We must never allow Rust code to operate on the underlying Rust struct after
// the destructor has been called, and must never call the destructor more than once.
// Doing so may trigger memory unsafety.
//
// * To mitigate many of the risks of leaking memory and use-after-free unsafety, a `Cleaner`
// is implemented to call the destructor when the Kotlin object becomes unreachable.
// This is done in a background thread. This is not a panacea, and client code should be aware that
// 1. the thread may starve if some there are objects that have poorly performing
// `drop` methods or do significant work in their `drop` methods.
// 2. the thread is shared across the whole library. This can be tuned by using `android_cleaner = true`,
// or `android = true` in the [`kotlin` section of the `uniffi.toml` file](https://mozilla.github.io/uniffi-rs/kotlin/configuration.html).
//
// If we try to implement this with mutual exclusion on access to the pointer, there is the
// possibility of a race between a method call and a concurrent call to `destroy`:
//
// * Thread A starts a method call, reads the value of the pointer, but is interrupted
// before it can pass the pointer over the FFI to Rust.
// * Thread B calls `destroy` and frees the underlying Rust struct.
// * Thread A resumes, passing the already-read pointer value to Rust and triggering
// a use-after-free.
//
// One possible solution would be to use a `ReadWriteLock`, with each method call taking
// a read lock (and thus allowed to run concurrently) and the special `destroy` method
// taking a write lock (and thus blocking on live method calls). However, we aim not to
// generate methods with any hidden blocking semantics, and a `destroy` method that might
// block if called incorrectly seems to meet that bar.
//
// So, we achieve our goals by giving each instance an associated `AtomicLong` counter to track
// the number of in-flight method calls, and an `AtomicBoolean` flag to indicate whether `destroy`
// has been called. These are updated according to the following rules:
//
// * The initial value of the counter is 1, indicating a live object with no in-flight calls.
// The initial value for the flag is false.
//
// * At the start of each method call, we atomically check the counter.
// If it is 0 then the underlying Rust struct has already been destroyed and the call is aborted.
// If it is nonzero them we atomically increment it by 1 and proceed with the method call.
//
// * At the end of each method call, we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// * When `destroy` is called, we atomically flip the flag from false to true.
// If the flag was already true we silently fail.
// Otherwise we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// Astute readers may observe that this all sounds very similar to the way that Rust's `Arc` works,
// and indeed it is, with the addition of a flag to guard against multiple calls to `destroy`.
//
// The overall effect is that the underlying Rust struct is destroyed only when `destroy` has been
// called *and* all in-flight method calls have completed, avoiding violating any of the expectations
// of the underlying Rust code.
//
// This makes a cleaner a better alternative to _not_ calling `destroy()` as
// and when the object is finished with, but the abstraction is not perfect: if the Rust object's `drop`
// method is slow, and/or there are many objects to cleanup, and it's on a low end Android device, then the cleaner
// thread may be starved, and the app will leak memory.
//
// In this case, `destroy`ing manually may be a better solution.
//
// The cleaner can live side by side with the manual calling of `destroy`. In the order of responsiveness, uniffi objects
// with Rust peers are reclaimed:
//
// 1. By calling the `destroy` method of the object, which calls `rustObject.free()`. If that doesn't happen:
// 2. When the object becomes unreachable, AND the Cleaner thread gets to call `rustObject.free()`. If the thread is starved then:
// 3. The memory is reclaimed when the process terminates.
//
// [1] https://stackoverflow.com/questions/24376768/can-java-finalize-an-object-when-it-is-still-in-scope/24380219
//
// The cleaner interface for Object finalization code to run.
// This is the entry point to any implementation that we're using.
//
// The cleaner registers objects and returns cleanables, so now we are
// defining a `UniffiCleaner` with a `UniffiClenaer.Cleanable` to abstract the
// different implmentations available at compile time.
interface UniffiCleaner {
interface Cleanable {
fun clean()
}
fun register(value: Any, cleanUpTask: Runnable): UniffiCleaner.Cleanable
companion object
}
// The fallback Jna cleaner, which is available for both Android, and the JVM.
private class UniffiJnaCleaner : UniffiCleaner {
private val cleaner = com.sun.jna.internal.Cleaner.getCleaner()
override fun register(value: Any, cleanUpTask: Runnable): UniffiCleaner.Cleanable =
UniffiJnaCleanable(cleaner.register(value, cleanUpTask))
}
private class UniffiJnaCleanable(
private val cleanable: com.sun.jna.internal.Cleaner.Cleanable,
) : UniffiCleaner.Cleanable {
override fun clean() = cleanable.clean()
}
// We decide at uniffi binding generation time whether we were
// using Android or not.
// There are further runtime checks to chose the correct implementation
// of the cleaner.
private fun UniffiCleaner.Companion.create(): UniffiCleaner =
try {
// For safety's sake: if the library hasn't been run in android_cleaner = true
// mode, but is being run on Android, then we still need to think about
// Android API versions.
// So we check if java.lang.ref.Cleaner is there, and use that…
java.lang.Class.forName("java.lang.ref.Cleaner")
JavaLangRefCleaner()
} catch (e: ClassNotFoundException) {
// … otherwise, fallback to the JNA cleaner.
UniffiJnaCleaner()
}
private class JavaLangRefCleaner : UniffiCleaner {
val cleaner = java.lang.ref.Cleaner.create()
override fun register(value: Any, cleanUpTask: Runnable): UniffiCleaner.Cleanable =
JavaLangRefCleanable(cleaner.register(value, cleanUpTask))
}
private class JavaLangRefCleanable(
val cleanable: java.lang.ref.Cleaner.Cleanable
) : UniffiCleaner.Cleanable {
override fun clean() = cleanable.clean()
}
public interface CoreCryptoInterface {
/**
* See [core_crypto::context::CentralContext::add_members_to_conversation]
*/
suspend fun `addClientsToConversation`(`conversationId`: kotlin.ByteArray, `keyPackages`: List): MemberAddedMessages
/**
* See [core_crypto::context::CentralContext::clear_pending_commit]
*/
suspend fun `clearPendingCommit`(`conversationId`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::clear_pending_group_from_external_commit]
*/
suspend fun `clearPendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::clear_pending_proposal]
*/
suspend fun `clearPendingProposal`(`conversationId`: kotlin.ByteArray, `proposalRef`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::get_or_create_client_keypackages]
*/
suspend fun `clientKeypackages`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType, `amountRequested`: kotlin.UInt): List
/**
* See [core_crypto::mls::MlsCentral::client_public_key]
*/
suspend fun `clientPublicKey`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::client_valid_key_packages_count]
*/
suspend fun `clientValidKeypackagesCount`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType): kotlin.ULong
/**
* See [core_crypto::context::CentralContext::commit_accepted]
*/
suspend fun `commitAccepted`(`conversationId`: kotlin.ByteArray): List?
/**
* See [core_crypto::context::CentralContext::commit_pending_proposals]
*/
suspend fun `commitPendingProposals`(`conversationId`: kotlin.ByteArray): CommitBundle?
/**
* See [core_crypto::mls::MlsCentral::conversation_ciphersuite]
*/
suspend fun `conversationCiphersuite`(`conversationId`: kotlin.ByteArray): Ciphersuite
/**
* See [core_crypto::mls::MlsCentral::conversation_epoch]
*/
suspend fun `conversationEpoch`(`conversationId`: kotlin.ByteArray): kotlin.ULong
/**
* See [core_crypto::mls::MlsCentral::conversation_exists]
*/
suspend fun `conversationExists`(`conversationId`: kotlin.ByteArray): kotlin.Boolean
/**
* See [core_crypto::context::CentralContext::new_conversation]
*/
suspend fun `createConversation`(`conversationId`: kotlin.ByteArray, `creatorCredentialType`: MlsCredentialType, `config`: ConversationConfiguration)
/**
* See [core_crypto::context::CentralContext::decrypt_message]
*/
suspend fun `decryptMessage`(`conversationId`: kotlin.ByteArray, `payload`: kotlin.ByteArray): DecryptedMessage
/**
* See [core_crypto::context::CentralContext::delete_keypackages]
*/
suspend fun `deleteKeypackages`(`refs`: List)
/**
* See [core_crypto::context::CentralContext::e2ei_conversation_state]
*/
suspend fun `e2eiConversationState`(`conversationId`: kotlin.ByteArray): E2eiConversationState
suspend fun `e2eiDumpPkiEnv`(): E2eiDumpedPkiEnv?
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash]
*/
suspend fun `e2eiEnrollmentStash`(`enrollment`: E2eiEnrollment): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash_pop]
*/
suspend fun `e2eiEnrollmentStashPop`(`handle`: kotlin.ByteArray): E2eiEnrollment
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_enabled]
*/
suspend fun `e2eiIsEnabled`(`ciphersuite`: Ciphersuite): kotlin.Boolean
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_pki_env_setup]
*/
suspend fun `e2eiIsPkiEnvSetup`(): kotlin.Boolean
/**
* See [core_crypto::context::CentralContext::e2ei_mls_init_only]
*/
suspend fun `e2eiMlsInitOnly`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `nbKeyPackage`: kotlin.UInt?): List?
/**
* See [core_crypto::context::CentralContext::e2ei_new_activation_enrollment]
*/
suspend fun `e2eiNewActivationEnrollment`(`displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite): E2eiEnrollment
/**
* See [core_crypto::context::CentralContext::e2ei_new_enrollment]
*/
suspend fun `e2eiNewEnrollment`(`clientId`: kotlin.String, `displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite): E2eiEnrollment
/**
* See [core_crypto::context::CentralContext::e2ei_new_rotate_enrollment]
*/
suspend fun `e2eiNewRotateEnrollment`(`displayName`: kotlin.String?, `handle`: kotlin.String?, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite): E2eiEnrollment
/**
* See [core_crypto::context::CentralContext::e2ei_register_acme_ca]
*/
suspend fun `e2eiRegisterAcmeCa`(`trustAnchorPem`: kotlin.String)
/**
* See [core_crypto::context::CentralContext::e2ei_register_crl]
*/
suspend fun `e2eiRegisterCrl`(`crlDp`: kotlin.String, `crlDer`: kotlin.ByteArray): CrlRegistration
/**
* See [core_crypto::context::CentralContext::e2ei_register_intermediate_ca_pem]
*/
suspend fun `e2eiRegisterIntermediateCa`(`certPem`: kotlin.String): List?
/**
* See [core_crypto::context::CentralContext::e2ei_rotate]
*/
suspend fun `e2eiRotate`(`conversationId`: kotlin.ByteArray): CommitBundle
/**
* See [core_crypto::context::CentralContext::e2ei_rotate_all]
*/
suspend fun `e2eiRotateAll`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `newKeyPackagesCount`: kotlin.UInt): RotateBundle
/**
* See [core_crypto::context::CentralContext::encrypt_message]
*/
suspend fun `encryptMessage`(`conversationId`: kotlin.ByteArray, `message`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::mls::MlsCentral::export_secret_key]
*/
suspend fun `exportSecretKey`(`conversationId`: kotlin.ByteArray, `keyLength`: kotlin.UInt): kotlin.ByteArray
/**
* See [core_crypto::mls::MlsCentral::get_client_ids]
*/
suspend fun `getClientIds`(`conversationId`: kotlin.ByteArray): List
/**
* See [core_crypto::mls::MlsCentral::get_credential_in_use]
*/
suspend fun `getCredentialInUse`(`groupInfo`: kotlin.ByteArray, `credentialType`: MlsCredentialType): E2eiConversationState
/**
* See [core_crypto::mls::MlsCentral::get_device_identities]
*/
suspend fun `getDeviceIdentities`(`conversationId`: kotlin.ByteArray, `deviceIds`: List): List
/**
* See [core_crypto::mls::MlsCentral::get_external_sender]
*/
suspend fun `getExternalSender`(`conversationId`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::mls::MlsCentral::get_user_identities]
*/
suspend fun `getUserIdentities`(`conversationId`: kotlin.ByteArray, `userIds`: List): Map>
/**
* See [core_crypto::context::CentralContext::join_by_external_commit]
*/
suspend fun `joinByExternalCommit`(`groupInfo`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration, `credentialType`: MlsCredentialType): ConversationInitBundle
/**
* See [core_crypto::context::CentralContext::mark_conversation_as_child_of]
*/
suspend fun `markConversationAsChildOf`(`childId`: kotlin.ByteArray, `parentId`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::merge_pending_group_from_external_commit]
*/
suspend fun `mergePendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray): List?
/**
* See [core_crypto::context::CentralContext::mls_generate_keypairs]
*/
suspend fun `mlsGenerateKeypairs`(`ciphersuites`: Ciphersuites): List
/**
* See [core_crypto::context::CentralContext::mls_init]
*/
suspend fun `mlsInit`(`clientId`: ClientId, `ciphersuites`: Ciphersuites, `nbKeyPackage`: kotlin.UInt?)
/**
* See [core_crypto::context::CentralContext::mls_init_with_client_id]
*/
suspend fun `mlsInitWithClientId`(`clientId`: ClientId, `tmpClientIds`: List, `ciphersuites`: Ciphersuites)
/**
* See [core_crypto::context::CentralContext::new_add_proposal]
*/
suspend fun `newAddProposal`(`conversationId`: kotlin.ByteArray, `keypackage`: kotlin.ByteArray): ProposalBundle
/**
* See [core_crypto::context::CentralContext::new_external_add_proposal]
*/
suspend fun `newExternalAddProposal`(`conversationId`: kotlin.ByteArray, `epoch`: kotlin.ULong, `ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::new_remove_proposal]
*/
suspend fun `newRemoveProposal`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId): ProposalBundle
/**
* See [core_crypto::context::CentralContext::new_update_proposal]
*/
suspend fun `newUpdateProposal`(`conversationId`: kotlin.ByteArray): ProposalBundle
/**
* See [core_crypto::context::CentralContext::process_raw_welcome_message]
*/
suspend fun `processWelcomeMessage`(`welcomeMessage`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration): WelcomeBundle
/**
* See [core_crypto::proteus::ProteusCentral::cryptobox_migrate]
*/
suspend fun `proteusCryptoboxMigrate`(`path`: kotlin.String)
/**
* See [core_crypto::proteus::ProteusCentral::decrypt]
*/
suspend fun `proteusDecrypt`(`sessionId`: kotlin.String, `ciphertext`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::proteus::ProteusCentral::encrypt]
*/
suspend fun `proteusEncrypt`(`sessionId`: kotlin.String, `plaintext`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::proteus::ProteusCentral::encrypt_batched]
*/
suspend fun `proteusEncryptBatched`(`sessions`: List, `plaintext`: kotlin.ByteArray): Map
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint]
*/
suspend fun `proteusFingerprint`(): kotlin.String
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_local]
*/
suspend fun `proteusFingerprintLocal`(`sessionId`: kotlin.String): kotlin.String
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_prekeybundle]
* NOTE: uniffi doesn't support associated functions, so we have to have the self here
*/
fun `proteusFingerprintPrekeybundle`(`prekey`: kotlin.ByteArray): kotlin.String
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_remote]
*/
suspend fun `proteusFingerprintRemote`(`sessionId`: kotlin.String): kotlin.String
/**
* See [core_crypto::proteus::ProteusCentral::try_new]
*/
suspend fun `proteusInit`()
/**
* Returns the latest proteus error code. If 0, no error has occured
*
* NOTE: This will clear the last error code.
*/
fun `proteusLastErrorCode`(): kotlin.UInt
/**
* See [core_crypto::proteus::ProteusCentral::last_resort_prekey]
*/
suspend fun `proteusLastResortPrekey`(): kotlin.ByteArray
/**
* See [core_crypto::proteus::ProteusCentral::last_resort_prekey_id]
*/
fun `proteusLastResortPrekeyId`(): kotlin.UShort
/**
* See [core_crypto::proteus::ProteusCentral::new_prekey]
*/
suspend fun `proteusNewPrekey`(`prekeyId`: kotlin.UShort): kotlin.ByteArray
/**
* See [core_crypto::proteus::ProteusCentral::new_prekey_auto]
*/
suspend fun `proteusNewPrekeyAuto`(): ProteusAutoPrekeyBundle
/**
* See [core_crypto::proteus::ProteusCentral::session_delete]
*/
suspend fun `proteusSessionDelete`(`sessionId`: kotlin.String)
/**
* See [core_crypto::proteus::ProteusCentral::session_exists]
*/
suspend fun `proteusSessionExists`(`sessionId`: kotlin.String): kotlin.Boolean
/**
* See [core_crypto::proteus::ProteusCentral::session_from_message]
*/
suspend fun `proteusSessionFromMessage`(`sessionId`: kotlin.String, `envelope`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::proteus::ProteusCentral::session_from_prekey]
*/
suspend fun `proteusSessionFromPrekey`(`sessionId`: kotlin.String, `prekey`: kotlin.ByteArray)
/**
* See [core_crypto::proteus::ProteusCentral::session_save]
* **Note**: This isn't usually needed as persisting sessions happens automatically when decrypting/encrypting messages and initializing Sessions
*/
suspend fun `proteusSessionSave`(`sessionId`: kotlin.String)
/**
* See [core_crypto::mls::MlsCentral::random_bytes]
*/
suspend fun `randomBytes`(`len`: kotlin.UInt): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::remove_members_from_conversation]
*/
suspend fun `removeClientsFromConversation`(`conversationId`: kotlin.ByteArray, `clients`: List): CommitBundle
/**
* see [core_crypto::prelude::MlsCryptoProvider::reseed]
*/
suspend fun `reseedRng`(`seed`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::proteus_reload_sessions]
*/
suspend fun `restoreFromDisk`()
/**
* See [core_crypto::mls::MlsCentral::callbacks]
*/
suspend fun `setCallbacks`(`callbacks`: CoreCryptoCallbacks)
/**
* Starts a new transaction in Core Crypto. If the callback succeeds, it will be committed,
* otherwise, every operation performed with the context will be discarded.
*/
suspend fun `transaction`(`command`: CoreCryptoCommand)
/**
* See [core_crypto::mls::MlsCentral::close]
*/
suspend fun `unload`()
/**
* See [core_crypto::context::CentralContext::update_keying_material]
*/
suspend fun `updateKeyingMaterial`(`conversationId`: kotlin.ByteArray): CommitBundle
/**
* See [core_crypto::mls::MlsCentral::wipe]
*/
suspend fun `wipe`()
/**
* see [core_crypto::context::CentralContext::wipe_conversation]
*/
suspend fun `wipeConversation`(`conversationId`: kotlin.ByteArray)
companion object
}
open class CoreCrypto: Disposable, AutoCloseable, CoreCryptoInterface {
constructor(pointer: Pointer) {
this.pointer = pointer
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
/**
* This constructor can be used to instantiate a fake object. Only used for tests. Any
* attempt to actually use an object constructed this way will fail as there is no
* connected Rust object.
*/
@Suppress("UNUSED_PARAMETER")
constructor(noPointer: NoPointer) {
this.pointer = null
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
// Note no constructor generated for this object as it is async.
protected val pointer: Pointer?
protected val cleanable: UniffiCleaner.Cleanable
private val wasDestroyed = AtomicBoolean(false)
private val callCounter = AtomicLong(1)
override fun destroy() {
// Only allow a single call to this method.
// TODO: maybe we should log a warning if called more than once?
if (this.wasDestroyed.compareAndSet(false, true)) {
// This decrement always matches the initial count of 1 given at creation time.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
@Synchronized
override fun close() {
this.destroy()
}
internal inline fun callWithPointer(block: (ptr: Pointer) -> R): R {
// Check and increment the call counter, to keep the object alive.
// This needs a compare-and-set retry loop in case of concurrent updates.
do {
val c = this.callCounter.get()
if (c == 0L) {
throw IllegalStateException("${this.javaClass.simpleName} object has already been destroyed")
}
if (c == Long.MAX_VALUE) {
throw IllegalStateException("${this.javaClass.simpleName} call counter would overflow")
}
} while (! this.callCounter.compareAndSet(c, c + 1L))
// Now we can safely do the method call without the pointer being freed concurrently.
try {
return block(this.uniffiClonePointer())
} finally {
// This decrement always matches the increment we performed above.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
// Use a static inner class instead of a closure so as not to accidentally
// capture `this` as part of the cleanable's action.
private class UniffiCleanAction(private val pointer: Pointer?) : Runnable {
override fun run() {
pointer?.let { ptr ->
uniffiRustCall { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_free_corecrypto(ptr, status)
}
}
}
}
fun uniffiClonePointer(): Pointer {
return uniffiRustCall() { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_clone_corecrypto(pointer!!, status)
}
}
/**
* See [core_crypto::context::CentralContext::add_members_to_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `addClientsToConversation`(`conversationId`: kotlin.ByteArray, `keyPackages`: List) : MemberAddedMessages {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_add_clients_to_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceByteArray.lower(`keyPackages`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeMemberAddedMessages.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::clear_pending_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clearPendingCommit`(`conversationId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_clear_pending_commit(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::clear_pending_group_from_external_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clearPendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_clear_pending_group_from_external_commit(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::clear_pending_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clearPendingProposal`(`conversationId`: kotlin.ByteArray, `proposalRef`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_clear_pending_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`proposalRef`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::get_or_create_client_keypackages]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clientKeypackages`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType, `amountRequested`: kotlin.UInt) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_client_keypackages(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),FfiConverterUInt.lower(`amountRequested`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::client_public_key]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clientPublicKey`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_client_public_key(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::client_valid_key_packages_count]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clientValidKeypackagesCount`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType) : kotlin.ULong {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_client_valid_keypackages_count(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_u64(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_u64(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_u64(future) },
// lift function
{ FfiConverterULong.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::commit_accepted]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `commitAccepted`(`conversationId`: kotlin.ByteArray) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_commit_accepted(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceTypeBufferedDecryptedMessage.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::commit_pending_proposals]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `commitPendingProposals`(`conversationId`: kotlin.ByteArray) : CommitBundle? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_commit_pending_proposals(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::conversation_ciphersuite]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `conversationCiphersuite`(`conversationId`: kotlin.ByteArray) : Ciphersuite {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_conversation_ciphersuite(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_u16(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_u16(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_u16(future) },
// lift function
{ FfiConverterTypeCiphersuite.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::conversation_epoch]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `conversationEpoch`(`conversationId`: kotlin.ByteArray) : kotlin.ULong {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_conversation_epoch(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_u64(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_u64(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_u64(future) },
// lift function
{ FfiConverterULong.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::conversation_exists]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `conversationExists`(`conversationId`: kotlin.ByteArray) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_conversation_exists(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `createConversation`(`conversationId`: kotlin.ByteArray, `creatorCredentialType`: MlsCredentialType, `config`: ConversationConfiguration) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_create_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterTypeMlsCredentialType.lower(`creatorCredentialType`),FfiConverterTypeConversationConfiguration.lower(`config`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::decrypt_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `decryptMessage`(`conversationId`: kotlin.ByteArray, `payload`: kotlin.ByteArray) : DecryptedMessage {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_decrypt_message(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`payload`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeDecryptedMessage.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::delete_keypackages]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `deleteKeypackages`(`refs`: List) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_delete_keypackages(
thisPtr,
FfiConverterSequenceByteArray.lower(`refs`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_conversation_state]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiConversationState`(`conversationId`: kotlin.ByteArray) : E2eiConversationState {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_conversation_state(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeE2eiConversationState.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiDumpPkiEnv`() : E2eiDumpedPkiEnv? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_dump_pki_env(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalTypeE2eiDumpedPkiEnv.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiEnrollmentStash`(`enrollment`: E2eiEnrollment) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_enrollment_stash(
thisPtr,
FfiConverterTypeE2eiEnrollment.lower(`enrollment`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash_pop]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiEnrollmentStashPop`(`handle`: kotlin.ByteArray) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_enrollment_stash_pop(
thisPtr,
FfiConverterByteArray.lower(`handle`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_enabled]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiIsEnabled`(`ciphersuite`: Ciphersuite) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_is_enabled(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_pki_env_setup]
*/
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiIsPkiEnvSetup`() : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_is_pki_env_setup(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
UniffiNullRustCallStatusErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_mls_init_only]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiMlsInitOnly`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `nbKeyPackage`: kotlin.UInt?) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_mls_init_only(
thisPtr,
FfiConverterTypeE2eiEnrollment.lower(`enrollment`),FfiConverterString.lower(`certificateChain`),FfiConverterOptionalUInt.lower(`nbKeyPackage`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_new_activation_enrollment]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiNewActivationEnrollment`(`displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_new_activation_enrollment(
thisPtr,
FfiConverterString.lower(`displayName`),FfiConverterString.lower(`handle`),FfiConverterOptionalString.lower(`team`),FfiConverterUInt.lower(`expirySec`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_new_enrollment]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiNewEnrollment`(`clientId`: kotlin.String, `displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_new_enrollment(
thisPtr,
FfiConverterString.lower(`clientId`),FfiConverterString.lower(`displayName`),FfiConverterString.lower(`handle`),FfiConverterOptionalString.lower(`team`),FfiConverterUInt.lower(`expirySec`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_new_rotate_enrollment]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiNewRotateEnrollment`(`displayName`: kotlin.String?, `handle`: kotlin.String?, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_new_rotate_enrollment(
thisPtr,
FfiConverterOptionalString.lower(`displayName`),FfiConverterOptionalString.lower(`handle`),FfiConverterOptionalString.lower(`team`),FfiConverterUInt.lower(`expirySec`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_register_acme_ca]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRegisterAcmeCa`(`trustAnchorPem`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_register_acme_ca(
thisPtr,
FfiConverterString.lower(`trustAnchorPem`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_register_crl]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRegisterCrl`(`crlDp`: kotlin.String, `crlDer`: kotlin.ByteArray) : CrlRegistration {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_register_crl(
thisPtr,
FfiConverterString.lower(`crlDp`),FfiConverterByteArray.lower(`crlDer`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCrlRegistration.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_register_intermediate_ca_pem]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRegisterIntermediateCa`(`certPem`: kotlin.String) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_register_intermediate_ca(
thisPtr,
FfiConverterString.lower(`certPem`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_rotate]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRotate`(`conversationId`: kotlin.ByteArray) : CommitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_rotate(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_rotate_all]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRotateAll`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `newKeyPackagesCount`: kotlin.UInt) : RotateBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_e2ei_rotate_all(
thisPtr,
FfiConverterTypeE2eiEnrollment.lower(`enrollment`),FfiConverterString.lower(`certificateChain`),FfiConverterUInt.lower(`newKeyPackagesCount`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeRotateBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::encrypt_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `encryptMessage`(`conversationId`: kotlin.ByteArray, `message`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_encrypt_message(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`message`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::export_secret_key]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `exportSecretKey`(`conversationId`: kotlin.ByteArray, `keyLength`: kotlin.UInt) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_export_secret_key(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterUInt.lower(`keyLength`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_client_ids]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getClientIds`(`conversationId`: kotlin.ByteArray) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_get_client_ids(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceTypeClientId.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_credential_in_use]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getCredentialInUse`(`groupInfo`: kotlin.ByteArray, `credentialType`: MlsCredentialType) : E2eiConversationState {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_get_credential_in_use(
thisPtr,
FfiConverterByteArray.lower(`groupInfo`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeE2eiConversationState.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_device_identities]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getDeviceIdentities`(`conversationId`: kotlin.ByteArray, `deviceIds`: List) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_get_device_identities(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceTypeClientId.lower(`deviceIds`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceTypeWireIdentity.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_external_sender]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getExternalSender`(`conversationId`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_get_external_sender(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_user_identities]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getUserIdentities`(`conversationId`: kotlin.ByteArray, `userIds`: List) : Map> {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_get_user_identities(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceString.lower(`userIds`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterMapStringSequenceTypeWireIdentity.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::join_by_external_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `joinByExternalCommit`(`groupInfo`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration, `credentialType`: MlsCredentialType) : ConversationInitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_join_by_external_commit(
thisPtr,
FfiConverterByteArray.lower(`groupInfo`),FfiConverterTypeCustomConfiguration.lower(`customConfiguration`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeConversationInitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mark_conversation_as_child_of]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `markConversationAsChildOf`(`childId`: kotlin.ByteArray, `parentId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_mark_conversation_as_child_of(
thisPtr,
FfiConverterByteArray.lower(`childId`),FfiConverterByteArray.lower(`parentId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::merge_pending_group_from_external_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mergePendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_merge_pending_group_from_external_commit(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceTypeBufferedDecryptedMessage.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mls_generate_keypairs]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mlsGenerateKeypairs`(`ciphersuites`: Ciphersuites) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_mls_generate_keypairs(
thisPtr,
FfiConverterTypeCiphersuites.lower(`ciphersuites`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceTypeClientId.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mls_init]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mlsInit`(`clientId`: ClientId, `ciphersuites`: Ciphersuites, `nbKeyPackage`: kotlin.UInt?) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_mls_init(
thisPtr,
FfiConverterTypeClientId.lower(`clientId`),FfiConverterTypeCiphersuites.lower(`ciphersuites`),FfiConverterOptionalUInt.lower(`nbKeyPackage`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mls_init_with_client_id]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mlsInitWithClientId`(`clientId`: ClientId, `tmpClientIds`: List, `ciphersuites`: Ciphersuites) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_mls_init_with_client_id(
thisPtr,
FfiConverterTypeClientId.lower(`clientId`),FfiConverterSequenceTypeClientId.lower(`tmpClientIds`),FfiConverterTypeCiphersuites.lower(`ciphersuites`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_add_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newAddProposal`(`conversationId`: kotlin.ByteArray, `keypackage`: kotlin.ByteArray) : ProposalBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_new_add_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`keypackage`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProposalBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_external_add_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newExternalAddProposal`(`conversationId`: kotlin.ByteArray, `epoch`: kotlin.ULong, `ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_new_external_add_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterULong.lower(`epoch`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_remove_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newRemoveProposal`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId) : ProposalBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_new_remove_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterTypeClientId.lower(`clientId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProposalBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_update_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newUpdateProposal`(`conversationId`: kotlin.ByteArray) : ProposalBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_new_update_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProposalBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::process_raw_welcome_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `processWelcomeMessage`(`welcomeMessage`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration) : WelcomeBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_process_welcome_message(
thisPtr,
FfiConverterByteArray.lower(`welcomeMessage`),FfiConverterTypeCustomConfiguration.lower(`customConfiguration`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeWelcomeBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::cryptobox_migrate]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusCryptoboxMigrate`(`path`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_cryptobox_migrate(
thisPtr,
FfiConverterString.lower(`path`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::decrypt]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusDecrypt`(`sessionId`: kotlin.String, `ciphertext`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_decrypt(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`ciphertext`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::encrypt]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusEncrypt`(`sessionId`: kotlin.String, `plaintext`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_encrypt(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`plaintext`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::encrypt_batched]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusEncryptBatched`(`sessions`: List, `plaintext`: kotlin.ByteArray) : Map {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_encrypt_batched(
thisPtr,
FfiConverterSequenceString.lower(`sessions`),FfiConverterByteArray.lower(`plaintext`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterMapStringByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusFingerprint`() : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_local]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusFingerprintLocal`(`sessionId`: kotlin.String) : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint_local(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_prekeybundle]
* NOTE: uniffi doesn't support associated functions, so we have to have the self here
*/
@Throws(CoreCryptoException::class)override fun `proteusFingerprintPrekeybundle`(`prekey`: kotlin.ByteArray): kotlin.String {
return FfiConverterString.lift(
callWithPointer {
uniffiRustCallWithError(CoreCryptoException) { _status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint_prekeybundle(
it, FfiConverterByteArray.lower(`prekey`),_status)
}
}
)
}
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_remote]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusFingerprintRemote`(`sessionId`: kotlin.String) : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_fingerprint_remote(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::try_new]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusInit`() {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_init(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* Returns the latest proteus error code. If 0, no error has occured
*
* NOTE: This will clear the last error code.
*/override fun `proteusLastErrorCode`(): kotlin.UInt {
return FfiConverterUInt.lift(
callWithPointer {
uniffiRustCall() { _status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_last_error_code(
it, _status)
}
}
)
}
/**
* See [core_crypto::proteus::ProteusCentral::last_resort_prekey]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusLastResortPrekey`() : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_last_resort_prekey(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::last_resort_prekey_id]
*/
@Throws(CoreCryptoException::class)override fun `proteusLastResortPrekeyId`(): kotlin.UShort {
return FfiConverterUShort.lift(
callWithPointer {
uniffiRustCallWithError(CoreCryptoException) { _status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_last_resort_prekey_id(
it, _status)
}
}
)
}
/**
* See [core_crypto::proteus::ProteusCentral::new_prekey]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusNewPrekey`(`prekeyId`: kotlin.UShort) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_new_prekey(
thisPtr,
FfiConverterUShort.lower(`prekeyId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::new_prekey_auto]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusNewPrekeyAuto`() : ProteusAutoPrekeyBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_new_prekey_auto(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProteusAutoPrekeyBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::session_delete]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionDelete`(`sessionId`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_delete(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::session_exists]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionExists`(`sessionId`: kotlin.String) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_exists(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::session_from_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionFromMessage`(`sessionId`: kotlin.String, `envelope`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_from_message(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`envelope`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::session_from_prekey]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionFromPrekey`(`sessionId`: kotlin.String, `prekey`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_from_prekey(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`prekey`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::session_save]
* **Note**: This isn't usually needed as persisting sessions happens automatically when decrypting/encrypting messages and initializing Sessions
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionSave`(`sessionId`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_proteus_session_save(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::random_bytes]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `randomBytes`(`len`: kotlin.UInt) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_random_bytes(
thisPtr,
FfiConverterUInt.lower(`len`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::remove_members_from_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `removeClientsFromConversation`(`conversationId`: kotlin.ByteArray, `clients`: List) : CommitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_remove_clients_from_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceTypeClientId.lower(`clients`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* see [core_crypto::prelude::MlsCryptoProvider::reseed]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `reseedRng`(`seed`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_reseed_rng(
thisPtr,
FfiConverterByteArray.lower(`seed`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_reload_sessions]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `restoreFromDisk`() {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_restore_from_disk(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::callbacks]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `setCallbacks`(`callbacks`: CoreCryptoCallbacks) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_set_callbacks(
thisPtr,
FfiConverterTypeCoreCryptoCallbacks.lower(`callbacks`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* Starts a new transaction in Core Crypto. If the callback succeeds, it will be committed,
* otherwise, every operation performed with the context will be discarded.
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `transaction`(`command`: CoreCryptoCommand) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_transaction(
thisPtr,
FfiConverterTypeCoreCryptoCommand.lower(`command`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::close]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `unload`() {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_unload(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::update_keying_material]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `updateKeyingMaterial`(`conversationId`: kotlin.ByteArray) : CommitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_update_keying_material(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::wipe]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `wipe`() {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_wipe(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* see [core_crypto::context::CentralContext::wipe_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `wipeConversation`(`conversationId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecrypto_wipe_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
companion object
}
public object FfiConverterTypeCoreCrypto: FfiConverter {
override fun lower(value: CoreCrypto): Pointer {
return value.uniffiClonePointer()
}
override fun lift(value: Pointer): CoreCrypto {
return CoreCrypto(value)
}
override fun read(buf: ByteBuffer): CoreCrypto {
// The Rust code always writes pointers as 8 bytes, and will
// fail to compile if they don't fit.
return lift(Pointer(buf.getLong()))
}
override fun allocationSize(value: CoreCrypto) = 8UL
override fun write(value: CoreCrypto, buf: ByteBuffer) {
// The Rust code always expects pointers written as 8 bytes,
// and will fail to compile if they don't fit.
buf.putLong(Pointer.nativeValue(lower(value)))
}
}
// This template implements a class for working with a Rust struct via a Pointer/Arc
// to the live Rust struct on the other side of the FFI.
//
// Each instance implements core operations for working with the Rust `Arc` and the
// Kotlin Pointer to work with the live Rust struct on the other side of the FFI.
//
// There's some subtlety here, because we have to be careful not to operate on a Rust
// struct after it has been dropped, and because we must expose a public API for freeing
// theq Kotlin wrapper object in lieu of reliable finalizers. The core requirements are:
//
// * Each instance holds an opaque pointer to the underlying Rust struct.
// Method calls need to read this pointer from the object's state and pass it in to
// the Rust FFI.
//
// * When an instance is no longer needed, its pointer should be passed to a
// special destructor function provided by the Rust FFI, which will drop the
// underlying Rust struct.
//
// * Given an instance, calling code is expected to call the special
// `destroy` method in order to free it after use, either by calling it explicitly
// or by using a higher-level helper like the `use` method. Failing to do so risks
// leaking the underlying Rust struct.
//
// * We can't assume that calling code will do the right thing, and must be prepared
// to handle Kotlin method calls executing concurrently with or even after a call to
// `destroy`, and to handle multiple (possibly concurrent!) calls to `destroy`.
//
// * We must never allow Rust code to operate on the underlying Rust struct after
// the destructor has been called, and must never call the destructor more than once.
// Doing so may trigger memory unsafety.
//
// * To mitigate many of the risks of leaking memory and use-after-free unsafety, a `Cleaner`
// is implemented to call the destructor when the Kotlin object becomes unreachable.
// This is done in a background thread. This is not a panacea, and client code should be aware that
// 1. the thread may starve if some there are objects that have poorly performing
// `drop` methods or do significant work in their `drop` methods.
// 2. the thread is shared across the whole library. This can be tuned by using `android_cleaner = true`,
// or `android = true` in the [`kotlin` section of the `uniffi.toml` file](https://mozilla.github.io/uniffi-rs/kotlin/configuration.html).
//
// If we try to implement this with mutual exclusion on access to the pointer, there is the
// possibility of a race between a method call and a concurrent call to `destroy`:
//
// * Thread A starts a method call, reads the value of the pointer, but is interrupted
// before it can pass the pointer over the FFI to Rust.
// * Thread B calls `destroy` and frees the underlying Rust struct.
// * Thread A resumes, passing the already-read pointer value to Rust and triggering
// a use-after-free.
//
// One possible solution would be to use a `ReadWriteLock`, with each method call taking
// a read lock (and thus allowed to run concurrently) and the special `destroy` method
// taking a write lock (and thus blocking on live method calls). However, we aim not to
// generate methods with any hidden blocking semantics, and a `destroy` method that might
// block if called incorrectly seems to meet that bar.
//
// So, we achieve our goals by giving each instance an associated `AtomicLong` counter to track
// the number of in-flight method calls, and an `AtomicBoolean` flag to indicate whether `destroy`
// has been called. These are updated according to the following rules:
//
// * The initial value of the counter is 1, indicating a live object with no in-flight calls.
// The initial value for the flag is false.
//
// * At the start of each method call, we atomically check the counter.
// If it is 0 then the underlying Rust struct has already been destroyed and the call is aborted.
// If it is nonzero them we atomically increment it by 1 and proceed with the method call.
//
// * At the end of each method call, we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// * When `destroy` is called, we atomically flip the flag from false to true.
// If the flag was already true we silently fail.
// Otherwise we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// Astute readers may observe that this all sounds very similar to the way that Rust's `Arc` works,
// and indeed it is, with the addition of a flag to guard against multiple calls to `destroy`.
//
// The overall effect is that the underlying Rust struct is destroyed only when `destroy` has been
// called *and* all in-flight method calls have completed, avoiding violating any of the expectations
// of the underlying Rust code.
//
// This makes a cleaner a better alternative to _not_ calling `destroy()` as
// and when the object is finished with, but the abstraction is not perfect: if the Rust object's `drop`
// method is slow, and/or there are many objects to cleanup, and it's on a low end Android device, then the cleaner
// thread may be starved, and the app will leak memory.
//
// In this case, `destroy`ing manually may be a better solution.
//
// The cleaner can live side by side with the manual calling of `destroy`. In the order of responsiveness, uniffi objects
// with Rust peers are reclaimed:
//
// 1. By calling the `destroy` method of the object, which calls `rustObject.free()`. If that doesn't happen:
// 2. When the object becomes unreachable, AND the Cleaner thread gets to call `rustObject.free()`. If the thread is starved then:
// 3. The memory is reclaimed when the process terminates.
//
// [1] https://stackoverflow.com/questions/24376768/can-java-finalize-an-object-when-it-is-still-in-scope/24380219
//
/**
* This is needed instead of the original trait ([core_crypto::CoreCryptoCallbacks]) to use the
* custom type [ClientId], that UniFFi can handle.
*/
public interface CoreCryptoCallbacks {
suspend fun `authorize`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId): kotlin.Boolean
suspend fun `userAuthorize`(`conversationId`: kotlin.ByteArray, `externalClientId`: ClientId, `existingClients`: List): kotlin.Boolean
suspend fun `clientIsExistingGroupUser`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId, `existingClients`: List, `parentConversationClients`: List?): kotlin.Boolean
companion object
}
/**
* This is needed instead of the original trait ([core_crypto::CoreCryptoCallbacks]) to use the
* custom type [ClientId], that UniFFi can handle.
*/
open class CoreCryptoCallbacksImpl: Disposable, AutoCloseable, CoreCryptoCallbacks {
constructor(pointer: Pointer) {
this.pointer = pointer
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
/**
* This constructor can be used to instantiate a fake object. Only used for tests. Any
* attempt to actually use an object constructed this way will fail as there is no
* connected Rust object.
*/
@Suppress("UNUSED_PARAMETER")
constructor(noPointer: NoPointer) {
this.pointer = null
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
protected val pointer: Pointer?
protected val cleanable: UniffiCleaner.Cleanable
private val wasDestroyed = AtomicBoolean(false)
private val callCounter = AtomicLong(1)
override fun destroy() {
// Only allow a single call to this method.
// TODO: maybe we should log a warning if called more than once?
if (this.wasDestroyed.compareAndSet(false, true)) {
// This decrement always matches the initial count of 1 given at creation time.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
@Synchronized
override fun close() {
this.destroy()
}
internal inline fun callWithPointer(block: (ptr: Pointer) -> R): R {
// Check and increment the call counter, to keep the object alive.
// This needs a compare-and-set retry loop in case of concurrent updates.
do {
val c = this.callCounter.get()
if (c == 0L) {
throw IllegalStateException("${this.javaClass.simpleName} object has already been destroyed")
}
if (c == Long.MAX_VALUE) {
throw IllegalStateException("${this.javaClass.simpleName} call counter would overflow")
}
} while (! this.callCounter.compareAndSet(c, c + 1L))
// Now we can safely do the method call without the pointer being freed concurrently.
try {
return block(this.uniffiClonePointer())
} finally {
// This decrement always matches the increment we performed above.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
// Use a static inner class instead of a closure so as not to accidentally
// capture `this` as part of the cleanable's action.
private class UniffiCleanAction(private val pointer: Pointer?) : Runnable {
override fun run() {
pointer?.let { ptr ->
uniffiRustCall { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_free_corecryptocallbacks(ptr, status)
}
}
}
}
fun uniffiClonePointer(): Pointer {
return uniffiRustCall() { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_clone_corecryptocallbacks(pointer!!, status)
}
}
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `authorize`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocallbacks_authorize(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterTypeClientId.lower(`clientId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
UniffiNullRustCallStatusErrorHandler,
)
}
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `userAuthorize`(`conversationId`: kotlin.ByteArray, `externalClientId`: ClientId, `existingClients`: List) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocallbacks_user_authorize(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterTypeClientId.lower(`externalClientId`),FfiConverterSequenceTypeClientId.lower(`existingClients`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
UniffiNullRustCallStatusErrorHandler,
)
}
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clientIsExistingGroupUser`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId, `existingClients`: List, `parentConversationClients`: List?) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocallbacks_client_is_existing_group_user(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterTypeClientId.lower(`clientId`),FfiConverterSequenceTypeClientId.lower(`existingClients`),FfiConverterOptionalSequenceTypeClientId.lower(`parentConversationClients`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
UniffiNullRustCallStatusErrorHandler,
)
}
companion object
}
// Magic number for the Rust proxy to call using the same mechanism as every other method,
// to free the callback once it's dropped by Rust.
internal const val IDX_CALLBACK_FREE = 0
// Callback return codes
internal const val UNIFFI_CALLBACK_SUCCESS = 0
internal const val UNIFFI_CALLBACK_ERROR = 1
internal const val UNIFFI_CALLBACK_UNEXPECTED_ERROR = 2
public abstract class FfiConverterCallbackInterface: FfiConverter {
internal val handleMap = UniffiHandleMap()
internal fun drop(handle: Long) {
handleMap.remove(handle)
}
override fun lift(value: Long): CallbackInterface {
return handleMap.get(value)
}
override fun read(buf: ByteBuffer) = lift(buf.getLong())
override fun lower(value: CallbackInterface) = handleMap.insert(value)
override fun allocationSize(value: CallbackInterface) = 8UL
override fun write(value: CallbackInterface, buf: ByteBuffer) {
buf.putLong(lower(value))
}
}
// Put the implementation in an object so we don't pollute the top-level namespace
internal object uniffiCallbackInterfaceCoreCryptoCallbacks {
internal object `authorize`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod0 {
override fun callback(`uniffiHandle`: Long,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,`uniffiFutureCallback`: UniffiForeignFutureCompleteI8,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,) {
val uniffiObj = FfiConverterTypeCoreCryptoCallbacks.handleMap.get(uniffiHandle)
val makeCall = suspend { ->
uniffiObj.`authorize`(
FfiConverterByteArray.lift(`conversationId`),
FfiConverterTypeClientId.lift(`clientId`),
)
}
val uniffiHandleSuccess = { returnValue: kotlin.Boolean ->
val uniffiResult = UniffiForeignFutureStructI8.UniffiByValue(
FfiConverterBoolean.lower(returnValue),
UniffiRustCallStatus.ByValue()
)
uniffiResult.write()
uniffiFutureCallback.callback(uniffiCallbackData, uniffiResult)
}
val uniffiHandleError = { callStatus: UniffiRustCallStatus.ByValue ->
uniffiFutureCallback.callback(
uniffiCallbackData,
UniffiForeignFutureStructI8.UniffiByValue(
0.toByte(),
callStatus,
),
)
}
uniffiOutReturn.uniffiSetValue(
uniffiTraitInterfaceCallAsync(
makeCall,
uniffiHandleSuccess,
uniffiHandleError
)
)
}
}
internal object `userAuthorize`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod1 {
override fun callback(`uniffiHandle`: Long,`conversationId`: RustBuffer.ByValue,`externalClientId`: RustBuffer.ByValue,`existingClients`: RustBuffer.ByValue,`uniffiFutureCallback`: UniffiForeignFutureCompleteI8,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,) {
val uniffiObj = FfiConverterTypeCoreCryptoCallbacks.handleMap.get(uniffiHandle)
val makeCall = suspend { ->
uniffiObj.`userAuthorize`(
FfiConverterByteArray.lift(`conversationId`),
FfiConverterTypeClientId.lift(`externalClientId`),
FfiConverterSequenceTypeClientId.lift(`existingClients`),
)
}
val uniffiHandleSuccess = { returnValue: kotlin.Boolean ->
val uniffiResult = UniffiForeignFutureStructI8.UniffiByValue(
FfiConverterBoolean.lower(returnValue),
UniffiRustCallStatus.ByValue()
)
uniffiResult.write()
uniffiFutureCallback.callback(uniffiCallbackData, uniffiResult)
}
val uniffiHandleError = { callStatus: UniffiRustCallStatus.ByValue ->
uniffiFutureCallback.callback(
uniffiCallbackData,
UniffiForeignFutureStructI8.UniffiByValue(
0.toByte(),
callStatus,
),
)
}
uniffiOutReturn.uniffiSetValue(
uniffiTraitInterfaceCallAsync(
makeCall,
uniffiHandleSuccess,
uniffiHandleError
)
)
}
}
internal object `clientIsExistingGroupUser`: UniffiCallbackInterfaceCoreCryptoCallbacksMethod2 {
override fun callback(`uniffiHandle`: Long,`conversationId`: RustBuffer.ByValue,`clientId`: RustBuffer.ByValue,`existingClients`: RustBuffer.ByValue,`parentConversationClients`: RustBuffer.ByValue,`uniffiFutureCallback`: UniffiForeignFutureCompleteI8,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,) {
val uniffiObj = FfiConverterTypeCoreCryptoCallbacks.handleMap.get(uniffiHandle)
val makeCall = suspend { ->
uniffiObj.`clientIsExistingGroupUser`(
FfiConverterByteArray.lift(`conversationId`),
FfiConverterTypeClientId.lift(`clientId`),
FfiConverterSequenceTypeClientId.lift(`existingClients`),
FfiConverterOptionalSequenceTypeClientId.lift(`parentConversationClients`),
)
}
val uniffiHandleSuccess = { returnValue: kotlin.Boolean ->
val uniffiResult = UniffiForeignFutureStructI8.UniffiByValue(
FfiConverterBoolean.lower(returnValue),
UniffiRustCallStatus.ByValue()
)
uniffiResult.write()
uniffiFutureCallback.callback(uniffiCallbackData, uniffiResult)
}
val uniffiHandleError = { callStatus: UniffiRustCallStatus.ByValue ->
uniffiFutureCallback.callback(
uniffiCallbackData,
UniffiForeignFutureStructI8.UniffiByValue(
0.toByte(),
callStatus,
),
)
}
uniffiOutReturn.uniffiSetValue(
uniffiTraitInterfaceCallAsync(
makeCall,
uniffiHandleSuccess,
uniffiHandleError
)
)
}
}
internal object uniffiFree: UniffiCallbackInterfaceFree {
override fun callback(handle: Long) {
FfiConverterTypeCoreCryptoCallbacks.handleMap.remove(handle)
}
}
internal var vtable = UniffiVTableCallbackInterfaceCoreCryptoCallbacks.UniffiByValue(
`authorize`,
`userAuthorize`,
`clientIsExistingGroupUser`,
uniffiFree,
)
// Registers the foreign callback with the Rust side.
// This method is generated for each callback interface.
internal fun register(lib: UniffiLib) {
lib.uniffi_core_crypto_ffi_fn_init_callback_vtable_corecryptocallbacks(vtable)
}
}
public object FfiConverterTypeCoreCryptoCallbacks: FfiConverter {
internal val handleMap = UniffiHandleMap()
override fun lower(value: CoreCryptoCallbacks): Pointer {
return Pointer(handleMap.insert(value))
}
override fun lift(value: Pointer): CoreCryptoCallbacks {
return CoreCryptoCallbacksImpl(value)
}
override fun read(buf: ByteBuffer): CoreCryptoCallbacks {
// The Rust code always writes pointers as 8 bytes, and will
// fail to compile if they don't fit.
return lift(Pointer(buf.getLong()))
}
override fun allocationSize(value: CoreCryptoCallbacks) = 8UL
override fun write(value: CoreCryptoCallbacks, buf: ByteBuffer) {
// The Rust code always expects pointers written as 8 bytes,
// and will fail to compile if they don't fit.
buf.putLong(Pointer.nativeValue(lower(value)))
}
}
// This template implements a class for working with a Rust struct via a Pointer/Arc
// to the live Rust struct on the other side of the FFI.
//
// Each instance implements core operations for working with the Rust `Arc` and the
// Kotlin Pointer to work with the live Rust struct on the other side of the FFI.
//
// There's some subtlety here, because we have to be careful not to operate on a Rust
// struct after it has been dropped, and because we must expose a public API for freeing
// theq Kotlin wrapper object in lieu of reliable finalizers. The core requirements are:
//
// * Each instance holds an opaque pointer to the underlying Rust struct.
// Method calls need to read this pointer from the object's state and pass it in to
// the Rust FFI.
//
// * When an instance is no longer needed, its pointer should be passed to a
// special destructor function provided by the Rust FFI, which will drop the
// underlying Rust struct.
//
// * Given an instance, calling code is expected to call the special
// `destroy` method in order to free it after use, either by calling it explicitly
// or by using a higher-level helper like the `use` method. Failing to do so risks
// leaking the underlying Rust struct.
//
// * We can't assume that calling code will do the right thing, and must be prepared
// to handle Kotlin method calls executing concurrently with or even after a call to
// `destroy`, and to handle multiple (possibly concurrent!) calls to `destroy`.
//
// * We must never allow Rust code to operate on the underlying Rust struct after
// the destructor has been called, and must never call the destructor more than once.
// Doing so may trigger memory unsafety.
//
// * To mitigate many of the risks of leaking memory and use-after-free unsafety, a `Cleaner`
// is implemented to call the destructor when the Kotlin object becomes unreachable.
// This is done in a background thread. This is not a panacea, and client code should be aware that
// 1. the thread may starve if some there are objects that have poorly performing
// `drop` methods or do significant work in their `drop` methods.
// 2. the thread is shared across the whole library. This can be tuned by using `android_cleaner = true`,
// or `android = true` in the [`kotlin` section of the `uniffi.toml` file](https://mozilla.github.io/uniffi-rs/kotlin/configuration.html).
//
// If we try to implement this with mutual exclusion on access to the pointer, there is the
// possibility of a race between a method call and a concurrent call to `destroy`:
//
// * Thread A starts a method call, reads the value of the pointer, but is interrupted
// before it can pass the pointer over the FFI to Rust.
// * Thread B calls `destroy` and frees the underlying Rust struct.
// * Thread A resumes, passing the already-read pointer value to Rust and triggering
// a use-after-free.
//
// One possible solution would be to use a `ReadWriteLock`, with each method call taking
// a read lock (and thus allowed to run concurrently) and the special `destroy` method
// taking a write lock (and thus blocking on live method calls). However, we aim not to
// generate methods with any hidden blocking semantics, and a `destroy` method that might
// block if called incorrectly seems to meet that bar.
//
// So, we achieve our goals by giving each instance an associated `AtomicLong` counter to track
// the number of in-flight method calls, and an `AtomicBoolean` flag to indicate whether `destroy`
// has been called. These are updated according to the following rules:
//
// * The initial value of the counter is 1, indicating a live object with no in-flight calls.
// The initial value for the flag is false.
//
// * At the start of each method call, we atomically check the counter.
// If it is 0 then the underlying Rust struct has already been destroyed and the call is aborted.
// If it is nonzero them we atomically increment it by 1 and proceed with the method call.
//
// * At the end of each method call, we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// * When `destroy` is called, we atomically flip the flag from false to true.
// If the flag was already true we silently fail.
// Otherwise we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// Astute readers may observe that this all sounds very similar to the way that Rust's `Arc` works,
// and indeed it is, with the addition of a flag to guard against multiple calls to `destroy`.
//
// The overall effect is that the underlying Rust struct is destroyed only when `destroy` has been
// called *and* all in-flight method calls have completed, avoiding violating any of the expectations
// of the underlying Rust code.
//
// This makes a cleaner a better alternative to _not_ calling `destroy()` as
// and when the object is finished with, but the abstraction is not perfect: if the Rust object's `drop`
// method is slow, and/or there are many objects to cleanup, and it's on a low end Android device, then the cleaner
// thread may be starved, and the app will leak memory.
//
// In this case, `destroy`ing manually may be a better solution.
//
// The cleaner can live side by side with the manual calling of `destroy`. In the order of responsiveness, uniffi objects
// with Rust peers are reclaimed:
//
// 1. By calling the `destroy` method of the object, which calls `rustObject.free()`. If that doesn't happen:
// 2. When the object becomes unreachable, AND the Cleaner thread gets to call `rustObject.free()`. If the thread is starved then:
// 3. The memory is reclaimed when the process terminates.
//
// [1] https://stackoverflow.com/questions/24376768/can-java-finalize-an-object-when-it-is-still-in-scope/24380219
//
public interface CoreCryptoCommand {
/**
* Will be called inside a transaction in CoreCrypto
*/
suspend fun `execute`(`context`: CoreCryptoContext)
companion object
}
open class CoreCryptoCommandImpl: Disposable, AutoCloseable, CoreCryptoCommand {
constructor(pointer: Pointer) {
this.pointer = pointer
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
/**
* This constructor can be used to instantiate a fake object. Only used for tests. Any
* attempt to actually use an object constructed this way will fail as there is no
* connected Rust object.
*/
@Suppress("UNUSED_PARAMETER")
constructor(noPointer: NoPointer) {
this.pointer = null
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
protected val pointer: Pointer?
protected val cleanable: UniffiCleaner.Cleanable
private val wasDestroyed = AtomicBoolean(false)
private val callCounter = AtomicLong(1)
override fun destroy() {
// Only allow a single call to this method.
// TODO: maybe we should log a warning if called more than once?
if (this.wasDestroyed.compareAndSet(false, true)) {
// This decrement always matches the initial count of 1 given at creation time.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
@Synchronized
override fun close() {
this.destroy()
}
internal inline fun callWithPointer(block: (ptr: Pointer) -> R): R {
// Check and increment the call counter, to keep the object alive.
// This needs a compare-and-set retry loop in case of concurrent updates.
do {
val c = this.callCounter.get()
if (c == 0L) {
throw IllegalStateException("${this.javaClass.simpleName} object has already been destroyed")
}
if (c == Long.MAX_VALUE) {
throw IllegalStateException("${this.javaClass.simpleName} call counter would overflow")
}
} while (! this.callCounter.compareAndSet(c, c + 1L))
// Now we can safely do the method call without the pointer being freed concurrently.
try {
return block(this.uniffiClonePointer())
} finally {
// This decrement always matches the increment we performed above.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
// Use a static inner class instead of a closure so as not to accidentally
// capture `this` as part of the cleanable's action.
private class UniffiCleanAction(private val pointer: Pointer?) : Runnable {
override fun run() {
pointer?.let { ptr ->
uniffiRustCall { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_free_corecryptocommand(ptr, status)
}
}
}
}
fun uniffiClonePointer(): Pointer {
return uniffiRustCall() { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_clone_corecryptocommand(pointer!!, status)
}
}
/**
* Will be called inside a transaction in CoreCrypto
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `execute`(`context`: CoreCryptoContext) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocommand_execute(
thisPtr,
FfiConverterTypeCoreCryptoContext.lower(`context`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
companion object
}
// Put the implementation in an object so we don't pollute the top-level namespace
internal object uniffiCallbackInterfaceCoreCryptoCommand {
internal object `execute`: UniffiCallbackInterfaceCoreCryptoCommandMethod0 {
override fun callback(`uniffiHandle`: Long,`context`: Pointer,`uniffiFutureCallback`: UniffiForeignFutureCompleteVoid,`uniffiCallbackData`: Long,`uniffiOutReturn`: UniffiForeignFuture,) {
val uniffiObj = FfiConverterTypeCoreCryptoCommand.handleMap.get(uniffiHandle)
val makeCall = suspend { ->
uniffiObj.`execute`(
FfiConverterTypeCoreCryptoContext.lift(`context`),
)
}
val uniffiHandleSuccess = { _: Unit ->
val uniffiResult = UniffiForeignFutureStructVoid.UniffiByValue(
UniffiRustCallStatus.ByValue()
)
uniffiResult.write()
uniffiFutureCallback.callback(uniffiCallbackData, uniffiResult)
}
val uniffiHandleError = { callStatus: UniffiRustCallStatus.ByValue ->
uniffiFutureCallback.callback(
uniffiCallbackData,
UniffiForeignFutureStructVoid.UniffiByValue(
callStatus,
),
)
}
uniffiOutReturn.uniffiSetValue(
uniffiTraitInterfaceCallAsyncWithError(
makeCall,
uniffiHandleSuccess,
uniffiHandleError,
{ e: CoreCryptoException -> FfiConverterTypeCoreCryptoError.lower(e) }
)
)
}
}
internal object uniffiFree: UniffiCallbackInterfaceFree {
override fun callback(handle: Long) {
FfiConverterTypeCoreCryptoCommand.handleMap.remove(handle)
}
}
internal var vtable = UniffiVTableCallbackInterfaceCoreCryptoCommand.UniffiByValue(
`execute`,
uniffiFree,
)
// Registers the foreign callback with the Rust side.
// This method is generated for each callback interface.
internal fun register(lib: UniffiLib) {
lib.uniffi_core_crypto_ffi_fn_init_callback_vtable_corecryptocommand(vtable)
}
}
public object FfiConverterTypeCoreCryptoCommand: FfiConverter {
internal val handleMap = UniffiHandleMap()
override fun lower(value: CoreCryptoCommand): Pointer {
return Pointer(handleMap.insert(value))
}
override fun lift(value: Pointer): CoreCryptoCommand {
return CoreCryptoCommandImpl(value)
}
override fun read(buf: ByteBuffer): CoreCryptoCommand {
// The Rust code always writes pointers as 8 bytes, and will
// fail to compile if they don't fit.
return lift(Pointer(buf.getLong()))
}
override fun allocationSize(value: CoreCryptoCommand) = 8UL
override fun write(value: CoreCryptoCommand, buf: ByteBuffer) {
// The Rust code always expects pointers written as 8 bytes,
// and will fail to compile if they don't fit.
buf.putLong(Pointer.nativeValue(lower(value)))
}
}
// This template implements a class for working with a Rust struct via a Pointer/Arc
// to the live Rust struct on the other side of the FFI.
//
// Each instance implements core operations for working with the Rust `Arc` and the
// Kotlin Pointer to work with the live Rust struct on the other side of the FFI.
//
// There's some subtlety here, because we have to be careful not to operate on a Rust
// struct after it has been dropped, and because we must expose a public API for freeing
// theq Kotlin wrapper object in lieu of reliable finalizers. The core requirements are:
//
// * Each instance holds an opaque pointer to the underlying Rust struct.
// Method calls need to read this pointer from the object's state and pass it in to
// the Rust FFI.
//
// * When an instance is no longer needed, its pointer should be passed to a
// special destructor function provided by the Rust FFI, which will drop the
// underlying Rust struct.
//
// * Given an instance, calling code is expected to call the special
// `destroy` method in order to free it after use, either by calling it explicitly
// or by using a higher-level helper like the `use` method. Failing to do so risks
// leaking the underlying Rust struct.
//
// * We can't assume that calling code will do the right thing, and must be prepared
// to handle Kotlin method calls executing concurrently with or even after a call to
// `destroy`, and to handle multiple (possibly concurrent!) calls to `destroy`.
//
// * We must never allow Rust code to operate on the underlying Rust struct after
// the destructor has been called, and must never call the destructor more than once.
// Doing so may trigger memory unsafety.
//
// * To mitigate many of the risks of leaking memory and use-after-free unsafety, a `Cleaner`
// is implemented to call the destructor when the Kotlin object becomes unreachable.
// This is done in a background thread. This is not a panacea, and client code should be aware that
// 1. the thread may starve if some there are objects that have poorly performing
// `drop` methods or do significant work in their `drop` methods.
// 2. the thread is shared across the whole library. This can be tuned by using `android_cleaner = true`,
// or `android = true` in the [`kotlin` section of the `uniffi.toml` file](https://mozilla.github.io/uniffi-rs/kotlin/configuration.html).
//
// If we try to implement this with mutual exclusion on access to the pointer, there is the
// possibility of a race between a method call and a concurrent call to `destroy`:
//
// * Thread A starts a method call, reads the value of the pointer, but is interrupted
// before it can pass the pointer over the FFI to Rust.
// * Thread B calls `destroy` and frees the underlying Rust struct.
// * Thread A resumes, passing the already-read pointer value to Rust and triggering
// a use-after-free.
//
// One possible solution would be to use a `ReadWriteLock`, with each method call taking
// a read lock (and thus allowed to run concurrently) and the special `destroy` method
// taking a write lock (and thus blocking on live method calls). However, we aim not to
// generate methods with any hidden blocking semantics, and a `destroy` method that might
// block if called incorrectly seems to meet that bar.
//
// So, we achieve our goals by giving each instance an associated `AtomicLong` counter to track
// the number of in-flight method calls, and an `AtomicBoolean` flag to indicate whether `destroy`
// has been called. These are updated according to the following rules:
//
// * The initial value of the counter is 1, indicating a live object with no in-flight calls.
// The initial value for the flag is false.
//
// * At the start of each method call, we atomically check the counter.
// If it is 0 then the underlying Rust struct has already been destroyed and the call is aborted.
// If it is nonzero them we atomically increment it by 1 and proceed with the method call.
//
// * At the end of each method call, we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// * When `destroy` is called, we atomically flip the flag from false to true.
// If the flag was already true we silently fail.
// Otherwise we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// Astute readers may observe that this all sounds very similar to the way that Rust's `Arc` works,
// and indeed it is, with the addition of a flag to guard against multiple calls to `destroy`.
//
// The overall effect is that the underlying Rust struct is destroyed only when `destroy` has been
// called *and* all in-flight method calls have completed, avoiding violating any of the expectations
// of the underlying Rust code.
//
// This makes a cleaner a better alternative to _not_ calling `destroy()` as
// and when the object is finished with, but the abstraction is not perfect: if the Rust object's `drop`
// method is slow, and/or there are many objects to cleanup, and it's on a low end Android device, then the cleaner
// thread may be starved, and the app will leak memory.
//
// In this case, `destroy`ing manually may be a better solution.
//
// The cleaner can live side by side with the manual calling of `destroy`. In the order of responsiveness, uniffi objects
// with Rust peers are reclaimed:
//
// 1. By calling the `destroy` method of the object, which calls `rustObject.free()`. If that doesn't happen:
// 2. When the object becomes unreachable, AND the Cleaner thread gets to call `rustObject.free()`. If the thread is starved then:
// 3. The memory is reclaimed when the process terminates.
//
// [1] https://stackoverflow.com/questions/24376768/can-java-finalize-an-object-when-it-is-still-in-scope/24380219
//
public interface CoreCryptoContextInterface {
/**
* See [core_crypto::context::CentralContext::add_members_to_conversation]
*/
suspend fun `addClientsToConversation`(`conversationId`: kotlin.ByteArray, `keyPackages`: List): MemberAddedMessages
/**
* See [core_crypto::context::CentralContext::clear_pending_commit]
*/
suspend fun `clearPendingCommit`(`conversationId`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::clear_pending_group_from_external_commit]
*/
suspend fun `clearPendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::clear_pending_proposal]
*/
suspend fun `clearPendingProposal`(`conversationId`: kotlin.ByteArray, `proposalRef`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::get_or_create_client_keypackages]
*/
suspend fun `clientKeypackages`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType, `amountRequested`: kotlin.UInt): List
/**
* See [core_crypto::mls::MlsCentral::client_public_key]
*/
suspend fun `clientPublicKey`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::client_valid_key_packages_count]
*/
suspend fun `clientValidKeypackagesCount`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType): kotlin.ULong
/**
* See [core_crypto::context::CentralContext::commit_accepted]
*/
suspend fun `commitAccepted`(`conversationId`: kotlin.ByteArray): List?
/**
* See [core_crypto::context::CentralContext::commit_pending_proposals]
*/
suspend fun `commitPendingProposals`(`conversationId`: kotlin.ByteArray): CommitBundle?
/**
* See [core_crypto::mls::MlsCentral::conversation_ciphersuite]
*/
suspend fun `conversationCiphersuite`(`conversationId`: kotlin.ByteArray): Ciphersuite
/**
* See [core_crypto::mls::MlsCentral::conversation_epoch]
*/
suspend fun `conversationEpoch`(`conversationId`: kotlin.ByteArray): kotlin.ULong
/**
* See [core_crypto::mls::MlsCentral::conversation_exists]
*/
suspend fun `conversationExists`(`conversationId`: kotlin.ByteArray): kotlin.Boolean
/**
* See [core_crypto::context::CentralContext::new_conversation]
*/
suspend fun `createConversation`(`conversationId`: kotlin.ByteArray, `creatorCredentialType`: MlsCredentialType, `config`: ConversationConfiguration)
/**
* See [core_crypto::context::CentralContext::decrypt_message]
*/
suspend fun `decryptMessage`(`conversationId`: kotlin.ByteArray, `payload`: kotlin.ByteArray): DecryptedMessage
/**
* See [core_crypto::context::CentralContext::delete_keypackages]
*/
suspend fun `deleteKeypackages`(`refs`: List)
/**
* See [core_crypto::context::CentralContext::e2ei_conversation_state]
*/
suspend fun `e2eiConversationState`(`conversationId`: kotlin.ByteArray): E2eiConversationState
suspend fun `e2eiDumpPkiEnv`(): E2eiDumpedPkiEnv?
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash]
*/
suspend fun `e2eiEnrollmentStash`(`enrollment`: E2eiEnrollment): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash_pop]
*/
suspend fun `e2eiEnrollmentStashPop`(`handle`: kotlin.ByteArray): E2eiEnrollment
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_enabled]
*/
suspend fun `e2eiIsEnabled`(`ciphersuite`: Ciphersuite): kotlin.Boolean
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_pki_env_setup]
*/
suspend fun `e2eiIsPkiEnvSetup`(): kotlin.Boolean
/**
* See [core_crypto::context::CentralContext::e2ei_mls_init_only]
*/
suspend fun `e2eiMlsInitOnly`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `nbKeyPackage`: kotlin.UInt?): List?
/**
* See [core_crypto::context::CentralContext::e2ei_new_activation_enrollment]
*/
suspend fun `e2eiNewActivationEnrollment`(`displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite): E2eiEnrollment
/**
* See [core_crypto::context::CentralContext::e2ei_new_enrollment]
*/
suspend fun `e2eiNewEnrollment`(`clientId`: kotlin.String, `displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite): E2eiEnrollment
/**
* See [core_crypto::context::CentralContext::e2ei_new_rotate_enrollment]
*/
suspend fun `e2eiNewRotateEnrollment`(`displayName`: kotlin.String?, `handle`: kotlin.String?, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite): E2eiEnrollment
/**
* See [core_crypto::context::CentralContext::e2ei_register_acme_ca]
*/
suspend fun `e2eiRegisterAcmeCa`(`trustAnchorPem`: kotlin.String)
/**
* See [core_crypto::context::CentralContext::e2ei_register_crl]
*/
suspend fun `e2eiRegisterCrl`(`crlDp`: kotlin.String, `crlDer`: kotlin.ByteArray): CrlRegistration
/**
* See [core_crypto::context::CentralContext::e2ei_register_intermediate_ca_pem]
*/
suspend fun `e2eiRegisterIntermediateCa`(`certPem`: kotlin.String): List?
/**
* See [core_crypto::context::CentralContext::e2ei_rotate]
*/
suspend fun `e2eiRotate`(`conversationId`: kotlin.ByteArray): CommitBundle
/**
* See [core_crypto::context::CentralContext::e2ei_rotate_all]
*/
suspend fun `e2eiRotateAll`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `newKeyPackagesCount`: kotlin.UInt): RotateBundle
/**
* See [core_crypto::context::CentralContext::encrypt_message]
*/
suspend fun `encryptMessage`(`conversationId`: kotlin.ByteArray, `message`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::mls::MlsCentral::export_secret_key]
*/
suspend fun `exportSecretKey`(`conversationId`: kotlin.ByteArray, `keyLength`: kotlin.UInt): kotlin.ByteArray
/**
* See [core_crypto::mls::MlsCentral::get_client_ids]
*/
suspend fun `getClientIds`(`conversationId`: kotlin.ByteArray): List
/**
* See [core_crypto::mls::MlsCentral::get_credential_in_use]
*/
suspend fun `getCredentialInUse`(`groupInfo`: kotlin.ByteArray, `credentialType`: MlsCredentialType): E2eiConversationState
/**
* See [core_crypto::context::CentralContext::get_data].
*/
suspend fun `getData`(): kotlin.ByteArray?
/**
* See [core_crypto::mls::MlsCentral::get_device_identities]
*/
suspend fun `getDeviceIdentities`(`conversationId`: kotlin.ByteArray, `deviceIds`: List): List
/**
* See [core_crypto::mls::MlsCentral::get_external_sender]
*/
suspend fun `getExternalSender`(`conversationId`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::mls::MlsCentral::get_user_identities]
*/
suspend fun `getUserIdentities`(`conversationId`: kotlin.ByteArray, `userIds`: List): Map>
/**
* See [core_crypto::context::CentralContext::join_by_external_commit]
*/
suspend fun `joinByExternalCommit`(`groupInfo`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration, `credentialType`: MlsCredentialType): ConversationInitBundle
/**
* See [core_crypto::context::CentralContext::mark_conversation_as_child_of]
*/
suspend fun `markConversationAsChildOf`(`childId`: kotlin.ByteArray, `parentId`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::merge_pending_group_from_external_commit]
*/
suspend fun `mergePendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray): List?
/**
* See [core_crypto::context::CentralContext::mls_generate_keypairs]
*/
suspend fun `mlsGenerateKeypairs`(`ciphersuites`: Ciphersuites): List
/**
* See [core_crypto::context::CentralContext::mls_init]
*/
suspend fun `mlsInit`(`clientId`: ClientId, `ciphersuites`: Ciphersuites, `nbKeyPackage`: kotlin.UInt?)
/**
* See [core_crypto::context::CentralContext::mls_init_with_client_id]
*/
suspend fun `mlsInitWithClientId`(`clientId`: ClientId, `tmpClientIds`: List, `ciphersuites`: Ciphersuites)
/**
* See [core_crypto::context::CentralContext::new_add_proposal]
*/
suspend fun `newAddProposal`(`conversationId`: kotlin.ByteArray, `keypackage`: kotlin.ByteArray): ProposalBundle
/**
* See [core_crypto::context::CentralContext::new_external_add_proposal]
*/
suspend fun `newExternalAddProposal`(`conversationId`: kotlin.ByteArray, `epoch`: kotlin.ULong, `ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::new_remove_proposal]
*/
suspend fun `newRemoveProposal`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId): ProposalBundle
/**
* See [core_crypto::context::CentralContext::new_update_proposal]
*/
suspend fun `newUpdateProposal`(`conversationId`: kotlin.ByteArray): ProposalBundle
/**
* See [core_crypto::context::CentralContext::process_raw_welcome_message]
*/
suspend fun `processWelcomeMessage`(`welcomeMessage`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration): WelcomeBundle
/**
* See [core_crypto::context::CentralContext::proteus_cryptobox_migrate]
*/
suspend fun `proteusCryptoboxMigrate`(`path`: kotlin.String)
/**
* See [core_crypto::context::CentralContext::proteus_decrypt]
*/
suspend fun `proteusDecrypt`(`sessionId`: kotlin.String, `ciphertext`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::proteus_encrypt]
*/
suspend fun `proteusEncrypt`(`sessionId`: kotlin.String, `plaintext`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::proteus_encrypt_batched]
*/
suspend fun `proteusEncryptBatched`(`sessions`: List, `plaintext`: kotlin.ByteArray): Map
/**
* See [core_crypto::context::CentralContext::proteus_fingerprint]
*/
suspend fun `proteusFingerprint`(): kotlin.String
/**
* See [core_crypto::context::CentralContext::proteus_fingerprint_local]
*/
suspend fun `proteusFingerprintLocal`(`sessionId`: kotlin.String): kotlin.String
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_prekeybundle]
* NOTE: uniffi doesn't support associated functions, so we have to have the self here
*/
fun `proteusFingerprintPrekeybundle`(`prekey`: kotlin.ByteArray): kotlin.String
/**
* See [core_crypto::context::CentralContext::proteus_fingerprint_remote]
*/
suspend fun `proteusFingerprintRemote`(`sessionId`: kotlin.String): kotlin.String
/**
* Returns the latest proteus error code. If 0, no error has occured
*
* NOTE: This will clear the last error code.
*/
fun `proteusLastErrorCode`(): kotlin.UInt
/**
* See [core_crypto::context::CentralContext::proteus_last_resort_prekey]
*/
suspend fun `proteusLastResortPrekey`(): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::proteus_last_resort_prekey_id]
*/
fun `proteusLastResortPrekeyId`(): kotlin.UShort
/**
* See [core_crypto::context::CentralContext::proteus_new_prekey]
*/
suspend fun `proteusNewPrekey`(`prekeyId`: kotlin.UShort): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::proteus_new_prekey_auto]
*/
suspend fun `proteusNewPrekeyAuto`(): ProteusAutoPrekeyBundle
/**
* See [core_crypto::context::CentralContext::proteus_session_delete]
*/
suspend fun `proteusSessionDelete`(`sessionId`: kotlin.String)
/**
* See [core_crypto::context::CentralContext::proteus_session_exists]
*/
suspend fun `proteusSessionExists`(`sessionId`: kotlin.String): kotlin.Boolean
/**
* See [core_crypto::context::CentralContext::proteus_session_from_message]
*/
suspend fun `proteusSessionFromMessage`(`sessionId`: kotlin.String, `envelope`: kotlin.ByteArray): kotlin.ByteArray
/**
* See [core_crypto::context::CentralContext::proteus_session_from_prekey]
*/
suspend fun `proteusSessionFromPrekey`(`sessionId`: kotlin.String, `prekey`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::proteus_session_save]
* **Note**: This isn't usually needed as persisting sessions happens automatically when decrypting/encrypting messages and initializing Sessions
*/
suspend fun `proteusSessionSave`(`sessionId`: kotlin.String)
/**
* See [core_crypto::context::CentralContext::remove_members_from_conversation]
*/
suspend fun `removeClientsFromConversation`(`conversationId`: kotlin.ByteArray, `clients`: List): CommitBundle
/**
* See [core_crypto::context::CentralContext::set_data].
*/
suspend fun `setData`(`data`: kotlin.ByteArray)
/**
* See [core_crypto::context::CentralContext::update_keying_material]
*/
suspend fun `updateKeyingMaterial`(`conversationId`: kotlin.ByteArray): CommitBundle
/**
* see [core_crypto::context::CentralContext::wipe_conversation]
*/
suspend fun `wipeConversation`(`conversationId`: kotlin.ByteArray)
companion object
}
open class CoreCryptoContext: Disposable, AutoCloseable, CoreCryptoContextInterface {
constructor(pointer: Pointer) {
this.pointer = pointer
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
/**
* This constructor can be used to instantiate a fake object. Only used for tests. Any
* attempt to actually use an object constructed this way will fail as there is no
* connected Rust object.
*/
@Suppress("UNUSED_PARAMETER")
constructor(noPointer: NoPointer) {
this.pointer = null
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
protected val pointer: Pointer?
protected val cleanable: UniffiCleaner.Cleanable
private val wasDestroyed = AtomicBoolean(false)
private val callCounter = AtomicLong(1)
override fun destroy() {
// Only allow a single call to this method.
// TODO: maybe we should log a warning if called more than once?
if (this.wasDestroyed.compareAndSet(false, true)) {
// This decrement always matches the initial count of 1 given at creation time.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
@Synchronized
override fun close() {
this.destroy()
}
internal inline fun callWithPointer(block: (ptr: Pointer) -> R): R {
// Check and increment the call counter, to keep the object alive.
// This needs a compare-and-set retry loop in case of concurrent updates.
do {
val c = this.callCounter.get()
if (c == 0L) {
throw IllegalStateException("${this.javaClass.simpleName} object has already been destroyed")
}
if (c == Long.MAX_VALUE) {
throw IllegalStateException("${this.javaClass.simpleName} call counter would overflow")
}
} while (! this.callCounter.compareAndSet(c, c + 1L))
// Now we can safely do the method call without the pointer being freed concurrently.
try {
return block(this.uniffiClonePointer())
} finally {
// This decrement always matches the increment we performed above.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
// Use a static inner class instead of a closure so as not to accidentally
// capture `this` as part of the cleanable's action.
private class UniffiCleanAction(private val pointer: Pointer?) : Runnable {
override fun run() {
pointer?.let { ptr ->
uniffiRustCall { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_free_corecryptocontext(ptr, status)
}
}
}
}
fun uniffiClonePointer(): Pointer {
return uniffiRustCall() { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_clone_corecryptocontext(pointer!!, status)
}
}
/**
* See [core_crypto::context::CentralContext::add_members_to_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `addClientsToConversation`(`conversationId`: kotlin.ByteArray, `keyPackages`: List) : MemberAddedMessages {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_add_clients_to_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceByteArray.lower(`keyPackages`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeMemberAddedMessages.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::clear_pending_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clearPendingCommit`(`conversationId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_clear_pending_commit(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::clear_pending_group_from_external_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clearPendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_clear_pending_group_from_external_commit(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::clear_pending_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clearPendingProposal`(`conversationId`: kotlin.ByteArray, `proposalRef`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_clear_pending_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`proposalRef`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::get_or_create_client_keypackages]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clientKeypackages`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType, `amountRequested`: kotlin.UInt) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_client_keypackages(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),FfiConverterUInt.lower(`amountRequested`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::client_public_key]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clientPublicKey`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_client_public_key(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::client_valid_key_packages_count]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `clientValidKeypackagesCount`(`ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType) : kotlin.ULong {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_client_valid_keypackages_count(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_u64(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_u64(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_u64(future) },
// lift function
{ FfiConverterULong.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::commit_accepted]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `commitAccepted`(`conversationId`: kotlin.ByteArray) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_commit_accepted(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceTypeBufferedDecryptedMessage.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::commit_pending_proposals]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `commitPendingProposals`(`conversationId`: kotlin.ByteArray) : CommitBundle? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_commit_pending_proposals(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::conversation_ciphersuite]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `conversationCiphersuite`(`conversationId`: kotlin.ByteArray) : Ciphersuite {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_conversation_ciphersuite(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_u16(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_u16(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_u16(future) },
// lift function
{ FfiConverterTypeCiphersuite.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::conversation_epoch]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `conversationEpoch`(`conversationId`: kotlin.ByteArray) : kotlin.ULong {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_conversation_epoch(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_u64(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_u64(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_u64(future) },
// lift function
{ FfiConverterULong.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::conversation_exists]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `conversationExists`(`conversationId`: kotlin.ByteArray) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_conversation_exists(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `createConversation`(`conversationId`: kotlin.ByteArray, `creatorCredentialType`: MlsCredentialType, `config`: ConversationConfiguration) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_create_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterTypeMlsCredentialType.lower(`creatorCredentialType`),FfiConverterTypeConversationConfiguration.lower(`config`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::decrypt_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `decryptMessage`(`conversationId`: kotlin.ByteArray, `payload`: kotlin.ByteArray) : DecryptedMessage {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_decrypt_message(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`payload`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeDecryptedMessage.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::delete_keypackages]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `deleteKeypackages`(`refs`: List) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_delete_keypackages(
thisPtr,
FfiConverterSequenceByteArray.lower(`refs`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_conversation_state]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiConversationState`(`conversationId`: kotlin.ByteArray) : E2eiConversationState {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_conversation_state(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeE2eiConversationState.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiDumpPkiEnv`() : E2eiDumpedPkiEnv? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_dump_pki_env(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalTypeE2eiDumpedPkiEnv.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiEnrollmentStash`(`enrollment`: E2eiEnrollment) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_enrollment_stash(
thisPtr,
FfiConverterTypeE2eiEnrollment.lower(`enrollment`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_enrollment_stash_pop]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiEnrollmentStashPop`(`handle`: kotlin.ByteArray) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_enrollment_stash_pop(
thisPtr,
FfiConverterByteArray.lower(`handle`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_enabled]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiIsEnabled`(`ciphersuite`: Ciphersuite) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_is_enabled(
thisPtr,
FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::e2ei_is_pki_env_setup]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiIsPkiEnvSetup`() : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_is_pki_env_setup(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_mls_init_only]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiMlsInitOnly`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `nbKeyPackage`: kotlin.UInt?) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_mls_init_only(
thisPtr,
FfiConverterTypeE2eiEnrollment.lower(`enrollment`),FfiConverterString.lower(`certificateChain`),FfiConverterOptionalUInt.lower(`nbKeyPackage`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_new_activation_enrollment]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiNewActivationEnrollment`(`displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_new_activation_enrollment(
thisPtr,
FfiConverterString.lower(`displayName`),FfiConverterString.lower(`handle`),FfiConverterOptionalString.lower(`team`),FfiConverterUInt.lower(`expirySec`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_new_enrollment]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiNewEnrollment`(`clientId`: kotlin.String, `displayName`: kotlin.String, `handle`: kotlin.String, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_new_enrollment(
thisPtr,
FfiConverterString.lower(`clientId`),FfiConverterString.lower(`displayName`),FfiConverterString.lower(`handle`),FfiConverterOptionalString.lower(`team`),FfiConverterUInt.lower(`expirySec`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_new_rotate_enrollment]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiNewRotateEnrollment`(`displayName`: kotlin.String?, `handle`: kotlin.String?, `team`: kotlin.String?, `expirySec`: kotlin.UInt, `ciphersuite`: Ciphersuite) : E2eiEnrollment {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_new_rotate_enrollment(
thisPtr,
FfiConverterOptionalString.lower(`displayName`),FfiConverterOptionalString.lower(`handle`),FfiConverterOptionalString.lower(`team`),FfiConverterUInt.lower(`expirySec`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_pointer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_pointer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_pointer(future) },
// lift function
{ FfiConverterTypeE2eiEnrollment.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_register_acme_ca]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRegisterAcmeCa`(`trustAnchorPem`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_register_acme_ca(
thisPtr,
FfiConverterString.lower(`trustAnchorPem`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_register_crl]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRegisterCrl`(`crlDp`: kotlin.String, `crlDer`: kotlin.ByteArray) : CrlRegistration {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_register_crl(
thisPtr,
FfiConverterString.lower(`crlDp`),FfiConverterByteArray.lower(`crlDer`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCrlRegistration.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_register_intermediate_ca_pem]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRegisterIntermediateCa`(`certPem`: kotlin.String) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_register_intermediate_ca(
thisPtr,
FfiConverterString.lower(`certPem`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_rotate]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRotate`(`conversationId`: kotlin.ByteArray) : CommitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_rotate(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::e2ei_rotate_all]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `e2eiRotateAll`(`enrollment`: E2eiEnrollment, `certificateChain`: kotlin.String, `newKeyPackagesCount`: kotlin.UInt) : RotateBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_e2ei_rotate_all(
thisPtr,
FfiConverterTypeE2eiEnrollment.lower(`enrollment`),FfiConverterString.lower(`certificateChain`),FfiConverterUInt.lower(`newKeyPackagesCount`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeRotateBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::encrypt_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `encryptMessage`(`conversationId`: kotlin.ByteArray, `message`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_encrypt_message(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`message`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::export_secret_key]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `exportSecretKey`(`conversationId`: kotlin.ByteArray, `keyLength`: kotlin.UInt) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_export_secret_key(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterUInt.lower(`keyLength`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_client_ids]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getClientIds`(`conversationId`: kotlin.ByteArray) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_client_ids(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceTypeClientId.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_credential_in_use]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getCredentialInUse`(`groupInfo`: kotlin.ByteArray, `credentialType`: MlsCredentialType) : E2eiConversationState {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_credential_in_use(
thisPtr,
FfiConverterByteArray.lower(`groupInfo`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeE2eiConversationState.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::get_data].
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getData`() : kotlin.ByteArray? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_data(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_device_identities]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getDeviceIdentities`(`conversationId`: kotlin.ByteArray, `deviceIds`: List) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_device_identities(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceTypeClientId.lower(`deviceIds`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceTypeWireIdentity.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_external_sender]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getExternalSender`(`conversationId`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_external_sender(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::mls::MlsCentral::get_user_identities]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getUserIdentities`(`conversationId`: kotlin.ByteArray, `userIds`: List) : Map> {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_get_user_identities(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceString.lower(`userIds`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterMapStringSequenceTypeWireIdentity.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::join_by_external_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `joinByExternalCommit`(`groupInfo`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration, `credentialType`: MlsCredentialType) : ConversationInitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_join_by_external_commit(
thisPtr,
FfiConverterByteArray.lower(`groupInfo`),FfiConverterTypeCustomConfiguration.lower(`customConfiguration`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeConversationInitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mark_conversation_as_child_of]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `markConversationAsChildOf`(`childId`: kotlin.ByteArray, `parentId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_mark_conversation_as_child_of(
thisPtr,
FfiConverterByteArray.lower(`childId`),FfiConverterByteArray.lower(`parentId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::merge_pending_group_from_external_commit]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mergePendingGroupFromExternalCommit`(`conversationId`: kotlin.ByteArray) : List? {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_merge_pending_group_from_external_commit(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterOptionalSequenceTypeBufferedDecryptedMessage.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mls_generate_keypairs]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mlsGenerateKeypairs`(`ciphersuites`: Ciphersuites) : List {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_mls_generate_keypairs(
thisPtr,
FfiConverterTypeCiphersuites.lower(`ciphersuites`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterSequenceTypeClientId.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mls_init]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mlsInit`(`clientId`: ClientId, `ciphersuites`: Ciphersuites, `nbKeyPackage`: kotlin.UInt?) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_mls_init(
thisPtr,
FfiConverterTypeClientId.lower(`clientId`),FfiConverterTypeCiphersuites.lower(`ciphersuites`),FfiConverterOptionalUInt.lower(`nbKeyPackage`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::mls_init_with_client_id]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `mlsInitWithClientId`(`clientId`: ClientId, `tmpClientIds`: List, `ciphersuites`: Ciphersuites) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_mls_init_with_client_id(
thisPtr,
FfiConverterTypeClientId.lower(`clientId`),FfiConverterSequenceTypeClientId.lower(`tmpClientIds`),FfiConverterTypeCiphersuites.lower(`ciphersuites`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_add_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newAddProposal`(`conversationId`: kotlin.ByteArray, `keypackage`: kotlin.ByteArray) : ProposalBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_add_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterByteArray.lower(`keypackage`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProposalBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_external_add_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newExternalAddProposal`(`conversationId`: kotlin.ByteArray, `epoch`: kotlin.ULong, `ciphersuite`: Ciphersuite, `credentialType`: MlsCredentialType) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_external_add_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterULong.lower(`epoch`),FfiConverterTypeCiphersuite.lower(`ciphersuite`),FfiConverterTypeMlsCredentialType.lower(`credentialType`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_remove_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newRemoveProposal`(`conversationId`: kotlin.ByteArray, `clientId`: ClientId) : ProposalBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_remove_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterTypeClientId.lower(`clientId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProposalBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::new_update_proposal]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newUpdateProposal`(`conversationId`: kotlin.ByteArray) : ProposalBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_new_update_proposal(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProposalBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::process_raw_welcome_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `processWelcomeMessage`(`welcomeMessage`: kotlin.ByteArray, `customConfiguration`: CustomConfiguration) : WelcomeBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_process_welcome_message(
thisPtr,
FfiConverterByteArray.lower(`welcomeMessage`),FfiConverterTypeCustomConfiguration.lower(`customConfiguration`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeWelcomeBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_cryptobox_migrate]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusCryptoboxMigrate`(`path`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_cryptobox_migrate(
thisPtr,
FfiConverterString.lower(`path`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_decrypt]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusDecrypt`(`sessionId`: kotlin.String, `ciphertext`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_decrypt(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`ciphertext`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_encrypt]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusEncrypt`(`sessionId`: kotlin.String, `plaintext`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_encrypt(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`plaintext`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_encrypt_batched]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusEncryptBatched`(`sessions`: List, `plaintext`: kotlin.ByteArray) : Map {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_encrypt_batched(
thisPtr,
FfiConverterSequenceString.lower(`sessions`),FfiConverterByteArray.lower(`plaintext`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterMapStringByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_fingerprint]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusFingerprint`() : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_fingerprint_local]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusFingerprintLocal`(`sessionId`: kotlin.String) : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint_local(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::proteus::ProteusCentral::fingerprint_prekeybundle]
* NOTE: uniffi doesn't support associated functions, so we have to have the self here
*/
@Throws(CoreCryptoException::class)override fun `proteusFingerprintPrekeybundle`(`prekey`: kotlin.ByteArray): kotlin.String {
return FfiConverterString.lift(
callWithPointer {
uniffiRustCallWithError(CoreCryptoException) { _status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint_prekeybundle(
it, FfiConverterByteArray.lower(`prekey`),_status)
}
}
)
}
/**
* See [core_crypto::context::CentralContext::proteus_fingerprint_remote]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusFingerprintRemote`(`sessionId`: kotlin.String) : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_fingerprint_remote(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* Returns the latest proteus error code. If 0, no error has occured
*
* NOTE: This will clear the last error code.
*/override fun `proteusLastErrorCode`(): kotlin.UInt {
return FfiConverterUInt.lift(
callWithPointer {
uniffiRustCall() { _status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_last_error_code(
it, _status)
}
}
)
}
/**
* See [core_crypto::context::CentralContext::proteus_last_resort_prekey]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusLastResortPrekey`() : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_last_resort_prekey(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_last_resort_prekey_id]
*/
@Throws(CoreCryptoException::class)override fun `proteusLastResortPrekeyId`(): kotlin.UShort {
return FfiConverterUShort.lift(
callWithPointer {
uniffiRustCallWithError(CoreCryptoException) { _status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_last_resort_prekey_id(
it, _status)
}
}
)
}
/**
* See [core_crypto::context::CentralContext::proteus_new_prekey]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusNewPrekey`(`prekeyId`: kotlin.UShort) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_new_prekey(
thisPtr,
FfiConverterUShort.lower(`prekeyId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_new_prekey_auto]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusNewPrekeyAuto`() : ProteusAutoPrekeyBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_new_prekey_auto(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeProteusAutoPrekeyBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_session_delete]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionDelete`(`sessionId`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_delete(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_session_exists]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionExists`(`sessionId`: kotlin.String) : kotlin.Boolean {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_exists(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_i8(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_i8(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_i8(future) },
// lift function
{ FfiConverterBoolean.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_session_from_message]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionFromMessage`(`sessionId`: kotlin.String, `envelope`: kotlin.ByteArray) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_from_message(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`envelope`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_session_from_prekey]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionFromPrekey`(`sessionId`: kotlin.String, `prekey`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_from_prekey(
thisPtr,
FfiConverterString.lower(`sessionId`),FfiConverterByteArray.lower(`prekey`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::proteus_session_save]
* **Note**: This isn't usually needed as persisting sessions happens automatically when decrypting/encrypting messages and initializing Sessions
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `proteusSessionSave`(`sessionId`: kotlin.String) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_proteus_session_save(
thisPtr,
FfiConverterString.lower(`sessionId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::remove_members_from_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `removeClientsFromConversation`(`conversationId`: kotlin.ByteArray, `clients`: List) : CommitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_remove_clients_from_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),FfiConverterSequenceTypeClientId.lower(`clients`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::set_data].
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `setData`(`data`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_set_data(
thisPtr,
FfiConverterByteArray.lower(`data`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::context::CentralContext::update_keying_material]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `updateKeyingMaterial`(`conversationId`: kotlin.ByteArray) : CommitBundle {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_update_keying_material(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeCommitBundle.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* see [core_crypto::context::CentralContext::wipe_conversation]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `wipeConversation`(`conversationId`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptocontext_wipe_conversation(
thisPtr,
FfiConverterByteArray.lower(`conversationId`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
companion object
}
public object FfiConverterTypeCoreCryptoContext: FfiConverter {
override fun lower(value: CoreCryptoContext): Pointer {
return value.uniffiClonePointer()
}
override fun lift(value: Pointer): CoreCryptoContext {
return CoreCryptoContext(value)
}
override fun read(buf: ByteBuffer): CoreCryptoContext {
// The Rust code always writes pointers as 8 bytes, and will
// fail to compile if they don't fit.
return lift(Pointer(buf.getLong()))
}
override fun allocationSize(value: CoreCryptoContext) = 8UL
override fun write(value: CoreCryptoContext, buf: ByteBuffer) {
// The Rust code always expects pointers written as 8 bytes,
// and will fail to compile if they don't fit.
buf.putLong(Pointer.nativeValue(lower(value)))
}
}
// This template implements a class for working with a Rust struct via a Pointer/Arc
// to the live Rust struct on the other side of the FFI.
//
// Each instance implements core operations for working with the Rust `Arc` and the
// Kotlin Pointer to work with the live Rust struct on the other side of the FFI.
//
// There's some subtlety here, because we have to be careful not to operate on a Rust
// struct after it has been dropped, and because we must expose a public API for freeing
// theq Kotlin wrapper object in lieu of reliable finalizers. The core requirements are:
//
// * Each instance holds an opaque pointer to the underlying Rust struct.
// Method calls need to read this pointer from the object's state and pass it in to
// the Rust FFI.
//
// * When an instance is no longer needed, its pointer should be passed to a
// special destructor function provided by the Rust FFI, which will drop the
// underlying Rust struct.
//
// * Given an instance, calling code is expected to call the special
// `destroy` method in order to free it after use, either by calling it explicitly
// or by using a higher-level helper like the `use` method. Failing to do so risks
// leaking the underlying Rust struct.
//
// * We can't assume that calling code will do the right thing, and must be prepared
// to handle Kotlin method calls executing concurrently with or even after a call to
// `destroy`, and to handle multiple (possibly concurrent!) calls to `destroy`.
//
// * We must never allow Rust code to operate on the underlying Rust struct after
// the destructor has been called, and must never call the destructor more than once.
// Doing so may trigger memory unsafety.
//
// * To mitigate many of the risks of leaking memory and use-after-free unsafety, a `Cleaner`
// is implemented to call the destructor when the Kotlin object becomes unreachable.
// This is done in a background thread. This is not a panacea, and client code should be aware that
// 1. the thread may starve if some there are objects that have poorly performing
// `drop` methods or do significant work in their `drop` methods.
// 2. the thread is shared across the whole library. This can be tuned by using `android_cleaner = true`,
// or `android = true` in the [`kotlin` section of the `uniffi.toml` file](https://mozilla.github.io/uniffi-rs/kotlin/configuration.html).
//
// If we try to implement this with mutual exclusion on access to the pointer, there is the
// possibility of a race between a method call and a concurrent call to `destroy`:
//
// * Thread A starts a method call, reads the value of the pointer, but is interrupted
// before it can pass the pointer over the FFI to Rust.
// * Thread B calls `destroy` and frees the underlying Rust struct.
// * Thread A resumes, passing the already-read pointer value to Rust and triggering
// a use-after-free.
//
// One possible solution would be to use a `ReadWriteLock`, with each method call taking
// a read lock (and thus allowed to run concurrently) and the special `destroy` method
// taking a write lock (and thus blocking on live method calls). However, we aim not to
// generate methods with any hidden blocking semantics, and a `destroy` method that might
// block if called incorrectly seems to meet that bar.
//
// So, we achieve our goals by giving each instance an associated `AtomicLong` counter to track
// the number of in-flight method calls, and an `AtomicBoolean` flag to indicate whether `destroy`
// has been called. These are updated according to the following rules:
//
// * The initial value of the counter is 1, indicating a live object with no in-flight calls.
// The initial value for the flag is false.
//
// * At the start of each method call, we atomically check the counter.
// If it is 0 then the underlying Rust struct has already been destroyed and the call is aborted.
// If it is nonzero them we atomically increment it by 1 and proceed with the method call.
//
// * At the end of each method call, we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// * When `destroy` is called, we atomically flip the flag from false to true.
// If the flag was already true we silently fail.
// Otherwise we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// Astute readers may observe that this all sounds very similar to the way that Rust's `Arc` works,
// and indeed it is, with the addition of a flag to guard against multiple calls to `destroy`.
//
// The overall effect is that the underlying Rust struct is destroyed only when `destroy` has been
// called *and* all in-flight method calls have completed, avoiding violating any of the expectations
// of the underlying Rust code.
//
// This makes a cleaner a better alternative to _not_ calling `destroy()` as
// and when the object is finished with, but the abstraction is not perfect: if the Rust object's `drop`
// method is slow, and/or there are many objects to cleanup, and it's on a low end Android device, then the cleaner
// thread may be starved, and the app will leak memory.
//
// In this case, `destroy`ing manually may be a better solution.
//
// The cleaner can live side by side with the manual calling of `destroy`. In the order of responsiveness, uniffi objects
// with Rust peers are reclaimed:
//
// 1. By calling the `destroy` method of the object, which calls `rustObject.free()`. If that doesn't happen:
// 2. When the object becomes unreachable, AND the Cleaner thread gets to call `rustObject.free()`. If the thread is starved then:
// 3. The memory is reclaimed when the process terminates.
//
// [1] https://stackoverflow.com/questions/24376768/can-java-finalize-an-object-when-it-is-still-in-scope/24380219
//
/**
* This trait is used to provide a callback mechanism to hook up the rerspective platform logging system
*/
public interface CoreCryptoLogger {
/**
* Function to setup a hook for the logging messages. Core Crypto will call this method
* whenever it needs to log a message.
*/
fun `log`(`level`: CoreCryptoLogLevel, `jsonMsg`: kotlin.String)
companion object
}
/**
* This trait is used to provide a callback mechanism to hook up the rerspective platform logging system
*/
open class CoreCryptoLoggerImpl: Disposable, AutoCloseable, CoreCryptoLogger {
constructor(pointer: Pointer) {
this.pointer = pointer
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
/**
* This constructor can be used to instantiate a fake object. Only used for tests. Any
* attempt to actually use an object constructed this way will fail as there is no
* connected Rust object.
*/
@Suppress("UNUSED_PARAMETER")
constructor(noPointer: NoPointer) {
this.pointer = null
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
protected val pointer: Pointer?
protected val cleanable: UniffiCleaner.Cleanable
private val wasDestroyed = AtomicBoolean(false)
private val callCounter = AtomicLong(1)
override fun destroy() {
// Only allow a single call to this method.
// TODO: maybe we should log a warning if called more than once?
if (this.wasDestroyed.compareAndSet(false, true)) {
// This decrement always matches the initial count of 1 given at creation time.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
@Synchronized
override fun close() {
this.destroy()
}
internal inline fun callWithPointer(block: (ptr: Pointer) -> R): R {
// Check and increment the call counter, to keep the object alive.
// This needs a compare-and-set retry loop in case of concurrent updates.
do {
val c = this.callCounter.get()
if (c == 0L) {
throw IllegalStateException("${this.javaClass.simpleName} object has already been destroyed")
}
if (c == Long.MAX_VALUE) {
throw IllegalStateException("${this.javaClass.simpleName} call counter would overflow")
}
} while (! this.callCounter.compareAndSet(c, c + 1L))
// Now we can safely do the method call without the pointer being freed concurrently.
try {
return block(this.uniffiClonePointer())
} finally {
// This decrement always matches the increment we performed above.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
// Use a static inner class instead of a closure so as not to accidentally
// capture `this` as part of the cleanable's action.
private class UniffiCleanAction(private val pointer: Pointer?) : Runnable {
override fun run() {
pointer?.let { ptr ->
uniffiRustCall { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_free_corecryptologger(ptr, status)
}
}
}
}
fun uniffiClonePointer(): Pointer {
return uniffiRustCall() { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_clone_corecryptologger(pointer!!, status)
}
}
/**
* Function to setup a hook for the logging messages. Core Crypto will call this method
* whenever it needs to log a message.
*/override fun `log`(`level`: CoreCryptoLogLevel, `jsonMsg`: kotlin.String)
=
callWithPointer {
uniffiRustCall() { _status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_corecryptologger_log(
it, FfiConverterTypeCoreCryptoLogLevel.lower(`level`),FfiConverterString.lower(`jsonMsg`),_status)
}
}
companion object
}
// Put the implementation in an object so we don't pollute the top-level namespace
internal object uniffiCallbackInterfaceCoreCryptoLogger {
internal object `log`: UniffiCallbackInterfaceCoreCryptoLoggerMethod0 {
override fun callback(`uniffiHandle`: Long,`level`: RustBuffer.ByValue,`jsonMsg`: RustBuffer.ByValue,`uniffiOutReturn`: Pointer,uniffiCallStatus: UniffiRustCallStatus,) {
val uniffiObj = FfiConverterTypeCoreCryptoLogger.handleMap.get(uniffiHandle)
val makeCall = { ->
uniffiObj.`log`(
FfiConverterTypeCoreCryptoLogLevel.lift(`level`),
FfiConverterString.lift(`jsonMsg`),
)
}
val writeReturn = { _: Unit -> Unit }
uniffiTraitInterfaceCall(uniffiCallStatus, makeCall, writeReturn)
}
}
internal object uniffiFree: UniffiCallbackInterfaceFree {
override fun callback(handle: Long) {
FfiConverterTypeCoreCryptoLogger.handleMap.remove(handle)
}
}
internal var vtable = UniffiVTableCallbackInterfaceCoreCryptoLogger.UniffiByValue(
`log`,
uniffiFree,
)
// Registers the foreign callback with the Rust side.
// This method is generated for each callback interface.
internal fun register(lib: UniffiLib) {
lib.uniffi_core_crypto_ffi_fn_init_callback_vtable_corecryptologger(vtable)
}
}
public object FfiConverterTypeCoreCryptoLogger: FfiConverter {
internal val handleMap = UniffiHandleMap()
override fun lower(value: CoreCryptoLogger): Pointer {
return Pointer(handleMap.insert(value))
}
override fun lift(value: Pointer): CoreCryptoLogger {
return CoreCryptoLoggerImpl(value)
}
override fun read(buf: ByteBuffer): CoreCryptoLogger {
// The Rust code always writes pointers as 8 bytes, and will
// fail to compile if they don't fit.
return lift(Pointer(buf.getLong()))
}
override fun allocationSize(value: CoreCryptoLogger) = 8UL
override fun write(value: CoreCryptoLogger, buf: ByteBuffer) {
// The Rust code always expects pointers written as 8 bytes,
// and will fail to compile if they don't fit.
buf.putLong(Pointer.nativeValue(lower(value)))
}
}
// This template implements a class for working with a Rust struct via a Pointer/Arc
// to the live Rust struct on the other side of the FFI.
//
// Each instance implements core operations for working with the Rust `Arc` and the
// Kotlin Pointer to work with the live Rust struct on the other side of the FFI.
//
// There's some subtlety here, because we have to be careful not to operate on a Rust
// struct after it has been dropped, and because we must expose a public API for freeing
// theq Kotlin wrapper object in lieu of reliable finalizers. The core requirements are:
//
// * Each instance holds an opaque pointer to the underlying Rust struct.
// Method calls need to read this pointer from the object's state and pass it in to
// the Rust FFI.
//
// * When an instance is no longer needed, its pointer should be passed to a
// special destructor function provided by the Rust FFI, which will drop the
// underlying Rust struct.
//
// * Given an instance, calling code is expected to call the special
// `destroy` method in order to free it after use, either by calling it explicitly
// or by using a higher-level helper like the `use` method. Failing to do so risks
// leaking the underlying Rust struct.
//
// * We can't assume that calling code will do the right thing, and must be prepared
// to handle Kotlin method calls executing concurrently with or even after a call to
// `destroy`, and to handle multiple (possibly concurrent!) calls to `destroy`.
//
// * We must never allow Rust code to operate on the underlying Rust struct after
// the destructor has been called, and must never call the destructor more than once.
// Doing so may trigger memory unsafety.
//
// * To mitigate many of the risks of leaking memory and use-after-free unsafety, a `Cleaner`
// is implemented to call the destructor when the Kotlin object becomes unreachable.
// This is done in a background thread. This is not a panacea, and client code should be aware that
// 1. the thread may starve if some there are objects that have poorly performing
// `drop` methods or do significant work in their `drop` methods.
// 2. the thread is shared across the whole library. This can be tuned by using `android_cleaner = true`,
// or `android = true` in the [`kotlin` section of the `uniffi.toml` file](https://mozilla.github.io/uniffi-rs/kotlin/configuration.html).
//
// If we try to implement this with mutual exclusion on access to the pointer, there is the
// possibility of a race between a method call and a concurrent call to `destroy`:
//
// * Thread A starts a method call, reads the value of the pointer, but is interrupted
// before it can pass the pointer over the FFI to Rust.
// * Thread B calls `destroy` and frees the underlying Rust struct.
// * Thread A resumes, passing the already-read pointer value to Rust and triggering
// a use-after-free.
//
// One possible solution would be to use a `ReadWriteLock`, with each method call taking
// a read lock (and thus allowed to run concurrently) and the special `destroy` method
// taking a write lock (and thus blocking on live method calls). However, we aim not to
// generate methods with any hidden blocking semantics, and a `destroy` method that might
// block if called incorrectly seems to meet that bar.
//
// So, we achieve our goals by giving each instance an associated `AtomicLong` counter to track
// the number of in-flight method calls, and an `AtomicBoolean` flag to indicate whether `destroy`
// has been called. These are updated according to the following rules:
//
// * The initial value of the counter is 1, indicating a live object with no in-flight calls.
// The initial value for the flag is false.
//
// * At the start of each method call, we atomically check the counter.
// If it is 0 then the underlying Rust struct has already been destroyed and the call is aborted.
// If it is nonzero them we atomically increment it by 1 and proceed with the method call.
//
// * At the end of each method call, we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// * When `destroy` is called, we atomically flip the flag from false to true.
// If the flag was already true we silently fail.
// Otherwise we atomically decrement and check the counter.
// If it has reached zero then we destroy the underlying Rust struct.
//
// Astute readers may observe that this all sounds very similar to the way that Rust's `Arc` works,
// and indeed it is, with the addition of a flag to guard against multiple calls to `destroy`.
//
// The overall effect is that the underlying Rust struct is destroyed only when `destroy` has been
// called *and* all in-flight method calls have completed, avoiding violating any of the expectations
// of the underlying Rust code.
//
// This makes a cleaner a better alternative to _not_ calling `destroy()` as
// and when the object is finished with, but the abstraction is not perfect: if the Rust object's `drop`
// method is slow, and/or there are many objects to cleanup, and it's on a low end Android device, then the cleaner
// thread may be starved, and the app will leak memory.
//
// In this case, `destroy`ing manually may be a better solution.
//
// The cleaner can live side by side with the manual calling of `destroy`. In the order of responsiveness, uniffi objects
// with Rust peers are reclaimed:
//
// 1. By calling the `destroy` method of the object, which calls `rustObject.free()`. If that doesn't happen:
// 2. When the object becomes unreachable, AND the Cleaner thread gets to call `rustObject.free()`. If the thread is starved then:
// 3. The memory is reclaimed when the process terminates.
//
// [1] https://stackoverflow.com/questions/24376768/can-java-finalize-an-object-when-it-is-still-in-scope/24380219
//
/**
* See [core_crypto::e2e_identity::E2eiEnrollment]
*/
public interface E2eiEnrollmentInterface {
/**
* See [core_crypto::prelude::E2eiEnrollment::certificate_request]
*/
suspend fun `certificateRequest`(`previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::check_order_request]
*/
suspend fun `checkOrderRequest`(`orderUrl`: kotlin.String, `previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::check_order_response]
*/
suspend fun `checkOrderResponse`(`order`: kotlin.ByteArray): kotlin.String
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_oidc_challenge_response]
*/
suspend fun `contextNewOidcChallengeResponse`(`cc`: CoreCryptoContext, `challenge`: kotlin.ByteArray)
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::create_dpop_token]
*/
suspend fun `createDpopToken`(`expirySecs`: kotlin.UInt, `backendNonce`: kotlin.String): kotlin.String
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::directory_response]
*/
suspend fun `directoryResponse`(`directory`: kotlin.ByteArray): AcmeDirectory
/**
* See [core_crypto::prelude::E2eiEnrollment::finalize_request]
*/
suspend fun `finalizeRequest`(`previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::prelude::E2eiEnrollment::finalize_response]
*/
suspend fun `finalizeResponse`(`finalize`: kotlin.ByteArray): kotlin.String
/**
* See [core_crypto::prelude::E2eiEnrollment::get_refresh_token]
*/
suspend fun `getRefreshToken`(): kotlin.String
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_account_request]
*/
suspend fun `newAccountRequest`(`previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_account_response]
*/
suspend fun `newAccountResponse`(`account`: kotlin.ByteArray)
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_authz_request]
*/
suspend fun `newAuthzRequest`(`url`: kotlin.String, `previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_authz_response]
*/
suspend fun `newAuthzResponse`(`authz`: kotlin.ByteArray): NewAcmeAuthz
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_dpop_challenge_request]
*/
suspend fun `newDpopChallengeRequest`(`accessToken`: kotlin.String, `previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_dpop_challenge_response]
*/
suspend fun `newDpopChallengeResponse`(`challenge`: kotlin.ByteArray)
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_oidc_challenge_request]
*/
suspend fun `newOidcChallengeRequest`(`idToken`: kotlin.String, `refreshToken`: kotlin.String, `previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_oidc_challenge_response]
*/
suspend fun `newOidcChallengeResponse`(`cc`: CoreCrypto, `challenge`: kotlin.ByteArray)
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_order_request]
*/
suspend fun `newOrderRequest`(`previousNonce`: kotlin.String): kotlin.ByteArray
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_order_response]
*/
suspend fun `newOrderResponse`(`order`: kotlin.ByteArray): NewAcmeOrder
companion object
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment]
*/
open class E2eiEnrollment: Disposable, AutoCloseable, E2eiEnrollmentInterface {
constructor(pointer: Pointer) {
this.pointer = pointer
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
/**
* This constructor can be used to instantiate a fake object. Only used for tests. Any
* attempt to actually use an object constructed this way will fail as there is no
* connected Rust object.
*/
@Suppress("UNUSED_PARAMETER")
constructor(noPointer: NoPointer) {
this.pointer = null
this.cleanable = UniffiLib.CLEANER.register(this, UniffiCleanAction(pointer))
}
protected val pointer: Pointer?
protected val cleanable: UniffiCleaner.Cleanable
private val wasDestroyed = AtomicBoolean(false)
private val callCounter = AtomicLong(1)
override fun destroy() {
// Only allow a single call to this method.
// TODO: maybe we should log a warning if called more than once?
if (this.wasDestroyed.compareAndSet(false, true)) {
// This decrement always matches the initial count of 1 given at creation time.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
@Synchronized
override fun close() {
this.destroy()
}
internal inline fun callWithPointer(block: (ptr: Pointer) -> R): R {
// Check and increment the call counter, to keep the object alive.
// This needs a compare-and-set retry loop in case of concurrent updates.
do {
val c = this.callCounter.get()
if (c == 0L) {
throw IllegalStateException("${this.javaClass.simpleName} object has already been destroyed")
}
if (c == Long.MAX_VALUE) {
throw IllegalStateException("${this.javaClass.simpleName} call counter would overflow")
}
} while (! this.callCounter.compareAndSet(c, c + 1L))
// Now we can safely do the method call without the pointer being freed concurrently.
try {
return block(this.uniffiClonePointer())
} finally {
// This decrement always matches the increment we performed above.
if (this.callCounter.decrementAndGet() == 0L) {
cleanable.clean()
}
}
}
// Use a static inner class instead of a closure so as not to accidentally
// capture `this` as part of the cleanable's action.
private class UniffiCleanAction(private val pointer: Pointer?) : Runnable {
override fun run() {
pointer?.let { ptr ->
uniffiRustCall { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_free_e2eienrollment(ptr, status)
}
}
}
}
fun uniffiClonePointer(): Pointer {
return uniffiRustCall() { status ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_clone_e2eienrollment(pointer!!, status)
}
}
/**
* See [core_crypto::prelude::E2eiEnrollment::certificate_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `certificateRequest`(`previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_certificate_request(
thisPtr,
FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::check_order_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `checkOrderRequest`(`orderUrl`: kotlin.String, `previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_check_order_request(
thisPtr,
FfiConverterString.lower(`orderUrl`),FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::check_order_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `checkOrderResponse`(`order`: kotlin.ByteArray) : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_check_order_response(
thisPtr,
FfiConverterByteArray.lower(`order`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_oidc_challenge_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `contextNewOidcChallengeResponse`(`cc`: CoreCryptoContext, `challenge`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_context_new_oidc_challenge_response(
thisPtr,
FfiConverterTypeCoreCryptoContext.lower(`cc`),FfiConverterByteArray.lower(`challenge`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::create_dpop_token]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `createDpopToken`(`expirySecs`: kotlin.UInt, `backendNonce`: kotlin.String) : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_create_dpop_token(
thisPtr,
FfiConverterUInt.lower(`expirySecs`),FfiConverterString.lower(`backendNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::directory_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `directoryResponse`(`directory`: kotlin.ByteArray) : AcmeDirectory {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_directory_response(
thisPtr,
FfiConverterByteArray.lower(`directory`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeAcmeDirectory.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::prelude::E2eiEnrollment::finalize_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `finalizeRequest`(`previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_finalize_request(
thisPtr,
FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::prelude::E2eiEnrollment::finalize_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `finalizeResponse`(`finalize`: kotlin.ByteArray) : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_finalize_response(
thisPtr,
FfiConverterByteArray.lower(`finalize`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::prelude::E2eiEnrollment::get_refresh_token]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `getRefreshToken`() : kotlin.String {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_get_refresh_token(
thisPtr,
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterString.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_account_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newAccountRequest`(`previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_account_request(
thisPtr,
FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_account_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newAccountResponse`(`account`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_account_response(
thisPtr,
FfiConverterByteArray.lower(`account`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_authz_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newAuthzRequest`(`url`: kotlin.String, `previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_authz_request(
thisPtr,
FfiConverterString.lower(`url`),FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_authz_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newAuthzResponse`(`authz`: kotlin.ByteArray) : NewAcmeAuthz {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_authz_response(
thisPtr,
FfiConverterByteArray.lower(`authz`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeNewAcmeAuthz.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_dpop_challenge_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newDpopChallengeRequest`(`accessToken`: kotlin.String, `previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_dpop_challenge_request(
thisPtr,
FfiConverterString.lower(`accessToken`),FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_dpop_challenge_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newDpopChallengeResponse`(`challenge`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_dpop_challenge_response(
thisPtr,
FfiConverterByteArray.lower(`challenge`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_oidc_challenge_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newOidcChallengeRequest`(`idToken`: kotlin.String, `refreshToken`: kotlin.String, `previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_oidc_challenge_request(
thisPtr,
FfiConverterString.lower(`idToken`),FfiConverterString.lower(`refreshToken`),FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_oidc_challenge_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newOidcChallengeResponse`(`cc`: CoreCrypto, `challenge`: kotlin.ByteArray) {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_oidc_challenge_response(
thisPtr,
FfiConverterTypeCoreCrypto.lower(`cc`),FfiConverterByteArray.lower(`challenge`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_void(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_void(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_void(future) },
// lift function
{ Unit },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_order_request]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newOrderRequest`(`previousNonce`: kotlin.String) : kotlin.ByteArray {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_order_request(
thisPtr,
FfiConverterString.lower(`previousNonce`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterByteArray.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
/**
* See [core_crypto::e2e_identity::E2eiEnrollment::new_order_response]
*/
@Throws(CoreCryptoException::class)
@Suppress("ASSIGNED_BUT_NEVER_ACCESSED_VARIABLE")
override suspend fun `newOrderResponse`(`order`: kotlin.ByteArray) : NewAcmeOrder {
return uniffiRustCallAsync(
callWithPointer { thisPtr ->
UniffiLib.INSTANCE.uniffi_core_crypto_ffi_fn_method_e2eienrollment_new_order_response(
thisPtr,
FfiConverterByteArray.lower(`order`),
)
},
{ future, callback, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_poll_rust_buffer(future, callback, continuation) },
{ future, continuation -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_complete_rust_buffer(future, continuation) },
{ future -> UniffiLib.INSTANCE.ffi_core_crypto_ffi_rust_future_free_rust_buffer(future) },
// lift function
{ FfiConverterTypeNewAcmeOrder.lift(it) },
// Error FFI converter
CoreCryptoException.ErrorHandler,
)
}
companion object
}
public object FfiConverterTypeE2eiEnrollment: FfiConverter {
override fun lower(value: E2eiEnrollment): Pointer {
return value.uniffiClonePointer()
}
override fun lift(value: Pointer): E2eiEnrollment {
return E2eiEnrollment(value)
}
override fun read(buf: ByteBuffer): E2eiEnrollment {
// The Rust code always writes pointers as 8 bytes, and will
// fail to compile if they don't fit.
return lift(Pointer(buf.getLong()))
}
override fun allocationSize(value: E2eiEnrollment) = 8UL
override fun write(value: E2eiEnrollment, buf: ByteBuffer) {
// The Rust code always expects pointers written as 8 bytes,
// and will fail to compile if they don't fit.
buf.putLong(Pointer.nativeValue(lower(value)))
}
}
/**
* See [core_crypto::e2e_identity::types::E2eiAcmeChallenge]
*/
data class AcmeChallenge (
var `delegate`: kotlin.ByteArray,
var `url`: kotlin.String,
var `target`: kotlin.String
) {
companion object
}
public object FfiConverterTypeAcmeChallenge: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): AcmeChallenge {
return AcmeChallenge(
FfiConverterByteArray.read(buf),
FfiConverterString.read(buf),
FfiConverterString.read(buf),
)
}
override fun allocationSize(value: AcmeChallenge) = (
FfiConverterByteArray.allocationSize(value.`delegate`) +
FfiConverterString.allocationSize(value.`url`) +
FfiConverterString.allocationSize(value.`target`)
)
override fun write(value: AcmeChallenge, buf: ByteBuffer) {
FfiConverterByteArray.write(value.`delegate`, buf)
FfiConverterString.write(value.`url`, buf)
FfiConverterString.write(value.`target`, buf)
}
}
/**
* See [core_crypto::e2e_identity::types::E2eiAcmeDirectory]
*/
data class AcmeDirectory (
var `newNonce`: kotlin.String,
var `newAccount`: kotlin.String,
var `newOrder`: kotlin.String,
var `revokeCert`: kotlin.String
) {
companion object
}
public object FfiConverterTypeAcmeDirectory: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): AcmeDirectory {
return AcmeDirectory(
FfiConverterString.read(buf),
FfiConverterString.read(buf),
FfiConverterString.read(buf),
FfiConverterString.read(buf),
)
}
override fun allocationSize(value: AcmeDirectory) = (
FfiConverterString.allocationSize(value.`newNonce`) +
FfiConverterString.allocationSize(value.`newAccount`) +
FfiConverterString.allocationSize(value.`newOrder`) +
FfiConverterString.allocationSize(value.`revokeCert`)
)
override fun write(value: AcmeDirectory, buf: ByteBuffer) {
FfiConverterString.write(value.`newNonce`, buf)
FfiConverterString.write(value.`newAccount`, buf)
FfiConverterString.write(value.`newOrder`, buf)
FfiConverterString.write(value.`revokeCert`, buf)
}
}
/**
* because Uniffi does not support recursive structs
*/
data class BufferedDecryptedMessage (
var `message`: kotlin.ByteArray?,
var `proposals`: List,
var `isActive`: kotlin.Boolean,
var `commitDelay`: kotlin.ULong?,
var `senderClientId`: ClientId?,
var `hasEpochChanged`: kotlin.Boolean,
var `identity`: WireIdentity,
var `crlNewDistributionPoints`: List?
) {
companion object
}
public object FfiConverterTypeBufferedDecryptedMessage: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): BufferedDecryptedMessage {
return BufferedDecryptedMessage(
FfiConverterOptionalByteArray.read(buf),
FfiConverterSequenceTypeProposalBundle.read(buf),
FfiConverterBoolean.read(buf),
FfiConverterOptionalULong.read(buf),
FfiConverterOptionalTypeClientId.read(buf),
FfiConverterBoolean.read(buf),
FfiConverterTypeWireIdentity.read(buf),
FfiConverterOptionalSequenceString.read(buf),
)
}
override fun allocationSize(value: BufferedDecryptedMessage) = (
FfiConverterOptionalByteArray.allocationSize(value.`message`) +
FfiConverterSequenceTypeProposalBundle.allocationSize(value.`proposals`) +
FfiConverterBoolean.allocationSize(value.`isActive`) +
FfiConverterOptionalULong.allocationSize(value.`commitDelay`) +
FfiConverterOptionalTypeClientId.allocationSize(value.`senderClientId`) +
FfiConverterBoolean.allocationSize(value.`hasEpochChanged`) +
FfiConverterTypeWireIdentity.allocationSize(value.`identity`) +
FfiConverterOptionalSequenceString.allocationSize(value.`crlNewDistributionPoints`)
)
override fun write(value: BufferedDecryptedMessage, buf: ByteBuffer) {
FfiConverterOptionalByteArray.write(value.`message`, buf)
FfiConverterSequenceTypeProposalBundle.write(value.`proposals`, buf)
FfiConverterBoolean.write(value.`isActive`, buf)
FfiConverterOptionalULong.write(value.`commitDelay`, buf)
FfiConverterOptionalTypeClientId.write(value.`senderClientId`, buf)
FfiConverterBoolean.write(value.`hasEpochChanged`, buf)
FfiConverterTypeWireIdentity.write(value.`identity`, buf)
FfiConverterOptionalSequenceString.write(value.`crlNewDistributionPoints`, buf)
}
}
data class CommitBundle (
var `welcome`: kotlin.ByteArray?,
var `commit`: kotlin.ByteArray,
var `groupInfo`: GroupInfoBundle
) {
companion object
}
public object FfiConverterTypeCommitBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): CommitBundle {
return CommitBundle(
FfiConverterOptionalByteArray.read(buf),
FfiConverterByteArray.read(buf),
FfiConverterTypeGroupInfoBundle.read(buf),
)
}
override fun allocationSize(value: CommitBundle) = (
FfiConverterOptionalByteArray.allocationSize(value.`welcome`) +
FfiConverterByteArray.allocationSize(value.`commit`) +
FfiConverterTypeGroupInfoBundle.allocationSize(value.`groupInfo`)
)
override fun write(value: CommitBundle, buf: ByteBuffer) {
FfiConverterOptionalByteArray.write(value.`welcome`, buf)
FfiConverterByteArray.write(value.`commit`, buf)
FfiConverterTypeGroupInfoBundle.write(value.`groupInfo`, buf)
}
}
/**
* See [core_crypto::prelude::MlsConversationConfiguration]
*/
data class ConversationConfiguration (
var `ciphersuite`: Ciphersuite,
var `externalSenders`: List,
var `custom`: CustomConfiguration
) {
companion object
}
public object FfiConverterTypeConversationConfiguration: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): ConversationConfiguration {
return ConversationConfiguration(
FfiConverterTypeCiphersuite.read(buf),
FfiConverterSequenceByteArray.read(buf),
FfiConverterTypeCustomConfiguration.read(buf),
)
}
override fun allocationSize(value: ConversationConfiguration) = (
FfiConverterTypeCiphersuite.allocationSize(value.`ciphersuite`) +
FfiConverterSequenceByteArray.allocationSize(value.`externalSenders`) +
FfiConverterTypeCustomConfiguration.allocationSize(value.`custom`)
)
override fun write(value: ConversationConfiguration, buf: ByteBuffer) {
FfiConverterTypeCiphersuite.write(value.`ciphersuite`, buf)
FfiConverterSequenceByteArray.write(value.`externalSenders`, buf)
FfiConverterTypeCustomConfiguration.write(value.`custom`, buf)
}
}
data class ConversationInitBundle (
var `conversationId`: kotlin.ByteArray,
var `commit`: kotlin.ByteArray,
var `groupInfo`: GroupInfoBundle,
var `crlNewDistributionPoints`: List?
) {
companion object
}
public object FfiConverterTypeConversationInitBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): ConversationInitBundle {
return ConversationInitBundle(
FfiConverterByteArray.read(buf),
FfiConverterByteArray.read(buf),
FfiConverterTypeGroupInfoBundle.read(buf),
FfiConverterOptionalSequenceString.read(buf),
)
}
override fun allocationSize(value: ConversationInitBundle) = (
FfiConverterByteArray.allocationSize(value.`conversationId`) +
FfiConverterByteArray.allocationSize(value.`commit`) +
FfiConverterTypeGroupInfoBundle.allocationSize(value.`groupInfo`) +
FfiConverterOptionalSequenceString.allocationSize(value.`crlNewDistributionPoints`)
)
override fun write(value: ConversationInitBundle, buf: ByteBuffer) {
FfiConverterByteArray.write(value.`conversationId`, buf)
FfiConverterByteArray.write(value.`commit`, buf)
FfiConverterTypeGroupInfoBundle.write(value.`groupInfo`, buf)
FfiConverterOptionalSequenceString.write(value.`crlNewDistributionPoints`, buf)
}
}
/**
* Supporting struct for CRL registration result
*/
data class CrlRegistration (
/**
* Whether this CRL modifies the old CRL (i.e. has a different revocated cert list)
*/
var `dirty`: kotlin.Boolean,
/**
* Optional expiration timestamp
*/
var `expiration`: kotlin.ULong?
) {
companion object
}
public object FfiConverterTypeCrlRegistration: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): CrlRegistration {
return CrlRegistration(
FfiConverterBoolean.read(buf),
FfiConverterOptionalULong.read(buf),
)
}
override fun allocationSize(value: CrlRegistration) = (
FfiConverterBoolean.allocationSize(value.`dirty`) +
FfiConverterOptionalULong.allocationSize(value.`expiration`)
)
override fun write(value: CrlRegistration, buf: ByteBuffer) {
FfiConverterBoolean.write(value.`dirty`, buf)
FfiConverterOptionalULong.write(value.`expiration`, buf)
}
}
/**
* See [core_crypto::prelude::MlsCustomConfiguration]
*/
data class CustomConfiguration (
var `keyRotationSpan`: java.time.Duration?,
var `wirePolicy`: MlsWirePolicy?
) {
companion object
}
public object FfiConverterTypeCustomConfiguration: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): CustomConfiguration {
return CustomConfiguration(
FfiConverterOptionalDuration.read(buf),
FfiConverterOptionalTypeMlsWirePolicy.read(buf),
)
}
override fun allocationSize(value: CustomConfiguration) = (
FfiConverterOptionalDuration.allocationSize(value.`keyRotationSpan`) +
FfiConverterOptionalTypeMlsWirePolicy.allocationSize(value.`wirePolicy`)
)
override fun write(value: CustomConfiguration, buf: ByteBuffer) {
FfiConverterOptionalDuration.write(value.`keyRotationSpan`, buf)
FfiConverterOptionalTypeMlsWirePolicy.write(value.`wirePolicy`, buf)
}
}
/**
* See [core_crypto::prelude::decrypt::MlsConversationDecryptMessage]
*/
data class DecryptedMessage (
var `message`: kotlin.ByteArray?,
var `proposals`: List,
var `isActive`: kotlin.Boolean,
var `commitDelay`: kotlin.ULong?,
var `senderClientId`: ClientId?,
var `hasEpochChanged`: kotlin.Boolean,
var `identity`: WireIdentity,
var `bufferedMessages`: List?,
var `crlNewDistributionPoints`: List?
) {
companion object
}
public object FfiConverterTypeDecryptedMessage: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): DecryptedMessage {
return DecryptedMessage(
FfiConverterOptionalByteArray.read(buf),
FfiConverterSequenceTypeProposalBundle.read(buf),
FfiConverterBoolean.read(buf),
FfiConverterOptionalULong.read(buf),
FfiConverterOptionalTypeClientId.read(buf),
FfiConverterBoolean.read(buf),
FfiConverterTypeWireIdentity.read(buf),
FfiConverterOptionalSequenceTypeBufferedDecryptedMessage.read(buf),
FfiConverterOptionalSequenceString.read(buf),
)
}
override fun allocationSize(value: DecryptedMessage) = (
FfiConverterOptionalByteArray.allocationSize(value.`message`) +
FfiConverterSequenceTypeProposalBundle.allocationSize(value.`proposals`) +
FfiConverterBoolean.allocationSize(value.`isActive`) +
FfiConverterOptionalULong.allocationSize(value.`commitDelay`) +
FfiConverterOptionalTypeClientId.allocationSize(value.`senderClientId`) +
FfiConverterBoolean.allocationSize(value.`hasEpochChanged`) +
FfiConverterTypeWireIdentity.allocationSize(value.`identity`) +
FfiConverterOptionalSequenceTypeBufferedDecryptedMessage.allocationSize(value.`bufferedMessages`) +
FfiConverterOptionalSequenceString.allocationSize(value.`crlNewDistributionPoints`)
)
override fun write(value: DecryptedMessage, buf: ByteBuffer) {
FfiConverterOptionalByteArray.write(value.`message`, buf)
FfiConverterSequenceTypeProposalBundle.write(value.`proposals`, buf)
FfiConverterBoolean.write(value.`isActive`, buf)
FfiConverterOptionalULong.write(value.`commitDelay`, buf)
FfiConverterOptionalTypeClientId.write(value.`senderClientId`, buf)
FfiConverterBoolean.write(value.`hasEpochChanged`, buf)
FfiConverterTypeWireIdentity.write(value.`identity`, buf)
FfiConverterOptionalSequenceTypeBufferedDecryptedMessage.write(value.`bufferedMessages`, buf)
FfiConverterOptionalSequenceString.write(value.`crlNewDistributionPoints`, buf)
}
}
/**
* Dummy comment
*/
data class E2eiDumpedPkiEnv (
var `rootCa`: kotlin.String,
var `intermediates`: List,
var `crls`: List
) {
companion object
}
public object FfiConverterTypeE2eiDumpedPkiEnv: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): E2eiDumpedPkiEnv {
return E2eiDumpedPkiEnv(
FfiConverterString.read(buf),
FfiConverterSequenceString.read(buf),
FfiConverterSequenceString.read(buf),
)
}
override fun allocationSize(value: E2eiDumpedPkiEnv) = (
FfiConverterString.allocationSize(value.`rootCa`) +
FfiConverterSequenceString.allocationSize(value.`intermediates`) +
FfiConverterSequenceString.allocationSize(value.`crls`)
)
override fun write(value: E2eiDumpedPkiEnv, buf: ByteBuffer) {
FfiConverterString.write(value.`rootCa`, buf)
FfiConverterSequenceString.write(value.`intermediates`, buf)
FfiConverterSequenceString.write(value.`crls`, buf)
}
}
data class GroupInfoBundle (
var `encryptionType`: MlsGroupInfoEncryptionType,
var `ratchetTreeType`: MlsRatchetTreeType,
var `payload`: kotlin.ByteArray
) {
companion object
}
public object FfiConverterTypeGroupInfoBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): GroupInfoBundle {
return GroupInfoBundle(
FfiConverterTypeMlsGroupInfoEncryptionType.read(buf),
FfiConverterTypeMlsRatchetTreeType.read(buf),
FfiConverterByteArray.read(buf),
)
}
override fun allocationSize(value: GroupInfoBundle) = (
FfiConverterTypeMlsGroupInfoEncryptionType.allocationSize(value.`encryptionType`) +
FfiConverterTypeMlsRatchetTreeType.allocationSize(value.`ratchetTreeType`) +
FfiConverterByteArray.allocationSize(value.`payload`)
)
override fun write(value: GroupInfoBundle, buf: ByteBuffer) {
FfiConverterTypeMlsGroupInfoEncryptionType.write(value.`encryptionType`, buf)
FfiConverterTypeMlsRatchetTreeType.write(value.`ratchetTreeType`, buf)
FfiConverterByteArray.write(value.`payload`, buf)
}
}
/**
* see [core_crypto::prelude::MlsConversationCreationMessage]
*/
data class MemberAddedMessages (
var `welcome`: kotlin.ByteArray,
var `commit`: kotlin.ByteArray,
var `groupInfo`: GroupInfoBundle,
var `crlNewDistributionPoints`: List?
) {
companion object
}
public object FfiConverterTypeMemberAddedMessages: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): MemberAddedMessages {
return MemberAddedMessages(
FfiConverterByteArray.read(buf),
FfiConverterByteArray.read(buf),
FfiConverterTypeGroupInfoBundle.read(buf),
FfiConverterOptionalSequenceString.read(buf),
)
}
override fun allocationSize(value: MemberAddedMessages) = (
FfiConverterByteArray.allocationSize(value.`welcome`) +
FfiConverterByteArray.allocationSize(value.`commit`) +
FfiConverterTypeGroupInfoBundle.allocationSize(value.`groupInfo`) +
FfiConverterOptionalSequenceString.allocationSize(value.`crlNewDistributionPoints`)
)
override fun write(value: MemberAddedMessages, buf: ByteBuffer) {
FfiConverterByteArray.write(value.`welcome`, buf)
FfiConverterByteArray.write(value.`commit`, buf)
FfiConverterTypeGroupInfoBundle.write(value.`groupInfo`, buf)
FfiConverterOptionalSequenceString.write(value.`crlNewDistributionPoints`, buf)
}
}
/**
* See [core_crypto::e2e_identity::types::E2eiNewAcmeAuthz]
*/
data class NewAcmeAuthz (
var `identifier`: kotlin.String,
var `keyauth`: kotlin.String?,
var `challenge`: AcmeChallenge
) {
companion object
}
public object FfiConverterTypeNewAcmeAuthz: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): NewAcmeAuthz {
return NewAcmeAuthz(
FfiConverterString.read(buf),
FfiConverterOptionalString.read(buf),
FfiConverterTypeAcmeChallenge.read(buf),
)
}
override fun allocationSize(value: NewAcmeAuthz) = (
FfiConverterString.allocationSize(value.`identifier`) +
FfiConverterOptionalString.allocationSize(value.`keyauth`) +
FfiConverterTypeAcmeChallenge.allocationSize(value.`challenge`)
)
override fun write(value: NewAcmeAuthz, buf: ByteBuffer) {
FfiConverterString.write(value.`identifier`, buf)
FfiConverterOptionalString.write(value.`keyauth`, buf)
FfiConverterTypeAcmeChallenge.write(value.`challenge`, buf)
}
}
/**
* See [core_crypto::e2e_identity::types::E2eiNewAcmeOrder]
*/
data class NewAcmeOrder (
var `delegate`: kotlin.ByteArray,
var `authorizations`: List
) {
companion object
}
public object FfiConverterTypeNewAcmeOrder: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): NewAcmeOrder {
return NewAcmeOrder(
FfiConverterByteArray.read(buf),
FfiConverterSequenceString.read(buf),
)
}
override fun allocationSize(value: NewAcmeOrder) = (
FfiConverterByteArray.allocationSize(value.`delegate`) +
FfiConverterSequenceString.allocationSize(value.`authorizations`)
)
override fun write(value: NewAcmeOrder, buf: ByteBuffer) {
FfiConverterByteArray.write(value.`delegate`, buf)
FfiConverterSequenceString.write(value.`authorizations`, buf)
}
}
data class ProposalBundle (
var `proposal`: kotlin.ByteArray,
var `proposalRef`: kotlin.ByteArray,
var `crlNewDistributionPoints`: List?
) {
companion object
}
public object FfiConverterTypeProposalBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): ProposalBundle {
return ProposalBundle(
FfiConverterByteArray.read(buf),
FfiConverterByteArray.read(buf),
FfiConverterOptionalSequenceString.read(buf),
)
}
override fun allocationSize(value: ProposalBundle) = (
FfiConverterByteArray.allocationSize(value.`proposal`) +
FfiConverterByteArray.allocationSize(value.`proposalRef`) +
FfiConverterOptionalSequenceString.allocationSize(value.`crlNewDistributionPoints`)
)
override fun write(value: ProposalBundle, buf: ByteBuffer) {
FfiConverterByteArray.write(value.`proposal`, buf)
FfiConverterByteArray.write(value.`proposalRef`, buf)
FfiConverterOptionalSequenceString.write(value.`crlNewDistributionPoints`, buf)
}
}
data class ProteusAutoPrekeyBundle (
var `id`: kotlin.UShort,
var `pkb`: kotlin.ByteArray
) {
companion object
}
public object FfiConverterTypeProteusAutoPrekeyBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): ProteusAutoPrekeyBundle {
return ProteusAutoPrekeyBundle(
FfiConverterUShort.read(buf),
FfiConverterByteArray.read(buf),
)
}
override fun allocationSize(value: ProteusAutoPrekeyBundle) = (
FfiConverterUShort.allocationSize(value.`id`) +
FfiConverterByteArray.allocationSize(value.`pkb`)
)
override fun write(value: ProteusAutoPrekeyBundle, buf: ByteBuffer) {
FfiConverterUShort.write(value.`id`, buf)
FfiConverterByteArray.write(value.`pkb`, buf)
}
}
data class RotateBundle (
var `commits`: Map,
var `newKeyPackages`: List,
var `keyPackageRefsToRemove`: List,
var `crlNewDistributionPoints`: List?
) {
companion object
}
public object FfiConverterTypeRotateBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): RotateBundle {
return RotateBundle(
FfiConverterMapStringTypeCommitBundle.read(buf),
FfiConverterSequenceByteArray.read(buf),
FfiConverterSequenceByteArray.read(buf),
FfiConverterOptionalSequenceString.read(buf),
)
}
override fun allocationSize(value: RotateBundle) = (
FfiConverterMapStringTypeCommitBundle.allocationSize(value.`commits`) +
FfiConverterSequenceByteArray.allocationSize(value.`newKeyPackages`) +
FfiConverterSequenceByteArray.allocationSize(value.`keyPackageRefsToRemove`) +
FfiConverterOptionalSequenceString.allocationSize(value.`crlNewDistributionPoints`)
)
override fun write(value: RotateBundle, buf: ByteBuffer) {
FfiConverterMapStringTypeCommitBundle.write(value.`commits`, buf)
FfiConverterSequenceByteArray.write(value.`newKeyPackages`, buf)
FfiConverterSequenceByteArray.write(value.`keyPackageRefsToRemove`, buf)
FfiConverterOptionalSequenceString.write(value.`crlNewDistributionPoints`, buf)
}
}
/**
* see [core_crypto::prelude::MlsConversationCreationMessage]
*/
data class WelcomeBundle (
var `id`: kotlin.ByteArray,
var `crlNewDistributionPoints`: List?
) {
companion object
}
public object FfiConverterTypeWelcomeBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): WelcomeBundle {
return WelcomeBundle(
FfiConverterByteArray.read(buf),
FfiConverterOptionalSequenceString.read(buf),
)
}
override fun allocationSize(value: WelcomeBundle) = (
FfiConverterByteArray.allocationSize(value.`id`) +
FfiConverterOptionalSequenceString.allocationSize(value.`crlNewDistributionPoints`)
)
override fun write(value: WelcomeBundle, buf: ByteBuffer) {
FfiConverterByteArray.write(value.`id`, buf)
FfiConverterOptionalSequenceString.write(value.`crlNewDistributionPoints`, buf)
}
}
/**
* See [core_crypto::prelude::WireIdentity]
*/
data class WireIdentity (
var `clientId`: kotlin.String,
var `status`: DeviceStatus,
var `thumbprint`: kotlin.String,
var `credentialType`: MlsCredentialType,
var `x509Identity`: X509Identity?
) {
companion object
}
public object FfiConverterTypeWireIdentity: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): WireIdentity {
return WireIdentity(
FfiConverterString.read(buf),
FfiConverterTypeDeviceStatus.read(buf),
FfiConverterString.read(buf),
FfiConverterTypeMlsCredentialType.read(buf),
FfiConverterOptionalTypeX509Identity.read(buf),
)
}
override fun allocationSize(value: WireIdentity) = (
FfiConverterString.allocationSize(value.`clientId`) +
FfiConverterTypeDeviceStatus.allocationSize(value.`status`) +
FfiConverterString.allocationSize(value.`thumbprint`) +
FfiConverterTypeMlsCredentialType.allocationSize(value.`credentialType`) +
FfiConverterOptionalTypeX509Identity.allocationSize(value.`x509Identity`)
)
override fun write(value: WireIdentity, buf: ByteBuffer) {
FfiConverterString.write(value.`clientId`, buf)
FfiConverterTypeDeviceStatus.write(value.`status`, buf)
FfiConverterString.write(value.`thumbprint`, buf)
FfiConverterTypeMlsCredentialType.write(value.`credentialType`, buf)
FfiConverterOptionalTypeX509Identity.write(value.`x509Identity`, buf)
}
}
/**
* See [core_crypto::prelude::X509Identity]
*/
data class X509Identity (
var `handle`: kotlin.String,
var `displayName`: kotlin.String,
var `domain`: kotlin.String,
var `certificate`: kotlin.String,
var `serialNumber`: kotlin.String,
var `notBefore`: kotlin.ULong,
var `notAfter`: kotlin.ULong
) {
companion object
}
public object FfiConverterTypeX509Identity: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): X509Identity {
return X509Identity(
FfiConverterString.read(buf),
FfiConverterString.read(buf),
FfiConverterString.read(buf),
FfiConverterString.read(buf),
FfiConverterString.read(buf),
FfiConverterULong.read(buf),
FfiConverterULong.read(buf),
)
}
override fun allocationSize(value: X509Identity) = (
FfiConverterString.allocationSize(value.`handle`) +
FfiConverterString.allocationSize(value.`displayName`) +
FfiConverterString.allocationSize(value.`domain`) +
FfiConverterString.allocationSize(value.`certificate`) +
FfiConverterString.allocationSize(value.`serialNumber`) +
FfiConverterULong.allocationSize(value.`notBefore`) +
FfiConverterULong.allocationSize(value.`notAfter`)
)
override fun write(value: X509Identity, buf: ByteBuffer) {
FfiConverterString.write(value.`handle`, buf)
FfiConverterString.write(value.`displayName`, buf)
FfiConverterString.write(value.`domain`, buf)
FfiConverterString.write(value.`certificate`, buf)
FfiConverterString.write(value.`serialNumber`, buf)
FfiConverterULong.write(value.`notBefore`, buf)
FfiConverterULong.write(value.`notAfter`, buf)
}
}
enum class CiphersuiteName(val value: kotlin.UShort) {
/**
* DH KEM x25519 | AES-GCM 128 | SHA2-256 | Ed25519
*/
MLS_128_DHKEMX25519_AES128GCM_SHA256_ED25519(1u),
/**
* DH KEM P256 | AES-GCM 128 | SHA2-256 | EcDSA P256
*/
MLS_128_DHKEMP256_AES128GCM_SHA256_P256(2u),
/**
* DH KEM x25519 | Chacha20Poly1305 | SHA2-256 | Ed25519
*/
MLS_128_DHKEMX25519_CHACHA20POLY1305_SHA256_ED25519(3u),
/**
* DH KEM x448 | AES-GCM 256 | SHA2-512 | Ed448
*/
MLS_256_DHKEMX448_AES256GCM_SHA512_ED448(4u),
/**
* DH KEM P521 | AES-GCM 256 | SHA2-512 | EcDSA P521
*/
MLS_256_DHKEMP521_AES256GCM_SHA512_P521(5u),
/**
* DH KEM x448 | Chacha20Poly1305 | SHA2-512 | Ed448
*/
MLS_256_DHKEMX448_CHACHA20POLY1305_SHA512_ED448(6u),
/**
* DH KEM P384 | AES-GCM 256 | SHA2-384 | EcDSA P384
*/
MLS_256_DHKEMP384_AES256GCM_SHA384_P384(7u);
companion object
}
public object FfiConverterTypeCiphersuiteName: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
CiphersuiteName.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: CiphersuiteName) = 4UL
override fun write(value: CiphersuiteName, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
sealed class CoreCryptoException: kotlin.Exception() {
class CryptoException(
val `error`: CryptoError
) : CoreCryptoException() {
override val message
get() = "error=${ `error` }"
}
class E2eIdentityException(
val `error`: E2eIdentityError
) : CoreCryptoException() {
override val message
get() = "error=${ `error` }"
}
class ClientException(
val v1: kotlin.String
) : CoreCryptoException() {
override val message
get() = "v1=${ v1 }"
}
companion object ErrorHandler : UniffiRustCallStatusErrorHandler {
override fun lift(error_buf: RustBuffer.ByValue): CoreCryptoException = FfiConverterTypeCoreCryptoError.lift(error_buf)
}
}
public object FfiConverterTypeCoreCryptoError : FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): CoreCryptoException {
return when(buf.getInt()) {
1 -> CoreCryptoException.CryptoException(
FfiConverterTypeCryptoError.read(buf),
)
2 -> CoreCryptoException.E2eIdentityException(
FfiConverterTypeE2eIdentityError.read(buf),
)
3 -> CoreCryptoException.ClientException(
FfiConverterString.read(buf),
)
else -> throw RuntimeException("invalid error enum value, something is very wrong!!")
}
}
override fun allocationSize(value: CoreCryptoException): ULong {
return when(value) {
is CoreCryptoException.CryptoException -> (
// Add the size for the Int that specifies the variant plus the size needed for all fields
4UL
+ FfiConverterTypeCryptoError.allocationSize(value.`error`)
)
is CoreCryptoException.E2eIdentityException -> (
// Add the size for the Int that specifies the variant plus the size needed for all fields
4UL
+ FfiConverterTypeE2eIdentityError.allocationSize(value.`error`)
)
is CoreCryptoException.ClientException -> (
// Add the size for the Int that specifies the variant plus the size needed for all fields
4UL
+ FfiConverterString.allocationSize(value.v1)
)
}
}
override fun write(value: CoreCryptoException, buf: ByteBuffer) {
when(value) {
is CoreCryptoException.CryptoException -> {
buf.putInt(1)
FfiConverterTypeCryptoError.write(value.`error`, buf)
Unit
}
is CoreCryptoException.E2eIdentityException -> {
buf.putInt(2)
FfiConverterTypeE2eIdentityError.write(value.`error`, buf)
Unit
}
is CoreCryptoException.ClientException -> {
buf.putInt(3)
FfiConverterString.write(value.v1, buf)
Unit
}
}.let { /* this makes the `when` an expression, which ensures it is exhaustive */ }
}
}
/**
* Defines the log level for a CoreCrypto
*/
enum class CoreCryptoLogLevel {
OFF,
TRACE,
DEBUG,
INFO,
WARN,
ERROR;
companion object
}
public object FfiConverterTypeCoreCryptoLogLevel: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
CoreCryptoLogLevel.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: CoreCryptoLogLevel) = 4UL
override fun write(value: CoreCryptoLogLevel, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
enum class DeviceStatus(val value: kotlin.UByte) {
/**
* All is fine
*/
VALID(1u),
/**
* The Credential's certificate is expired
*/
EXPIRED(2u),
/**
* The Credential's certificate is revoked (not implemented yet)
*/
REVOKED(3u);
companion object
}
public object FfiConverterTypeDeviceStatus: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
DeviceStatus.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: DeviceStatus) = 4UL
override fun write(value: DeviceStatus, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
enum class E2eiConversationState(val value: kotlin.UByte) {
/**
* All clients have a valid E2EI certificate
*/
VERIFIED(1u),
/**
* Some clients are either still Basic or their certificate is expired
*/
NOT_VERIFIED(2u),
/**
* All clients are still Basic. If all client have expired certificates, [E2eiConversationState::NotVerified] is returned.
*/
NOT_ENABLED(3u);
companion object
}
public object FfiConverterTypeE2eiConversationState: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
E2eiConversationState.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: E2eiConversationState) = 4UL
override fun write(value: E2eiConversationState, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
enum class MlsCredentialType(val value: kotlin.UByte) {
/**
* Basic credential i.e. a KeyPair
*/
BASIC(1u),
/**
* A x509 certificate generally obtained through e2e identity enrollment process
*/
X509(2u);
companion object
}
public object FfiConverterTypeMlsCredentialType: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
MlsCredentialType.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: MlsCredentialType) = 4UL
override fun write(value: MlsCredentialType, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
enum class MlsGroupInfoEncryptionType(val value: kotlin.UByte) {
/**
* Unencrypted `GroupInfo`
*/
PLAINTEXT(1u),
/**
* `GroupInfo` encrypted in a JWE
*/
JWE_ENCRYPTED(2u);
companion object
}
public object FfiConverterTypeMlsGroupInfoEncryptionType: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
MlsGroupInfoEncryptionType.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: MlsGroupInfoEncryptionType) = 4UL
override fun write(value: MlsGroupInfoEncryptionType, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
enum class MlsRatchetTreeType(val value: kotlin.UByte) {
/**
* Plain old and complete `GroupInfo`
*/
FULL(1u),
/**
* Contains `GroupInfo` changes since previous epoch (not yet implemented)
* (see [draft](https://github.com/rohan-wire/ietf-drafts/blob/main/mahy-mls-ratchet-tree-delta/draft-mahy-mls-ratchet-tree-delta.md))
*/
DELTA(2u),
BY_REF(3u);
companion object
}
public object FfiConverterTypeMlsRatchetTreeType: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
MlsRatchetTreeType.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: MlsRatchetTreeType) = 4UL
override fun write(value: MlsRatchetTreeType, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
enum class MlsWirePolicy(val value: kotlin.UByte) {
/**
* Handshake messages are never encrypted
*/
PLAINTEXT(1u),
/**
* Handshake messages are always encrypted
*/
CIPHERTEXT(2u);
companion object
}
public object FfiConverterTypeMlsWirePolicy: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer) = try {
MlsWirePolicy.values()[buf.getInt() - 1]
} catch (e: IndexOutOfBoundsException) {
throw RuntimeException("invalid enum value, something is very wrong!!", e)
}
override fun allocationSize(value: MlsWirePolicy) = 4UL
override fun write(value: MlsWirePolicy, buf: ByteBuffer) {
buf.putInt(value.ordinal + 1)
}
}
public object FfiConverterOptionalUInt: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): kotlin.UInt? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterUInt.read(buf)
}
override fun allocationSize(value: kotlin.UInt?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterUInt.allocationSize(value)
}
}
override fun write(value: kotlin.UInt?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterUInt.write(value, buf)
}
}
}
public object FfiConverterOptionalULong: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): kotlin.ULong? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterULong.read(buf)
}
override fun allocationSize(value: kotlin.ULong?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterULong.allocationSize(value)
}
}
override fun write(value: kotlin.ULong?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterULong.write(value, buf)
}
}
}
public object FfiConverterOptionalString: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): kotlin.String? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterString.read(buf)
}
override fun allocationSize(value: kotlin.String?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterString.allocationSize(value)
}
}
override fun write(value: kotlin.String?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterString.write(value, buf)
}
}
}
public object FfiConverterOptionalByteArray: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): kotlin.ByteArray? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterByteArray.read(buf)
}
override fun allocationSize(value: kotlin.ByteArray?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterByteArray.allocationSize(value)
}
}
override fun write(value: kotlin.ByteArray?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterByteArray.write(value, buf)
}
}
}
public object FfiConverterOptionalDuration: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): java.time.Duration? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterDuration.read(buf)
}
override fun allocationSize(value: java.time.Duration?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterDuration.allocationSize(value)
}
}
override fun write(value: java.time.Duration?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterDuration.write(value, buf)
}
}
}
public object FfiConverterOptionalTypeCommitBundle: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): CommitBundle? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterTypeCommitBundle.read(buf)
}
override fun allocationSize(value: CommitBundle?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterTypeCommitBundle.allocationSize(value)
}
}
override fun write(value: CommitBundle?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterTypeCommitBundle.write(value, buf)
}
}
}
public object FfiConverterOptionalTypeE2eiDumpedPkiEnv: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): E2eiDumpedPkiEnv? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterTypeE2eiDumpedPkiEnv.read(buf)
}
override fun allocationSize(value: E2eiDumpedPkiEnv?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterTypeE2eiDumpedPkiEnv.allocationSize(value)
}
}
override fun write(value: E2eiDumpedPkiEnv?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterTypeE2eiDumpedPkiEnv.write(value, buf)
}
}
}
public object FfiConverterOptionalTypeX509Identity: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): X509Identity? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterTypeX509Identity.read(buf)
}
override fun allocationSize(value: X509Identity?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterTypeX509Identity.allocationSize(value)
}
}
override fun write(value: X509Identity?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterTypeX509Identity.write(value, buf)
}
}
}
public object FfiConverterOptionalTypeMlsWirePolicy: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): MlsWirePolicy? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterTypeMlsWirePolicy.read(buf)
}
override fun allocationSize(value: MlsWirePolicy?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterTypeMlsWirePolicy.allocationSize(value)
}
}
override fun write(value: MlsWirePolicy?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterTypeMlsWirePolicy.write(value, buf)
}
}
}
public object FfiConverterOptionalSequenceString: FfiConverterRustBuffer?> {
override fun read(buf: ByteBuffer): List? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterSequenceString.read(buf)
}
override fun allocationSize(value: List?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterSequenceString.allocationSize(value)
}
}
override fun write(value: List?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterSequenceString.write(value, buf)
}
}
}
public object FfiConverterOptionalSequenceTypeBufferedDecryptedMessage: FfiConverterRustBuffer?> {
override fun read(buf: ByteBuffer): List? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterSequenceTypeBufferedDecryptedMessage.read(buf)
}
override fun allocationSize(value: List?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterSequenceTypeBufferedDecryptedMessage.allocationSize(value)
}
}
override fun write(value: List?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterSequenceTypeBufferedDecryptedMessage.write(value, buf)
}
}
}
public object FfiConverterOptionalSequenceTypeClientId: FfiConverterRustBuffer?> {
override fun read(buf: ByteBuffer): List? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterSequenceTypeClientId.read(buf)
}
override fun allocationSize(value: List?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterSequenceTypeClientId.allocationSize(value)
}
}
override fun write(value: List?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterSequenceTypeClientId.write(value, buf)
}
}
}
public object FfiConverterOptionalTypeCiphersuites: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): Ciphersuites? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterTypeCiphersuites.read(buf)
}
override fun allocationSize(value: Ciphersuites?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterTypeCiphersuites.allocationSize(value)
}
}
override fun write(value: Ciphersuites?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterTypeCiphersuites.write(value, buf)
}
}
}
public object FfiConverterOptionalTypeClientId: FfiConverterRustBuffer {
override fun read(buf: ByteBuffer): ClientId? {
if (buf.get().toInt() == 0) {
return null
}
return FfiConverterTypeClientId.read(buf)
}
override fun allocationSize(value: ClientId?): ULong {
if (value == null) {
return 1UL
} else {
return 1UL + FfiConverterTypeClientId.allocationSize(value)
}
}
override fun write(value: ClientId?, buf: ByteBuffer) {
if (value == null) {
buf.put(0)
} else {
buf.put(1)
FfiConverterTypeClientId.write(value, buf)
}
}
}
public object FfiConverterSequenceUShort: FfiConverterRustBuffer> {
override fun read(buf: ByteBuffer): List {
val len = buf.getInt()
return List(len) {
FfiConverterUShort.read(buf)
}
}
override fun allocationSize(value: List): ULong {
val sizeForLength = 4UL
val sizeForItems = value.map { FfiConverterUShort.allocationSize(it) }.sum()
return sizeForLength + sizeForItems
}
override fun write(value: List, buf: ByteBuffer) {
buf.putInt(value.size)
value.iterator().forEach {
FfiConverterUShort.write(it, buf)
}
}
}
public object FfiConverterSequenceString: FfiConverterRustBuffer> {
override fun read(buf: ByteBuffer): List {
val len = buf.getInt()
return List(len) {
FfiConverterString.read(buf)
}
}
override fun allocationSize(value: List): ULong {
val sizeForLength = 4UL
val sizeForItems = value.map { FfiConverterString.allocationSize(it) }.sum()
return sizeForLength + sizeForItems
}
override fun write(value: List, buf: ByteBuffer) {
buf.putInt(value.size)
value.iterator().forEach {
FfiConverterString.write(it, buf)
}
}
}
public object FfiConverterSequenceByteArray: FfiConverterRustBuffer> {
override fun read(buf: ByteBuffer): List {
val len = buf.getInt()
return List(len) {
FfiConverterByteArray.read(buf)
}
}
override fun allocationSize(value: List): ULong {
val sizeForLength = 4UL
val sizeForItems = value.map { FfiConverterByteArray.allocationSize(it) }.sum()
return sizeForLength + sizeForItems
}
override fun write(value: List, buf: ByteBuffer) {
buf.putInt(value.size)
value.iterator().forEach {
FfiConverterByteArray.write(it, buf)
}
}
}
public object FfiConverterSequenceTypeBufferedDecryptedMessage: FfiConverterRustBuffer> {
override fun read(buf: ByteBuffer): List {
val len = buf.getInt()
return List(len) {
FfiConverterTypeBufferedDecryptedMessage.read(buf)
}
}
override fun allocationSize(value: List): ULong {
val sizeForLength = 4UL
val sizeForItems = value.map { FfiConverterTypeBufferedDecryptedMessage.allocationSize(it) }.sum()
return sizeForLength + sizeForItems
}
override fun write(value: List, buf: ByteBuffer) {
buf.putInt(value.size)
value.iterator().forEach {
FfiConverterTypeBufferedDecryptedMessage.write(it, buf)
}
}
}
public object FfiConverterSequenceTypeProposalBundle: FfiConverterRustBuffer> {
override fun read(buf: ByteBuffer): List {
val len = buf.getInt()
return List(len) {
FfiConverterTypeProposalBundle.read(buf)
}
}
override fun allocationSize(value: List): ULong {
val sizeForLength = 4UL
val sizeForItems = value.map { FfiConverterTypeProposalBundle.allocationSize(it) }.sum()
return sizeForLength + sizeForItems
}
override fun write(value: List, buf: ByteBuffer) {
buf.putInt(value.size)
value.iterator().forEach {
FfiConverterTypeProposalBundle.write(it, buf)
}
}
}
public object FfiConverterSequenceTypeWireIdentity: FfiConverterRustBuffer> {
override fun read(buf: ByteBuffer): List {
val len = buf.getInt()
return List(len) {
FfiConverterTypeWireIdentity.read(buf)
}
}
override fun allocationSize(value: List): ULong {
val sizeForLength = 4UL
val sizeForItems = value.map { FfiConverterTypeWireIdentity.allocationSize(it) }.sum()
return sizeForLength + sizeForItems
}
override fun write(value: List, buf: ByteBuffer) {
buf.putInt(value.size)
value.iterator().forEach {
FfiConverterTypeWireIdentity.write(it, buf)
}
}
}
public object FfiConverterSequenceTypeClientId: FfiConverterRustBuffer> {
override fun read(buf: ByteBuffer): List {
val len = buf.getInt()
return List(len) {
FfiConverterTypeClientId.read(buf)
}
}
override fun allocationSize(value: List): ULong {
val sizeForLength = 4UL
val sizeForItems = value.map { FfiConverterTypeClientId.allocationSize(it) }.sum()
return sizeForLength + sizeForItems
}
override fun write(value: List, buf: ByteBuffer) {
buf.putInt(value.size)
value.iterator().forEach {
FfiConverterTypeClientId.write(it, buf)
}
}
}
public object FfiConverterMapStringByteArray: FfiConverterRustBuffer