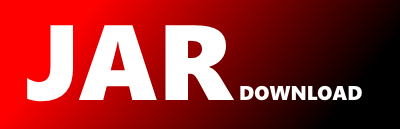
com.wiris.plugin.LibWIRIS Maven / Gradle / Ivy
package com.wiris.plugin;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.UnsupportedEncodingException;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import java.net.URLEncoder;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Iterator;
import java.util.Properties;
import javax.servlet.http.HttpServletRequest;
public abstract class LibWIRIS {
public static final String CONFIG_FILE = "/WEB-INF/pluginwiris/configuration.ini";
public static final String DEFAULT_STORAGE_CLASS = "com.wiris.plugin.storage.FileStorageAndCache";
public static Properties imageConfigProperties;
public static String[] xmlFileAttributes =
{ "bgColor", "symbolColor", "transparency", "fontSize", "numberColor", "identColor", "identMathvariant",
"numberMathvariant", "fontIdent", "fontNumber" };
static {
imageConfigProperties = new Properties();
imageConfigProperties.setProperty("bgColor", "wirisimagebgcolor");
imageConfigProperties.setProperty("symbolColor", "wirisimagesymbolcolor");
imageConfigProperties.setProperty("transparency", "wiristransparency");
imageConfigProperties.setProperty("fontSize", "wirisimagefontsize");
imageConfigProperties.setProperty("numberColor", "wirisimagenumbercolor");
imageConfigProperties.setProperty("identColor", "wirisimageidentcolor");
imageConfigProperties.setProperty("identMathvariant", "wirisimageidentmathvariant");
imageConfigProperties.setProperty("numberMathvariant", "wirisimagenumbermathvariant");
imageConfigProperties.setProperty("fontIdent", "wirisimagefontident");
imageConfigProperties.setProperty("fontNumber", "wirisimagefontnumber");
imageConfigProperties.setProperty("version", "wirisimageserviceversion");
}
public static String createIni(Properties properties) {
ByteArrayOutputStream out = new ByteArrayOutputStream();
try {
properties.store(out, "");
return out.toString("UTF-8");
} catch (IOException e) {
return "";
}
}
public static void flow(InputStream input, OutputStream output) throws IOException {
byte[] buffer = new byte[4 * 1024];
int bytesRead = input.read(buffer);
while (bytesRead != -1) {
output.write(buffer, 0, bytesRead);
bytesRead = input.read(buffer);
}
input.close();
output.flush();
}
public static String[] getAvailableCASLanguages(String languageString) {
String[] availableLanguages = languageString.split(",");
for (int i = availableLanguages.length - 1; i >= 0; --i) {
availableLanguages[i] = availableLanguages[i].trim();
}
// At least we should accept an empty language.
if (availableLanguages.length == 0) {
availableLanguages = new String[1];
availableLanguages[0] = "";
}
return availableLanguages;
}
public static InputStream getContents(URL url, Properties postVariables, String referer) throws Exception {
String postdata = LibWIRIS.httpBuildQuery(postVariables);
URLConnection connection = url.openConnection();
connection.addRequestProperty("Referer", referer);
if (postVariables != null) {
connection.setDoOutput(true);
OutputStreamWriter httpOutput = new OutputStreamWriter(connection.getOutputStream());
httpOutput.write(postdata);
httpOutput.flush();
httpOutput.close();
}
return connection.getInputStream();
}
public static URL getImageServiceURL(Properties config, String service) throws MalformedURLException {
// Protocol.
String protocol = config.getProperty("wirisimageserviceprotocol");
if (protocol == null) {
protocol = "http";
}
// Domain.
String domain = config.getProperty("wirisimageservicehost");
// Port.
String port = config.getProperty("wirisimageserviceport");
if (port == null) {
port = "";
} else {
port = ":" + port;
}
// Path.
String path = config.getProperty("wirisimageservicepath");
if (service != null) {
int end = path.lastIndexOf("/");
if (end == -1) {
path = service;
} else {
path = path.substring(0, end) + "/" + service;
}
}
return new URL(protocol + "://" + domain + port + path);
}
public static String getReferer(HttpServletRequest request) {
String scheme = request.getScheme();
String host = request.getServerName();
int port = request.getServerPort();
String uri = request.getRequestURI();
return scheme + "://" + host + ":" + port + uri;
}
public static String htmlentities(String input, boolean entQuotes) {
String returnValue = input.replace("&", "&").replace("<", "<").replace(">", "gt;");
if (entQuotes) {
return returnValue.replace("\"", """);
}
return returnValue;
}
public static String httpBuildQuery(Properties properties) throws UnsupportedEncodingException {
String returnValue = "";
Iterator keys = properties.keySet().iterator();
while (keys.hasNext()) {
String key = (String)keys.next();
String value = properties.getProperty(key);
returnValue += URLEncoder.encode(key, "UTF-8") + "=" + URLEncoder.encode(value, "UTF-8") + "&";
}
return returnValue;
}
public static boolean inArray(String needle, String[] stack) {
for (int i = stack.length - 1; i >= 0; --i) {
if (stack[i] != null && stack[i].equals(needle)) {
return true;
}
}
return false;
}
public static String md5(byte[] input) throws NoSuchAlgorithmException, UnsupportedEncodingException {
MessageDigest algorithm = MessageDigest.getInstance("MD5");
algorithm.reset();
algorithm.update(input);
byte[] md5 = algorithm.digest();
String toReturn = "";
for (int i = 0; i < md5.length; ++i) {
String tmp = (Integer.toHexString(0xFF & md5[i]));
if (tmp.length() == 1) {
toReturn += "0" + tmp;
} else {
toReturn += tmp;
}
}
return toReturn;
}
public static String replaceVariable(String value, String variableName, String variableValue) {
return value.replaceAll("%" + variableName, variableValue);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy