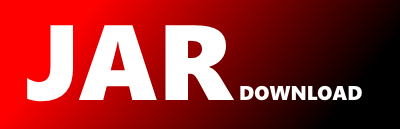
com.wiris.plugin.Main Maven / Gradle / Ivy
package com.wiris.plugin;
import com.wiris.plugin.configuration.ConfigurationUpdater;
import com.wiris.plugin.configuration.ConfigurationUpdater2;
import com.wiris.plugin.dispatchers.CASDispatcher;
import com.wiris.plugin.dispatchers.CreateCASImageDispatcher;
import com.wiris.plugin.dispatchers.CreateImageDispatcher;
import com.wiris.plugin.dispatchers.EditorDispatcher;
import com.wiris.plugin.dispatchers.GetMathMLDispatcher;
import com.wiris.plugin.dispatchers.ServiceDispatcher;
import com.wiris.plugin.dispatchers.ShowCASImageDispatcher;
import com.wiris.plugin.dispatchers.ShowImageDispatcher;
import com.wiris.plugin.dispatchers.TestDispatcher;
import com.wiris.plugin.storage.StorageAndCache;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class Main extends HttpServlet {
private Properties config;
private long configurationRefreshTime = 10000;
private long lastTimeConfigurationWasLoaded = 0;
public void init(ServletConfig servletConfig) throws ServletException {
super.init(servletConfig);
this.refreshConfiguration(true);
}
private Properties getConfigurationFromFile() {
InputStream configurationStream;
String configurationPath = this.getInitParameter("com.wiris.editor.configurationPath");
if (configurationPath != null) {
try {
configurationStream = new FileInputStream(configurationPath);
} catch (FileNotFoundException e) {
throw new Error(e);
}
} else {
configurationStream = this.getServletContext().getResourceAsStream(LibWIRIS.CONFIG_FILE);
}
try {
Properties configurationFromFile = new Properties();
configurationFromFile.load(configurationStream);
return configurationFromFile;
} catch (IOException e) {
throw new Error(e);
}
}
public ConfigurationUpdater getConfigurationUpdaterInstance() {
String className = this.config.getProperty("wirisconfigurationclass");
if (className == null || className.trim().length() == 0) {
return null;
}
try {
ConfigurationUpdater instance =
(ConfigurationUpdater)this.getClass().getClassLoader().loadClass(className).newInstance();
if (instance instanceof ConfigurationUpdater2) {
((ConfigurationUpdater2)instance).init(getServletContext());
} else {
instance.init();
}
return instance;
} catch (Exception e) {
throw new Error(e);
}
}
public StorageAndCache getStorageAndCacheInstance(HttpServletRequest request) {
String className = this.config.getProperty("wirisstorageclass");
if (className == null || className.trim().length() == 0) {
className = LibWIRIS.DEFAULT_STORAGE_CLASS;
}
try {
StorageAndCache instance = (StorageAndCache)this.getClass().getClassLoader().loadClass(className).newInstance();
instance.init(request, this.config);
return instance;
} catch (Exception e) {
throw new Error(e);
}
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.refreshConfiguration(false);
String pathInfo = request.getPathInfo();
if (pathInfo.equals("/cas")) {
CASDispatcher.dispatch(this.config, request, response);
} else if (pathInfo.equals("/editor")) {
EditorDispatcher.dispatch(this.config, request, response);
} else if (pathInfo.equals("/showcasimage")) {
ShowCASImageDispatcher.dispatch(this.getServletContext(), request, response,
this.getStorageAndCacheInstance(request));
} else if (pathInfo.equals("/showimage")) {
ShowImageDispatcher.dispatch(this.config, request, response, this.getStorageAndCacheInstance(request));
} else if (pathInfo.equals("/test")) {
TestDispatcher.dispatch(this.config, response, this.getStorageAndCacheInstance(request));
} else if (pathInfo.equals("/refreshconfiguration")) {
this.refreshConfiguration(true);
ServletOutputStream out = response.getOutputStream();
out.print("Configuration refreshed");
out.close();
}
}
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.refreshConfiguration(false);
String pathInfo = request.getPathInfo();
if (pathInfo.equals("/createcasimage")) {
CreateCASImageDispatcher.dispatch(request, response, this.getStorageAndCacheInstance(request));
} else if (pathInfo.equals("/createimage")) {
CreateImageDispatcher.dispatch(request, response, this.getStorageAndCacheInstance(request));
} else if (pathInfo.equals("/getmathml")) {
GetMathMLDispatcher.dispatch(this.config, request, response, this.getStorageAndCacheInstance(request));
} else if (pathInfo.equals("/service")) {
ServiceDispatcher.dispatch(this.config, request, response);
}
}
private void refreshConfiguration(boolean now) {
long currentTime = System.currentTimeMillis();
if (now ||
(this.configurationRefreshTime != -1 && currentTime - this.lastTimeConfigurationWasLoaded > this.configurationRefreshTime)) {
this.config = this.getConfigurationFromFile();
ConfigurationUpdater updater = this.getConfigurationUpdaterInstance();
if (updater != null) {
updater.updateConfiguration(this.config);
}
String newConfigurationRefreshTime = this.config.getProperty("wirisconfigurationrefreshtime");
if (newConfigurationRefreshTime == null) {
this.configurationRefreshTime = 10000;
} else {
this.configurationRefreshTime = Long.decode(newConfigurationRefreshTime);
}
this.lastTimeConfigurationWasLoaded = currentTime;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy