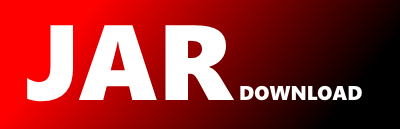
com.wiris.plugin.dispatchers.GetMathMLDispatcher Maven / Gradle / Ivy
package com.wiris.plugin.dispatchers;
import com.wiris.plugin.LibWIRIS;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.PrintWriter;
import java.io.StringReader;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.wiris.plugin.storage.StorageAndCache;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.InputStream;
import java.net.URL;
import java.util.Iterator;
import java.util.Properties;
public abstract class GetMathMLDispatcher {
public static void dispatch(Properties config, HttpServletRequest request, HttpServletResponse response,
StorageAndCache storage) throws IOException {
response.setContentType("text/plain; charset=utf-8");
String digest = null;
String md5Parameter = request.getParameter("md5");
if (md5Parameter != null && md5Parameter.length() == 32) { // Support for "generic simple" integration.
digest = md5Parameter;
} else {
String digestParameter = request.getParameter("digest");
if (digestParameter != null) { // Support for future integrations (where maybe they aren't using md5 sums).
digest = digestParameter;
}
}
PrintWriter out = response.getWriter();
if (digest != null) {
String content = storage.decodeDigest(digest);
if (content != null) {
if (content.startsWith("<")) {
BufferedReader fileBufferedReader = new BufferedReader(new StringReader(content));
String line;
if ((line = fileBufferedReader.readLine()) != null) {
out.print(line);
}
} else {
Properties formula = new Properties();
formula.load(new ByteArrayInputStream(content.getBytes("UTF-8")));
String mathml = formula.getProperty("mml");
if (mathml != null) {
out.print(mathml);
}
}
} else {
out.print("Error: formula not found.");
}
} else {
String latex = request.getParameter("latex");
if (latex != null) {
String urlString = config.getProperty("wirislatextomathmlurl");
URL url;
if (urlString != null) {
url = new URL(urlString);
} else {
url = LibWIRIS.getImageServiceURL(config, "latex2mathml");
}
Properties properties = new Properties();
properties.setProperty("latex", latex);
try {
InputStream inputStream = LibWIRIS.getContents(url, properties, LibWIRIS.getReferer(request));
ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
LibWIRIS.flow(inputStream, dataStream);
out.print(dataStream.toString("UTF-8"));
} catch (Exception e) {
out.print("Error: could not connect to the latex translator service.");
}
} else {
out.print("Error: no digest or latex has been sent.");
}
}
out.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy