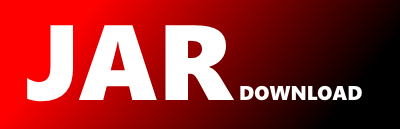
com.wiris.plugin.dispatchers.TestDispatcher Maven / Gradle / Ivy
package com.wiris.plugin.dispatchers;
import com.wiris.plugin.storage.StorageAndCache;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.Socket;
import java.util.Properties;
import javax.servlet.http.HttpServletResponse;
public class TestDispatcher {
public static void dispatch(Properties config, HttpServletResponse response, StorageAndCache storage) throws IOException {
response.setContentType("text/html; charset=utf-8");
PrintWriter out = response.getWriter();
out.print("Plugin WIRIS test page Plugin WIRIS test page
");
out.print("Connecting to WIRIS image server
");
// Connect test.
out.print("Connecting to " + config.getProperty("wirisimageservicehost") + " on port " +
config.getProperty("wirisimageserviceport") + "... ");
try {
Socket socket =
new Socket(config.getProperty("wirisimageservicehost"), Integer.parseInt(config.getProperty("wirisimageserviceport")));
TestDispatcher.wrs_assert(out, socket.isConnected());
socket.close();
} catch (IOException e) {
TestDispatcher.wrs_assert(out, false);
}
out.print("
Storing
");
// Generating digest.
out.print("Generating digest... ");
String digest = storage.codeDigest("test");
TestDispatcher.wrs_assert(out, true);
// Storing data.
out.print("Storing data... ");
storage.storeData(digest, "test".getBytes("UTF-8"));
TestDispatcher.wrs_assert(out, true);
out.print("
Retreiving
");
// Retreiving content.
out.print("Retreiving content... ");
storage.decodeDigest(digest);
TestDispatcher.wrs_assert(out, true);
// Retreiving data.
out.print("Retreiving data... ");
storage.retreiveData(digest);
TestDispatcher.wrs_assert(out, true);
out.print("
");
out.close();
}
private static void wrs_assert(PrintWriter out, boolean condition) {
if (condition) {
out.print("OK
");
} else {
out.print("ERROR
");
}
}
}