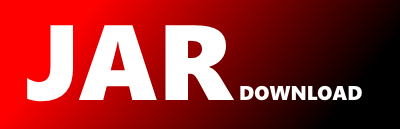
com.wiris.plugin.storage.CompressedStorageAndCache Maven / Gradle / Ivy
package com.wiris.plugin.storage;
import java.io.IOException;
import java.util.Properties;
import javax.servlet.http.HttpServletRequest;
import com.wiris.plugin.Base64;
import com.wiris.plugin.LibWIRIS;
public class CompressedStorageAndCache implements StorageAndCache {
private StorageAndCache cache = null;
public CompressedStorageAndCache() {
}
public String codeDigest(String content) {
try {
// Compress with GZIP using the standard JAVA library and encode in URL-safe Base64 (i.e., with '-' and '_' instead of '+' and '/')
return Base64.encodeBytes(content.getBytes("UTF-8"), Base64.GZIP | Base64.URL_SAFE);
} catch (IOException e) {
throw new Error(e);
}
}
public String decodeDigest(String digest) {
try {
// URL Base64-decode and GZIP decompress.
return new String(Base64.decode(digest, Base64.GZIP | Base64.URL_SAFE), "UTF-8");
} catch (IOException e) {
// Digest is corrupted.
throw new Error(e);
}
}
private String getFileName(String digest) {
try {
return LibWIRIS.md5(digest.getBytes("US-ASCII"));
} catch (Exception e) {
throw new Error(e);
}
}
public void init(HttpServletRequest request, Properties config) {
if (config.getProperty("wiriscachedirectory") != null) {
this.cache = new FileStorageAndCache();
this.cache.init(request, config);
}
}
public byte[] retreiveData(String digest) {
if (this.cache == null) {
return null;
}
String cacheDigest = this.getFileName(digest);
return this.cache.retreiveData(cacheDigest);
}
public void storeData(String digest, byte[] stream) {
if (this.cache != null) {
String cacheDigest = this.getFileName(digest);
this.cache.storeData(cacheDigest, stream);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy