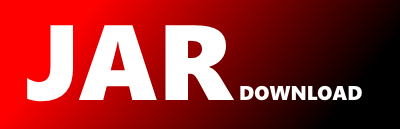
com.wiris.plugin.storage.FileStorageAndCache Maven / Gradle / Ivy
package com.wiris.plugin.storage;
import java.io.BufferedOutputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.util.Properties;
import javax.servlet.http.HttpServletRequest;
import com.wiris.plugin.LibWIRIS;
/**
* This class uses the md5 as a digest method and the "cache" folder to store the
* association of the digest with the formula source (MathML).
* The images of the formulas are also stored in the "formula" folder.
* Both the "cache" and "formula" folders are obtained from the WIRIS plugin
* configuration.
*/
public class FileStorageAndCache implements StorageAndCache {
String formulaDirectory;
String cacheDirectory;
public FileStorageAndCache() {
}
public String codeDigest(String content) {
try {
String digest = LibWIRIS.md5(content.getBytes("UTF-8"));
FileOutputStream fileOutputStream = new FileOutputStream(new File(this.formulaDirectory + "/" + digest + ".ini"));
fileOutputStream.write(content.getBytes("UTF-8"));
fileOutputStream.flush();
fileOutputStream.close();
return digest;
} catch (Exception e) {
throw new Error(e);
}
}
public String decodeDigest(String digest) {
digest = FileStorageAndCache.sanitizeDigest(digest);
String[] digestInformation = this.getDigestInformation(digest);
try {
File file = new File(this.formulaDirectory + "/" + digestInformation[0] + ".ini");
FileInputStream inputStream = new FileInputStream(file);
ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
LibWIRIS.flow(inputStream, dataStream);
String content = dataStream.toString();
if (digestInformation[1] != null) {
content += "\r\nformat=" + digestInformation[1];
}
return content;
} catch (FileNotFoundException e) {
return null;
} catch (Exception e) {
throw new Error(e);
}
}
public String[] getDigestInformation(String digest) {
String extension = null;
int dotPosition = digest.lastIndexOf(".");
if (dotPosition != -1) {
extension = digest.substring(dotPosition + 1, digest.length());
if (!extension.equals("png") && !extension.equals("swf")) {
extension = null;
} else {
digest = digest.substring(0, dotPosition);
}
}
return new String[] { digest, extension };
}
public void init(HttpServletRequest request, Properties config) {
this.cacheDirectory = config.getProperty("wiriscachedirectory");
if (this.cacheDirectory == null) {
throw new Error("wiriscachedirectory property is not defined in configuration.ini");
}
this.formulaDirectory = config.getProperty("wirisformuladirectory");
if (this.formulaDirectory == null) {
throw new Error("wirisformuladirectory property is not defined in configuration.ini");
}
}
public byte[] retreiveData(String digest) {
digest = FileStorageAndCache.sanitizeDigest(digest);
String[] digestInformation = this.getDigestInformation(digest);
if (digestInformation[1] == null) {
digestInformation[1] = "png";
}
try {
File file = new File(this.cacheDirectory + "/" + digestInformation[0] + "." + digestInformation[1]);
FileInputStream inputStream = new FileInputStream(file);
ByteArrayOutputStream dataStream = new ByteArrayOutputStream();
LibWIRIS.flow(inputStream, dataStream);
return dataStream.toByteArray();
} catch (FileNotFoundException e) {
return null;
} catch (Exception e) {
throw new Error(e);
}
}
private static String sanitizeDigest(String digest) {
return (new File(digest)).getName();
}
public void storeData(String digest, byte[] stream) {
digest = FileStorageAndCache.sanitizeDigest(digest);
String[] digestInformation = this.getDigestInformation(digest);
if (digestInformation[1] == null) {
digestInformation[1] = "png";
}
try {
BufferedOutputStream fileOutput =
new BufferedOutputStream(new FileOutputStream(new File(this.cacheDirectory + "/" + digestInformation[0] +
"." + digestInformation[1])));
fileOutput.write(stream);
fileOutput.flush();
fileOutput.close();
} catch (Exception e) {
throw new Error(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy