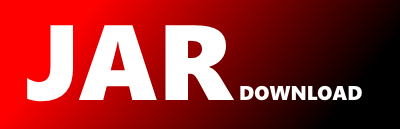
com.wizarius.orm.database.actions.builders.WizWhereQueryBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
package com.wizarius.orm.database.actions.builders;
import com.wizarius.orm.database.DBException;
import com.wizarius.orm.database.data.DBSignType;
import com.wizarius.orm.database.data.DBWhereType;
import com.wizarius.orm.database.data.WhereField;
import com.wizarius.orm.database.data.fieldfinder.FieldFinder;
import com.wizarius.orm.database.data.fieldfinder.FieldFinderResult;
import com.wizarius.orm.database.entityreader.DBSupportedTypes;
import com.wizarius.orm.database.entityreader.WizEntityManager;
import com.wizarius.orm.database.handlers.WritableHandler;
import com.wizarius.orm.database.handlers.WritableHandlers;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.concurrent.atomic.AtomicInteger;
/**
* @author Vladyslav Shyshkin
* Date: 2019-10-17
* Time: 23:23
*/
public class WizWhereQueryBuilder {
/**
* List of where queries
*/
private final Map> where = new LinkedHashMap<>();
/**
* Field finder
*/
private final FieldFinder fieldFinder;
/**
* DB where type
*/
private DBWhereType whereType = DBWhereType.AND;
/**
* Writable handlers
*/
private final WritableHandlers writableHandlers;
public WizWhereQueryBuilder(WritableHandlers writableHandlers, FieldFinder fieldsFinder) {
this.writableHandlers = writableHandlers;
this.fieldFinder = fieldsFinder;
}
/**
* Setup where filed
*
* @param field filed name
* @param object field value
*/
public void where(String field, Object object) {
where.put(field, new WhereField<>(object, DBSignType.EQUALS));
}
/**
* Setup where filed
*
* @param field filed name
* @param object field value
* @param signType sign type
*/
public void where(String field, Object object, DBSignType signType) {
where.put(field, new WhereField<>(object, signType));
}
/**
* Setup where filed
*
* @param key database field name
* @param value database field value
* @param clazz instance of object
* @throws DBException on unable to build where condition
*/
public void where(String key, Object value, Class> clazz) throws DBException {
where(key, value, DBSignType.EQUALS, clazz);
}
/**
* Build where clause
*
* @param key database field name
* @param value database field value
* @param signType where sign type
* @param clazz instance of object
* @throws DBException on unable to build where condition
*/
public void where(String key,
Object value,
DBSignType signType,
Class> clazz) throws DBException {
FieldFinderResult dbField;
if (clazz == null) {
dbField = fieldFinder.findDBField(key);
} else {
dbField = fieldFinder.findDBField(key, clazz);
}
where.put(dbField.getFindField().getDbFieldName(), new WhereField<>(value, signType));
}
/**
* Setup where query
*
* @param query custom query
*/
public void where(String query) {
where.put(query, new WhereField<>(query, DBSignType.NONE, true));
}
/**
* Set where type
*
* @param type new where type
*/
public void setWhereType(DBWhereType type) {
whereType = type;
}
/**
* Setup prepared delete query
*
* @param index index in prepared statement
* @param statement prepared query
* @throws DBException on unable to setup where values
*/
@SuppressWarnings({"rawtypes", "unchecked"})
public void setupWhereValues(AtomicInteger index, PreparedStatement statement) throws DBException {
for (WhereField> whereField : where.values()) {
if (whereField.isQuery()) {
continue;
}
Object value = whereField.getValue();
DBSupportedTypes supportedType = WizEntityManager.javaTypeToDBType(value.getClass());
WritableHandler handlerByType = writableHandlers.getHandlerByType(supportedType);
try {
handlerByType.set(value, index.getAndIncrement(), statement);
} catch (SQLException e) {
throw new DBException("Unable to builder where values " + e.getMessage(), e);
}
}
}
/**
* Setup prepared delete query
*
* @param statement prepared query
* @throws DBException on unable to setup where values
*/
public void setupWhereValues(PreparedStatement statement) throws DBException {
setupWhereValues(new AtomicInteger(1), statement);
}
/**
* Build where clause
*
* @return where prepared string
*/
public String buildWhereClause() {
StringBuilder sb = new StringBuilder();
if (!where.isEmpty()) {
sb.append(" WHERE ");
for (Map.Entry> entry : where.entrySet()) {
if (!entry.getValue().isQuery()) {
sb.append(entry.getKey())
.append(" ")
.append(entry.getValue().getSignType().getSign())
.append(entry.getValue().getSignType() == DBSignType.IN ? " (" : " ")
.append("?")
.append(entry.getValue().getSignType() == DBSignType.IN ? ") " : " ")
.append(whereType)
.append(" ");
} else {
sb.append(entry.getValue().getValue())
.append(" ")
.append(whereType)
.append(" ");
}
}
sb.setLength(sb.length() - (whereType.toString().length() + 1));
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy