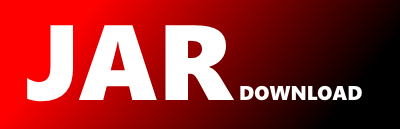
com.wizarius.orm.migrations.DBMigration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
package com.wizarius.orm.migrations;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.wizarius.orm.database.DBException;
import com.wizarius.orm.database.connection.DBConnection;
import com.wizarius.orm.database.connection.DBConnectionPool;
import lombok.extern.slf4j.Slf4j;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
* Created by Vladyslav Shyshkin on 24.11.2017.
*/
@SuppressWarnings("WeakerAccess")
@Slf4j
public abstract class DBMigration {
/**
* Migration level
*/
private final int level;
/**
* Migration name
*/
private final String name;
/**
* Connection pool
*/
private DBConnectionPool pool;
/**
* Commands to execute
*/
private final List commandsToExecute = new ArrayList<>();
public DBMigration(int level, String name) {
this.level = level;
this.name = name;
}
/**
* Execute migration
*
* @throws DBMigrationException on unable to execute
*/
final void execute() throws DBMigrationException {
if (isInTransaction()) {
executeInTransactionBlock();
} else {
executeQueries();
}
}
/**
* Start transaction before up
*
* @throws DBMigrationException on unable to start
*/
private void executeInTransactionBlock() throws DBMigrationException {
log.info("Execute migration command in transaction block where commands count = {}", commandsToExecute.size());
try (DBConnection connection = pool.getConnection()) {
connection.startTransaction();
for (String sql : commandsToExecute) {
log.trace("Execute migration sql = " + sql);
connection.executeQuery(sql);
}
connection.commitTransaction();
} catch (DBException e) {
// do not need to do rollback, it will happen in the close method
throw new DBMigrationException("Unable to get connection. " + e.getMessage(), e);
} catch (SQLException e) {
// do not need to do rollback, it will happen in the close method
throw new DBMigrationException("Unable to start or commit transaction. " + e.getMessage(), e);
}
}
/**
* Execute queries
*
* @throws DBMigrationException on unable to execute query
*/
private void executeQueries() throws DBMigrationException {
log.info("Execute migration command where commands count = {}", commandsToExecute.size());
try (DBConnection connection = pool.getConnection()) {
for (String sql : commandsToExecute) {
log.trace("Execute migration sql = " + sql);
connection.executeQuery(sql);
}
} catch (DBException e) {
throw new DBMigrationException("Unable to get connection. " + e.getMessage(), e);
} catch (SQLException e) {
throw new DBMigrationException("Unable to execute query. " + e.getMessage(), e);
}
}
/**
* On setup migration
*/
public abstract void up();
/**
* Execute sql
*
* @param sql sql string
*/
protected final void executeSQL(String sql) {
commandsToExecute.add(sql);
}
/**
* Returns true if need execute migration in transaction block
*
* @return execute in transaction block
*/
protected boolean isInTransaction() {
return true;
}
/**
* Execute sql
* Example
* InputStream in = getClass().getClassLoader().getResourceAsStream("migrations/initial.sql");
*
* @param stream input stream
* @throws IOException on unable to read from file
*/
protected final void executeSQL(InputStream stream) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(stream));
StringBuilder sb = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line);
}
String[] commands = sb.toString().split(";");
for (String command : commands) {
executeSQL(command);
}
}
/**
* Get migration level
*
* @return migration level
*/
public int getLevel() {
return level;
}
/**
* Get migration name
*
* @return migration name
*/
public String getName() {
return name;
}
/**
* Setup connection pool
*
* @param pool pool to setup
*/
public void setupConnectionPool(final DBConnectionPool pool) {
this.pool = pool;
}
/**
* Get connection pool to database
*
* @return connection pool
*/
@JsonIgnore
public DBConnectionPool getPool() {
return pool;
}
@Override
public String toString() {
return "DBMigration{" +
"level=" + level +
", name='" + name + '\'' +
", pool=" + pool +
", commandsToExecute=" + commandsToExecute +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy