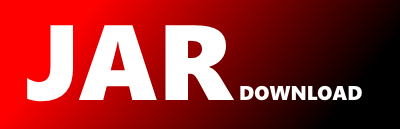
com.wizarius.orm.database.actions.IDBInsert Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
package com.wizarius.orm.database.actions;
import com.wizarius.orm.database.DBException;
import com.wizarius.orm.database.connection.DBConnection;
import java.util.List;
/**
* @author Vladyslav Shyshkin
* Date: 24.03.2020
* Time: 15:15
*/
public interface IDBInsert {
/**
* Execute insert query
*
* @param entity entity to insert
* @throws DBException on unable to insert
*/
void execute(Entity entity) throws DBException;
/**
* Execute insert query
* If connection is presented, it is assumed that the user himself wants to manage the connection
* The connection will not be automatically closed after the request
*
* @param entity entity to insert
* @param connection connection to database
* @throws DBException on unable to insert
*/
void execute(Entity entity, DBConnection connection) throws DBException;
/**
* Execute multiple insert query
*
* @param entities list of entities
* @throws DBException on unable to insert
*/
default void execute(List entities) throws DBException {
execute(entities, 500);
}
/**
* Execute multiple insert query
* If connection is presented, it is assumed that the user himself wants to manage the connection
* The connection will not be automatically closed after the request
*
* @param entities list of entities
* @param connection connection to database
* @throws DBException on unable to insert
*/
default void execute(List entities, DBConnection connection) throws DBException {
execute(entities, connection, 500);
}
/**
* Execute multiple insert query
* If connection is presented, it is assumed that the user himself wants to manage the connection
* The connection will not be automatically closed after the request
*
* @param entities list of entities
* @param connection connection to database
* @param truncateLength max items in one query
* @throws DBException on unable to insert
*/
void execute(List entities, DBConnection connection, int truncateLength) throws DBException;
/**
* Execute multiple insert query
*
* @param entities list of entities
* @param truncateLength max items in one query
* @throws DBException on unable to insert
*/
void execute(List entities, int truncateLength) throws DBException;
/**
* Returns sql query with all parameters and where conditions
*
* @param entity entity to delete
* @return query for execution
* @throws DBException unable to build query
*/
String toSQLQuery(Entity entity) throws DBException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy