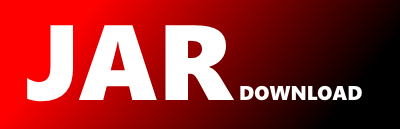
com.wizarius.orm.database.actions.builders.WizWhereQueryBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
package com.wizarius.orm.database.actions.builders;
import com.wizarius.orm.database.DBException;
import com.wizarius.orm.database.DBRuntimeException;
import com.wizarius.orm.database.data.*;
import com.wizarius.orm.database.data.fieldfinder.FieldFinder;
import com.wizarius.orm.database.data.fieldfinder.FieldFinderResult;
import com.wizarius.orm.database.entityreader.DBSupportedTypes;
import com.wizarius.orm.database.entityreader.WizEntityManager;
import com.wizarius.orm.database.handlers.WritableHandler;
import com.wizarius.orm.database.handlers.WritableHandlers;
import java.lang.reflect.Array;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
/**
* @author Vladyslav Shyshkin
* Date: 2019-10-17
* Time: 23:23
*/
public class WizWhereQueryBuilder {
/**
* List of where queries
*/
private final List where = new ArrayList<>();
/**
* Field finder
*/
private final FieldFinder fieldFinder;
/**
* DB where type
*/
private DBWhereType whereType = DBWhereType.AND;
/**
* Writable handlers
*/
private final WritableHandlers writableHandlers;
public WizWhereQueryBuilder(WritableHandlers writableHandlers, FieldFinder fieldsFinder) {
this.writableHandlers = writableHandlers;
this.fieldFinder = fieldsFinder;
}
/**
* Setup where filed
*
* @param field filed name
* @param value field value
*/
public void where(String field, Object value) {
if (value == null) {
throw new DBRuntimeException("Value can't be null");
}
where.add(new WhereBaseCondition<>(field, value, DBSignType.EQUALS));
}
/**
* Setup where filed
*
* @param field filed name
* @param value field value
* @param signType sign type
*/
public void where(String field, Object value, DBSignType signType) {
if (value == null) {
throw new DBRuntimeException("Value can't be null");
}
where.add(new WhereBaseCondition<>(field, value, signType));
}
/**
* Setup where filed
*
* @param key database field name
* @param value database field value
* @param clazz instance of object
* @throws DBException on unable to build where condition
*/
public void where(String key, Object value, Class> clazz) throws DBException {
if (value == null) {
throw new DBRuntimeException("Value can't be null");
}
where(key, value, DBSignType.EQUALS, clazz);
}
/**
* Build where clause
*
* @param key database field name
* @param value database field value
* @param signType where sign type
* @param clazz instance of object
* @throws DBException on unable to build where condition
*/
public void where(String key,
Object value,
DBSignType signType,
Class> clazz) throws DBException {
if (value == null) {
throw new DBRuntimeException("Value can't be null");
}
FieldFinderResult dbField;
if (clazz == null) {
dbField = fieldFinder.findDBField(key);
} else {
dbField = fieldFinder.findDBField(key, clazz);
}
where.add(new WhereBaseCondition<>(dbField.getFindField().getDbFieldName(), value, signType));
}
/**
* Setup where query
*
* @param query custom query
*/
public void where(String query) {
where.add(new WhereQueryCondition(query));
}
/**
* Setup where field in array
*
* @param field field name
* @param values array list
*/
public void whereIN(String field, Object values) {
if (values == null) {
throw new DBRuntimeException("Array must be present in where condition");
}
if (!values.getClass().isArray()) {
throw new DBRuntimeException("Class is not array");
}
Object[] array = buildArray(values);
if (array.length == 0) {
throw new DBRuntimeException("Array can't be empty for condition");
}
where.add(new WhereInCondition<>(field, array, DBSignType.IN));
}
/**
* Set where type
*
* @param type new where type
*/
public void setWhereType(DBWhereType type) {
whereType = type;
}
/**
* Setup prepared query where values
*
* @param index index in prepared statement
* @param statement prepared query
* @throws DBException on unable to setup where values
*/
public void setupWhereValues(AtomicInteger index, PreparedStatement statement) throws DBException {
for (WhereCondition condition : where) {
if (condition instanceof WhereBaseCondition>) {
WhereBaseCondition> item = (WhereBaseCondition>) condition;
Object value = item.getValue();
setupIndexValue(index, statement, value);
} else if (condition instanceof WhereInCondition) {
WhereInCondition> item = (WhereInCondition>) condition;
for (Object value : item.getValues()) {
setupIndexValue(index, statement, value);
}
}
}
}
/**
* Setup value for index
*
* @param index index
* @param statement statement
* @param value value
* @throws DBException on unable to setup value
*/
@SuppressWarnings({"rawtypes", "unchecked"})
private void setupIndexValue(AtomicInteger index, PreparedStatement statement, Object value) throws DBException {
DBSupportedTypes supportedType = WizEntityManager.javaTypeToDBType(value.getClass());
WritableHandler handlerByType = writableHandlers.getHandlerByType(supportedType);
try {
handlerByType.set(value, index.getAndIncrement(), statement);
} catch (SQLException e) {
throw new DBException("Unable to builder where values " + e.getMessage(), e);
}
}
/**
* Build where clause
*
* @return where prepared string
*/
public String buildWhereClause() {
StringBuilder sb = new StringBuilder();
if (!where.isEmpty()) {
sb.append(" WHERE ");
for (WhereCondition condition : where) {
if (condition instanceof WhereBaseCondition>) {
WhereBaseCondition> item = (WhereBaseCondition>) condition;
sb.append(item.getDbFieldName())
.append(" ")
.append(item.getSignType().getSign())
.append(item.getSignType() == DBSignType.IN ? " (" : " ")
.append("?")
.append(item.getSignType() == DBSignType.IN ? ") " : " ")
.append(whereType)
.append(" ");
} else if (condition instanceof WhereQueryCondition) {
WhereQueryCondition item = (WhereQueryCondition) condition;
sb.append(item.getQuery())
.append(" ")
.append(whereType)
.append(" ");
} else if (condition instanceof WhereInCondition) {
WhereInCondition> item = (WhereInCondition>) condition;
sb.append(item.getDbFieldName())
.append(" ")
.append(item.getSignType().getSign())
.append(" (")
.append(buildQuestionsCount(item.getValues().length))
.append(") ")
.append(whereType)
.append(" ");
}
}
sb.setLength(sb.length() - (whereType.toString().length() + 1));
}
return sb.toString();
}
/**
* Returns a string with the given number of question characters
*
* @param length questions count
* @return string like ?,?,?
*/
private String buildQuestionsCount(int length) {
if (length == 1) {
return "?";
}
StringBuilder sb = new StringBuilder();
for (int i = length; i > 0; i--) {
sb.append("?").append(",");
}
sb.setLength(sb.length() - 1);
return sb.toString();
}
/**
* Convert values array
*
* @param values value object
* @return object array for value
*/
private Object[] buildArray(Object values) {
if (values instanceof Object[]) {
return (Object[]) values;
}
int length = Array.getLength(values);
Object[] result = new Object[length];
for (int i = 0; i < length; ++i) {
result[i] = Array.get(values, i);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy