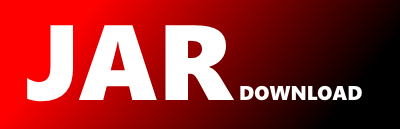
com.wizarius.orm.database.DatabaseStorage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
The newest version!
package com.wizarius.orm.database;
import com.wizarius.orm.database.connection.DBConnection;
import com.wizarius.orm.database.connection.DBConnectionPool;
import com.wizarius.orm.database.entityreader.EntityQuery;
import java.lang.reflect.ParameterizedType;
import java.util.List;
/**
* @author Vladyslav Shyshkin on 29.04.17.
*/
public class DatabaseStorage {
protected final DBConnectionPool pool;
private final Class clazz;
private final EntityQuery session;
public DatabaseStorage(DBConnectionPool pool, Class clazz) throws DBException {
this.pool = pool;
this.clazz = clazz;
this.session = pool.getEntityManager().getEntityQuery(clazz);
}
@SuppressWarnings("unchecked")
public DatabaseStorage(DBConnectionPool pool) throws DBException {
this.pool = pool;
this.clazz = (Class) ((ParameterizedType) getClass().getGenericSuperclass()).getActualTypeArguments()[0];
this.session = pool.getEntityManager().getEntityQuery(clazz);
}
/**
* Save or update
*
* @param entity entity
* @throws DBException on unable to save to database
*/
public void saveOrUpdate(Entity entity) throws DBException {
if (session.isNew(entity)) {
save(entity);
} else {
update(entity);
}
}
/**
* Save object if new entity, update otherwise
*
* @param entity entity
* @param connection connection
* @throws DBException on unable to execute query
*/
public void saveOrUpdate(Entity entity, DBConnection connection) throws DBException {
if (session.isNew(entity)) {
save(entity, connection);
} else {
update(entity, connection);
}
}
/**
* Save entity
*
* @param entity DBEntity
* @throws DBException on unable to save to database
*/
public void save(Entity entity) throws DBException {
session.getInsertQuery().execute(entity);
}
/**
* Save entity
*
* @param entity DBEntity
* @param connection database connection
* @throws DBException on unable to save to database
*/
public void save(Entity entity, DBConnection connection) throws DBException {
session.getInsertQuery().execute(entity, connection);
}
/**
* Save entities list
*
* @param entities entities
* @throws DBException on unable to save entity
*/
public void save(List entities) throws DBException {
session.getInsertQuery().execute(entities);
}
/**
* Save entities list
*
* @param entities entities
* @param connection database connection
* @throws DBException on unable to save entity
*/
public void save(List entities, DBConnection connection) throws DBException {
session.getInsertQuery().execute(entities, connection);
}
/**
* Get table name
*
* @return table name
*/
public String getTableName() {
return session.getFields().getTableName();
}
/**
* Update entity
*
* @param entity DBEntity
* @throws DBException on unable to update entity in database
*/
public void update(Entity entity) throws DBException {
session.getUpdateQuery().execute(entity);
}
/**
* Update entity
*
* @param entity entity
* @param connection database connection
* @throws DBException un unable to update entity in database
*/
public void update(Entity entity, DBConnection connection) throws DBException {
session.getUpdateQuery().execute(entity, connection);
}
/**
* Get entity by id
*
* @param id entity id
* @throws DBException on unable to get by id
*/
public Entity getByID(Object id) throws DBException {
return session.getSelectQuery().where(session.getIDDbFieldName(), id, clazz).getOne();
}
/**
* Delete entity
*
* @param entity entity to delete
* @throws DBException on unable to delete
*/
public void delete(Entity entity) throws DBException {
session.getDeleteQuery().execute(entity);
}
/**
* Delete entity
*
* @param entity entity to delete
* @param connection connection
* @throws DBException on unable to delete
*/
public void delete(Entity entity, DBConnection connection) throws DBException {
session.getDeleteQuery().execute(entity, connection);
}
/**
* Delete entity by id
*
* @param id entity id
* @throws DBException on unable to delete by id
*/
public void deleteByID(Object id) throws DBException {
session.getDeleteQuery().where(session.getIDDbFieldName(), id).execute();
}
/**
* Delete entity by id
*
* @param id entity id
* @param connection database connection
* @throws DBException on unable to delete by id
*/
public void deleteByID(Object id, DBConnection connection) throws DBException {
session.getDeleteQuery().where(session.getIDDbFieldName(), id).execute(connection);
}
/**
* Get all records in database
*
* @return array list of entities
* @throws DBException on unable to get all
*/
public List getAll() throws DBException {
return session.getSelectQuery().execute();
}
/**
* Delete all records from table
*
* @throws DBException on unable to clear
*/
public void clear() throws DBException {
session.getDeleteQuery().execute();
}
/**
* Get count elements in table
*
* @return count elements in table
* @throws DBException database exception
*/
public long count() throws DBException {
return session.getSelectQuery().getCount();
}
/**
* Returns entity session
*
* @return entity session
*/
public EntityQuery getSession() {
return session;
}
/**
* Returns connection pool
*
* @return database connection pool
*/
public DBConnectionPool getPool() {
return pool;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy