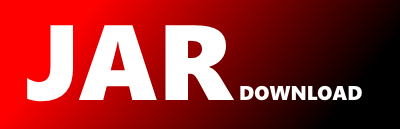
com.wizarius.orm.database.actions.IDBSelect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
The newest version!
package com.wizarius.orm.database.actions;
import com.wizarius.orm.database.DBException;
import com.wizarius.orm.database.connection.DBConnection;
import java.util.List;
/**
* @author Vladyslav Shyshkin
* Date: 25.03.2020
* Time: 19:27
*/
public interface IDBSelect {
/**
* Returns count of entities in table
*
* @return count entities in query
* @throws DBException on unable to execute select query
*/
long getCount() throws DBException;
/**
* Execute select query to get one item
*
* @return entity
* @throws DBException on unable to execute select query
*/
Entity getOne() throws DBException;
/**
* Execute select query
* If connection is presented, it is assumed that the user himself wants to manage the connection
* The connection will not be automatically closed after the request
*
* @param connection connection to database
* @return entity
* @throws DBException on unable to execute select query
*/
Entity getOne(DBConnection connection) throws DBException;
/**
* Execute select query
*
* @return list of entities
* @throws DBException on unable to execute select query
*/
List execute() throws DBException;
/**
* Execute custom select query and convert to entities list
*
* @param query query
* @return entities list
* @throws DBException on unable to execute custom query
*/
List execute(String query) throws DBException;
/**
* Execute custom select query and convert to entities list
*
* @param query query
* @param connection connection
* @return entities list
* @throws DBException on unable to execute custom query
*/
List execute(String query, DBConnection connection) throws DBException;
/**
* Execute select query
* If connection is presented, it is assumed that the user himself wants to manage the connection
* The connection will not be automatically closed after the request
*
* @param connection connection to database
* @return list of entities
* @throws DBException on unable to execute select query
*/
List execute(DBConnection connection) throws DBException;
/**
* Returns sql query with all parameters and where conditions
*
* @return query for execution
* @throws DBException unable to build query
* @throws DBException on unable to execute select query
*/
String toSQLQuery() throws DBException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy