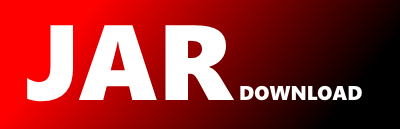
com.wizarius.orm.database.actions.WizDBDelete Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
The newest version!
package com.wizarius.orm.database.actions;
import com.wizarius.orm.database.DBException;
import com.wizarius.orm.database.connection.DBConnection;
import com.wizarius.orm.database.connection.DBConnectionPool;
import com.wizarius.orm.database.entityreader.DBParsedField;
import com.wizarius.orm.database.entityreader.DBParsedFieldsList;
import lombok.extern.slf4j.Slf4j;
import java.lang.reflect.Field;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.concurrent.atomic.AtomicInteger;
/**
* @author Vladyslav Shyshkin on 21.01.17.
*/
@Slf4j
public class WizDBDelete extends WizAbstractWhereAction> implements IDBDelete {
public WizDBDelete(DBConnectionPool pool, DBParsedFieldsList fields) {
super(pool, fields);
}
/**
* Execute delete prepared statement
*
* @throws DBException on unable to build query or execute query
*/
@Override
public void execute() throws DBException {
try (DBConnection connection = pool.getConnection()) {
execute(connection);
}
}
/**
* Execute delete query
*
* @param connection database connection
* @throws DBException on unable to execute query
*/
@Override
public void execute(DBConnection connection) throws DBException {
try {
PreparedStatement preparedStatement = toPreparedSQLQuery(connection);
preparedStatement.executeUpdate();
} catch (SQLException e) {
throw new DBException("Unable to execute query " + e.getMessage(), e);
}
}
/**
* Execute insert query
*
* @param entity entity to insert
* @throws DBException on unable to insert
*/
@Override
public void execute(Entity entity) throws DBException {
try (DBConnection connection = pool.getConnection()) {
execute(entity, connection);
}
}
/**
* Execute insert query
* If connection is presented, it is assumed that the user himself wants to manage the connection
* The connection will not be automatically closed after the request
*
* @param entity entity to insert
* @param connection connection to database
* @throws DBException on unable to insert
*/
@Override
public void execute(Entity entity, DBConnection connection) throws DBException {
try {
PreparedStatement preparedStatement = toPreparedSQLQuery(entity, connection);
preparedStatement.executeUpdate();
} catch (SQLException e) {
throw new DBException("Unable to execute query " + e.getMessage(), e);
}
}
/**
* Returns sql query with all parameters and where conditions
*
* @param entity entity to delete
* @return query for execution
* @throws DBException unable to build query
*/
@Override
public String toSQLQuery(Entity entity) throws DBException {
try (DBConnection connection = pool.getConnection()) {
return toPreparedSQLQuery(entity, connection).toString();
}
}
/**
* Get sql query with all parameters and where conditions
*/
@Override
public String toSQLQuery() throws DBException {
try (DBConnection connection = pool.getConnection()) {
return toPreparedSQLQuery(connection).toString();
}
}
/**
* Get sql query with all parameters and where conditions
*
* @param connection jdbc connection
* @return sql query
* @throws DBException on unable to build query
*/
private PreparedStatement toPreparedSQLQuery(DBConnection connection) throws DBException {
try {
PreparedStatement prepareStatement = connection.createPrepareStatement(toPreparedSQLQuery());
whereQueryBuilder.setupWhereValues(new AtomicInteger(1), prepareStatement);
return prepareStatement;
} catch (SQLException e) {
throw new DBException("Unable to build prepared statement query. " + e.getMessage(), e);
}
}
/**
* Get sql query with all parameters and where conditions
*
* @param connection jdbc connection
* @return sql query
* @throws DBException on unable to build query
*/
private PreparedStatement toPreparedSQLQuery(Entity entity, DBConnection connection) throws DBException {
DBParsedField primaryKey = fields.getPrimaryKey();
if (primaryKey == null) {
throw new DBException("ID must be present");
}
try {
Field field = primaryKey.getField();
field.setAccessible(true);
where(primaryKey.getDbFieldName(), field.get(entity));
} catch (IllegalAccessException e) {
throw new DBException("Unable to get primary key value");
}
try {
PreparedStatement prepareStatement = connection.createPrepareStatement(toPreparedSQLQuery());
whereQueryBuilder.setupWhereValues(new AtomicInteger(1), prepareStatement);
return prepareStatement;
} catch (SQLException e) {
throw new DBException("Unable to build prepared statement query. " + e.getMessage(), e);
}
}
/**
* Get prepared sql query
*
* @return prepared sql query
*/
private String toPreparedSQLQuery() {
return "DELETE FROM " + getTableName() + "\n" + whereQueryBuilder.getWhereQuery();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy