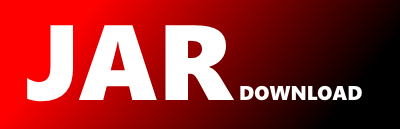
com.wizarius.orm.database.entityreader.EntityQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
The newest version!
package com.wizarius.orm.database.entityreader;
import com.wizarius.orm.database.DBException;
import com.wizarius.orm.database.actions.WizDBDelete;
import com.wizarius.orm.database.actions.WizDBInsert;
import com.wizarius.orm.database.actions.WizDBSelect;
import com.wizarius.orm.database.actions.WizDBUpdate;
import com.wizarius.orm.database.connection.DBConnectionPool;
import java.lang.reflect.Field;
/**
* @author Vladyslav Shyshkin
* Date: 23.03.2020
* Time: 22:31
*/
public class EntityQuery {
private final DBConnectionPool pool;
private final DBParsedFieldsList fields;
public EntityQuery(DBConnectionPool pool,
DBParsedFieldsList fields) {
this.pool = pool;
this.fields = fields;
}
/**
* Returns select query
*
* @return db select query
*/
public WizDBSelect getSelectQuery() {
return new WizDBSelect<>(pool, fields);
}
/**
* Returns insert query
*
* @return db insert query
*/
public WizDBInsert getInsertQuery() {
return new WizDBInsert<>(pool, fields);
}
/**
* Returns update query
*
* @return db update query
*/
public WizDBUpdate getUpdateQuery() {
return new WizDBUpdate<>(pool, fields);
}
/**
* Returns update query
*
* @return db update query
*/
public WizDBDelete getDeleteQuery() {
return new WizDBDelete<>(pool, fields);
}
/**
* Returns connection pool
*
* @return connection pool
*/
public DBConnectionPool getPool() {
return pool;
}
/**
* Return parsed fields
*
* @return parsed fields
*/
public DBParsedFieldsList getFields() {
return fields;
}
/**
* Returns true if object new
*
* @param entity entity
* @return true if new entity
* @throws DBException on unable to check value
*/
public boolean isNew(Entity entity) throws DBException {
DBParsedField primaryKey = fields.getPrimaryKey();
if (primaryKey == null) {
throw new DBException("ID must be present to use this method");
}
Field field = primaryKey.getField();
field.setAccessible(true);
try {
if (primaryKey.getFieldType() == DBSupportedTypes.INTEGER) {
Integer value = (Integer) field.get(entity);
return value == null || value == 0;
} else if (primaryKey.getFieldType() == DBSupportedTypes.LONG) {
Long value = (Long) field.get(entity);
return value == null || value == 0;
} else {
throw new DBException("Incorrect ID type");
}
} catch (IllegalAccessException e) {
throw new DBException("Unable to get field value", e);
}
}
/**
* Returns ID database field name
*
* @return database field name
* @throws DBException on unable to get id field name
*/
public String getIDDbFieldName() throws DBException {
DBParsedField primaryKey = fields.getPrimaryKey();
if (primaryKey == null) {
throw new DBException("ID must be present to use this method");
}
return primaryKey.getDbFieldName();
}
/**
* Returns id value
*
* @return id value
* @throws DBException id value
*/
public Object getIDValue(Entity entity) throws DBException {
DBParsedField primaryKey = fields.getPrimaryKey();
if (primaryKey == null) {
throw new DBException("ID must be present to use this method");
}
Field field = primaryKey.getField();
field.setAccessible(true);
try {
return field.get(entity);
} catch (IllegalAccessException e) {
throw new DBException("Unable to get field value", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy