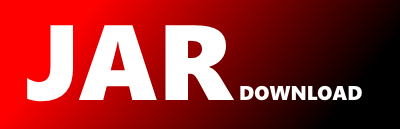
com.wizarius.orm.migrations.savers.DBFileMigrationSaver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
The newest version!
package com.wizarius.orm.migrations.savers;
import com.wizarius.orm.migrations.DBMigrationException;
import com.wizarius.orm.migrations.entities.DBMigrationEntity;
import com.wizarius.orm.migrations.entities.DBMigrationEntityList;
import com.wizarius.orm.utils.WizariusJsonUtils;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
/**
* Created by Vladyslav Shyshkin on 24.11.2017.
*/
public class DBFileMigrationSaver implements IMigrationSaver {
private final String path;
/**
* DB Json migration saver
*
* @param path path to dump file
*/
public DBFileMigrationSaver(String path) throws DBMigrationException {
this.path = path;
if (!isCorrectName()) {
throw new DBMigrationException("Wrong filename");
}
if (!isDumpFileExist()) {
createEmptyFile(path);
}
}
/**
* Save migration
*
* @param migration migration
* @throws DBMigrationException on unable to save
*/
@Override
public void save(DBMigrationEntity migration) throws DBMigrationException {
DBMigrationEntityList migrations = read();
if (migrations.getByLevels(migration.getLevel()) == null) {
migrations.add(migration);
try (FileOutputStream fos = new FileOutputStream(new File(path))) {
String json = WizariusJsonUtils.toPrettyJson(migrations);
if (json == null) {
throw new DBMigrationException("Unable to convert migrations to json");
}
fos.write(json.getBytes());
} catch (IOException e) {
throw new DBMigrationException("Unable to save to file " + e.getMessage(), e);
}
}
}
/**
* Read from dump file
*
* @return connection migrations list
* @throws DBMigrationException on unable to read froom file
*/
@Override
public DBMigrationEntityList read() throws DBMigrationException {
File file = new File(path);
try (FileInputStream fos = new FileInputStream(file)) {
byte[] result = new byte[(int) file.length()];
int read = fos.read(result);
String json = new String(result, StandardCharsets.UTF_8);
if (read != -1 && json.length() != 0) {
return WizariusJsonUtils.fromJson(json, DBMigrationEntityList.class);
} else {
return new DBMigrationEntityList();
}
} catch (IOException e) {
throw new DBMigrationException("Unable to save to file " + e.getMessage(), e);
}
}
/**
* Check if file is exist
*
* @return true if file exist
*/
private boolean isDumpFileExist() {
File file = new File(path);
return file.exists() && file.isFile();
}
/**
* Create new empty file
*
* @param path path to file
*/
private void createEmptyFile(String path) throws DBMigrationException {
File file = new File(path);
try {
if (!file.createNewFile()) {
throw new DBMigrationException("Unable to create connection migration file by path " + path);
}
} catch (IOException e) {
throw new DBMigrationException("Unable to create connection migration file " + e.getMessage());
}
}
/**
* Check if file is correct
*
* @return true if file exist
*/
private boolean isCorrectName() {
return path.endsWith("wizariusDBMigration");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy