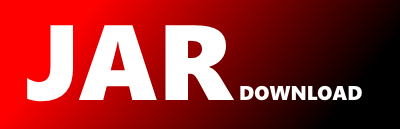
com.wizarius.orm.utils.WizariusJsonUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wizarius-orm Show documentation
Show all versions of wizarius-orm Show documentation
Java orm for Postgres or Mysql with migration system and connection pool
The newest version!
package com.wizarius.orm.utils;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
/**
* Created by Vladyslav Shyshkin on 28.09.17.
*/
@Slf4j
public class WizariusJsonUtils {
private static final ObjectMapper mapper = new ObjectMapper();
static {
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
mapper.configure(MapperFeature.ACCEPT_CASE_INSENSITIVE_PROPERTIES, true);
}
/**
* Object to json
*
* @param object object
* @return json object
*/
public static String toJson(Object object) {
try {
return mapper.writeValueAsString(object);
} catch (JsonProcessingException e) {
log.error("Unable to convert object to json by jackson library", e);
return null;
}
}
/**
* To pretty json
*
* @param object object to convert
* @return pretty json
*/
public static String toPrettyJson(Object object) {
try {
return mapper.writerWithDefaultPrettyPrinter().writeValueAsString(object);
} catch (JsonProcessingException e) {
log.error("Unable to convert object to json by jackson library", e);
return null;
}
}
/**
* From json
*
* @param json json string
* @param clazz class name
* @return converted object
*/
public static T fromJson(String json, Class clazz) {
try {
return mapper.readValue(json, clazz);
} catch (IOException e) {
log.error("Unable to read object from json by jackson library", e);
return null;
}
}
/**
* Read json to object
*
* @param json json string
* @param clazz class to convert
* @param class instance
* @return object
* @throws JsonProcessingException on unable to process json
*/
public static T fromJsonWithError(String json, Class clazz) throws JsonProcessingException {
return mapper.readValue(json, clazz);
}
/**
* Write object as json
*
* @param object object to convert
* @return json string
* @throws JsonProcessingException on unable to process json
*/
public static String toJsonWithError(Object object) throws JsonProcessingException {
return mapper.writeValueAsString(object);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy