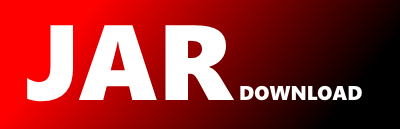
com.wizzdi.messaging.request.MessageCreate Maven / Gradle / Ivy
package com.wizzdi.messaging.request;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.wizzdi.flexicore.security.request.BasicCreate;
import com.wizzdi.messaging.model.Chat;
import com.wizzdi.messaging.model.ChatUser;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class MessageCreate extends BasicCreate {
private Map chatUsers;
private List media;
private String content;
@JsonIgnore
private Map other = new HashMap<>();
private String chatId;
@JsonIgnore
private Chat chat;
@JsonIgnore
private ChatUser sender;
public String getChatId() {
return chatId;
}
public T setChatId(String chatId) {
this.chatId = chatId;
return (T) this;
}
@JsonIgnore
public Chat getChat() {
return chat;
}
public T setChat(Chat chat) {
this.chat = chat;
return (T) this;
}
@JsonIgnore
public ChatUser getSender() {
return sender;
}
public T setSender(ChatUser sender) {
this.sender = sender;
return (T) this;
}
@JsonIgnore
public Map getOther() {
return other;
}
public T setOther(Map other) {
this.other = other;
return (T) this;
}
@JsonAnySetter
public void set(String key, Object val) {
other.put(key, val);
}
@JsonAnyGetter
public Object get(String key) {
return other.get(key);
}
public Map getChatUsers() {
return chatUsers;
}
public T setChatUsers(Map chatUsers) {
this.chatUsers = chatUsers;
return (T) this;
}
public List getMedia() {
return media;
}
public T setMedia(List media) {
this.media = media;
return (T) this;
}
public String getContent() {
return content;
}
public T setContent(String content) {
this.content = content;
return (T) this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy