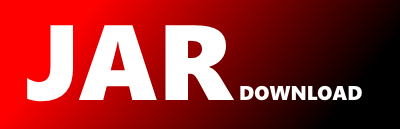
com.wl4g.infra.support.notification.NotificationAutoConfiguration Maven / Gradle / Ivy
/*
* Copyright 2017 ~ 2025 the original author or authors. James Wong
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.wl4g.infra.support.notification;
import static com.wl4g.infra.common.notification.NoneMessageNotifier.DEFAULT_NO_OP;
import static com.wl4g.infra.support.constant.SupportInfraConstant.CONF_PREFIX_INFRA_SUPPORT_NOTIFY;
import static java.util.stream.Collectors.toList;
import java.util.List;
import javax.validation.Validator;
import org.springframework.boot.autoconfigure.condition.ConditionalOnBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import com.wl4g.infra.common.framework.operator.GenericOperatorAdapter;
import com.wl4g.infra.common.notification.MessageNotifier;
import com.wl4g.infra.common.notification.MessageNotifier.NotifierKind;
import com.wl4g.infra.common.notification.apns.ApnsMessageNotifier;
import com.wl4g.infra.common.notification.apns.ApnsNotifierProperties;
import com.wl4g.infra.common.notification.bark.BarkMessageNotifier;
import com.wl4g.infra.common.notification.bark.BarkNotifierProperties;
import com.wl4g.infra.common.notification.dingtalk.DingtalkMessageNotifier;
import com.wl4g.infra.common.notification.dingtalk.DingtalkNotifierProperties;
import com.wl4g.infra.common.notification.email.EmailMessageNotifier;
import com.wl4g.infra.common.notification.email.EmailNotifierProperties;
import com.wl4g.infra.common.notification.facebook.FacebookMessageNotifier;
import com.wl4g.infra.common.notification.facebook.FacebookNotifierProperties;
import com.wl4g.infra.common.notification.qq.QqMessageNotifier;
import com.wl4g.infra.common.notification.qq.QqNotifierProperties;
import com.wl4g.infra.common.notification.sms.AliyunSmsMessageNotifier;
import com.wl4g.infra.common.notification.sms.SmsNotifierProperties;
import com.wl4g.infra.common.notification.twitter.TwitterMessageNotifier;
import com.wl4g.infra.common.notification.twitter.TwitterNotifierProperties;
import com.wl4g.infra.common.notification.vms.AliyunVmsMessageNotifier;
import com.wl4g.infra.common.notification.vms.VmsNotifierProperties;
import com.wl4g.infra.common.notification.wechat.WechatMessageNotifier;
import com.wl4g.infra.common.notification.wechat.WechatNotifierProperties;
/**
* Notification message service auto configuration
*
* @author James Wong
* @version 2019-09-28
* @since v2.0.0
*/
public class NotificationAutoConfiguration {
//
// --- Notify properties. ---
//
@Bean(name = "apnsNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".apns.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".apns")
public ApnsNotifierProperties apnsNotifierProperties() {
return new ApnsNotifierProperties();
}
@Bean(name = "barkNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".bark.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".bark")
public BarkNotifierProperties barkNotifierProperties() {
return new BarkNotifierProperties();
}
@Bean(name = "dingtalkNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".dingtalk.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".dingtalk")
public DingtalkNotifierProperties dingtalkNotifierProperties() {
return new DingtalkNotifierProperties();
}
@Bean(name = "facebookNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".facebook.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".facebook")
public FacebookNotifierProperties facebookNotifierProperties() {
return new FacebookNotifierProperties();
}
@Bean(name = "mailNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".mail.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".mail")
public EmailNotifierProperties emailNotifierProperties() {
return new EmailNotifierProperties();
}
@Bean(name = "qqNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".qq.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".qq")
public QqNotifierProperties qqNotifierProperties() {
return new QqNotifierProperties();
}
@Bean(name = "smsNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".sms.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".sms")
public SmsNotifierProperties smsNotifierProperties() {
return new SmsNotifierProperties();
}
@Bean(name = "vmsNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".vms.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".vms")
public VmsNotifierProperties vmsNotifierProperties() {
return new VmsNotifierProperties();
}
@Bean(name = "wechatNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".wechat.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".wechat")
public WechatNotifierProperties wechatNotifierProperties() {
return new WechatNotifierProperties();
}
@Bean(name = "twitterNotifyProperties")
@ConditionalOnProperty(name = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".twitter.enabled", matchIfMissing = false)
@ConfigurationProperties(prefix = CONF_PREFIX_INFRA_SUPPORT_NOTIFY + ".twitter")
public TwitterNotifierProperties twitterNotifierProperties() {
return new TwitterNotifierProperties();
}
//
// --- Message notifier. ---
//
@Bean
@ConditionalOnBean(ApnsNotifierProperties.class)
public ApnsMessageNotifier apnsMessageNotifier(ApnsNotifierProperties config, Validator validator) {
return new ApnsMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(BarkNotifierProperties.class)
public BarkMessageNotifier barkMessageNotifier(BarkNotifierProperties config, Validator validator) {
return new BarkMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(DingtalkNotifierProperties.class)
public DingtalkMessageNotifier dingtalkMessageNotifier(DingtalkNotifierProperties config, Validator validator) {
return new DingtalkMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(FacebookNotifierProperties.class)
public FacebookMessageNotifier facebookMessageNotifier(FacebookNotifierProperties config, Validator validator) {
return new FacebookMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(EmailNotifierProperties.class)
public EmailMessageNotifier emailMessageNotifier(EmailNotifierProperties config, Validator validator) {
return new EmailMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(QqNotifierProperties.class)
public QqMessageNotifier qqMessageNotifier(QqNotifierProperties config, Validator validator) {
return new QqMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(SmsNotifierProperties.class)
public AliyunSmsMessageNotifier aliyunSmsMessageNotifier(SmsNotifierProperties config, Validator validator) {
return new AliyunSmsMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(VmsNotifierProperties.class)
public AliyunVmsMessageNotifier aliyunVmsMessageNotifier(VmsNotifierProperties config, Validator validator) {
return new AliyunVmsMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(WechatNotifierProperties.class)
public WechatMessageNotifier wechatMessageNotifier(WechatNotifierProperties config, Validator validator) {
return new WechatMessageNotifier(config, validator);
}
@Bean
@ConditionalOnBean(TwitterNotifierProperties.class)
public TwitterMessageNotifier twitterMessageNotifier(TwitterNotifierProperties config, Validator validator) {
return new TwitterMessageNotifier(config, validator);
}
/**
* 1, Cannot inject at this time:
*
*
* @Bean
* public CompositeMessageNotifier compositeMessageNotifier(List<MessageNotifier<NotifierParam>> notifiers) {
* ...
* }
*
*
* 2, Can operate correctly:
*
*
* @Bean
* public CompositeMessageNotifier compositeMessageNotifier(List<MessageNotifier<? extends NotifierParam>> notifiers) {
* ...
* }
*
*
* @param notifiers
* @return
*/
@Bean(BEAN_NOTIFIER_ADAPTER)
@ConditionalOnBean(MessageNotifier.class)
public GenericOperatorAdapter compositeMessageNotifierAdapter(
List notifiers) {
return new GenericOperatorAdapter(
notifiers.stream().map(n -> ((MessageNotifier) n)).collect(toList()), DEFAULT_NO_OP) {
};
}
/**
* Default NONE message notifier.
*/
@Bean
@ConditionalOnMissingBean(name = BEAN_NOTIFIER_ADAPTER)
public NoneMessageNotifierAdapter noneCompositeMessageNotifierAdapter() {
return new NoneMessageNotifierAdapter();
}
/**
* {@link NoneMessageNotifierAdapter}
*
* @author James Wong
* @version v1.0 2020年6月4日
* @since
*/
public static class NoneMessageNotifierAdapter extends GenericOperatorAdapter {
public NoneMessageNotifierAdapter() {
super(DEFAULT_NO_OP);
}
}
public static final String BEAN_NOTIFIER_ADAPTER = "compositeMessageNotifierAdapter";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy