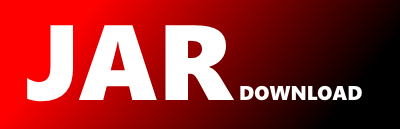
com.orientechnologies.common.util.OArrays Maven / Gradle / Ivy
The newest version!
package com.orientechnologies.common.util;
import java.lang.reflect.Array;
import com.orientechnologies.common.log.OLogManager;
@SuppressWarnings("unchecked")
public class OArrays {
public static T[] copyOf(final T[] iSource, final int iNewSize) {
return (T[]) copyOf(iSource, iNewSize, iSource.getClass());
}
public static T[] copyOf(final U[] iSource, final int iNewSize, final Class extends T[]> iNewType) {
final T[] copy = ((Object) iNewType == (Object) Object[].class) ? (T[]) new Object[iNewSize] : (T[]) Array.newInstance(
iNewType.getComponentType(), iNewSize);
System.arraycopy(iSource, 0, copy, 0, Math.min(iSource.length, iNewSize));
return copy;
}
public static S[] copyOfRange(final S[] iSource, final int iBegin, final int iEnd) {
return copyOfRange(iSource, iBegin, iEnd, (Class) iSource.getClass());
}
public static D[] copyOfRange(final S[] iSource, final int iBegin, final int iEnd, final Class extends D[]> iClass) {
final int newLength = iEnd - iBegin;
if (newLength < 0)
throw new IllegalArgumentException(iBegin + " > " + iEnd);
final D[] copy = ((Object) iClass == (Object) Object[].class) ? (D[]) new Object[newLength] : (D[]) Array.newInstance(
iClass.getComponentType(), newLength);
System.arraycopy(iSource, iBegin, copy, 0, Math.min(iSource.length - iBegin, newLength));
return copy;
}
public static byte[] copyOfRange(final byte[] iSource, final int iBegin, final int iEnd) {
final int newLength = iEnd - iBegin;
if (newLength < 0)
throw new IllegalArgumentException(iBegin + " > " + iEnd);
try {
final byte[] copy = new byte[newLength];
System.arraycopy(iSource, iBegin, copy, 0, Math.min(iSource.length - iBegin, newLength));
return copy;
} catch (OutOfMemoryError e) {
OLogManager.instance().error(null, "Error on copying buffer of size %d bytes", e, newLength);
throw e;
}
}
public static int[] copyOf(final int[] iSource, final int iNewSize) {
final int[] copy = new int[iNewSize];
System.arraycopy(iSource, 0, copy, 0, Math.min(iSource.length, iNewSize));
return copy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy