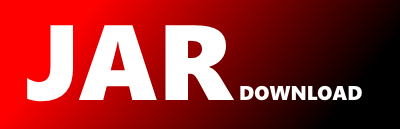
com.orientechnologies.orient.object.serialization.OObjectCustomSerializerSet Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2010-2012 Luca Molino (molino.luca--at--gmail.com)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.orientechnologies.orient.object.serialization;
import java.io.Serializable;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Set;
import com.orientechnologies.orient.core.record.ORecord;
import com.orientechnologies.orient.object.enhancement.OObjectEntitySerializer;
/**
*
* @author Luca Molino (molino.luca--at--gmail.com)
*
*/
@SuppressWarnings("unchecked")
public class OObjectCustomSerializerSet extends HashSet implements OLazyObjectCustomSerializer, Serializable {
private static final long serialVersionUID = -7698875159671927472L;
private final ORecord> sourceRecord;
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy