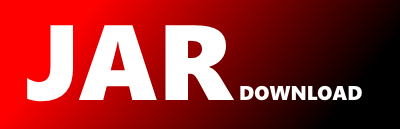
com.spring.boxes.gateway.exception.GatewayExceptionHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boxes-gateway-starter Show documentation
Show all versions of spring-boxes-gateway-starter Show documentation
spring-cookie-boxes-boot-starter
package com.spring.boxes.gateway.exception;
import com.spring.boxes.dollar.CollectionUtils;
import com.spring.boxes.dollar.JSONUtils;
import com.spring.boxes.dollar.support.ApiException;
import com.spring.boxes.dollar.support.ApiResult;
import com.spring.boxes.dollar.support.ViolationError;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.web.reactive.error.ErrorWebExceptionHandler;
import org.springframework.core.Ordered;
import org.springframework.core.annotation.Order;
import org.springframework.core.io.buffer.DataBuffer;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.server.reactive.ServerHttpResponse;
import org.springframework.web.bind.support.WebExchangeBindException;
import org.springframework.web.server.ServerWebExchange;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.Objects;
@Slf4j
@Order(Ordered.HIGHEST_PRECEDENCE)
public class GatewayExceptionHandler implements ErrorWebExceptionHandler {
@Override
public Mono handle(ServerWebExchange exchange, Throwable ex) {
ServerHttpResponse response = exchange.getResponse();
HttpStatus status = response.getStatusCode();
if (response.isCommitted()) {
return Mono.error(ex);
}
if(Objects.isNull(ex)){
response.setStatusCode(HttpStatus.OK);
response.getHeaders().setContentType(MediaType.APPLICATION_JSON_UTF8);
String value = JSONUtils.toJSON(ApiResult.failure());
log.info("[异常机制] 应用异常! 结果:{}", value);
DataBuffer buffer = response.bufferFactory().wrap(value.getBytes(StandardCharsets.UTF_8));
return response.writeWith(Flux.just(buffer));
}
String originPath = exchange.getRequest().getPath().value();
log.info("[异常机制] originPath:{} status:{} message:{}", originPath, status, ex.getLocalizedMessage());
if (ex instanceof WebExchangeBindException) {
WebExchangeBindException ge = (WebExchangeBindException) ex;
ApiResult
© 2015 - 2025 Weber Informatics LLC | Privacy Policy