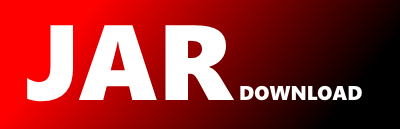
com.springframework.boxes.jmetric.starter.pipeline.ElasticPipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boxes-metric-starter Show documentation
Show all versions of spring-boxes-metric-starter Show documentation
spring-metric-boxes-boot-starter
package com.springframework.boxes.jmetric.starter.pipeline;
import com.alibaba.fastjson.JSONObject;
import com.google.common.collect.Maps;
import com.springframework.boxes.jmetric.starter.BoxesMetricCollector;
import com.springframework.boxes.jmetric.starter.BoxesMetricPipeline;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections4.MapUtils;
import org.apache.commons.lang3.StringUtils;
import org.elasticsearch.action.ActionListener;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.bulk.BulkResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentType;
import java.net.InetAddress;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
@Slf4j
public class ElasticPipeline implements BoxesMetricPipeline {
public static final String DEFAULT_INDEX = "metric";
private String indexName;
private RestHighLevelClient restHighLevelClient;
public ElasticPipeline() {
}
public ElasticPipeline(RestHighLevelClient restHighLevelClient) {
this(DEFAULT_INDEX, restHighLevelClient);
}
public ElasticPipeline(String indexName, RestHighLevelClient restHighLevelClient) {
this.indexName = StringUtils.isBlank(indexName) ? indexName : DEFAULT_INDEX;
this.restHighLevelClient = restHighLevelClient;
}
@Override
public void pipeline(BoxesMetricCollector collector, String key, Object value, long milliseconds) {
Map map = new HashMap<>();
map.put(key, value);
this.pipeline(collector, map, milliseconds);
}
@Override
public void pipeline(BoxesMetricCollector collector, Map metrics, long milliseconds) {
if (null == restHighLevelClient) {
throw new IllegalArgumentException("empty RestHighLevelClient.");
}
if (MapUtils.isEmpty(metrics)) {
return;
}
BulkRequest request = new BulkRequest();
Optional.ofNullable(metrics).orElse(Maps.newHashMap()).forEach((k, v) -> {
JSONObject object = new JSONObject();
object.put("host_name", getHostIP());
object.put("metric_key", k);
object.put("metric_val", v);
object.put("create_time", milliseconds);
// _type the same as _index
request.add(new IndexRequest(StringUtils.trim(indexName).toLowerCase(), StringUtils.trim(indexName).toLowerCase()).source(object, XContentType.JSON));
});
log.debug(request.getDescription());
restHighLevelClient.bulkAsync(
request,
RequestOptions.DEFAULT,
new ActionListener() {
@Override
public void onResponse(BulkResponse bulkItemResponses) {
log.debug(bulkItemResponses.toString());
}
@Override
public void onFailure(Exception e) {
log.error(e.getLocalizedMessage(), e);
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy