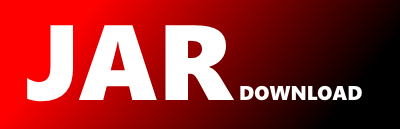
com.springframework.boxes.jmetric.starter.pipeline.FalconPipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boxes-metric-starter Show documentation
Show all versions of spring-boxes-metric-starter Show documentation
spring-metric-boxes-boot-starter
/*--------------------------------------------------------------------------
* Copyright (c) 2010-2020, Elon.su All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* Neither the name of the elon developer nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
* Author: Elon.su, you can also mail [email protected]
*--------------------------------------------------------------------------
*/
package com.springframework.boxes.jmetric.starter.pipeline;
import com.alibaba.fastjson.JSON;
import com.github.rholder.retry.*;
import com.google.common.base.Predicates;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.springframework.boxes.jmetric.starter.BoxesMetricCollector;
import com.springframework.boxes.jmetric.starter.BoxesMetricPipeline;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.http.converter.StringHttpMessageConverter;
import org.springframework.web.client.RestTemplate;
import java.net.InetAddress;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.Callable;
import java.util.concurrent.TimeUnit;
@Slf4j
@Getter
@NoArgsConstructor
public class FalconPipeline implements BoxesMetricPipeline {
private static final Logger LOG = LoggerFactory.getLogger(FalconPipeline.class);
public static final String DEFAULT_AGENT_URL = "http://127.0.0.1:1988/v1/push";
private String httpUrl;
private String endpoint;
private String tags;
private int step;
private static RestTemplate template = new RestTemplate();
static {
template.getMessageConverters().add(new StringHttpMessageConverter(StandardCharsets.UTF_8));
}
private Retryer retry = RetryerBuilder
.newBuilder().retryIfException()
.retryIfResult(Predicates.equalTo(false))
.withBlockStrategy(BlockStrategies.threadSleepStrategy())
.withStopStrategy(StopStrategies.stopAfterAttempt(2))
.withWaitStrategy(WaitStrategies.fixedWait(50L, TimeUnit.MILLISECONDS)).build();
public FalconPipeline(String tags, int step) {
this(DEFAULT_AGENT_URL, tags, step);
}
public FalconPipeline(String url, String tags, int step) {
this(url, InetAddress.getLoopbackAddress().getHostAddress(), tags, step);
}
public FalconPipeline(String httpUrl, String endpoint, String tags, int step) {
this.httpUrl = httpUrl;
this.endpoint = endpoint;
this.tags = tags;
this.step = step;
}
@Override
public void pipeline(BoxesMetricCollector collector, String key, Object value, long milliseconds) {
Map map = new HashMap<>();
map.put(key, value);
this.pipeline(collector, map, milliseconds);
}
@Override
public void pipeline(BoxesMetricCollector collector, Map metrics, long milliseconds) {
List metas = builder(metrics, milliseconds);
try {
retry.call(new Callable() {
@Override
public Boolean call() {
ResponseEntity responseEntity = template.postForEntity(endpoint, JSON.toJSONString(metas), String.class);
if (HttpStatus.OK != responseEntity.getStatusCode()) {
LOG.error("falcon agent post error !");
return false;
}
return true;
}
});
} catch (Exception e) {
log.error(e.getLocalizedMessage(), e);
}
}
public FalconMeta builder(String key, Object value, long milliseconds) {
FalconMeta meta = new FalconMeta();
meta.setEndpoint(this.endpoint);
meta.setStep(this.step);
meta.setTags(this.tags);
meta.setCounterType("GAUGE");
meta.setMetric(StringUtils.trim(key));
meta.setValue(String.valueOf(value));
meta.setTimestamp(milliseconds / 1000);
return meta;
}
public List builder(Map metrics, long milliseconds) {
List list = Lists.newArrayList();
Optional.ofNullable(metrics).orElse(Maps.newHashMap()).forEach((k, v) -> {
FalconMeta meta = builder(k, v, milliseconds);
if (null != meta) {
list.add(meta);
}
});
return list;
}
@Data
@NoArgsConstructor
@AllArgsConstructor
public static class FalconMeta {
private String endpoint;
private String metric;
private String value;
private long timestamp;
private int step;
private String counterType;
private String tags;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy