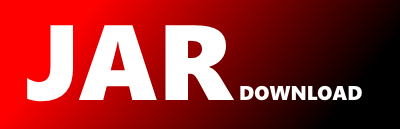
com.springframework.boxes.jmetric.starter.pipeline.MySQLPipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boxes-metric-starter Show documentation
Show all versions of spring-boxes-metric-starter Show documentation
spring-metric-boxes-boot-starter
package com.springframework.boxes.jmetric.starter.pipeline;
import com.alibaba.fastjson.JSON;
import com.google.common.collect.Maps;
import com.springframework.boxes.jmetric.starter.BoxesMetricCollector;
import com.springframework.boxes.jmetric.starter.BoxesMetricPipeline;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections4.MapUtils;
import org.apache.commons.lang3.tuple.Triple;
import org.springframework.jdbc.core.BatchPreparedStatementSetter;
import org.springframework.jdbc.core.JdbcTemplate;
import java.net.InetAddress;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.util.*;
@Slf4j
public class MySQLPipeline implements BoxesMetricPipeline {
private static final String SQL = "insert into metric(host_name, metric_key, metric_val, create_time) values (?, ?, ?, ?)";
private JdbcTemplate jdbcTemplate;
public MySQLPipeline() {
}
public MySQLPipeline(JdbcTemplate jdbcTemplate) {
this.jdbcTemplate = jdbcTemplate;
}
@Override
public void pipeline(BoxesMetricCollector collector, String key, Object value, long milliseconds) {
Map map = new HashMap<>();
map.put(key, value);
this.pipeline(collector, map, milliseconds);
}
@Override
public void pipeline(BoxesMetricCollector collector, Map metrics, long milliseconds) {
if (null == jdbcTemplate) {
throw new IllegalArgumentException("empty JdbcTemplate.");
}
if (MapUtils.isEmpty(metrics)) {
return;
}
List> params = new ArrayList<>();
Optional.ofNullable(metrics).orElse(Maps.newHashMap()).forEach((k, v) -> {
params.add(Triple.of(k, String.valueOf(v), milliseconds));
});
log.debug("mysql pipeline:{}", JSON.toJSON(params));
try {
jdbcTemplate.batchUpdate(SQL, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
Triple args = params.get(i);
ps.setString(1, getHostIP());
ps.setString(2, args.getLeft());
ps.setString(3, args.getMiddle());
ps.setTimestamp(4, new Timestamp(args.getRight()));
}
@Override
public int getBatchSize() {
return params.size();
}
});
} catch (Exception e) {
log.error(e.getLocalizedMessage(), e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy