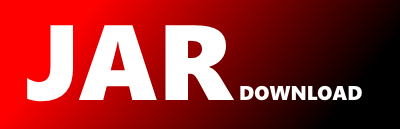
com.spring.boxes.mutant.options.GatewayRouteOptions Maven / Gradle / Ivy
The newest version!
package com.spring.boxes.mutant.options;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import com.spring.boxes.dollar.CollectionUtils;
import com.spring.boxes.dollar.JSONUtils;
import com.spring.boxes.dollar.ValueUtils;
import com.spring.boxes.dollar.enums.OnlineTypeEnum;
import com.spring.boxes.dollar.support.mutant.beans.GatewayInfo;
import lombok.AllArgsConstructor;
import lombok.NoArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.data.redis.core.StringRedisTemplate;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import java.util.stream.Collectors;
@Slf4j
@NoArgsConstructor
@AllArgsConstructor
public class GatewayRouteOptions {
public static final String GATEWAY__INFO_KEY = "_infra:gateway:router:path:%s";
private StringRedisTemplate stringRedisTemplate;
public static GatewayRouteOptions newInstance(StringRedisTemplate stringRedisTemplate) {
return new GatewayRouteOptions(stringRedisTemplate);
}
/**
* 获取所有路由信息
*
* @return 路由信息列表
*/
public List routes() {
Set keys = routeKeys(false);
if (CollectionUtils.isEmpty(keys)) {
return Lists.newArrayList();
}
Set values = keys.stream().map(key -> this.stringRedisTemplate.opsForValue().get(key)).collect(Collectors.toSet());
if (CollectionUtils.isEmpty(values)) {
return Lists.newArrayList();
}
List routes = Lists.newArrayList();
for (String value : values) {
GatewayInfo gatewayInfo = JSONUtils.fromJSON(value, GatewayInfo.class);
if (Objects.nonNull(gatewayInfo)) {
routes.add(gatewayInfo);
}
}
return routes;
}
/**
* 添加或更新路由
*
* @param gatewayInfo 要添加或更新的路由信息
*/
public void route(GatewayInfo gatewayInfo) {
Preconditions.checkArgument(Objects.nonNull(gatewayInfo) && gatewayInfo.getId() > 0);
if (OnlineTypeEnum.ONLINE.getValue() == gatewayInfo.getStatus()) {
String key = getKey(gatewayInfo.getPath(), false);
String val = JSONUtils.toJSON(gatewayInfo);
stringRedisTemplate.opsForValue().set(key, ValueUtils.val(val));
} else {
String key = getKey(gatewayInfo.getPath(), false);
stringRedisTemplate.delete(key);
}
}
/**
* 根据路径获取键
*
* @param path 路径
* @return 键
*/
public String getKey(String path, boolean debug) {
String key = com.spring.boxes.dollar.StringUtils.trimToEmpty(path);
return debug ? String.format("debug:" + GATEWAY__INFO_KEY, key) : String.format(GATEWAY__INFO_KEY, key);
}
/**
* 获取所有路由的键
*
* @return 路由键集合
*/
public Set routeKeys(boolean debug) {
return this.stringRedisTemplate.keys(getKey("*", debug));
}
/**
* 获取路由数量
*
* @return 路由数量
*/
public int total() {
Set keys = routeKeys(false);
return CollectionUtils.size(keys);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy