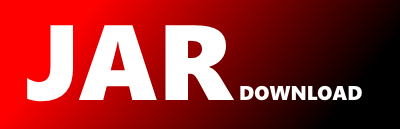
com.spring.boxes.redis.lock.redisson.RedissonLockTemplate Maven / Gradle / Ivy
The newest version!
package com.spring.boxes.redis.lock.redisson;
import com.spring.boxes.redis.lock.support.AbstractLockTemplate;
import org.redisson.api.RLock;
import org.redisson.api.RedissonClient;
import java.util.concurrent.TimeUnit;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class RedissonLockTemplate extends AbstractLockTemplate {
private final RedissonClient redissonClient;
public RedissonLockTemplate(RedissonClient redissonClient) {
this.redissonClient = redissonClient;
}
@Override
public boolean tryLock(String lockKey, long waitTime, long leaseTime) {
boolean success;
try {
RLock lock = redissonClient.getLock(lockKey);
success = lock.tryLock(waitTime, leaseTime, TimeUnit.MILLISECONDS);
} catch (Throwable e) {
String error = String.format("LOCK FAILED: key=%s||tryLockTime=%s||lockExpiredTime=%s", lockKey, waitTime, leaseTime);
throw new IllegalStateException(error, e);
}
return success;
}
@Override
public void unlock(String lockKey) {
try {
RLock lock = redissonClient.getLock(lockKey);
if (lock != null && lock.isHeldByCurrentThread()) {
lock.unlock();
}
} catch (Throwable e) {
String error = String.format("UNLOCK FAILED: key=%s", lockKey);
throw new IllegalStateException(error, e);
}
}
public boolean isLocked(String lockName) {
return redissonClient.getLock(lockName).isLocked();
}
public boolean isHeldByCurrentThread(String lockName) {
return redissonClient.getLock(lockName).isHeldByCurrentThread();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy