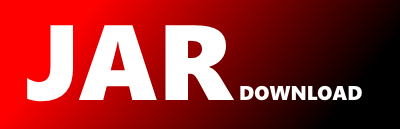
com.spring.boxes.valve.starter.ValveKeyTemplate Maven / Gradle / Ivy
The newest version!
package com.spring.boxes.valve.starter;
import com.google.common.base.Preconditions;
import com.google.common.collect.Maps;
import com.spring.boxes.dollar.support.TerminalType;
import com.spring.boxes.dollar.support.valve.ValveExecutor;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import java.util.Map;
import java.util.Objects;
@Data
@Slf4j
@NoArgsConstructor
@AllArgsConstructor
public class ValveKeyTemplate {
private ValveKeyProperties valveKeyProperties;
public boolean getDeviceValve(String key, String region, TerminalType terminal, String deviceId) {
ValveKeyConfigure valveKeyConfigure = getValveKeyConfigure(key);
Preconditions.checkArgument(Objects.nonNull(key), "ValveKey Not Configure");
return ValveExecutor.getDeviceValve(valveKeyConfigure.getConfigure(), region, terminal, deviceId);
}
public boolean getUserIdValve(String key, String region, TerminalType terminal, long userId) {
ValveKeyConfigure valveKeyConfigure = getValveKeyConfigure(key);
Preconditions.checkArgument(Objects.nonNull(key), "ValveKey Not Configure");
return ValveExecutor.getUserIdValve(valveKeyConfigure.getConfigure(), region, terminal, userId);
}
public Map getUserIdValve(String region, TerminalType terminal, long userId) {
Map valveValues = Maps.newHashMap();
valveKeyProperties.getItems().forEach(e -> {
valveValues.put(e.getKey(), this.getUserIdValve(e.getKey(), region, terminal, userId));
});
return valveValues;
}
public Map getDeviceValve(String region, TerminalType terminal, String deviceId) {
Map valveValues = Maps.newHashMap();
valveKeyProperties.getItems().forEach(e -> {
valveValues.put(e.getKey(), this.getDeviceValve(e.getKey(), region, terminal, deviceId));
});
return valveValues;
}
public ValveKeyConfigure getValveKeyConfigure(String valveKey) {
return valveKeyProperties.getItems().stream().filter(e -> e.getKey().equalsIgnoreCase(valveKey)).findFirst().orElse(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy