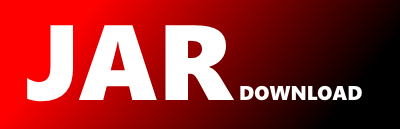
com.spring.boxes.dollar.CollectionUtils Maven / Gradle / Ivy
package com.spring.boxes.dollar;
import com.google.common.base.Function;
import com.google.common.collect.Maps;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections4.ListUtils;
import java.lang.reflect.Array;
import java.util.*;
import java.util.stream.Collectors;
@Slf4j
public class CollectionUtils {
public static boolean isNotEmpty(final Collection> coll) {
return !isEmpty(coll);
}
public static boolean isEmpty(Collection> coll) {
return coll == null || coll.isEmpty();
}
public static int size(Object object) {
return org.apache.commons.collections4.CollectionUtils.size(object);
}
public static void reverseArray(Object[] array) {
int i = 0;
for(int j = array.length - 1; j > i; ++i) {
Object tmp = array[j];
array[j] = array[i];
array[i] = tmp;
--j;
}
}
@SafeVarargs
public static Set ofImmutableSet(E... es) {
Objects.requireNonNull(es, "args es is null.");
return Arrays.stream(es).collect(Collectors.toSet());
}
@SafeVarargs
public static List ofImmutableList(E... es) {
Objects.requireNonNull(es, "args es is null.");
return Arrays.stream(es).collect(Collectors.toList());
}
public static List transform(Collection srcList, Function fun) {
if (isEmpty(srcList)) {
return Collections.EMPTY_LIST;
}
List descList = new ArrayList<>(srcList.size());
srcList.forEach(x -> descList.add(fun.apply(x)));
return descList;
}
public static > Map sortByValue(Map map) {
List> list = new LinkedList<>(map.entrySet());
list.sort(Map.Entry.comparingByValue());
Map result = new LinkedHashMap();
for (Map.Entry entry : list) {
result.put(entry.getKey(), entry.getValue());
}
return result;
}
public static T[] addAll(T[]... arrays) {
if (arrays.length == 1) {
return arrays[0];
}
int length = 0;
for (T[] array : arrays) {
if (array == null) {
continue;
}
length += array.length;
}
T[] result = newArray(arrays.getClass().getComponentType().getComponentType(), length);
length = 0;
for (T[] array : arrays) {
if (array == null) {
continue;
}
System.arraycopy(array, 0, result, length, array.length);
length += array.length;
}
return result;
}
@SuppressWarnings("unchecked")
public static T [] newArray(Class> componentType, int newSize) {
return (T[]) Array.newInstance(componentType, newSize);
}
public static Map getPairMap(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy