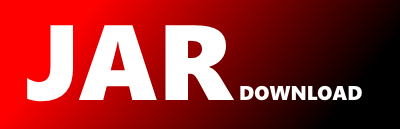
com.spring.boxes.dollar.support.FutureValue Maven / Gradle / Ivy
package com.spring.boxes.dollar.support;
import com.spring.boxes.dollar.TimeUtils;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections4.ListUtils;
import org.apache.commons.lang3.ArrayUtils;
import java.text.MessageFormat;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
@Slf4j
public class FutureValue {
public static T getNoThrow(String actionName, CompletableFuture future, T valueIfException) {
return getNoThrow(actionName, TimeUtils.DEFAULT_TIMEOUT_MILLS, future, valueIfException);
}
public static T getNoThrow(String actionName, int timeoutMills, CompletableFuture future, T valueIfException) {
return getNoThrow(actionName, timeoutMills, false, future, valueIfException);
}
public static T getNoThrow(String actionName, int timeoutMills, boolean cancelIfTimeOut, CompletableFuture future, T valueIfException) {
try {
return future.get(TimeUtils.safeTimeoutMills(timeoutMills), TimeUnit.MILLISECONDS);
} catch (InterruptedException e) {
log.warn("actionName: {} interrupted, error:", actionName, e);
} catch (ExecutionException e) {
log.warn("actionName: {} error:", actionName, e);
reportExecutionException(actionName, new CompletableFuture[]{future});
} catch (TimeoutException e) {
log.warn("actionName: {} timeout, cancelIfTimeOut:{}, error:", actionName, cancelIfTimeOut, e);
reportTimeoutException(actionName, new CompletableFuture[]{future});
future.cancel(cancelIfTimeOut);
} catch (Exception e) {
log.warn("getNoThrow occur Exception, error:", e);
reportExecutionException(actionName, new CompletableFuture[]{future});
}
return valueIfException;
}
public static T getOrThrow(String actionName, CompletableFuture future) {
return getOrThrow(actionName, TimeUtils.DEFAULT_TIMEOUT_MILLS, future);
}
public static T getOrThrow(String actionName, int timeoutMills, CompletableFuture future) {
return getOrThrow(actionName, timeoutMills, false, future);
}
public static T getOrThrow(String actionName, int timeoutMills, boolean cancelIfTimeOut, CompletableFuture future) {
try {
return future.get(TimeUtils.safeTimeoutMills(timeoutMills), TimeUnit.MILLISECONDS);
} catch (InterruptedException e) {
log.warn("actionName: {} interrupted, error:", actionName, e);
throw new ApiException(e);
} catch (ExecutionException e) {
log.warn("actionName: {} error:", actionName, e);
reportExecutionException(actionName, new CompletableFuture[]{future});
throw new ApiException(MessageFormat.format("actionName:{0} execute exception", actionName), e);
} catch (TimeoutException e) {
log.warn("actionName: {} timeout, cancelIfTimeOut:{}, error:", actionName, cancelIfTimeOut, e);
reportTimeoutException(actionName, new CompletableFuture[]{future});
future.cancel(cancelIfTimeOut);
throw new ApiException(MessageFormat.format("actionName:{0} execute timeout", actionName), e);
}
}
public static void waitDoneOrThrow(String actionName, List> futures) {
waitDoneOrThrow(actionName, TimeUtils.DEFAULT_TIMEOUT_MILLS, ListUtils.emptyIfNull(futures).toArray(new CompletableFuture>[0]));
}
public static void waitDoneOrThrow(String actionName, CompletableFuture>... futures) {
waitDoneOrThrow(actionName, TimeUtils.DEFAULT_TIMEOUT_MILLS, futures);
}
public static void waitDoneOrThrow(String actionName, int timeoutMills, List> futures) {
waitDoneOrThrow(actionName, timeoutMills, false, ListUtils.emptyIfNull(futures).toArray(new CompletableFuture>[0]));
}
public static void waitDoneOrThrow(String actionName, int timeoutMills, CompletableFuture>... futures) {
waitDoneOrThrow(actionName, timeoutMills, false, futures);
}
public static void waitDoneOrThrow(String actionName, int timeoutMills, boolean cancelIfTimeOut, CompletableFuture>... futures) {
if (ArrayUtils.isEmpty(futures)) {
return;
}
CompletableFuture allFutures = CompletableFuture.allOf(futures);
try {
allFutures.get(TimeUtils.safeTimeoutMills(timeoutMills), TimeUnit.MILLISECONDS);
} catch (InterruptedException e) {
log.warn("actionName: {} interrupted, error:", actionName, e);
throw new ApiException(e);
} catch (ExecutionException e) {
log.warn("actionName: {} error:", actionName, e);
reportExecutionException(actionName, futures);
throw new ApiException(e);
} catch (TimeoutException e) {
log.warn("actionName: {} timeout, cancelIfTimeOut:{}, error:", actionName, cancelIfTimeOut, e);
reportTimeoutException(actionName, futures);
allFutures.cancel(cancelIfTimeOut);
throw new ApiException(MessageFormat.format("actionName: {0} execute timeout", actionName), e);
}
}
public static void waitDoneNoThrow(String actionName, List> futures) {
waitDoneNoThrow(actionName, TimeUtils.DEFAULT_TIMEOUT_MILLS, futures.toArray(new CompletableFuture>[0]));
}
public static void waitDoneNoThrow(String actionName, CompletableFuture>... futures) {
waitDoneNoThrow(actionName, TimeUtils.DEFAULT_TIMEOUT_MILLS, futures);
}
public static void waitDoneNoThrow(String actionName, int timeoutMills, List> futures) {
waitDoneNoThrow(actionName, timeoutMills, false, ListUtils.emptyIfNull(futures).toArray(new CompletableFuture>[0]));
}
public static void waitDoneNoThrow(String actionName, int timeoutMills, CompletableFuture>... futures) {
waitDoneNoThrow(actionName, timeoutMills, false, futures);
}
public static void waitDoneNoThrow(String actionName, int timeoutMills, boolean cancelIfTimeOut, CompletableFuture>... futures) {
if (ArrayUtils.isEmpty(futures)) {
return;
}
CompletableFuture allFeature = CompletableFuture.allOf(futures);
try {
allFeature.get(TimeUtils.safeTimeoutMills(timeoutMills), TimeUnit.MILLISECONDS);
} catch (InterruptedException e) {
log.warn("actionName: {} interrupted, error:", actionName, e);
} catch (ExecutionException e) {
log.warn("actionName: {} error:", actionName, e);
reportExecutionException(actionName, futures);
} catch (TimeoutException e) {
log.warn("actionName: {} timeout, cancelIfTimeOut:{}, error:", actionName, cancelIfTimeOut, e);
reportTimeoutException(actionName, futures);
allFeature.cancel(cancelIfTimeOut);
} catch (Exception e) {
log.warn("actionName: {} error:", actionName, e);
reportExecutionException(actionName, futures);
}
}
private static void reportExecutionException(String actionName, CompletableFuture>[] futures) {
for (int i = 0; i < futures.length; i++) {
if (futures[i].isCompletedExceptionally()) {
log.warn("[FutureValue] {} futures[{}] isCompletedExceptionally", actionName, i);
break;
}
}
}
private static void reportTimeoutException(String actionName, CompletableFuture>[] futures) {
for (int i = 0; i < futures.length; i++) {
if (!futures[i].isDone()) {
log.warn("[FutureValue] {} futures[{}] execute timeout", actionName, i);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy