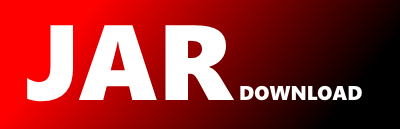
com.spring.boxes.dollar.support.graphql.GraphqlPaths Maven / Gradle / Ivy
The newest version!
package com.spring.boxes.dollar.support.graphql;
import com.spring.boxes.dollar.support.graphql.instrument.NestFutureTask;
import com.spring.boxes.dollar.support.graphql.instrument.NestInstrumentationState;
import graphql.analysis.QueryVisitorFieldEnvironment;
import graphql.execution.ResultPath;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.collections4.MapUtils;
import org.apache.commons.lang3.StringUtils;
import java.util.Map;
import java.util.Objects;
@Slf4j
public class GraphqlPaths {
public static final String PATH_SEPARATOR = "#";
// 处理子任务,如果子任务有多个子任务回溯处理
public static void completeSubTask(NestFutureTask
© 2015 - 2024 Weber Informatics LLC | Privacy Policy