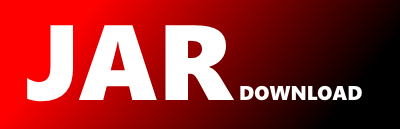
com.spring.boxes.dollar.support.graphql.GraphqlUtils Maven / Gradle / Ivy
The newest version!
package com.spring.boxes.dollar.support.graphql;
import cn.hutool.core.bean.BeanUtil;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.netflix.graphql.dgs.DgsDataFetchingEnvironment;
import com.spring.boxes.dollar.support.MoreStream;
import com.spring.boxes.dollar.term.Authority;
import graphql.ExecutionResult;
import graphql.GraphQLError;
import graphql.schema.DataFetchingEnvironment;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.collections4.ListUtils;
import org.apache.commons.collections4.MapUtils;
import org.reflections.ReflectionUtils;
import java.lang.reflect.Field;
import java.util.*;
import java.util.stream.Collectors;
public class GraphqlUtils {
public static Object getVariableValue(DataFetchingEnvironment dfe, String key){
DgsDataFetchingEnvironment environment = (DgsDataFetchingEnvironment) dfe;
Map variables = environment.getVariables();
return MapUtils.getObject(variables, key);
}
public static T getArgumentValue(DataFetchingEnvironment dfe, String key){
DgsDataFetchingEnvironment environment = (DgsDataFetchingEnvironment) dfe;
return environment.getArgument(key);
}
public static List getGraphQLErrors(ExecutionResult executionResult) {
if (CollectionUtils.isEmpty(executionResult.getErrors())) {
return Lists.newArrayList();
}
return MoreStream.toList(executionResult.getErrors(), GraphQLError::getMessage);
}
@SuppressWarnings("unchecked")
public static Map getGraphqlFields(Class> clazz) {
Set fieldSet = ReflectionUtils.getAllFields(clazz);
Map> fieldTypes = ListUtils.emptyIfNull(Lists.newArrayList(fieldSet))
.stream()
.collect(Collectors.toMap(Field::getName, Field::getType, (o, n) -> o));
return GraphqlTypes.getGraphqlType(fieldTypes);
}
public static Map sourceToMap(DataFetchingEnvironment environment) {
Map variable = Maps.newHashMap();
Object source = environment.getSource();
if (source instanceof Map) {
Map map = (Map) source;
if (MapUtils.isNotEmpty(map)) {
variable.putAll(map);
}
} else {
Map map = BeanUtil.beanToMap(source);
variable.putAll(map);
}
return variable;
}
public static void main(String[] args) {
BeanUtil.beanToMap(new Authority());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy