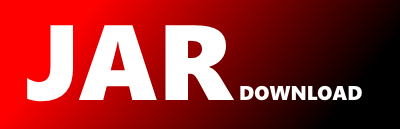
com.spring.boxes.dollar.support.graphql.scalar.LongScalar Maven / Gradle / Ivy
The newest version!
package com.spring.boxes.dollar.support.graphql.scalar;
import graphql.language.IntValue;
import graphql.schema.Coercing;
import graphql.schema.CoercingParseLiteralException;
import graphql.schema.CoercingParseValueException;
import graphql.schema.CoercingSerializeException;
import lombok.Value;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class LongScalar implements Coercing {
@Override
public Long serialize(Object dataFetcherResult) throws CoercingSerializeException {
log.debug("input.serialize:" + dataFetcherResult.toString());
if (dataFetcherResult instanceof Long || dataFetcherResult instanceof Integer || dataFetcherResult instanceof Byte) {
return (Long) dataFetcherResult;
}
if (dataFetcherResult instanceof IntValue) {
IntValue intValue = (IntValue) dataFetcherResult;
return Long.parseLong(intValue.getValue().toString());
}
throw new CoercingSerializeException("Not a valid Long");
}
@Override
public Long parseValue(Object input) throws CoercingParseValueException {
log.debug("input.parseValue:" + input.toString());
return Long.parseLong(input.toString());
}
@Override
public Long parseLiteral(Object input) throws CoercingParseLiteralException {
log.debug("input:" + input.toString());
if (input instanceof Long || input instanceof Integer || input instanceof Byte) {
return Long.parseLong(input.toString());
}
if (input instanceof IntValue) {
IntValue intValue = (IntValue) input;
return Long.parseLong(intValue.getValue().toString());
}
throw new CoercingParseLiteralException("Value is not a valid Long");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy