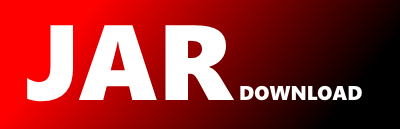
com.xavax.util.CollectionFactory Maven / Gradle / Ivy
//
// Copyright 2010 by Xavax, Inc. All Rights Reserved.
// Use of this software is allowed under the Xavax Open Software License.
// http://www.xavax.com/xosl.html
//
package com.xavax.util;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.Map;
import java.util.PriorityQueue;
import java.util.Queue;
import java.util.Set;
import java.util.Stack;
import java.util.TreeSet;
import java.util.TreeMap;
import java.util.WeakHashMap;
import java.util.concurrent.ConcurrentLinkedQueue;
/**
* CollectionFactory creates new collections from the Collections library.
* This reduces imports and in pre-Java7 code eliminates the need to
* specify the type parameters on the right side of a statement.
*
* @author [email protected]
*/
public final class CollectionFactory {
/**
* Private constructor provided to keep the compiler from generating
* a public default constructor.
*/
private CollectionFactory() {}
/**
* Returns a new array list.
*
* @param the list element type.
* @return a new array list.
*/
public static List arrayList()
{
return new ArrayList();
}
/**
* Returns a new array list with the specified initial capacity.
*
* @param the list element type.
* @param capacity the initial capacity of the list.
* @return a new array list.
*/
public static List arrayList(final int capacity)
{
return new ArrayList(capacity);
}
/**
* Returns a new concurrent linked queue.
*
* @param the queue element type.
* @return a new concurrent linked queue.
*/
public static ConcurrentLinkedQueue concurrentLinkedQueue() {
return new ConcurrentLinkedQueue();
}
/**
* Returns a new hash map.
*
* @param the map key type.
* @param the map value type.
* @return a new hash map.
*/
public static Map hashMap()
{
return new HashMap();
}
/**
* Returns a new hash set.
*
* @param the hash set element type.
* @return a new hash set.
*/
public static Set hashSet()
{
return new HashSet();
}
/**
* Returns a new linked hash map.
*
* @param the map key type.
* @param the map value type.
* @return a new linked hash map.
*/
public static Map linkedHashMap() {
return new LinkedHashMap();
}
/**
* Returns a new linked hash set.
*
* @param the set element type.
* @return a new linked hash set.
*/
public static Set linkedHashSet() {
return new LinkedHashSet();
}
/**
* Returns a new linked list.
*
* @param the list element type.
* @return a new linked list.
*/
public static List linkedList()
{
return new LinkedList();
}
/**
* Returns a new priority queue.
*
* @param the queue element type.
* @return a new priority queue.
*/
public static Queue priorityQueue() {
return new PriorityQueue();
}
/**
* Returns a new stack.
*
* @param the stack value type.
* @return a new stack.
*/
public static Stack stack() {
return new Stack();
}
/**
* Returns a new tree map.
*
* @param the map key type.
* @param the map value type.
* @return a new tree map.
*/
public static Map treeMap()
{
return new TreeMap();
}
/**
* Returns a new tree map.
*
* @param the map key type.
* @param the map value type.
* @param comparator the comparator used to compare keys.
* @return a new tree map.
*/
public static Map treeMap(final Comparator comparator)
{
return new TreeMap(comparator);
}
/**
* Returns a new tree set.
*
* @param the set element type.
* @return a new tree set.
*/
public static Set treeSet() {
return new TreeSet();
}
/**
* Returns a new weak hash map.
*
* @param the map key type.
* @param the map value type.
* @return a new weak hash map.
*/
public static Map weakHashMap() {
return new WeakHashMap();
}
/**
* Returns a new iterable enumeration.
*
* @param the element type.
* @param enumeration the enumeration to be iterated.
* @return a new iterable enumeration.
*/
public static IterableEnumeration
iterableEnumeration(final Enumeration enumeration) {
return new IterableEnumeration(enumeration);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy