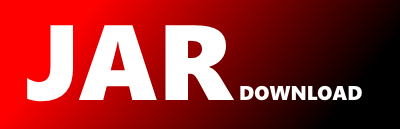
com.xavax.util.IterableEnumeration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xcore Show documentation
Show all versions of xcore Show documentation
Core classes used by other Xavax projects.
//
// Copyright 2011 by Xavax, Inc. All Rights Reserved.
// Use of this software is allowed under the Xavax Open Software License.
// http://www.xavax.com/xosl.html
//
package com.xavax.util;
import java.util.Enumeration;
import java.util.Iterator;
/**
* IterableEnumeration is an adaptor that allows an Enumeration to
* implement Iterable so it can be used in a for-each statement.
* The design was originally described by Dr. Heinz M. Kabutz in
* the following article.
* http://www.javaspecialists.eu/archive/Issue107.html
*
* @param the enumeration element type.
*/
public class IterableEnumeration implements Iterable {
private final Enumeration enumeration;
/**
* Construct an InterableEnumeration from an existing enumeration.
*
* @param enumeration the enumeration to be iterated.
*/
public IterableEnumeration(final Enumeration enumeration) {
this.enumeration = enumeration;
}
/**
* Returns an Iterator for the underlying Enumeration, thereby
* making it iterable.
*
* @return an Iterator for this enumeration.
*/
public Iterator iterator() {
return new Iterator() {
/**
* Returns true if this iterator has more items.
*
* @return true if this iterator has more items.
*/
@Override
@SuppressWarnings("PMD.CommentRequired")
public boolean hasNext() {
return enumeration.hasMoreElements();
}
/**
* Returns the next item.
*
* @return the next item.
*/
public T next() {
return enumeration.nextElement();
}
/**
* This method of the Iterator interface is not supported.
*/
public void remove() {
throw new UnsupportedOperationException();
}
};
}
/**
* Returns an IterableEnumeration created from the enumeration.
*
* @param the enumeration element type.
* @param enumeration the enumeration to be iterated.
* @return an IterableEnumeration created from the enumeration.
*/
public static IterableEnumeration create(final Enumeration enumeration) {
return new IterableEnumeration(enumeration);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy