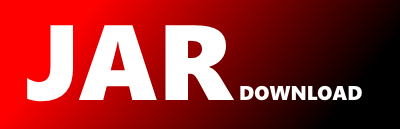
com.xceptance.xlt.nocoding.util.ActionSubItemUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xlt-nocoding Show documentation
Show all versions of xlt-nocoding Show documentation
A library based on XLT to run Web test cases that are written in either YAML or CSV format.
The newest version!
/*
* Copyright (c) 2013-2023 Xceptance Software Technologies GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.xceptance.xlt.nocoding.util;
import java.util.List;
import com.xceptance.xlt.nocoding.command.action.AbstractActionSubItem;
import com.xceptance.xlt.nocoding.command.action.request.Request;
import com.xceptance.xlt.nocoding.command.action.response.Response;
import com.xceptance.xlt.nocoding.command.action.subrequest.AbstractSubrequest;
import com.xceptance.xlt.nocoding.util.context.Context;
import com.xceptance.xlt.nocoding.util.storage.DataStorage;
/**
* Utility class for {@link AbstractActionSubItem}. Provides static methods for verifying the order, and filling default
* data.
*
* @author ckeiner
*/
public class ActionSubItemUtil
{
/**
* Asserts that the order of {@link Request}, {@link Response}, and {@link AbstractSubrequest} is correct.
*
* @param actionItems
* The list of {@link AbstractActionSubItem}s you want to assert the order of
*/
public static void assertOrder(final List actionItems)
{
boolean hasRequest = false;
boolean hasResponse = false;
boolean hasSubrequest = false;
for (final AbstractActionSubItem actionItem : actionItems)
{
// If it is a Request
if (actionItem instanceof Request)
{
// Verify there is no other request, response or subrequest before it
if (hasResponse || hasSubrequest || hasRequest)
{
throw new IllegalArgumentException("Request cannot be defined after a response or subrequest.");
}
// Set hasRequest to true
hasRequest = true;
}
// If it is a Response, set hasResponse to true
else if (actionItem instanceof Response)
{
hasResponse = true;
// If an AbstractSubrequest is before Response,throw an error
if (hasSubrequest)
{
throw new IllegalArgumentException("Response mustn't be defined after subrequests.");
}
}
// If an AbstractSubrequest is found, set hasSubrequest to true
else if (actionItem instanceof AbstractSubrequest && !hasSubrequest)
{
hasSubrequest = true;
}
}
}
/**
* Adds a default {@link Request} and default {@link Response} if none is found in the list of
* {@link AbstractActionSubItem}.
*
* @param actionItems
* The list of {@link AbstractActionSubItem}s to add the default items to
* @param context
* The {@link Context} with the {@link DataStorage}.
*/
public static void fillDefaultData(final List actionItems, final Context> context)
{
boolean hasRequest = false;
boolean hasResponse = false;
for (final AbstractActionSubItem actionItem : actionItems)
{
if (actionItem instanceof Request)
{
hasRequest = true;
}
if (actionItem instanceof Response)
{
hasResponse = true;
}
}
if (!hasRequest)
{
actionItems.add(0, new Request());
}
if (!hasResponse)
{
actionItems.add(1, new Response());
}
}
/**
* Either gets a default name from {@link Context#getDefaultItems()} or calculates a name out of the
* start
and {@link Context#getActionIndex()}.
*
* @param start
* The String the defaultName should start with if no default name was defined
* @param context
* The Context with the {@link DataStorage}
* @return String that starts with start + "-"
and adds the current index of the action items to it
*/
public static String getDefaultName(final Context> context, final String start)
{
// Get the default name from the data storage
String output = context.getDefaultItems().get(Constants.NAME);
// If there is no default name
if (output == null || output.isEmpty())
{
// Assign start + "-x" to name, whereas x is the index of the current scriptItem
output = start + "-" + context.getActionIndex();
}
return output;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy