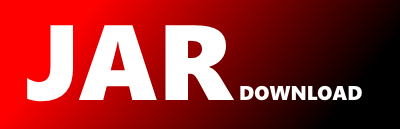
xdev.ui.XdevNmDualListBox Maven / Gradle / Ivy
package xdev.ui;
/*-
* #%L
* XDEV Component Suite
* %%
* Copyright (C) 2011 - 2021 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import xdev.db.DBConnection;
import xdev.db.DBException;
import xdev.db.sql.Condition;
import xdev.ui.ManyToMany.State;
import xdev.util.IntList;
import xdev.util.StringUtils;
import xdev.util.logging.LoggerFactory;
import xdev.util.logging.XdevLogger;
import xdev.vt.KeyValues;
import xdev.vt.VirtualTable;
import xdev.vt.VirtualTable.VirtualTableRow;
import xdev.vt.VirtualTableColumn;
import xdev.vt.VirtualTableException;
/**
* A specialized version of {@link XdevDualListBox} to be used for editing
* many-to-many relationships within {@link VirtualTable} instances. This class
* can be used within {@link Formular} instances to select multiple
* {@link VirtualTableRow}s from a detail table to a master record.
*
* Prior to using this component, its
* {@link #setModel(VirtualTable, String, String, boolean, SelectionMode)}
* method has to be called providing information on a {@link VirtualTable} that
* represents the N:M relationship. The related master table is retrieved
* automatically from the surrounding {@link Formular} component of this
* instance.
*
*
* @author XDEV Software
* @see XdevDualListBox
* @see XdevNmListBox
*/
public class XdevNmDualListBox extends XdevDualListBox implements ManyToManyComponent
{
/**
* Logger instance for this class.
*/
private static final XdevLogger log = LoggerFactory
.getLogger(XdevNmDualListBox.class);
/**
* serialVersionUID.
*/
private static final long serialVersionUID = 1L;
/**
* Instance of helper class for managing nm state.
*/
private State state;
/**
* The state of the nm-table at the time of refreshing.
*/
private VirtualTable savedState;
/**
* The state of the available lists virtual table at the time of refreshing.
*/
private VirtualTable availableSavedState;
/**
* the column name used as item representation.
*/
private String itemColumn;
/**
* the column name used as data representation.
*/
private String dataColumn;
/**
* the list with the current selected items.
*/
// private List selectionState;
/**
* Creates a new instance of {@link XdevNmDualListBox}.
*/
public XdevNmDualListBox()
{
super();
// don't display sort buttons per default, as sorting doesn't happen in
// nm-table
this.setShowSortButtons(false);
}
/**
* Updates the underlying model with data from the {@link VirtualTable} vt.
*
* @param nmVirtualTable
* the {@link VirtualTable} containing the data for the model
* @param itemCol
* columnname to fill item
from or string with
* variables like "{%SURNAME} {%NAME} - {%AGE}"
* @param dataCol
* column name to fill data
from, or multiple
* columns names, comma-separated
* @param queryData
* if {@code true}, the best fitting select for this {@code vt}
* is used
*
* @see ItemList#setModel(VirtualTable, String, String, boolean)
* @see StringUtils#format(String, xdev.util.StringUtils.ParameterProvider)
*/
public void setModel(VirtualTable nmVirtualTable, String itemCol, String dataCol,
boolean queryData)
{
setModel(nmVirtualTable,itemCol,dataCol,queryData,false);
}
/**
* Updates the underlying model with data from the {@link VirtualTable} vt.
*
* @param nmVirtualTable
* the {@link VirtualTable} containing the data for the model
* @param itemCol
* columnname to fill item
from or string with
* variables like "{%SURNAME} {%NAME} - {%AGE}"
* @param dataCol
* column name to fill data
from, or multiple
* columns names, comma-separated
* @param queryData
* if {@code true}, the best fitting select for this {@code vt}
* is used
* @param selectiveQuery
* if {@code true}, only the used columns
are
* queried
*
* @see ItemList#setModel(VirtualTable, String, String, boolean, boolean)
* @see StringUtils#format(String, xdev.util.StringUtils.ParameterProvider)
*
* @since 5.0
*/
public void setModel(VirtualTable nmVirtualTable, final String itemCol, final String dataCol,
final boolean queryData, final boolean selectiveQuery)
{
this.itemColumn = itemCol;
this.dataColumn = dataCol;
/*
* Dont't use unique indices in this VT because most of the values are
* not used, default values will be added and
* UniqueIndexDoubleValuesException are thrown
*/
nmVirtualTable = nmVirtualTable.clone(!queryData);
nmVirtualTable.setCheckUniqueIndexDoubleValues(false);
availableList.setModel(nmVirtualTable,this.itemColumn,this.dataColumn,queryData,selectiveQuery);
nmVirtualTable = nmVirtualTable.clone(!queryData);
nmVirtualTable.setCheckUniqueIndexDoubleValues(false);
selectedList.setModel(nmVirtualTable,this.itemColumn,this.dataColumn,queryData,selectiveQuery);
/*
* Don't query all the data of the nm-table, use the refresh method
* instead to get a reasonable result.
*/
if(queryData)
{
Formular form = FormularSupport.getFormularOf(this);
if(form != null)
{
VirtualTable masterVT = form.getVirtualTable();
if(masterVT != null)
{
refresh(masterVT.createRow());
}
}
else
{
// #11981 avoid initial fill of the selectedList, if no form
// is configured
selectedList.getItemList().clear();
}
}
availableItems = availableList.getItemList();
selectedItems = selectedList.getItemList();
update();
// selectionState = selectedItems.getDataAsList();
}
/**
* Updates the underlying model with data from the {@link VirtualTable} vt.
*
* @param nmVirtualTable
* the {@link VirtualTable} containing the data for the model
* @param itemCol
* columnname to fill item
from or string with
* variables like "{%SURNAME} {%NAME} - {%AGE}"
* @param dataCol
* column name to fill data
from, or multiple
* columns names, comma-separated
* @param queryData
* if {@code true}, the best fitting select for this {@code vt}
* is used
*
* @see ItemList#setModel(VirtualTable, String, String, boolean)
* @see StringUtils#format(String, xdev.util.StringUtils.ParameterProvider)
*/
public void setModel(VirtualTable nmVirtualTable, String itemCol, String dataCol,
boolean queryData, SelectionMode selectionMode)
{
setModel(nmVirtualTable,itemCol,dataCol,queryData,false,selectionMode);
}
/**
* Updates the underlying model with data from the {@link VirtualTable} vt.
*
* @param nmVirtualTable
* the {@link VirtualTable} containing the data for the model
* @param itemCol
* columnname to fill item
from or string with
* variables like "{%SURNAME} {%NAME} - {%AGE}"
* @param dataCol
* column name to fill data
from, or multiple
* columns names, comma-separated
* @param queryData
* if {@code true}, the best fitting select for this {@code vt}
* is used
* @param selectiveQuery
* if {@code true}, only the used columns
are
* queried
*
* @see ItemList#setModel(VirtualTable, String, String, boolean, boolean)
* @see StringUtils#format(String, xdev.util.StringUtils.ParameterProvider)
*
* @since 5.0
*/
public void setModel(final VirtualTable nmVirtualTable, final String itemCol, final String dataCol,
final boolean queryData, final boolean selectiveQuery, SelectionMode selectionMode)
{
this.selectionMode = selectionMode;
this.setModel(nmVirtualTable,itemCol,dataCol,queryData,selectiveQuery);
}
/**
* Refresh updates the displayed state of the component according to the
* specified master record.
*
* @param masterRecord
* the master record of this component.
*/
@Override
public void refresh(VirtualTableRow masterRecord)
{
try
{
state = new State(masterRecord,availableList.getVirtualTable());
selectedList.getItemList().clear();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy