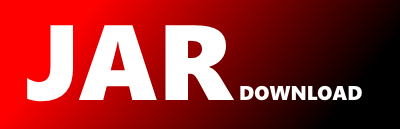
xdev.ui.ganttchart.model.EntryVTMapper Maven / Gradle / Ivy
package xdev.ui.ganttchart.model;
/*-
* #%L
* XDEV BI Suite
* %%
* Copyright (C) 2011 - 2021 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import static xdev.util.ObjectUtils.notNull;
import xdev.ui.ganttchart.RangeProvider;
import xdev.ui.ganttchart.SpecialRelationalUpdatableEntryCreator;
import xdev.ui.ganttchart.UpdateableGanttEntry;
import xdev.vt.VirtualTable;
import xdev.vt.VirtualTable.VirtualTableRow;
import com.jidesoft.gantt.GanttEntry;
import com.jidesoft.range.Range;
/**
* An implementation of {@link GanttEntryMapper} for mapping
* {@link VirtualTableRow} instances to {@link GanttEntry}s.
*
*
* @param
* the data type, for example Date
or
* Integer
.
* @param
* the customized {@link GanttEntry} type.
*
* @author XDEV Software jwill
* @since 4.0
*/
public class EntryVTMapper, T> implements
GanttEntryMapper
{
/**
* The apropriate range provider logic for the handled type T.
*/
private final RangeProvider rangeProvider;
/**
* The special EntryCreator for {@link VirtualTable} based implementations.
*/
private final SpecialRelationalUpdatableEntryCreator entryCreator;
/**
* The data container for VirtualTableRow (2d) mappings, to avoid unsafe
* casts.
*/
private final XdevGanttEntryVTMappings dataContainer;
public XdevGanttEntryVTMappings getDataContainer()
{
return dataContainer;
}
/**
*
* @param rangeProvider
* the {@link RangeProvider} which returns the a concrete
* {@link Range} for the set data type T
(see class
* description).
* @param entryCreator
* the {@link SpecialRelationalUpdatableEntryCreator} which
* returns a concrete customized {@link GanttEntry} for the set
* type S
(see class descpription).
* @param dataContainer
* the {@link XdevGanttEntryVTMappings} which provides data
* mapping information.
*/
public EntryVTMapper(final RangeProvider rangeProvider,
final SpecialRelationalUpdatableEntryCreator entryCreator,
XdevGanttEntryVTMappings dataContainer)
{
super();
this.rangeProvider = notNull(rangeProvider);
this.entryCreator = notNull(entryCreator);
this.dataContainer = notNull(dataContainer);
}
/**
* {@inheritDoc}
*/
@Override
public RangeProvider getRangeProvider()
{
return rangeProvider;
}
/**
* Returns the given concrete EntryCreator which provides new Entries
* related to the given EntryType.
*
* @return the concrete entryCreator for EntryType.
*/
public SpecialRelationalUpdatableEntryCreator getEntryCreator()
{
return entryCreator;
}
/**
* {@inheritDoc}
*/
@Override
public S dataToGanttEntry(final VirtualTableRow row)
{
final S entry = this.getTypeEntry(row);
if(this.dataContainer.getId() != null)
{
entry.setId(row.get(this.dataContainer.getId()));
}
if(this.dataContainer.getCompletion() != null)
{
if(row.get(dataContainer.getCompletion()) != null)
{
entry.setCompletion(row.get(dataContainer.getCompletion()));
}
else
{
// default value
entry.setCompletion(0.0);
}
}
if(this.dataContainer.getDescription() != null)
{
if(row.get(dataContainer.getDescription()) != null)
{
entry.setName(row.get(dataContainer.getDescription()));
}
else
{
// default value
entry.setName("New Entry");
}
}
if(this.dataContainer.getRoot() != null)
{
entry.setRoot(row.get(dataContainer.getRoot()));
}
return entry;
}
/**
* Prepares the {@link GanttEntry} converted from the given
* {@link VirtualTableRow}.
*
* @param row
* the VirtualTableRow
to get the
* GanttEntry
from.
* @return the prepared GanttEntry
.
*/
private S getTypeEntry(final VirtualTableRow row)
{
final T start = row.get(this.dataContainer.getStart());
final T end = row.get(this.dataContainer.getEnd());
final Range range = this.rangeProvider.provideRange(start,end);
final S entry = this.entryCreator.createRelationalUpdatableEntry("",range,
this.dataContainer);
return entry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy