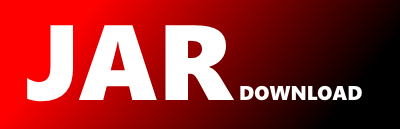
com.xdev.jadoth.collections.XGettingCollection Maven / Gradle / Ivy
/**
*
*/
package com.xdev.jadoth.collections;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import com.xdev.jadoth.Jadoth;
import com.xdev.jadoth.lang.Equalator;
import com.xdev.jadoth.lang.functional.Executing;
import com.xdev.jadoth.lang.functional.Operation;
import com.xdev.jadoth.lang.functional.Predicate;
import com.xdev.jadoth.lang.functional.aggregates.Aggregate;
import com.xdev.jadoth.lang.functional.aggregates.TAggregate;
import com.xdev.jadoth.lang.functional.controlflow.TExecuting;
import com.xdev.jadoth.lang.functional.controlflow.TOperation;
import com.xdev.jadoth.lang.functional.controlflow.TPredicate;
import com.xdev.jadoth.util.VarChar;
/**
* @author TM
*
*/
public interface XGettingCollection
extends
Collection,
Executing>,
TExecuting>
{
// java.util.Collection Query Operations
public int size();
public boolean isEmpty();
/**
* Deprecated {@link Collection} method kept for compatability reasons.
* Alias for {@link #contains(Object, Jadoth.EQUALS)}.
*
* Use the approriate choice between {@link #containsId(Object)} and {@link #contains(Object, Equalator)} instead.
*
* @param o
* @return
*/
@Deprecated
public boolean contains(Object o);
public Iterator iterator();
public Object[] toArray();
public T[] toArray(T[] a);
/**
* Throws an immediate {@link UnsupportedOperationException}.
* @param e
* @return
*/
public boolean add(E e);
/**
* Throws an immediate {@link UnsupportedOperationException}.
* @param o
* @return
*/
public boolean remove(Object o);
// java.util.Collection Bulk Modification Operations
@Deprecated
public boolean containsAll(Collection> c);
/**
* Throws an immediate {@link UnsupportedOperationException}.
* @param c
* @return
*/
public boolean addAll(Collection extends E> c);
/**
* Throws an immediate {@link UnsupportedOperationException}.
* @param index
* @param c
* @return
*/
public boolean addAll(int index, Collection extends E> c);
/**
* Throws an immediate {@link UnsupportedOperationException}.
* @param c
* @return
*/
@Deprecated
public boolean removeAll(Collection> c);
/**
* Throws an immediate {@link UnsupportedOperationException}.
* @param c
* @return
*/
@Deprecated
public boolean retainAll(Collection> c);
/**
* Throws an immediate {@link UnsupportedOperationException}.
*/
public void clear();
// java.util.Collection Comparison and hashing
/**
* Performs an equality comparison according to the specification in {@link Collection}.
*
* Note that it is this interface's author opinion that the whole usage of equals() in standard Java, especially
* in the collection implementations, is deeply flawed.
* The reason is because all kinds of comparison types are mixed up in one method, from identity comparison over
* data indentity comparison to content comparison.
* In order to get the right behaviour in every situation, one has to distinct between those three different types
* (and maybe more, but then that's what comparators are for).
*
* This means several things:
* 1.) You can't just say for example an ArrayList is the "same" as a LinkedList just because they contain the
* same content.
* There are different implementations for a good reason, so you have to distinct them when comparing.
* There are simple code examples which create massive misbehaviour that will catastrophically ruin the runtime
* behaviour of a programm due to this error in Java / JDK / SUN / whatever.
* 2.) You can't always determine equality of two collections by determining equality of each element as
* {@link Collection} defines it.
*
* As a conclusion: don't use this method!
* Be clear what type of comparison you really need, then use one of the following methods
* and proper comparators:
* {@link #equals(XGettingCollection, Equalator)}
* {@link #equalsContent(Collection, Equalator)}
*
* {@inheritDoc}
*
* @param o
* @return
*/
@Deprecated
public boolean equals(Object o);
@Deprecated
public int hashCode();
public interface Factory
{
public XGettingCollection createInstance();
}
/**
*
* @param type
* @return
*/
public E[] toArray(Class type);
public E max(Comparator comparator);
public E min(Comparator comparator);
public boolean containsAll(Collection c, boolean ignoreNulls, Equalator equalator);
public boolean containsAll(Collection c, boolean ignoreNulls);
public XGettingCollection execute( Operation operation);
public XGettingCollection execute(TOperation operation);
public XGettingCollection process( Operation operation);
public XGettingCollection process(TOperation operation);
public R aggregate(Aggregate aggregate);
public R aggregate(TAggregate aggregate);
/**
* Actual contains() method renamed to containsId() due to name clash with deprecated method
* {@link #contains(Object)}.
*/
public boolean containsId(E element);
public boolean contains (E element, Equalator equalator);
public boolean contains( Predicate predicate);
public boolean contains(TPredicate predicate);
public boolean applies( Predicate predicate);
public boolean applies(TPredicate predicate);
public int count(E element);
public int count(E element, Equalator equalator);
public int count( Predicate predicate);
public int count(TPredicate predicate);
public > C distinct(C targetCollection);
public > C distinct(C targetCollection, Equalator equalator);
public > C copyTo(C targetCollection, Predicate predicate);
public > C copyTo(C targetCollection, TPredicate predicate);
public > C copyTo(C targetCollection, Predicate predicate, int skip, Integer limit);
public > C copyTo(C targetCollection, TPredicate predicate, int skip, Integer limit);
public boolean hasUniqueValues(boolean ignoreMultipleNulls);
public boolean hasUniqueValues(boolean ignoreMultipleNulls, Equalator comparator);
public E search(E element, Equalator equalator);
public E search( Predicate predicate);
public E search(TPredicate predicate);
/**
* Returns true if the passed list is of the same type as this list and
* this.equalsContent(list, equalator)
yields true.
*
* @param list
* @param equalator
* @return
*/
public boolean equals(XGettingCollection list, Equalator equalator);
/**
* Returns true if all elements of this list and the passed list are sequentially equal as defined
* by the passed equalator.
* @param list the list that this list shall be compared to
* @param equalator the equalator to use to determine the equality of each element
* @return true if this list is equal to the passed list, false otherwise
*/
public boolean equalsContent(Collection list, Equalator comparator);
public void appendTo(VarChar vc);
public void appendTo(VarChar vc, char seperator);
public void appendTo(VarChar vc, String seperator);
public > C union (XGettingCollection other, Equalator comparator, C target);
public > C intersect(XGettingCollection other, Equalator comparator, C target);
public > C except (XGettingCollection other, Equalator comparator, C target);
}