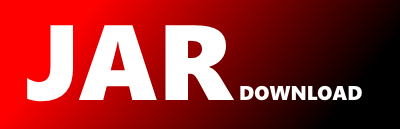
com.xdev.jadoth.collections.XSettingCollection Maven / Gradle / Ivy
/**
*
*/
package com.xdev.jadoth.collections;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Map;
import com.xdev.jadoth.lang.Equalator;
import com.xdev.jadoth.lang.functional.Operation;
import com.xdev.jadoth.lang.functional.Predicate;
import com.xdev.jadoth.lang.functional.controlflow.TOperation;
import com.xdev.jadoth.lang.functional.controlflow.TPredicate;
/**
* @author Thomas Muenz
*
*/
public interface XSettingCollection extends XGettingCollection
{
public interface Factory extends XGettingCollection.Factory
{
@Override
public XSettingCollection createInstance();
}
public XSettingCollection execute(Operation operation);
public XSettingCollection execute(TOperation operation);
public XSettingCollection process(Operation operation);
public XSettingCollection process(TOperation operation);
/**
*
* @param oldElement
* @param newElement
* @return a value greater than or equal to 0 if an element has been found and replaced, a negative value otherwise.
*/
public int replaceOne(E oldElement, E newElement);
/**
*
* @param oldElement
* @param newElement
* @param equalator
* @return a value greater than or equal to 0 if an element has been found and replaced, a negative value otherwise.
*/
public int replaceOne(E oldElement, E newElement, Equalator equalator);
/**
*
* @param oldElement
* @param newElement
* @return the amount of replacements that has been executed by the call of this method.
*/
public int replace(E oldElement, E newElement);
/**
*
* @param oldElement
* @param newElement
* @param equalator
* @return the amount of replacements that has been executed by the call of this method.
*/
public int replace(E oldElement, E newElement, Equalator equalator);
/**
*
* @param oldElement
* @param newElement
* @param limit
* @return the amount of replacements that has been executed by the call of this method.
*/
public int replace(E oldElement, E newElement, int skip, Integer limit);
/**
*
* @param oldElement
* @param newElement
* @param limit
* @param equalator
* @return the amount of replacements that has been executed by the call of this method.
*/
public int replace(E oldElement, E newElement, int skip, Integer limit, Equalator equalator);
//ignores null values in multiples
/**
*
* @param replacementMapping
* @return the amount of replacements that has been executed by the call of this method.
*/
public int replaceAll(Map replacementMapping);
public int replaceAll(E[] oldElements, E newElement);
public int replaceAll(E[] oldElements, E newElement, Equalator equalator);
public int replaceAll(XGettingCollection oldElements, E newElement);
public int replaceAll(XGettingCollection oldElements, E newElement, Equalator equalator);
public int replaceAll(E[] oldElements, E newElement, int skip, Integer limit);
public int replaceAll(E[] oldElements, E newElement, int skip, Integer limit, Equalator equalator);
public int replaceAll(XGettingCollection oldElements, E newElement, int skip, Integer limit);
public int replaceAll(XGettingCollection oldElements, E newElement, int skip, Integer limit, Equalator equalator);
public int replaceOne( Predicate predicate, E newElement);
public int replaceOne(TPredicate predicate, E newElement);
public int replace( Predicate predicate, E newElement);
public int replace(TPredicate predicate, E newElement);
public int replace( Predicate predicate, E newElement, int skip, Integer limit);
public int replace(TPredicate predicate, E newElement, int skip, Integer limit);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy