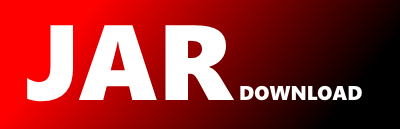
com.xdev.jadoth.cql.CQL Maven / Gradle / Ivy
/**
*
*/
package com.xdev.jadoth.cql;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Comparator;
import com.xdev.jadoth.Jadoth;
import com.xdev.jadoth.collections.Collecting;
import com.xdev.jadoth.collections.GrowList;
import com.xdev.jadoth.collections.Sortable;
import com.xdev.jadoth.collections.XGettingCollection;
import com.xdev.jadoth.lang.ComparatorSequence;
import com.xdev.jadoth.lang.Equalator;
import com.xdev.jadoth.lang.EqualatorSequence;
import com.xdev.jadoth.lang.functional.Condition;
import com.xdev.jadoth.lang.functional.Predicate;
/**
* Collection Query Language
* @author Thomas Muenz
*
*/
public class CQL
{
public static final CqlProjector.Implementation defaultProjection(
final int initialCapactity,
final Class type
)
{
return new CqlProjector.Implementation(initialCapactity);
}
// (28.09.2010)TODO use VarList instead of GrowList with trivial index when completed
public static final GrowList selectFrom(
final XGettingCollection data,
final Predicate predicate
)
{
return data.copyTo(new GrowList(), predicate);
}
public static final GrowList selectFrom(
final XGettingCollection data,
final Predicate predicate,
final int skip,
final int limit
)
{
return data.copyTo(new GrowList(), predicate, skip, limit);
}
@SuppressWarnings("unchecked")
public static final > P select(
final CqlProjector projection,
final XGettingCollection dataSource,
final Predicate condition,
final Comparator sorting,
final int skip,
final int limit
)
{
// nifty & naughty way to optimize storage behaviour without additional overloaded methods
final C storage = projection.provideCollector();
// only apply sorting if result is actually sortable
final C result = dataSource.copyTo(storage, condition, skip, limit);
if(result instanceof Sortable>){
((Sortable)result).sort(sorting);
}
// apply projection and return projection result
return projection.project(result);
}
/**
*
* @param
* @param data
* @param condition
* @param distinction
* @param sorting
* @param limit
* @return
*/
public static final > P select(
final CqlProjector projection,
final XGettingCollection dataSource,
final Predicate condition,
final Equalator distinction,
final Comparator sorting,
final int skip,
final int limit
)
{
return select(
projection,
dataSource,
extendConditionForAggregating(projection, condition, distinction),
sorting,
skip,
limit
);
}
private static Predicate extendConditionForAggregating(
final CqlProjector projection,
final Predicate condition,
final Equalator distinction
)
{
final Predicate aggregationExtendedCondition;
if(projection instanceof CqlAggregator, ?, ?>){
final CqlAggregator aggregator = (CqlAggregator)projection;
aggregationExtendedCondition = new Predicate() {
@Override
public boolean apply(final E e)
{
return condition.apply(e) && aggregator.aggregate(e, distinction);
}
};
}
else {
// (28.09.2010)XXX: replace GrowList with Identity XSet when completed
final GrowList keys = new GrowList();
aggregationExtendedCondition = new Predicate() {
@Override
public boolean apply(final E t)
{
return condition.apply(t) && keys.contains(t, distinction);
}
};
}
return aggregationExtendedCondition;
}
public static XGettingCollection from(final T... data)
{
return Jadoth.list(data);
}
/**
* Dummy method for SQL-style syntax.
* @param
* @param dataSource
* @return
*/
public static XGettingCollection from(final XGettingCollection dataSource)
{
return dataSource;
}
/**
* Dummy method for SQL-style syntax.
* @param limit
* @return
*/
public static int fetchFirst(final int limit)
{
return limit;
}
/**
* Dummy method for SQL-style syntax.
* @param skip
* @return
*/
public static int skip(final int skip)
{
return skip;
}
/**
* sql-like alias for {@link #condition(Predicate)}
* @param
* @param predicate
* @return
*/
public static final Condition where(final Predicate predicate)
{
return new Condition(){
@Override public boolean apply(final T t)
{
return predicate.apply(t);
}
};
}
public static final Condition or(final Predicate predicate1, final Predicate predicate2)
{
return new Condition(){
@Override public boolean apply(final T t)
{
return predicate1.apply(t) || predicate2.apply(t);
}
};
}
public static final Condition and(final Predicate predicate1, final Predicate predicate2)
{
return new Condition(){
@Override public boolean apply(final T t)
{
return predicate1.apply(t) && predicate2.apply(t);
}
};
}
/**
* sql-like alias for {@link #condition(Predicate)}
* @param
* @param predicate
* @return
*/
public static final Comparator orderBy(final Comparator... sortings)
{
return new ComparatorSequence(sortings);
}
/**
* SQL-style syntax dummy method for using the passed comparator itself.
* @param
* @param comparator the comparator to use as itself [:-)].
* @return the passed comparator itself.
*/
public static final Comparator asc(final Comparator comparator)
{
return comparator;
}
/**
* SQL-style syntax dummy method for inverting the passed comparator.
* See {@link #invert(Comparator)}.
* @param
* @param comparator the comparator whose order shall be inverted.
* @return a {@link Comparator} instance that inverts the passed comparator's results.
*/
public static final Comparator desc(final Comparator comparator)
{
return new Comparator() {
@Override
public int compare(final T o1, final T o2)
{
return comparator.compare(o1, o2) * (-1);
}
};
}
/**
* Inverts the result of the passed comparator by multiplying it with (-1), effectively inverting the order
* it defines.
*
* @param
* @param comparator the comparator whose order shall be inverted.
* @return a {@link Comparator} instance that inverts the passed comparator's results.
*/
public static final Comparator invert(final Comparator comparator)
{
return new Comparator() {
@Override
public int compare(final T o1, final T o2)
{
return comparator.compare(o1, o2) * (-1);
}
};
}
// (28.09.2010)TODO NULLS FIRST / LAST ?
public static final Equalator groupBy(final Equalator... distinction)
{
return new EqualatorSequence(distinction);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy