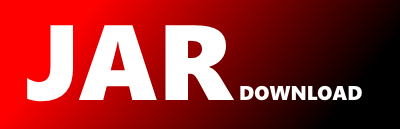
com.xdev.jadoth.sqlengine.dbms.DbmsSyntax Maven / Gradle / Ivy
/**
*
*/
package com.xdev.jadoth.sqlengine.dbms;
/*-
* #%L
* XDEV Application Framework
* %%
* Copyright (C) 2003 - 2020 XDEV Software
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import static com.xdev.jadoth.Jadoth.mergeInto;
import java.util.HashMap;
import java.util.Iterator;
/**
* @author Thomas Muenz
*
*/
public interface DbmsSyntax> extends Iterable
{
/**
* Note that reserved word
and keyword
are different types of words.
* According to SQL Standard, a keyword
is either a reserved word
* or a non-reserved word
.
*
* Note: non-reserved word
does not mean "not a reserved word" but describes another list of words
* in the SQL Standard. Not sure yet where the difference is.
*
* @param s
* @return
*/
public boolean isKeyword(String s);
public boolean isNormalizedKeyword(String s);
public boolean isKeyword(String s, boolean normalize);
public Iterable iterateKeywords();
public boolean isReservedWord(String s);
public boolean isNormalizedReservedWord(String s);
public boolean isReservedWord(String s, boolean normalize);
public Iterable iterateReservedWords();
public boolean isNonReservedWord(String s);
public boolean isNormalizedNonReservedWord(String s);
public boolean isNonReservedWord(String s, boolean normalize);
public Iterable iterateNonReservedWords();
public abstract class Implementation> implements DbmsSyntax
{
///////////////////////////////////////////////////////////////////////////
// static methods //
///////////////////
/**
*
* @param words
* @return
*/
public static final HashMap wordSet(final String... words)
{
if(words == null) return new HashMap(0);
final HashMap map = new HashMap(words.length*20);
for(final String word : words) {
map.put(word, null);
}
return map;
}
/**
*
* @param reservedWords
* @param nonReservedWords
* @return
*/
@SuppressWarnings("unchecked")
public static final HashMap createKeywordSet(
final HashMap reservedWords, final HashMap nonReservedWords
)
{
if(reservedWords == null){
if(nonReservedWords == null){
return new HashMap(0);
}
return nonReservedWords;
}
else if(nonReservedWords == null){
return reservedWords;
}
return mergeInto(
new HashMap((reservedWords.size()+nonReservedWords.size())*2),
reservedWords, nonReservedWords
);
}
///////////////////////////////////////////////////////////////////////////
// instance fields //
////////////////////
private final HashMap reservedWords;
private final HashMap nonReservedWords;
private final HashMap keywords;
///////////////////////////////////////////////////////////////////////////
// constructors //
/////////////////
/**
* @param reservedWords
* @param nonReservedWords
* @param keywords
*/
protected Implementation(
final HashMap reservedWords,
final HashMap nonReservedWords
)
{
super();
this.reservedWords = reservedWords != null ?reservedWords :new HashMap(0);
this.nonReservedWords = nonReservedWords != null ?nonReservedWords :new HashMap(0);
this.keywords = createKeywordSet(reservedWords, nonReservedWords);
}
///////////////////////////////////////////////////////////////////////////
// override methods //
/////////////////////
/**
* Checks case sensitive for performance reasons.
* See isKeyword(String, boolean)
for checking case insensitive.
*
* @param s
* @return
*/
@Override
public boolean isKeyword(final String s)
{
return keywords.containsKey(s.toUpperCase());
}
/**
* @param s
* @param normalize
* @return
*/
@Override
public boolean isKeyword(final String s, final boolean normalize)
{
return keywords.containsKey(normalize ?s.toUpperCase() :s);
}
/**
* @param s
* @return
*/
@Override
public boolean isNonReservedWord(final String s)
{
return nonReservedWords.containsKey(s.toUpperCase());
}
/**
* @param s
* @param normalize
* @return
*/
@Override
public boolean isNonReservedWord(final String s, final boolean normalize)
{
return nonReservedWords.containsKey(normalize ?s.toUpperCase() :s);
}
/**
* @param s
* @return
*/
@Override
public boolean isReservedWord(final String s)
{
return reservedWords.containsKey(s.toUpperCase());
}
/**
* @param s
* @param normalize
* @return
*/
@Override
public boolean isReservedWord(final String s, final boolean normalize)
{
return reservedWords.containsKey(normalize ?s.toUpperCase() :s);
}
/**
* @param s
* @return
*/
@Override
public boolean isNormalizedKeyword(String s)
{
return this.keywords.containsKey(s);
}
/**
* @param s
* @return
*/
@Override
public boolean isNormalizedNonReservedWord(final String s)
{
return this.nonReservedWords.containsKey(s);
}
/**
* @param s
* @return
*/
@Override
public boolean isNormalizedReservedWord(String s)
{
return this.reservedWords.containsKey(s);
}
/**
* @return
*/
@Override
public Iterable iterateKeywords()
{
return keywords.keySet();
}
/**
* @return
*/
@Override
public Iterable iterateNonReservedWords()
{
return reservedWords.keySet();
}
/**
* @return
*/
@Override
public Iterable iterateReservedWords()
{
return reservedWords.keySet();
}
/**
* @return
*/
@Override
public Iterator iterator()
{
return this.keywords.keySet().iterator();
}
}
}