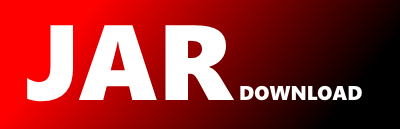
com.xdev.jadoth.collections.Snake Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package com.xdev.jadoth.collections;
import com.xdev.jadoth.util.VarChar;
/**
* @author Thomas Muenz
*
*/
public interface Snake //extends VarChar.Appendable
{
E value();
Snake tail();
Snake head();
Snake next();
Snake prev();
boolean hasNext();
boolean hasPrev();
Snake hop(int index);
Snake add(E value);
E set(E value);
class Implementation implements Snake
{
///////////////////////////////////////////////////////////////////////////
// instance fields //
/////////////////////
Object value;
Snake.Implementation next = null;
Snake.Implementation prev = null;
///////////////////////////////////////////////////////////////////////////
// constructors //
/////////////////////
public Implementation()
{
super();
this.value = null;
}
public Implementation(final Object value)
{
super();
this.value = value;
}
/**
* @return
* @see com.xdev.jadoth.collections.Snake#head()
*/
@Override
public Snake head()
{
Snake.Implementation link = this;
while(link.next != null){
link = link.next;
}
return link;
}
/**
* @param index
* @return
* @see com.xdev.jadoth.collections.Snake#hop(int)
*/
@Override
public Snake hop(int index)
{
Snake.Implementation link = this;
if(index < 0){
while(index ++> 0){
link = link.prev;
}
}
else {
while(index --> 0){
link = link.next;
}
}
return link;
}
/**
* @return
* @see com.xdev.jadoth.collections.Snake#tail()
*/
@Override
public Snake tail()
{
Snake.Implementation link = this;
while(link.prev != null){
link = link.prev;
}
return link;
}
/**
* @return
* @see com.xdev.jadoth.collections.Snake#value()
*/
@SuppressWarnings("unchecked")
@Override
public E value()
{
return (E)this.value;
}
/**
* @return
* @see com.xdev.jadoth.collections.Snake#next()
*/
@Override
public Snake next()
{
return this.next();
}
/**
* @return
* @see com.xdev.jadoth.collections.Snake#prev()
*/
@Override
public Snake prev()
{
return this.prev();
}
/**
* @param value
* @return
* @see com.xdev.jadoth.collections.Snake#add(java.lang.Object)
*/
@Override
public Snake add(final E value)
{
final Snake.Implementation newLink = new Snake.Implementation();
newLink.value = value;
newLink.prev = this;
this.next = newLink;
return newLink;
}
/**
* @return
* @see com.xdev.jadoth.collections.Snake#hasNext()
*/
@Override
public boolean hasNext()
{
return this.next != null;
}
/**
* @return
* @see com.xdev.jadoth.collections.Snake#hasPrev()
*/
@Override
public boolean hasPrev()
{
return this.prev != null;
}
/**
* @param value
* @return
* @see com.xdev.jadoth.collections.Snake#set(java.lang.Object)
*/
@Override
public E set(final E value)
{
final E oldValue = (E)this.value;
this.value = value;
return oldValue;
}
/**
* @return
* @see java.lang.Object#toString()
*/
@Override
public String toString()
{
if(this.next == null){
//head
final VarChar vc = new VarChar().append('Ö');
Snake.Implementation link = this;
do {
vc.append('(').append(link.value).append(')');
}
while((link = link.prev) != null);
return vc.append('>').toString();
}
if(this.prev == null){
//tail
final VarChar vc = new VarChar().append('<');
Snake.Implementation link = this;
do {
vc.append('(').append(link.value).append(')');
}
while((link = link.next) != null);
return vc.append('Ö').toString();
}
return String.valueOf(this.value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy