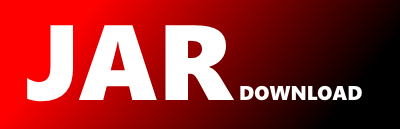
com.xdev.jadoth.collections.XAddingCollection Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
/**
*
*/
package com.xdev.jadoth.collections;
import java.util.Collection;
import com.xdev.jadoth.lang.functional.Predicate;
import com.xdev.jadoth.lang.functional.controlflow.TPredicate;
/**
* @author Thomas Muenz
*
*/
public interface XAddingCollection extends XGettingCollection, Collecting
{
public interface Factory extends XGettingCollection.Factory
{
@Override
public XAddingCollection createInstance();
}
// reasonable methods adapted from java.util.Collection. Provide modification information instead of chaining.
public boolean add(E e);
public boolean addAll(Collection extends E> c);
public XAddingCollection add(E... elements);
public XAddingCollection add(Iterable extends E> elements);
/**
* Adds all of the srcLength
elements in array elements
at srcIndex
for which
* predicate
applies to this collection up to a maximum of limit
elements.
* The first skip
elements to which predicate
applies are skipped. Skipped elements
* to NOT count towards limit.
*
* Additional information on parameters:
*
* elements
may not be null.
* srcIndex
may not exceed elements
's bounds.
* srcLength
may be negative, causing the elements to be added in reversed order.
* predicate
may not be null.
* skip
may not be negative.
* limit
may be null to indicate that no limiting shall be applied.
* limit
may be equal to or less than 0, resulting in an immediate return of this method.
*
*
* @param elements the source array containing the elements to be added.
* @param srcIndex the source array index of the first element to be (potentially) added.
* @param srcLength the amount of elements to be (potentially) added.
* @param predicate the predicate to evaluate if an element shall be added. May not be null.
* @param skip the number of positively evaluated elements to skip.
* @param limit the maximum number of elements to be actually added. A value of null indicates no limit.
* @return this collection
* @throws ArrayIndexOutOfBoundsException if the values for srcIndex
and srcLength
or
* their combination exceed elements
's bounds.
*/
public XAddingCollection add(E[] elements, int srcIndex, int srcLength, Predicate predicate, int skip, Integer limit);
/**
* Adds all of the srcLength
elements in array elements
at srcIndex
for which
* predicate
applies to this collection up to a maximum of limit
elements.
* The first skip
elements to which predicate
applies are skipped. Skipped elements
* to NOT count towards limit.
*
* Additional information on parameters:
*
* elements
may not be null.
* srcIndex
may not exceed elements
's bounds.
* srcLength
may be negative, causing the elements to be added in reversed order.
* predicate
may not be null.
* skip
may not be negative.
* limit
may be null to indicate that no limiting shall be applied.
* limit
may be equal to or less than 0, resulting in an immediate return of this method.
*
*
* @param elements the source array containing the elements to be added.
* @param srcIndex the source array index of the first element to be (potentially) added.
* @param srcLength the amount of elements to be (potentially) added.
* @param predicate the predicate to evaluate if an element shall be added. May not be null.
* @param skip the number of positively evaluated elements to skip.
* @param limit the maximum number of elements to be actually added. A value of null indicates no limit.
* @return this collection
* @throws ArrayIndexOutOfBoundsException if the values for srcIndex
and srcLength
or
* their combination exceed elements
's bounds.
*/
public XAddingCollection add(E[] elements, int srcIndex, int srcLength, TPredicate predicate, int skip, Integer limit);
public XAddingCollection add(Iterable extends E> elements, int srcOffset, Integer srcLength, Predicate predicate, int skip, Integer limit);
public XAddingCollection add(Iterable extends E> elements, int srcOffset, Integer srcLength, TPredicate predicate, int skip, Integer limit);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy