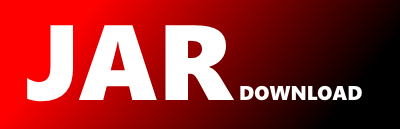
com.xdev.jadoth.collections.XRemovingCollection Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
/**
*
*/
package com.xdev.jadoth.collections;
import com.xdev.jadoth.lang.Equalator;
import com.xdev.jadoth.lang.functional.Operation;
import com.xdev.jadoth.lang.functional.Predicate;
import com.xdev.jadoth.lang.functional.Processable;
import com.xdev.jadoth.lang.functional.controlflow.TOperation;
import com.xdev.jadoth.lang.functional.controlflow.TPredicate;
import com.xdev.jadoth.lang.functional.controlflow.TProcessable;
/**
* @author Thomas Muenz
*
*/
public interface XRemovingCollection
extends
XGettingCollection,
Processable>,
TProcessable>
{
public interface Factory extends XGettingCollection.Factory
{
@Override
public XRemovingCollection createInstance();
}
public XRemovingCollection execute( Operation operation);
public XRemovingCollection execute(TOperation operation);
public XRemovingCollection process( Operation operation);
public XRemovingCollection process(TOperation operation);
public void clear();
public E removeOne(E element);
public E removeOne(E element, Equalator equalator);
public int removeId(E element);
public int remove (E element, Equalator equalator);
public int removeId(E element, int skip, Integer limit);
public int remove (E element, int skip, Integer limit, Equalator equalator);
public int removeAllId(XCollection c, boolean ignoreNulls);
public int removeAll (XCollection c, boolean ignoreNulls, Equalator equalator);
public int retainAllId(XCollection c);
public int retainAll (XCollection c, Equalator equalator);
public int removeDuplicates(boolean ignoreNulls);
public int removeDuplicates(boolean ignoreNulls, Equalator equalator);
// intentionally no removeDuplicates(..., skip, limit) as this wouldn't make much sense
public int reduce( Predicate predicate);
public int reduce(TPredicate predicate);
public int reduce( Predicate predicate, int skip, Integer limit);
public int reduce(TPredicate predicate, int skip, Integer limit);
public > C moveTo(C target, Predicate predicate);
public > C moveTo(C target, TPredicate predicate);
public > C moveTo(C target, Predicate predicate, int skip, Integer limit);
public > C moveTo(C target, TPredicate predicate, int skip, Integer limit);
/**
* Clears (and reinitializes if needed) this collection in the fastest possible way, i.e. by allocating a new and
* empty internal storage. The collection will be empty after calling this method.
* @return this collection
*/
public XRemovingCollection truncate();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy