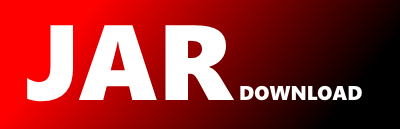
com.xdev.jadoth.lang.functional.iterables.ChainedIterables Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package com.xdev.jadoth.lang.functional.iterables;
import java.util.ArrayList;
import java.util.Iterator;
/**
* @author Thomas Muenz
*
*/
public class ChainedIterables implements Iterable
{
private final ArrayList> iterables;
public ChainedIterables(final Iterable... iterables)
{
super();
final ArrayList> set = new ArrayList>(iterables.length);
this.iterables = set;
if(iterables == null) return;
for(final Iterable iterable : iterables) {
if(iterable == null) continue;
set.add(iterable);
}
}
/**
* @return
* @see java.lang.Iterable#iterator()
*/
@Override
public Iterator iterator()
{
return new ChainedIterator();
}
public Iterable add(final Iterable iterable)
{
this.iterables.add(iterable);
return this;
}
public ChainedIterables add(final Iterable... iterables)
{
for(final Iterable iterable : iterables) {
this.iterables.add(iterable);
}
return this;
}
public Iterable remove(final Iterable iterable)
{
this.iterables.remove(iterable);
return iterable;
}
public int remove(final Iterable... iterables)
{
int removedCount = 0;
for(final Iterable i : iterables) {
if(this.iterables.remove(i)) {
removedCount++;
}
}
return removedCount;
}
public boolean contains(final Iterable iterable)
{
return this.iterables.contains(iterable);
}
protected class ChainedIterator implements Iterator
{
///////////////////////////////////////////////////////////////////////////
// instance fields //
/////////////////////
// private Iterator> iterablesIterator; //o_0\\
private Iterator currentIterator = null;
private int currentIndex = 0;
///////////////////////////////////////////////////////////////////////////
// constructors //
/////////////////////
private ChainedIterator()
{
super();
this.nextIterator();
}
/**
* @return
* @see java.util.Iterator#hasNext()
*/
@Override
public boolean hasNext()
{
if(this.currentIterator.hasNext()) return true;
while(this.nextIterator()){
if(this.currentIterator.hasNext()) return true;
}
return false;
}
/**
* @return
* @see java.util.Iterator#next()
*/
@Override
public T next()
{
return this.currentIterator.next();
}
protected boolean nextIterator()
{
final ArrayList> iterables = ChainedIterables.this.iterables;
Iterable loopIterable = null;
//the loops skips null elements until the first existing iterable is encountered
while(loopIterable == null && this.currentIndex < iterables.size()){
loopIterable = iterables.get(this.currentIndex++);
}
//if either currentIndex was already at the end or loop scrolled to the end, there are no more iterables
if(loopIterable == null) return false;
//otherwise, the next iterable has been found.
this.currentIterator = loopIterable.iterator();
return true;
}
/**
*
* @see java.util.Iterator#remove()
*/
@Override
public void remove()
{
this.currentIterator.remove();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy