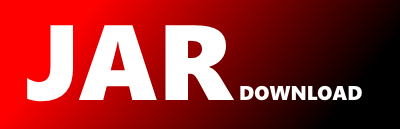
com.xdev.jadoth.lang.reference.LinkReference Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package com.xdev.jadoth.lang.reference;
import java.util.Iterator;
/**
* @author Thomas Muenz
*
*/
public interface LinkReference extends LinkingReference
{
public LinkReference next();
/**
* Sets linkedReference
as this LinkedReference
object's
* linked LinkedReference
object.
*
* Note that the so far linked LinkedReference
object is returned, not this object itself!
* @param linkedReference
* @return
*/
public LinkReference setNext(LinkReference linkedReference);
/**
* Sets linkedReference
as this LinkedReference
object's
* linked LinkedReference
object.
*
* Note that the reference is returned, not this object itself!
* @param linkedReference
* @return the linked LinkedReference
object (NOT this!)
*/
public LinkReference link(LinkReference linkedReference);
/**
* Alias for link(new LinkedReference(nextRef))
.
* @param nextRef
* @return
*/
public LinkReference link(T nextRef);
public LinkReference insert(LinkReference linkedReference);
public LinkReference removeNext();
public class Implementation extends Reference.Implementation implements LinkReference
{
private LinkReference next = null;
/**
* @param ref
*/
public Implementation(final T ref)
{
super(ref);
}
/**
* @return
*/
@Override
public LinkReference next()
{
return this.next;
}
/**
* @return
*/
@Override
public boolean hasNext()
{
return this.next != null;
}
/**
* @param linkedReference
* @return
*/
@Override
public boolean isNext(final LinkingReferencing linkedReference)
{
return this.next == linkedReference;
}
/**
* @param linkedReference
* @return
*/
@Override
public LinkReference link(final LinkReference linkedReference)
{
this.next = linkedReference;
return linkedReference;
}
/**
* @param linkedReference
* @return
*/
@Override
public LinkReference setNext(final LinkReference linkedReference)
{
final LinkReference old = this.next;
this.next = linkedReference;
return old;
}
/**
* @param nextRef
* @return
*/
@Override
public LinkReference link(final T nextRef)
{
return this.link(new LinkReference.Implementation(nextRef));
}
/**
* @param linkedReference
* @return
* @throws NullPointer, if linkedReference
is null.
*/
@Override
public LinkReference insert(final LinkReference linkedReference)
{
final LinkReference next = this.next;
this.next = linkedReference;
if(next != null){
linkedReference.setNext(next); //provoke NullPointer for argument
}
return this;
}
/**
* @return
*/
@Override
public LinkReference removeNext()
{
final LinkReference next = this.next;
this.next = next==null ?null :next.next();
return next;
}
/**
* @return
*/
@Override
public Object[] toArray()
{
LinkReference loopNext = this;
int i = 1;
while((loopNext = loopNext.next()) != null){
i++; //this is presumable faster than using an ArrayList or LinkedList for collection
}
final Object[] array = new Object[i];
loopNext = this;
i = 0;
do{
array[i++] = loopNext.get();
}
while((loopNext = loopNext.next()) != null);
return array;
}
/**
* @return
*/
@Override
public Iterator iterator()
{
return new ChainIterator(this);
}
/**
* @return
* @see java.lang.Object#toString()
*/
@Override
public String toString()
{
final String e = String.valueOf(this.get());
final StringBuilder sb = new StringBuilder(e.length()+3);
return sb.append('(').append(e).append(')').append(this.hasNext() ?'-' :'x').toString();
}
/**
* @return
* @see com.xdev.jadoth.lang.reference.LinkingReferencing#toChainString()
*/
@Override
public String toChainString()
{
final StringBuilder sb = new StringBuilder(1024);
sb.append('(').append(this.get()).append(')');
for(LinkingReferencing r = this.next; r != null; r = r.next()){
sb.append('-').append('(').append(r.get()).append(')');
}
return sb.toString();
}
}
final class ChainIterator implements Iterator
{
private LinkReference current;
/**
* @param current
*/
private ChainIterator(final LinkReference current)
{
super();
this.current = current;
}
@Override
public boolean hasNext()
{
return current.next() != null;
}
@Override
public T next()
{
final LinkReference currentCurrent = this.current;
this.current = currentCurrent.next();
return currentCurrent.get();
}
@Override
public void remove() throws UnsupportedOperationException
{
throw new UnsupportedOperationException(
"Can't remove current element in a one directional chain"
);
}
}
}