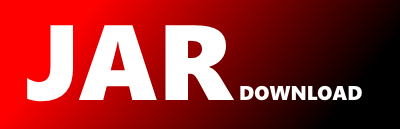
xdev.db.AbstractDBDataSource Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package xdev.db;
import java.util.List;
import java.util.Random;
import java.util.Vector;
import xdev.db.event.DBDataSourceEvent;
import xdev.db.event.DBDataSourceListener;
import xdev.util.AbstractDataSource;
import xdev.util.auth.Password;
/**
* Abstract base class for all {@link DBDataSource}s including the handling of
* common {@link xdev.util.DataSource.Parameter}s and
* {@link DBDataSourceListener}s.
*
* @see DBDataSource
* @see AbstractDataSource
*
* @author XDEV Software
*
*/
public abstract class AbstractDBDataSource> extends AbstractDataSource
implements DBDataSource
{
/**
* @since 3.1
*/
public final static Parameter EMBEDDED;
public final static Parameter HOST;
public final static Parameter PORT;
public final static Parameter USERNAME;
public final static Parameter PASSWORD;
public final static Parameter CATALOG;
public final static Parameter SCHEMA;
/**
* @since 4.0
*/
public final static Parameter URL_EXTENSION;
public final static Parameter CONNECTION_POOL_SIZE;
public final static Parameter KEEP_CONNECTIONS_ALIVE;
public final static Parameter IS_SERVER_DATASOURCE;
public final static Parameter SERVER_URL;
public final static Parameter AUTH_KEY;
public final static Parameter VERBOSE;
static
{
EMBEDDED = new Parameter("embedded",false);
HOST = new Parameter("host","localhost");
PORT = new Parameter("port",0);
USERNAME = new Parameter("username","");
PASSWORD = new Parameter("password",new Password(""));
CATALOG = new Parameter("catalog","");
SCHEMA = new Parameter("schema","");
URL_EXTENSION = new Parameter("urlExtension","");
CONNECTION_POOL_SIZE = new Parameter("connectionPoolSize",-1);
KEEP_CONNECTIONS_ALIVE = new Parameter("keepConnectionsAlive",true);
IS_SERVER_DATASOURCE = new Parameter("isServerDataSource",false);
SERVER_URL = new Parameter("serverURL","http://localhost:8080/servlet/");
AUTH_KEY = new Parameter("authKey",Long.toHexString(System.nanoTime()).toUpperCase()
.concat(Long.toHexString(new Random().nextLong()).toUpperCase()));
VERBOSE = new Parameter("verbose",false);
rankParams(EMBEDDED,HOST,PORT,USERNAME,PASSWORD,CATALOG,SCHEMA,URL_EXTENSION,
CONNECTION_POOL_SIZE,KEEP_CONNECTIONS_ALIVE,IS_SERVER_DATASOURCE,SERVER_URL,
AUTH_KEY,VERBOSE);
}
protected List listeners = new Vector();
/**
* {@inheritDoc}
*/
@Override
public void addDBDataSourceListener(DBDataSourceListener l)
{
listeners.add(l);
}
/**
* {@inheritDoc}
*/
@Override
public void removeDBDataSourceListener(DBDataSourceListener l)
{
listeners.remove(l);
}
/**
* {@inheritDoc}
*/
@Override
public DBDataSourceListener[] getDBDataSourceListeners()
{
return listeners.toArray(new DBDataSourceListener[listeners.size()]);
}
/**
* Returns the value of the {@link #EMBEDDED} parameter.
*
* @return true
if embedded is set, false
* otherwise
* @since 3.1
*/
public boolean isEmbedded()
{
return getParameterValue(EMBEDDED);
}
/**
* Returns the host of this {@link AbstractDBDataSource}.
*
* @return the host of this {@link AbstractDBDataSource}
*/
public String getHost()
{
return getParameterValue(HOST);
}
/**
* Returns the port of this {@link AbstractDBDataSource}.
*
* @return the port of this {@link AbstractDBDataSource}
*/
public int getPort()
{
return getParameterValue(PORT);
}
/**
* Returns the user name of this {@link AbstractDBDataSource}.
*
* @return the user name of this {@link AbstractDBDataSource}
*/
public String getUserName()
{
return getParameterValue(USERNAME);
}
/**
* Returns the password of this {@link AbstractDBDataSource}.
*
* @return the password of this {@link AbstractDBDataSource}
*/
public Password getPassword()
{
return getParameterValue(PASSWORD);
}
/**
* Returns the catalog of this {@link AbstractDBDataSource}.
*
* @return the catalog of this {@link AbstractDBDataSource}
*/
public String getCatalog()
{
return getParameterValue(CATALOG);
}
/**
* Returns the schema of this {@link AbstractDBDataSource}.
*
* @return the schema of this {@link AbstractDBDataSource}
*/
public String getSchema()
{
return getParameterValue(SCHEMA);
}
public String getUrlExtension()
{
return getParameterValue(URL_EXTENSION);
}
public void setUrlExtension(String urlExtension)
{
getParameter(URL_EXTENSION).setValue(urlExtension);
}
/**
* Returns the connection pool size of this {@link AbstractDBDataSource}.
*
* @return the connection pool size of this {@link AbstractDBDataSource}
*/
public int getConnectionPoolSize()
{
return getParameterValue(CONNECTION_POOL_SIZE);
}
/**
* Returns true
if the connection of this
* {@link AbstractDataSource} is alive.
*
* @return true
if the connection of this
* {@link AbstractDataSource} is alive, otherwise false
* .
*/
public boolean keepConnectionsAlive()
{
return getParameterValue(KEEP_CONNECTIONS_ALIVE);
}
/**
* Determines if this data source is a distibuted client-server data source.
*
* @return true
if this data source is a distibuted
* client-server data source, false
otherwise
*/
public boolean isServerDataSource()
{
return getParameterValue(IS_SERVER_DATASOURCE);
}
/**
* Returns the password of this {@link AbstractDBDataSource}.
*
* @return the password of this {@link AbstractDBDataSource}
*/
public String getServerURL()
{
return getParameterValue(SERVER_URL);
}
/**
* Returns the authentification key of this {@link AbstractDBDataSource}.
*
* @return the authentification key of this {@link AbstractDBDataSource}
*/
public String getAuthKey()
{
return getParameterValue(AUTH_KEY);
}
/**
* Sets the key which is used to authentificate this client to communicate
* with the servlet-part of this data source.
*
* @param authKey
* the new authorization key
*/
public void setAuthKey(String authKey)
{
getParameter(AUTH_KEY).setValue(authKey);
}
/**
* {@inheritDoc}
*/
@Override
public boolean isParameterRequired(Parameter p)
{
if(SERVER_URL.equals(p) || AUTH_KEY.equals(p))
{
return isServerDataSource() && !isEmbedded();
}
else if(HOST.equals(p) || PORT.equals(p) || CONNECTION_POOL_SIZE.equals(p)
|| KEEP_CONNECTIONS_ALIVE.equals(p) || IS_SERVER_DATASOURCE.equals(p))
{
return !isEmbedded();
}
return super.isParameterRequired(p);
}
@Override
public final DBConnection openConnection() throws DBException
{
DBConnection con = openConnectionImpl();
if(listeners.size() > 0)
{
DBDataSourceEvent event = new DBDataSourceEvent(this,con);
for(DBDataSourceListener listener : getDBDataSourceListeners())
{
listener.connectionOpened(event);
}
}
return con;
}
protected abstract DBConnection openConnectionImpl() throws DBException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy