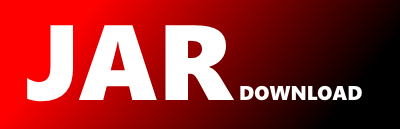
xdev.db.Result Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package xdev.db;
import java.io.PrintStream;
/**
* A table of data representing a database result set, which is usually
* generated by executing a statement that queries the database.
*
* A Result object maintains a cursor pointing to its current row of data.
* Initially the cursor is positioned before the first row. The {@link #next()}
* method moves the cursor to the next row, and because it returns false when
* there are no more rows in the Result object, it can be used in a while loop
* to iterate through the result set.
*
* The Result interface provides getter methods ({@link #getInt(int)},
* {@link #getString(int)} , and so on) for retrieving column values from the
* current row. Values can be retrieved using either the index number of the
* column or the name of the column. In general, using the column index will be
* more efficient. Columns are numbered from 0. For maximum portability, result
* set columns within each row should be read in left-to-right order, and each
* column should be read only once. For the getter methods, a driver attempts to
* convert the underlying data to the Java type specified in the getter method
* and returns a suitable Java value.
*
* Column names used as input to getter methods are case insensitive. When a
* getter method is called with a column name and several columns have the same
* name, the value of the first matching column will be returned. The column
* name option is designed to be used when column names are used in the SQL
* query that generated the result set. For columns that are NOT explicitly
* named in the query, it is best to use column numbers. If column names are
* used, the programmer should take care to guarantee that they uniquely refer
* to the intended columns, which can be assured with the SQL AS clause.
*
* The number, types and properties of a Results object's column are provided by
* the {@link ColumnMetaData} object returned by the {@link #getMetadata(int)}
* method.
*
* @author XDEV Software
*
*/
public abstract class Result implements AutoCloseable
{
private transient DBDataSource dataSource;
private Integer maxRowCount;
private transient QueryInfo queryInfo;
/**
* Sets the dataSource
for this {@link Result}.
*
* @param dataSource
* the {@link DBDataSource} of this {@link Result}
*/
public void setDataSource(DBDataSource dataSource)
{
this.dataSource = dataSource;
}
/**
* Gets the {@link DBDataSource} of this {@link Result}.
*
* @return the {@link DBDataSource} of this {@link Result}
*/
public DBDataSource getDataSource()
{
return dataSource;
}
/**
* Sets the queryInfo
for this {@link Result}.
*
* @param queryInfo
* the {@link QueryInfo} of this {@link Result}
*/
public void setQueryInfo(QueryInfo queryInfo)
{
this.queryInfo = queryInfo;
}
/**
* Gets the {@link QueryInfo} of this {@link Result}.
*
* @return the {@link QueryInfo} of this {@link Result}
*/
public QueryInfo getQueryInfo()
{
return queryInfo;
}
/**
* Sets the maxRowCount for this Result
instance,
* null
means not specified.
*
* @param maxRowCount
*/
public void setMaxRowCount(Integer maxRowCount)
{
this.maxRowCount = maxRowCount;
}
/**
* Returns the maxRowCount
for this {@link Result}.
*
* @return the maxRowCount for this Result
instance or
* null if none has been specified.
*/
public Integer getMaxRowCount()
{
return maxRowCount;
}
/**
* Returns the number of columns.
*
* @return the number of columns as int
value.
*/
public abstract int getColumnCount();
/**
* Returns the cached ColumnMetadata
for the column with index
* col
.
*
* Note that col
starts with 0 for the first column.
*
*
* @param col
* the index of the column whose ColumnMetadata
* shall be returned, starting at 0
* @return the cached ColumnMetadata
of the column with index
* col
*/
public abstract ColumnMetaData getMetadata(int col);
/**
*
*
*
* @return true
if there is another row to be returned,
* otherwise false
.
*
* @throws DBException
* if any error occurred.
*/
public abstract boolean next() throws DBException;
/**
* Skips over the specified count of rows.
*
* The skip method may, for a variety of reasons, end up skipping over some
* smaller number of rows, possibly 0. This may be caused by reaching the
* end of the result.
*
* @param count
* the number of rows to skip
* @return the actual number of rows skipped
* @throws DBException
* if any error occured
*/
public abstract int skip(int count) throws DBException;
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an Object
.
*
*
* @param col
* the index of the column
*
* @return a java.lang.Object
holding the column value
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs.
*/
public abstract Object getObject(int col) throws DBException;
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an Object
.
*
*
*
* @param colName
* the name of the column
*
* @return a java.lang.Object
holding the column value
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs.
*/
public Object getObject(String colName) throws DBException
{
return getObject(getColumnIndex(colName));
}
/**
* Returns the index of the column with the specified name.
*
* @param colName
* as a {@link String}
*
* @return the index for the specified column as int value
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs.
*/
public int getColumnIndex(String colName) throws DBException
{
int c = getColumnCount();
for(int i = 0; i < c; i++)
{
if(getMetadata(i).getName().equalsIgnoreCase(colName))
{
return i;
}
}
throw new DBException(dataSource,"Column '" + colName + "' not found");
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an String
.
*
*
* @param col
* the index of the column
*
* @return a {@link String} holding the column value
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs.
*
* @see #getString(int, String)
*/
public String getString(int col) throws DBException
{
return getString(col,null);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an String
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default String
*
* @return a {@link String} holding the column value, if the column value is
* null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs.
*
* @see #getObject(int)
*/
public String getString(int col, String defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
return o.toString();
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an String
.
*
*
*
* @param colName
* the name of the column
*
* @return a {@link String} holding the column value
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs.
*/
public String getString(String colName) throws DBException
{
return getString(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an String
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default String
*
* @return a {@link String} holding the column value, if the column value is
* null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs.
*
* @see #getColumnIndex(String)
* @see #getString(int, String)
*/
public String getString(String colName, String defaultValue) throws DBException
{
return getString(getColumnIndex(colName),defaultValue);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an byte
.
*
*
* @param col
* the index of the column
*
* @return a byte
holding the column value; If the column value
* is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getByte(int, byte)
*/
public byte getByte(int col) throws DBException
{
return getByte(col,(byte)0);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an byte
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default byte
*
* @return a byte
holding the column value, if the column value
* is null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getObject(int)
*/
public byte getByte(int col, byte defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
try
{
return ((Number)o).byteValue();
}
catch(ClassCastException cce)
{
throw new DBException(dataSource,cce);
}
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an byte
.
*
*
* @param colName
* the name of the column
*
* @return a byte
holding the column value; If the column value
* is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getByte(int)
*/
public byte getByte(String colName) throws DBException
{
return getByte(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an byte
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default byte
*
* @return a byte
holding the column value, if the column value
* is null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getByte(int, byte)
*/
public byte getByte(String colName, byte defaultValue) throws DBException
{
return getByte(getColumnIndex(colName),defaultValue);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an short
.
*
*
* @param col
* the index of the column
*
* @return a short
holding the column value; If the column
* value is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getShort(int, short)
*/
public short getShort(int col) throws DBException
{
return getShort(col,(short)0);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an short
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default byte
*
* @return a short
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getObject(int)
*/
public short getShort(int col, short defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
try
{
return ((Number)o).shortValue();
}
catch(ClassCastException cce)
{
throw new DBException(dataSource,cce);
}
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an short
.
*
*
* @param colName
* the name of the column
*
* @return a short
holding the column value; If the column
* value is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getShort(int)
*/
public short getShort(String colName) throws DBException
{
return getShort(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an short
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default short
*
* @return a short
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getShort(int, short)
*/
public short getShort(String colName, short defaultValue) throws DBException
{
return getShort(getColumnIndex(colName),defaultValue);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an int
.
*
*
* @param col
* the index of the column
*
* @return a int
holding the column value. If the column value
* is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getInt(int, int)
*/
public int getInt(int col) throws DBException
{
return getInt(col,0);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an int
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default int
*
* @return a int
holding the column value, if the column value
* is null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getObject(int)
*/
public int getInt(int col, int defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
try
{
return ((Number)o).intValue();
}
catch(ClassCastException cce)
{
throw new DBException(dataSource,cce);
}
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an int
.
*
*
*
* @param colName
* the name of the column
*
* @return a int
holding the column value. If the column value
* is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getInt(int)
*/
public int getInt(String colName) throws DBException
{
return getInt(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an int
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default int
*
* @return a int
holding the column value, if the column value
* is null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getInt(int, int)
*/
public int getInt(String colName, int defaultValue) throws DBException
{
return getInt(getColumnIndex(colName),defaultValue);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an long
.
*
*
* @param col
* the index of the column
*
* @return a long
holding the column value. If the column value
* is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getLong(int, long)
*/
public long getLong(int col) throws DBException
{
return getLong(col,0);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an long
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default long
*
* @return a long
holding the column value, if the column value
* is null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getObject(int)
*/
public long getLong(int col, long defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
try
{
return ((Number)o).longValue();
}
catch(ClassCastException cce)
{
throw new DBException(dataSource,cce);
}
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an long
.
*
*
*
* @param colName
* the name of the column
*
* @return a long
holding the column value. If the column value
* is null
, 0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getLong(int)
*/
public long getLong(String colName) throws DBException
{
return getLong(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an long
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default long
*
* @return a long
holding the column value, if the column value
* is null
the defaultValue
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getLong(int, long)
*/
public long getLong(String colName, long defaultValue) throws DBException
{
return getLong(getColumnIndex(colName),defaultValue);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an float
.
*
*
* @param col
* the index of the column
*
* @return a float
holding the column value. If the column
* value is null
, 0f is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getFloat(int, float)
*/
public float getFloat(int col) throws DBException
{
return getFloat(col,0f);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an float
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default float
*
* @return a float
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getObject(int)
*/
public float getFloat(int col, float defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
try
{
return ((Number)o).floatValue();
}
catch(ClassCastException cce)
{
throw new DBException(dataSource,cce);
}
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an float
.
*
*
*
* @param colName
* the name of the column
*
* @return a float
holding the column value. If the column
* value is null
, 0f is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getFloat(int)
*/
public float getFloat(String colName) throws DBException
{
return getFloat(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an float
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default float
*
* @return a float
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getFloat(int, float)
*/
public float getFloat(String colName, float defaultValue) throws DBException
{
return getFloat(getColumnIndex(colName),defaultValue);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an double
.
*
*
* @param col
* the index of the column
*
* @return a double
holding the column value. If the column
* value is null
, 0.0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getDouble(int, double)
*/
public double getDouble(int col) throws DBException
{
return getDouble(col,0.0);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an double
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default double
*
* @return a double
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getObject(int)
*/
public double getDouble(int col, double defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
try
{
return ((Number)o).doubleValue();
}
catch(ClassCastException cce)
{
throw new DBException(dataSource,cce);
}
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an double
.
*
*
*
* @param colName
* the name of the column
*
* @return a double
holding the column value. If the column
* value is null
, 0.0 is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*/
public double getDouble(String colName) throws DBException
{
return getDouble(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an double
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default double
*
* @return a double
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getDouble(int, double)
*/
public double getDouble(String colName, double defaultValue) throws DBException
{
return getDouble(getColumnIndex(colName),defaultValue);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an boolean
.
*
*
* @param col
* the index of the column
*
* @return a boolean
holding the column value. If the column
* value is null
, false
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getBoolean(int, boolean)
*/
public boolean getBoolean(int col) throws DBException
{
return getBoolean(col,false);
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an boolean
.
*
*
* @param col
* the index of the column
*
* @param defaultValue
* the default boolean
*
* @return a boolean
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getObject(int)
*/
public boolean getBoolean(int col, boolean defaultValue) throws DBException
{
Object o = getObject(col);
if(o == null)
{
return defaultValue;
}
try
{
return (Boolean)o;
}
catch(ClassCastException cce)
{
throw new DBException(dataSource,cce);
}
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an boolean
.
*
*
*
* @param colName
* the name of the column
*
* @return a boolean
holding the column value. If the column
* value is null
, false
is returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getBoolean(int)
*/
public boolean getBoolean(String colName) throws DBException
{
return getBoolean(getColumnIndex(colName));
}
/**
*
* Gets the value of the designated column in the current row of this
* {@link Result} object as an boolean
.
*
*
*
* @param colName
* the name of the column
*
* @param defaultValue
* the default boolean
*
* @return a boolean
holding the column value, if the column
* value is null
the defaultValue
is
* returned.
*
* @throws DBException
* if the columnIndex is not valid; if a database access error
* occurs or the value can not be cast.
*
* @see #getColumnIndex(String)
* @see #getBoolean(int, boolean)
*/
public boolean getBoolean(String colName, boolean defaultValue) throws DBException
{
return getBoolean(getColumnIndex(colName),defaultValue);
}
/**
* Closes this {@link Result} and releases all used resources.
*
* @throws DBException
* if a database access error occurs.
*/
public abstract void close() throws DBException;
/**
* Prints the contents of this result to {@link System#out}.
*
* @since 3.1
*/
public void dump() throws DBException
{
dump(System.out);
}
/**
* Prints the contents of this result to out
.
*
* @param out
* the stream to print to
*
* @since 3.1
*/
public void dump(PrintStream out) throws DBException
{
int columnCount = getColumnCount();
for(int col = 0; col < columnCount; col++)
{
if(col > 0)
{
out.print("\t");
}
ColumnMetaData meta = getMetadata(col);
out.print(meta.getName() + "(" + meta.getType().name() + ")");
}
out.println();
out.println();
while(next())
{
for(int col = 0; col < columnCount; col++)
{
if(col > 0)
{
out.print("\t");
}
out.print(String.valueOf(getObject(col)));
}
out.println();
}
}
}