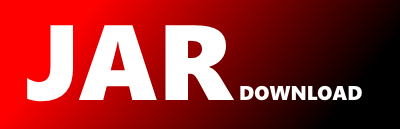
xdev.ui.FormularComponent Maven / Gradle / Ivy
/*
* XDEV Application Framework - XDEV Application Framework
* Copyright © 2003 XDEV Software (https://xdev.software)
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package xdev.ui;
import java.util.EventListener;
import java.util.Map;
import javax.swing.JComponent;
import xdev.db.Operator;
import xdev.vt.VirtualTable;
/**
* Enables a {@link JComponent} to be used as a {@link FormularComponent}. A
* {@link FormularComponent} provides methods that allows the {@link Formular} to
* set and read values from the component automatically.
*
* @param
* type of the implementing {@link JComponent}
*
* @see BooleanFormularComponent
* @see NumberFormularComponent
* @see DateFormularComponent
* @see ImageFormularComponent
* @see MasterDetailComponent
*/
public interface FormularComponent
{
/**
* Returns the name of the component in the formular context.
*
* @return the name of the component in the formular context.
*/
public String getFormularName();
/**
* Separator which is used if a data field contains more entries, e.g. a
* multi column foreign key
*
* @since 3.2
*/
public final static String DATA_FIELD_SEPARATOR = ",";
/**
* Sets the name of the bound data field of this formular component.
*
* @param dataField
* the new bound data field
* @since 3.1
*/
public void setDataField(String dataField);
/**
* Returns the name of the bound data field of this formular component.
*
* @return the name of the bound data field
* @since 3.1
*/
public String getDataField();
/**
* Sets the value of the component according to the provided value
* value
. The value is formatted using the column format of the
* provided {@link VirtualTable} and columnIndex
.
*
* @param vt
* {@link VirtualTable} to use the format from
* @param columnIndex
* of the column to use the format from
* @param value
* value to set
* @deprecated replaced by {@link #setFormularValue(VirtualTable, Map)
*/
@Deprecated
public void setFormularValue(VirtualTable vt, int columnIndex, Object value);
/**
* Sets the value of the component, taking the value(s) of the record
* according to {@link #getDataField()}.
*
* @param vt
* the underlying virtual table
* @param record
* the data <column,value>
* @since 3.2
*/
public void setFormularValue(VirtualTable vt, Map record);
/**
* Returns the value of the component.
*
* @return the value of the component.
*/
public Object getFormularValue();
/**
* Saves the state of the component internally.
*
* A saved state can be restored using {@link #restoreState()}.
*
*/
public void saveState();
/**
* Restores the internally saved state of the component.
*
* The state of the component can be saved using {@link #saveState()}.
*
*/
public void restoreState();
/**
* Checks if the component's state since the last call of
* {@link #saveState()} has changed.
*
* @return true
if the component's state has changed,
* false
otherwise
*
* @since 3.1
*/
public boolean hasStateChanged();
/**
* Registers a {@link ValueChangeListener}.
*
* @param l
* the listener to register
*/
public void addValueChangeListener(ValueChangeListener l);
/**
* Listener interface which is informed when the value changes.
*
* @see FormularComponent#addValueChangeListener(ValueChangeListener)
*/
public static interface ValueChangeListener extends EventListener
{
/**
* Invoked when the value of a {@link FormularComponent} has changed.
*/
public void valueChanged(Object eventObject);
}
/**
* Returns whether the component supports multi selection or not.
*
*
* A component that supports multi selection can have more than one
* selected item / value.
*
*
* @return true
if the component supports multi
* selection, false
otherwise.
*/
public boolean isMultiSelect();
/**
* Returns whether the component's value adheres all set constraints.
*
* This is a alternative method for {@link #validateState()}, but this
* method returns a boolean depending on the validation's result and doesn't
* throw an {@link ValidationException}.
*
* @return true
if the value of the component adheres all set
* constraints; otherwise false
.
*/
public boolean verify();
/**
* Adds a validator to this component
*
* @param validator
* the validator to add
* @see #validateState()
* @since 3.1
*/
public void addValidator(Validator validator);
/**
* Removes a validator from this component
*
* @param validator
* the validator to remove
* @see #validateState()
* @since 3.1
*/
public void removeValidator(Validator validator);
/**
* Returns all validators of this component.
*
* If no validator is present an empty array is returned.
*
* @return all validators of this component
* @since 3.1
*/
public Validator[] getValidators();
/**
* Calls {@link Validator#validate(Object)} of all registered
* {@link Validator}s.
*
* @throws ValidationException
* @see #verify
* @since 3.1
*/
public void validateState() throws ValidationException;
/**
* Calls {@link Validator#validate(Object)} of all registered
* {@link Validator}s.
*
* Every {@link ValidationException} is recorded in the
* validation
object, and if
* {@link Validation#continueValidation(ValidationException)} returns
* false
this exception is re-thrown by this method.
*
* @param validation
* the validation process object
* @throws ValidationException
* @see #verify
* @since 3.1
*/
public void validateState(Validation validation) throws ValidationException;
/**
* Returns the operator used in {@link Formular#createCondition(String)}
*
* @return the component's filter operator
* @since 3.1
*/
public Operator getFilterOperator();
/**
* Sets the filter operator used in {@link Formular#createCondition(String)}
*
* @param filterOperator
* the new filter operator
* @since 3.1
*/
public void setFilterOperator(Operator filterOperator);
/**
* Determines whether this component is only used to display values.
*
* @return true
if this component is only used to display
* values.
* @since 3.2
*/
public boolean isReadOnly();
/**
* Sets if this form component is only used to display values.
*
* @param readOnly
* true
to only display values
* @since 3.2
*/
public void setReadOnly(boolean readOnly);
/**
* Determines whether this component is visible or not.
*
* @return true
if the component is visible, false
* otherwise
*/
public boolean isVisible();
/**
* Determines whether this component is enabled or not.
*
* @return true
if the component is enabled, false
* otherwise
*/
public boolean isEnabled();
/**
* Adds an arbitrary key/value "client property" to this component.
*
* The get/putClientProperty
methods provide access to a small
* per-instance hashtable. Callers can use get/putClientProperty to annotate
* components that were created by another module. For example, a layout
* manager might store per child constraints this way. For example:
*
*
* componentA.putClientProperty("to the left of",componentB);
*
*
* If value is null
this method will remove the property.
* Changes to client properties are reported with
* PropertyChange
events. The name of the property (for the
* sake of PropertyChange events) is key.toString()
.
*
* The clientProperty
dictionary is not intended to support
* large scale extensions to form nor should be it considered an alternative
* to subclassing when designing a new component.
*
* @param key
* the new client property key
* @param value
* the new client property value; if null
this
* method will remove the property
* @see #getClientProperty
*/
public void putClientProperty(Object key, Object value);
/**
* Returns the value of the property with the specified key. Only properties
* added with putClientProperty
will return a non-
* null
value.
*
* @param key
* the being queried
* @return the value of this property or null
* @see #putClientProperty
*/
public Object getClientProperty(Object key);
}